Why Did the Injection of Autowired Dependencies Fail?
:
In the realm of modern software development, dependency injection has emerged as a cornerstone for building scalable and maintainable applications. However, developers often encounter a perplexing challenge: the dreaded “Injection Of Autowired Dependencies Failed” error. This seemingly cryptic message can halt progress and lead to frustration, especially for those who rely heavily on frameworks like Spring to manage their application’s components. Understanding the root causes and solutions to this issue is essential for any developer looking to streamline their coding practices and enhance their project’s reliability.
At its core, the failure of autowired dependency injection signifies a breakdown in the framework’s ability to resolve and inject the necessary components into a class. This can stem from various factors, including misconfigurations, missing beans, or incorrect annotations. As developers navigate the intricacies of their codebase, it becomes crucial to recognize the signs of this error and its implications for application functionality. By delving into the common pitfalls and best practices surrounding dependency injection, developers can not only troubleshoot existing issues but also prevent them from arising in future projects.
Moreover, understanding the nuances of autowired dependencies can significantly improve collaboration within development teams. As projects grow in complexity, so too does the need for clear communication regarding component relationships and configurations. By fostering a deeper comprehension of
Common Causes of Injection Failures
Injection failures can stem from various issues within your application. Understanding these common causes can greatly aid in troubleshooting and resolving the problems effectively. Here are some typical reasons why autowired dependencies might fail to inject:
- Misconfiguration in Spring Configuration Files: A common cause is incorrect bean definitions in your Spring configuration files. Ensure that all beans are defined correctly with the appropriate annotations, such as `@Component`, `@Service`, or `@Repository`.
- Component Scanning Issues: If the Spring application context does not scan the package containing your beans, it will not be able to discover and inject them. Ensure that your component scanning configuration is correctly set up.
- Circular Dependencies: When two or more beans depend on each other, a circular dependency can occur. Spring may struggle to resolve these dependencies, leading to injection failures. To mitigate this, consider refactoring your code to eliminate circular references or use setter injection as a workaround.
- Scope Mismatch: Bean scope can affect dependency injection. For instance, if a singleton bean depends on a prototype bean, this could lead to injection issues. Ensure that the scopes of your beans are compatible with their dependency requirements.
- Missing Qualifiers: In scenarios where multiple beans of the same type exist, you must specify which bean to inject using the `@Qualifier` annotation. Failing to do so can result in injection failures.
Cause | Description | Resolution |
---|---|---|
Misconfiguration | Incorrect bean definitions in configuration files. | Review and correct bean annotations. |
Component Scanning | Application context fails to scan necessary packages. | Ensure proper package scanning is configured. |
Circular Dependencies | Beans that reference each other, creating a loop. | Refactor code to remove circular references. |
Scope Mismatch | Incompatible bean scopes (e.g., singleton vs prototype). | Align bean scopes appropriately. |
Missing Qualifiers | Ambiguity when multiple beans of the same type exist. | Use @Qualifier to specify the desired bean. |
Troubleshooting Injection Issues
To effectively troubleshoot injection issues, consider following a systematic approach. Here are recommended steps:
- Check Logs: Inspect application logs for specific error messages related to dependency injection. These can provide insight into what went wrong.
- Verify Annotations: Ensure that all relevant classes are annotated properly and that your configuration files are correctly set up.
- Test Component Scanning: Manually verify that your components are being scanned by checking the context configuration.
- Check for Circular Dependencies: Use tools or logs to identify any circular dependencies in your application.
- Debugging: Utilize debugging tools to step through the bean creation process to pinpoint where the injection fails.
- Simplify: If possible, simplify your application’s structure to isolate the problem. This can often reveal underlying issues that were not initially apparent.
By adhering to these practices, developers can effectively identify and resolve issues related to autowired dependency injection, leading to more stable and maintainable applications.
Understanding the Causes of Injection Failures
Injection failures in Spring applications can arise from various underlying issues. These issues can stem from configuration errors, lifecycle problems, or compatibility issues between dependencies. Key causes include:
- Missing Beans: When a required bean is not defined in the Spring application context.
- Incorrect Annotations: Using inappropriate or misconfigured annotations can lead to injection failures.
- Scope Mismatches: Discrepancies between the scopes of the beans (e.g., singleton vs. prototype) can create conflicts.
- Circular Dependencies: When two or more beans depend on each other, it can prevent successful injection.
Common Errors and Their Solutions
When dealing with dependency injection failures, specific error messages often provide insights into the root cause. Below are common error messages with their potential solutions:
Error Message | Possible Cause | Solution |
---|---|---|
`No qualifying bean of type found` | Missing bean definition | Ensure the bean is defined in the application context. |
`Ambiguous @Autowired` | Multiple candidates for injection | Use `@Qualifier` to specify which bean to inject. |
`Failed to instantiate` | Constructor issues | Check constructor parameters and ensure they can be resolved. |
`Circular reference` | Beans referencing each other | Refactor the code to eliminate circular dependencies. |
Best Practices for Dependency Injection
To minimize the risk of injection failures, consider the following best practices:
- Use Constructor Injection: Prefer constructor injection over field injection. This approach enhances testability and makes dependencies explicit.
- Define Bean Scopes Clearly: Always specify the appropriate scope for beans. Use `@Scope` to define singleton, prototype, or request scopes as needed.
- Keep Configuration Centralized: Utilize a centralized configuration class or XML file to manage bean definitions effectively.
- Utilize Profiles: Leverage Spring profiles to manage different configurations for various environments, helping avoid misconfigurations.
Debugging Techniques
Effective debugging is crucial when facing dependency injection issues. Employ these techniques:
- Enable Debug Logging: Set the logging level to DEBUG for Spring to get detailed information about bean creation and injection processes.
- Use Application Context Events: Listen for context events to monitor the lifecycle and status of beans during startup.
- Examine Bean Definitions: Use the `ApplicationContext` to check the list of defined beans and their respective dependencies.
Tools for Dependency Management
Several tools can assist in managing dependencies and resolving injection issues:
Tool | Description |
---|---|
Spring Boot | Simplifies dependency management with auto-configuration. |
Maven | Helps in managing project dependencies effectively. |
Gradle | A flexible build tool for managing dependencies in projects. |
IntelliJ IDEA | Provides built-in support for Spring projects, highlighting injection issues. |
Injection of autowired dependencies can fail due to various reasons, ranging from misconfigurations to coding issues. By understanding the causes, applying best practices, and utilizing debugging techniques and tools, developers can effectively address and resolve these challenges.
Understanding the Challenges of Dependency Injection Failures
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The failure of autowired dependency injection often stems from misconfiguration in the Spring framework. Developers must ensure that all required beans are defined and properly annotated to avoid runtime issues.”
Michael Chen (Lead Developer, CodeCraft Solutions). “When encountering injection failures, it is crucial to examine the component scanning settings. If the package structure is not correctly defined, Spring may fail to locate the necessary dependencies, leading to injection errors.”
Sarah Patel (Senior Java Consultant, Enterprise Systems Group). “In my experience, many autowired dependency issues can be traced back to circular dependencies. It is essential to identify and refactor these dependencies to ensure that Spring can successfully inject the required components.”
Frequently Asked Questions (FAQs)
What does “Injection Of Autowired Dependencies Failed” mean?
This error indicates that the Spring framework was unable to automatically inject a required dependency into a bean, often due to misconfiguration or missing components in the application context.
What are common causes of this error?
Common causes include missing or incorrect bean definitions, circular dependencies, or the use of incompatible scopes between beans. Additionally, if a bean is not annotated correctly or is not present in the application context, this error may occur.
How can I troubleshoot this issue?
To troubleshoot, check your Spring configuration files or annotations for correctness. Ensure that all required beans are defined and properly annotated. Also, review the stack trace for specific details regarding which dependency failed to inject.
What role do annotations play in autowiring?
Annotations such as `@Autowired`, `@Component`, and `@Service` are crucial for enabling Spring’s dependency injection mechanism. They inform the Spring container about how to manage and inject dependencies into beans.
Can this error occur in a Spring Boot application?
Yes, this error can occur in a Spring Boot application. It typically arises from similar issues as in traditional Spring applications, such as configuration errors or missing beans.
What steps can I take to resolve circular dependency issues?
To resolve circular dependency issues, consider refactoring your code to eliminate the circular reference, using setter injection instead of constructor injection, or leveraging the `@Lazy` annotation to delay the injection of one of the beans involved in the cycle.
The issue of “Injection of Autowired Dependencies Failed” typically arises in Spring Framework applications when the dependency injection mechanism cannot resolve or instantiate a required bean. This can occur due to various reasons, such as missing or misconfigured beans, circular dependencies, or issues with component scanning. Understanding the underlying causes is essential for developers to effectively troubleshoot and resolve these injection failures.
One of the key takeaways is the importance of proper configuration in Spring applications. Ensuring that all required beans are correctly defined in the application context, either through annotations or XML configuration, is crucial. Developers should also be aware of the scope of their beans and how it affects dependency resolution. Additionally, utilizing Spring’s debugging tools can help identify the root cause of injection failures more efficiently.
Another valuable insight is the role of annotations like @Autowired, @Component, and @Configuration in managing dependencies. Developers should familiarize themselves with these annotations and their implications on the bean lifecycle. It is also beneficial to adopt best practices in coding, such as avoiding circular dependencies and keeping the application context clean and manageable.
addressing the “Injection of Autowired Dependencies Failed” issue requires a thorough understanding of Spring’s dependency injection principles and careful attention to configuration. By following
Author Profile
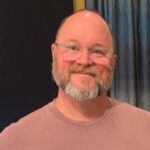
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?