How Can You Pass a Path to a Function in TypeScript?
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful tool that enhances JavaScript with static typing and robust tooling. As developers strive for cleaner, more maintainable code, understanding how to effectively manage paths within TypeScript functions becomes crucial. Whether you’re navigating file systems, API endpoints, or complex data structures, the ability to pass paths to functions not only streamlines your code but also enhances its readability and functionality. This article will delve into the nuances of passing paths in TypeScript, equipping you with the knowledge to optimize your development process.
When working with TypeScript, the concept of passing paths to functions can take various forms, depending on the context of your application. From defining file paths in Node.js applications to managing routes in web frameworks, the approach can differ significantly. Understanding how to handle these paths effectively can lead to better organization of your code and fewer runtime errors. Moreover, TypeScript’s type system provides a safety net that helps ensure the paths you work with are valid and correctly formatted, reducing the likelihood of bugs.
As we explore this topic, we’ll cover the different scenarios where passing paths is essential, the best practices for defining and using these paths, and how TypeScript’s features can enhance your coding experience. By the
Understanding File Paths in TypeScript
In TypeScript, managing file paths effectively is crucial for organizing and accessing modules, especially in larger applications. The paths can be absolute or relative, depending on how you reference the files in your project structure.
- Absolute Paths: These specify the complete path from the root of the project. They are useful for avoiding path traversal issues, particularly in complex folder structures.
- Relative Paths: These are based on the location of the file you are currently in. They start with `./` for the current directory or `../` for the parent directory.
Utilizing the correct type of path can streamline your import statements and reduce errors related to module resolution.
Passing Path as a Parameter to Functions
In TypeScript, you can define functions that accept file paths as parameters. This is particularly useful when working with file systems, APIs, or dynamic module loading. The function can then use the provided path to perform various operations, such as reading a file or importing a module dynamically.
Here’s a basic example of a function that takes a path as a parameter:
“`typescript
function readFile(path: string): void {
// Logic to read the file at the specified path
console.log(`Reading file from: ${path}`);
}
“`
When calling this function, you can pass either an absolute or relative path:
“`typescript
readFile(‘./data/file.txt’);
readFile(‘/usr/local/data/file.txt’);
“`
Using TypeScript’s `import` Functionality with Paths
TypeScript supports dynamic imports, allowing you to load modules at runtime based on the path you provide. This is particularly handy when implementing code-splitting in applications. The syntax for a dynamic import looks like this:
“`typescript
async function loadModule(modulePath: string) {
const module = await import(modulePath);
module.default();
}
“`
This function will load the module specified by the `modulePath`. You can pass either a string literal or a variable containing the path.
Best Practices for Handling Paths in TypeScript
When working with paths in TypeScript, following best practices can enhance code maintainability and reduce errors. Consider the following guidelines:
- Use Constants for Paths: Define constants for commonly used paths to avoid hardcoding strings throughout your application.
- Leverage Path Libraries: Utilize libraries like `path` in Node.js to handle and normalize paths. This ensures compatibility across different operating systems.
- Type Safety: Use TypeScript’s type system to ensure that paths passed to functions are valid strings, reducing runtime errors.
Here’s a comparison of using hardcoded paths versus constants:
Approach | Code Example | Advantages |
---|---|---|
Hardcoded Paths | readFile(‘./data/file.txt’); | Simple but less maintainable. |
Constants for Paths | const DATA_PATH = ‘./data/file.txt’; readFile(DATA_PATH); |
Improves maintainability and clarity. |
By adhering to these practices, developers can improve the robustness and clarity of their TypeScript code, especially when dealing with file paths and dynamic imports.
Defining a Function that Accepts a Path
In TypeScript, when you want to define a function that accepts a file path or a directory path, you can create a function with a parameter typed as a `string`. This approach allows you to leverage TypeScript’s type checking while passing paths effectively.
“`typescript
function processFilePath(path: string): void {
console.log(`Processing file at: ${path}`);
}
“`
This function simply logs the path provided. You can further extend its functionality as needed.
Using Type Aliases for Path Types
To enhance readability and maintainability, you can define a type alias for paths. This can make your code clearer, especially when dealing with various types of paths.
“`typescript
type FilePath = string;
function readFile(path: FilePath): void {
console.log(`Reading file from: ${path}`);
}
“`
This method allows you to specify that only file paths will be used throughout your code.
Handling Relative and Absolute Paths
When working with paths, differentiating between relative and absolute paths is crucial. You can implement a function that checks the type of path before proceeding.
“`typescript
function isAbsolutePath(path: string): boolean {
return path.startsWith(‘/’) || /^[A-Za-z]:\\/.test(path);
}
function handlePath(path: string): void {
if (isAbsolutePath(path)) {
console.log(`Absolute path detected: ${path}`);
} else {
console.log(`Relative path detected: ${path}`);
}
}
“`
This function utilizes a helper to determine the type of path and allows for appropriate handling based on the result.
Working with Path Libraries
TypeScript can seamlessly integrate with Node.js path utilities, which provide advanced functionalities for path manipulation. Using the `path` module can simplify many operations.
“`typescript
import * as path from ‘path’;
function getFileName(filePath: string): string {
return path.basename(filePath);
}
console.log(getFileName(‘/user/files/document.txt’)); // Output: document.txt
“`
Utilizing the `path` module ensures that your path manipulations are cross-platform compatible.
Asynchronous Path Handling
For applications that require asynchronous operations when working with files, you can utilize async functions. TypeScript supports async/await syntax, which can enhance readability when dealing with file paths.
“`typescript
import { promises as fs } from ‘fs’;
async function readFileAsync(filePath: string): Promise
try {
const data = await fs.readFile(filePath, ‘utf-8’);
console.log(`File data: ${data}`);
} catch (error) {
console.error(`Error reading file: ${error}`);
}
}
“`
This function reads the file content asynchronously and handles errors gracefully, demonstrating effective path handling in asynchronous operations.
Using Generics for Path Flexibility
Generics can be employed to create functions that handle various path types, including URLs or other string-based identifiers.
“`typescript
function logPath
console.log(`Path: ${path}`);
}
logPath(‘/user/data’); // Works with string paths
logPath(‘https://example.com’); // Also works with URLs
“`
This function utilizes a generic type `T`, allowing it to accept different string formats while maintaining type safety.
Expert Insights on Passing Paths to Functions in TypeScript
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “In TypeScript, passing paths to functions can significantly enhance code modularity and reusability. By using generics and type definitions, developers can ensure that the paths are strongly typed, reducing runtime errors and improving maintainability.”
Michael Torres (Lead Developer, CodeCraft Solutions). “Utilizing TypeScript’s advanced type system when passing paths to functions allows for better type inference and autocompletion in IDEs. This not only streamlines the development process but also aids in catching potential issues early on, ultimately leading to more robust applications.”
Sarah Patel (Technical Architect, FutureTech Labs). “When designing functions that accept paths in TypeScript, it’s crucial to consider the flexibility of the input types. Implementing union types can provide the necessary versatility while still enforcing type safety, which is a core advantage of using TypeScript in complex applications.”
Frequently Asked Questions (FAQs)
What is the purpose of passing a path to a function in TypeScript?
Passing a path to a function in TypeScript allows for dynamic file or directory handling, enabling the function to operate on various files based on the given path. This enhances code reusability and flexibility.
How do you define a function that accepts a file path in TypeScript?
You can define a function that accepts a file path by specifying the parameter type as `string`. For example: `function readFile(path: string): void { /* implementation */ }`.
Can TypeScript validate the file path format?
TypeScript itself does not validate file paths at runtime. However, you can implement custom validation logic within your function to check if the path adheres to specific criteria or formats.
Is it possible to use TypeScript with Node.js for file path operations?
Yes, TypeScript can be used with Node.js for file path operations. The `fs` module in Node.js allows you to work with the file system, and TypeScript provides type safety for path handling.
What are some common libraries for handling file paths in TypeScript?
Common libraries for handling file paths in TypeScript include `path`, which is built into Node.js, and third-party libraries like `fs-extra` that provide additional file system methods and utilities.
How can you ensure type safety when passing paths to functions in TypeScript?
To ensure type safety, you can create a custom type or interface for paths, or use template literal types for specific path formats. This helps catch errors at compile time rather than runtime.
In TypeScript, passing a path to a function is a common practice that enhances the flexibility and reusability of code. By defining functions that accept paths as parameters, developers can create more dynamic applications that can handle various file structures or URL endpoints. This approach allows for better abstraction, enabling functions to operate on different data sources without being tightly coupled to specific implementations.
One of the key advantages of using TypeScript for this purpose is its strong typing system. By leveraging TypeScript’s type annotations, developers can ensure that the paths being passed to functions are valid and conform to expected formats. This reduces runtime errors and improves code maintainability, as the compiler can catch potential issues during development rather than at runtime.
Additionally, using path parameters can facilitate better testing and debugging practices. Functions that accept paths can be easily tested with different inputs, allowing for comprehensive coverage of various scenarios. This modular approach not only enhances code quality but also promotes a more organized structure, making it easier for teams to collaborate and manage large codebases.
passing paths to functions in TypeScript is a powerful technique that promotes code flexibility, enhances type safety, and improves maintainability. By adopting this practice, developers can create robust applications that
Author Profile
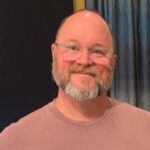
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?