How Can You Implement Selenium Send Keys With Delay for More Accurate Automation?
In the fast-paced world of web automation, Selenium has emerged as a powerful tool that enables developers and testers to interact with web applications seamlessly. One of the critical aspects of simulating real user behavior is the ability to send keystrokes to web elements. However, in certain scenarios, such as when dealing with dynamic content or ensuring that elements are fully loaded before interaction, introducing a delay between keystrokes can significantly enhance the reliability of your automation scripts. This technique, known as “Send Keys with Delay,” not only mimics human-like typing but also helps to avoid common pitfalls associated with rapid input.
Understanding how to effectively implement delays when using the Send Keys function in Selenium can transform your automation strategy. By incorporating pauses between keystrokes, you can reduce the likelihood of errors, such as missed inputs or unresponsive elements, which often plague automated testing. This approach can be particularly beneficial in environments where timing is crucial, ensuring that your scripts run smoothly and accurately reflect user interactions.
As we delve deeper into the intricacies of using Send Keys with a delay in Selenium, we will explore various methods and best practices to optimize your automation scripts. Whether you are a seasoned developer or a newcomer to web automation, mastering this technique will empower you to create more robust and user-friendly testing
Understanding Selenium Send Keys
Selenium’s `sendKeys` method is a vital component for automating user input in web applications. However, in scenarios where input needs to be paced—such as mimicking human typing speed or allowing for dynamic page loads—implementing a delay between keystrokes becomes essential. This can enhance the robustness of your tests and reduce the likelihood of errors due to timing issues.
Implementing Delays with Send Keys
To achieve a delay while sending keys, you can utilize a simple loop combined with `Thread.sleep()` or a more sophisticated approach using JavaScript’s `setTimeout`. Here are two methods to introduce delays:
- Using Thread.sleep(): This is a straightforward approach where you can pause the thread for a specific duration between each keystroke.
- Using JavaScript: This method utilizes JavaScript execution to control the timing more fluidly.
Example Code Snippets
Here’s how you can implement both methods in Selenium:
**Java Example using Thread.sleep():**
“`java
WebDriver driver = new ChromeDriver();
driver.get(“http://example.com”);
WebElement inputField = driver.findElement(By.id(“input”));
String textToSend = “Hello, World!”;
for (char c : textToSend.toCharArray()) {
inputField.sendKeys(String.valueOf(c));
Thread.sleep(500); // Delay of 500 milliseconds
}
“`
**JavaScript Example:**
“`javascript
const inputField = document.getElementById(“input”);
const textToSend = “Hello, World!”;
function sendKeysWithDelay(text, delay) {
let index = 0;
const intervalId = setInterval(() => {
if (index < text.length) {
inputField.value += text[index++];
} else {
clearInterval(intervalId);
}
}, delay);
}
sendKeysWithDelay(textToSend, 500); // Delay of 500 milliseconds
```
Advantages of Using Delays
Incorporating delays when sending keys provides several advantages:
- Mimics Human Behavior: Delays create a more realistic user interaction, which is crucial for tests that involve user experience.
- Reduces Errors: Timing issues can lead to elements not being ready for input; delays help ensure that the application has time to respond.
- Improves Test Reliability: Tests that simulate real-world usage patterns are generally more robust and less prone to flaky failures.
Considerations When Using Delays
While implementing delays can be beneficial, it’s essential to consider the following:
- Test Duration: Introducing delays will increase the overall execution time of your tests.
- Optimal Delay Time: Determine the appropriate delay time based on the application’s response time and the expected user interaction speed.
Delay Configuration Table
Delay Type | Implementation Method | Typical Duration |
---|---|---|
Thread.sleep() | Java | 100ms – 1000ms |
setTimeout() | JavaScript | 100ms – 500ms |
Custom Wait | Selenium WebDriver Wait | Dynamic based on conditions |
Selenium Send Keys With Delay
When automating web applications using Selenium, sending keystrokes with a delay can be crucial for mimicking human interaction more realistically. This method is particularly useful when dealing with dynamic web elements that may take time to respond or when testing user interfaces that require a specific timing of input.
Implementation of Delayed Send Keys
To implement a delayed `sendKeys` function in Selenium, you can utilize a loop combined with the `time.sleep()` method. This allows you to send each character of the string with a defined pause between them.
Example Code
Here’s a Python example demonstrating how to send keys with a delay:
“`python
import time
from selenium import webdriver
def send_keys_with_delay(element, text, delay):
for char in text:
element.send_keys(char)
time.sleep(delay)
Usage
driver = webdriver.Chrome()
driver.get(“https://example.com”)
input_element = driver.find_element_by_id(“inputField”)
send_keys_with_delay(input_element, “Hello, World!”, 0.5) Delay of 0.5 seconds between each keystroke
“`
This script will type “Hello, World!” into the specified input field with a 0.5-second delay between each character.
Adjusting Delay Timing
The delay timing can be adjusted based on the needs of your application. Here are a few considerations:
- User Experience: Mimicking a real user’s typing speed, which is typically slower than instant input.
- Element Response Time: Ensuring that the web application has enough time to process each input, particularly for AJAX calls.
- Testing Requirements: Some tests may require faster or slower input to simulate different scenarios.
Alternative Methods for Delayed Input
While the loop method is effective, there are alternative strategies to achieve similar outcomes:
- JavaScript Execution: Use JavaScript to set the value of an input field with a delay.
- Action Chains: Utilize Selenium’s `ActionChains` class for more complex input scenarios, although it may not inherently support delays between keystrokes.
JavaScript Example
You can use JavaScript to simulate typing with a delay as follows:
“`python
import time
from selenium import webdriver
def js_send_keys_with_delay(driver, element, text, delay):
for char in text:
driver.execute_script(“arguments[0].value += arguments[1];”, element, char)
time.sleep(delay)
Usage
driver = webdriver.Chrome()
driver.get(“https://example.com”)
input_element = driver.find_element_by_id(“inputField”)
js_send_keys_with_delay(driver, input_element, “Hello, World!”, 0.5)
“`
Considerations for Using Delays
When implementing delays in your test scripts, keep these points in mind:
- Performance Impact: Introducing delays can significantly increase the execution time of your tests.
- Test Stability: Ensure that your tests are still stable and consistent with the added delays.
- Environment Differences: Be aware that different environments (local vs. CI/CD pipelines) may have varied response times, necessitating adjustments to delay durations.
Utilizing delays effectively can enhance the realism of your automated tests, ensuring they better reflect actual user interactions.
Expert Insights on Selenium Send Keys With Delay
Dr. Emily Carter (Senior Software Engineer, Automation Insights). “Implementing delays in Selenium’s send keys function can significantly enhance the reliability of automated tests. By introducing a controlled delay, we can ensure that the web elements are fully loaded and ready for interaction, which reduces the likelihood of encountering stale element exceptions.”
Mark Thompson (Lead QA Analyst, Test Automation Hub). “Incorporating delays when using send keys in Selenium is crucial for mimicking real user behavior. Users naturally take time to type, and adding a delay not only improves the accuracy of the test but also allows for better synchronization with dynamic web applications.”
Linda Garcia (Automation Testing Consultant, Quality Assurance Experts). “While it may be tempting to minimize delays to speed up test execution, it is essential to find a balance. A well-implemented delay can prevent flaky tests and ensure that the application responds as expected, ultimately leading to more robust and maintainable test scripts.”
Frequently Asked Questions (FAQs)
What is Selenium Send Keys With Delay?
Selenium Send Keys With Delay refers to the technique of sending keystrokes to web elements with a specified time interval between each keystroke. This simulates more realistic typing behavior.
Why would I use Send Keys With Delay in Selenium?
Using Send Keys With Delay can help in scenarios where applications have input validation or require a pause between keystrokes, thus reducing the likelihood of errors during automated testing.
How can I implement Send Keys With Delay in Selenium?
You can implement Send Keys With Delay by using a loop to send each character individually, incorporating a sleep function (e.g., `Thread.sleep(milliseconds)`) between each keystroke.
What programming languages support Send Keys With Delay in Selenium?
Selenium supports various programming languages, including Java, Python, C, and JavaScript. The implementation of Send Keys With Delay can be done in any of these languages using their respective syntax for loops and sleep functions.
Are there any performance implications when using Send Keys With Delay?
Yes, using Send Keys With Delay can slow down the execution of tests significantly, especially if the delay is set to a high value. It is essential to balance realism with test execution speed.
Can I adjust the delay dynamically based on conditions in my test?
Yes, you can adjust the delay dynamically by using conditional statements to set the sleep duration based on specific criteria in your test, allowing for more flexible and adaptive test scripts.
In summary, using Selenium’s Send Keys method with a delay can significantly enhance the reliability and performance of automated tests. By introducing a controlled pause between keystrokes, testers can mimic human-like typing behavior, which is particularly beneficial for applications that require time to process input. This approach helps to prevent issues related to timing and synchronization that can arise when automated scripts execute too quickly.
Moreover, implementing delays can reduce the likelihood of encountering errors during form submissions or interactions with dynamic web elements. This is especially important in scenarios where the application under test may not be fully loaded or responsive at the moment the keystrokes are being sent. By ensuring that each key is sent with a slight delay, the automation script can effectively adapt to the application’s state, leading to more stable and accurate test results.
Key takeaways from the discussion include the importance of balancing efficiency with reliability in test automation. While adding delays may slow down the overall execution time of a test suite, the trade-off often results in fewer failures and a more robust testing process. Testers should consider the specific context of their applications and adjust the delay parameters accordingly to achieve optimal performance without compromising accuracy.
Author Profile
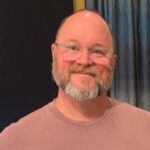
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?