How Can You Create an Interactive Menu in Python?
Creating a menu in Python can be a game-changer for your applications, whether you’re developing a simple text-based program or a more complex graphical interface. Menus serve as the backbone of user interaction, guiding users through the functionalities of your software with ease and efficiency. In a world where user experience is paramount, mastering the art of menu creation can elevate your projects from basic scripts to polished applications that users will love to navigate.
In this article, we will explore the various techniques and libraries available for crafting menus in Python. From command-line interfaces that allow users to make selections with a few keystrokes to graphical user interfaces that offer a more visually appealing experience, you’ll discover the tools and methods that suit your needs. We will also touch on best practices for menu design, ensuring that your menus are not only functional but also intuitive and user-friendly.
Whether you’re a beginner looking to enhance your programming skills or an experienced developer seeking to refine your user interface design, understanding how to create effective menus in Python is essential. Join us as we delve into the intricacies of menu creation, providing you with the knowledge and confidence to implement menus that enhance your applications and delight your users.
Using Functions to Create a Menu
Creating a menu in Python often involves using functions to handle various tasks. This modular approach allows for better code organization and easier maintenance. Below is a simple example of how to implement a menu using functions.
“`python
def view_items():
print(“Viewing items…”)
def add_item():
print(“Adding an item…”)
def delete_item():
print(“Deleting an item…”)
def exit_program():
print(“Exiting the program.”)
exit()
def display_menu():
print(“\nMenu:”)
print(“1. View Items”)
print(“2. Add Item”)
print(“3. Delete Item”)
print(“4. Exit”)
menu_options = {
‘1’: view_items,
‘2’: add_item,
‘3’: delete_item,
‘4’: exit_program
}
while True:
display_menu()
choice = input(“Select an option: “)
action = menu_options.get(choice)
if action:
action()
else:
print(“Invalid choice, please try again.”)
“`
In this example, each menu option corresponds to a specific function. The `menu_options` dictionary maps user input to function calls, providing a clean and efficient way to manage the menu’s behavior.
Using Classes for More Complex Menus
For more complex applications, utilizing classes can enhance the structure of your menu system. By encapsulating the menu functionality within a class, you can manage state and behavior more effectively. Below is a sample implementation:
“`python
class Menu:
def __init__(self):
self.menu_options = {
‘1’: self.view_items,
‘2’: self.add_item,
‘3’: self.delete_item,
‘4’: self.exit_program
}
def view_items(self):
print(“Viewing items…”)
def add_item(self):
print(“Adding an item…”)
def delete_item(self):
print(“Deleting an item…”)
def exit_program(self):
print(“Exiting the program.”)
exit()
def display_menu(self):
print(“\nMenu:”)
for key, value in self.menu_options.items():
print(f”{key}. {value.__name__.replace(‘_’, ‘ ‘).title()}”)
def run(self):
while True:
self.display_menu()
choice = input(“Select an option: “)
action = self.menu_options.get(choice)
if action:
action()
else:
print(“Invalid choice, please try again.”)
menu = Menu()
menu.run()
“`
This class-based implementation allows for easier expansion, where additional methods or attributes can be added without disrupting the overall structure.
Table Representation of Menu Options
Utilizing a table can aid in visualizing the various menu options and their corresponding functions. Below is a representation of the menu options discussed:
Option | Function |
---|---|
1 | View Items |
2 | Add Item |
3 | Delete Item |
4 | Exit Program |
This table can serve as a reference during development to ensure that all options are covered and appropriately linked to their respective functionalities.
Creating a Simple Text Menu
To create a basic text-based menu in Python, you can utilize a loop and conditional statements. This method allows users to interact with your program by selecting options.
“`python
def display_menu():
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
while True:
display_menu()
choice = input(“Please select an option: “)
if choice == ‘1’:
print(“You selected Option 1.”)
elif choice == ‘2’:
print(“You selected Option 2.”)
elif choice == ‘3’:
print(“You selected Option 3.”)
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid option, please try again.”)
“`
In this code snippet, a menu is displayed in the console, allowing the user to choose from multiple options. The loop continues until the user decides to exit by selecting the appropriate option.
Creating a Menu with Functions
To enhance modularity, you can create separate functions for each menu option. This approach promotes clearer code organization.
“`python
def option_1():
print(“You are now in Option 1.”)
def option_2():
print(“You are now in Option 2.”)
def option_3():
print(“You are now in Option 3.”)
def display_menu():
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
while True:
display_menu()
choice = input(“Please select an option: “)
if choice == ‘1’:
option_1()
elif choice == ‘2’:
option_2()
elif choice == ‘3’:
option_3()
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid option, please try again.”)
“`
This structure allows each option to execute its own function, making the code more maintainable and easier to expand.
Graphical User Interface (GUI) Menu
For a GUI application, libraries like Tkinter or PyQt can be used. Below is an example using Tkinter.
“`python
import tkinter as tk
from tkinter import messagebox
def option_selected(option):
messagebox.showinfo(“Selection”, f”You selected {option}”)
def create_menu():
root = tk.Tk()
root.title(“Menu Example”)
menu = tk.Menu(root)
root.config(menu=menu)
submenu = tk.Menu(menu)
menu.add_cascade(label=”Options”, menu=submenu)
submenu.add_command(label=”Option 1″, command=lambda: option_selected(“Option 1″))
submenu.add_command(label=”Option 2”, command=lambda: option_selected(“Option 2″))
submenu.add_command(label=”Option 3”, command=lambda: option_selected(“Option 3″))
submenu.add_separator()
submenu.add_command(label=”Exit”, command=root.quit)
root.mainloop()
create_menu()
“`
This Tkinter code creates a simple GUI with a drop-down menu. Users can select options, and a message box will display their selection.
Considerations for Menu Design
When designing a menu, consider the following best practices:
- Clarity: Ensure the options are clearly labeled and easily understandable.
- Accessibility: Make the menu easy to navigate, especially for users with disabilities.
- Error Handling: Provide feedback for invalid selections to enhance user experience.
- Scalability: Design the menu structure so it can accommodate future options without significant changes.
By implementing these practices, you can create an effective and user-friendly menu in Python.
Expert Insights on Creating Menus in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a menu in Python can significantly enhance user interaction. Utilizing libraries such as Tkinter or PyQt can provide a graphical interface that is both intuitive and visually appealing, making it easier for users to navigate applications.”
Mark Thompson (Python Developer Advocate, CodeMaster Solutions). “When designing a menu in Python, it is crucial to consider the user experience. Implementing clear labels and logical groupings of options can streamline navigation, especially in command-line interfaces. This approach not only improves usability but also enhances the overall functionality of the application.”
Linda Chen (Lead Instructor, Python Programming Academy). “For beginners, I recommend starting with simple text-based menus using functions and loops. This foundational knowledge is essential before moving on to more complex GUI frameworks. Understanding how to manage user input effectively is key to creating a responsive menu system.”
Frequently Asked Questions (FAQs)
How do I create a simple text-based menu in Python?
To create a simple text-based menu in Python, use a loop to display options and gather user input. Utilize the `input()` function to capture user choices and conditionally execute corresponding actions based on the input.
What libraries can I use to enhance menus in Python?
You can use libraries such as `curses` for terminal-based interfaces, `tkinter` for GUI applications, or `PyQt` for more advanced graphical menus. Each library provides tools to create interactive and visually appealing menus.
Can I create a menu that handles invalid inputs in Python?
Yes, you can implement input validation by checking user input against expected values. Use `try-except` blocks or conditional statements to prompt the user again if the input is invalid, ensuring a robust user experience.
How can I create a menu with submenus in Python?
To create a menu with submenus, define separate functions for each menu and call them based on user selections. This modular approach allows for cleaner code and easier management of complex menu structures.
Is it possible to save menu selections in Python?
Yes, you can save menu selections by writing them to a file or storing them in a database. Use Python’s built-in file handling capabilities or libraries like `sqlite3` to persist user choices for future sessions.
How can I make my Python menu more user-friendly?
To enhance user-friendliness, ensure clear instructions, maintain consistent formatting, and provide feedback for each action. Additionally, consider implementing keyboard shortcuts and help options to guide users through the menu.
Creating a menu in Python is a fundamental skill that enhances user interaction within applications. By utilizing various libraries such as Tkinter for graphical user interfaces or simply employing console-based menus, developers can design intuitive and user-friendly navigation systems. The process typically involves defining menu options, capturing user input, and executing corresponding actions based on that input.
Key takeaways include understanding the importance of structuring the menu logically to improve usability. A well-designed menu should prioritize clarity and accessibility, allowing users to navigate seamlessly. Additionally, implementing error handling ensures that the program remains robust, even when users make unexpected choices. This attention to detail not only enhances user experience but also contributes to the overall quality of the application.
In summary, mastering the creation of menus in Python is essential for developing effective applications. By focusing on user experience, leveraging appropriate libraries, and ensuring robust error handling, developers can create menus that significantly enhance the functionality and appeal of their programs. As you continue to explore Python, consider experimenting with different menu designs to find the best fit for your specific applications.
Author Profile
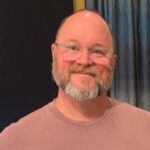
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?