How Can You Use PowerShell to Create a Folder Only If It Doesn’t Exist?
In the realm of system administration and automation, PowerShell stands out as a powerful tool for managing and manipulating files and directories. One common task that many administrators encounter is the need to create folders within the file system. However, doing so without inadvertently overwriting existing directories can be a challenge. This is where the concept of creating a folder only if it does not already exist becomes crucial. Mastering this skill not only enhances efficiency but also helps prevent errors that could disrupt workflows.
Creating a folder in PowerShell is a straightforward process, but it requires a keen understanding of how to check for existing directories. By leveraging built-in commands and conditional statements, users can ensure that their scripts are both robust and reliable. This approach not only streamlines file management but also empowers users to automate repetitive tasks with confidence.
As we delve deeper into the intricacies of PowerShell scripting, we will explore various methods to implement this functionality. From simple one-liners to more complex scripts, you’ll learn how to harness the full potential of PowerShell to create folders seamlessly and efficiently. Whether you are a seasoned administrator or a newcomer to the world of scripting, understanding how to create folders conditionally will be an invaluable addition to your toolkit.
Checking Folder Existence
To create a folder in PowerShell only if it does not already exist, the first step is to check for the folder’s presence. You can accomplish this using the `Test-Path` cmdlet, which returns a Boolean value indicating whether the specified path exists.
For example, the following command checks for the existence of a folder:
powershell
$folderPath = “C:\ExampleFolder”
$exists = Test-Path $folderPath
In this snippet, `$exists` will be `$true` if the folder exists and `$` if it does not. This check is crucial to ensure that you do not attempt to create a folder that is already present, which could lead to unnecessary errors or redundancy.
Creating the Folder
Once you have verified that the folder does not exist, you can create it using the `New-Item` cmdlet. This cmdlet allows you to specify the type of item to create, which in this case will be a directory.
The complete command to create the folder if it does not exist would look like this:
powershell
if (-not $exists) {
New-Item -Path $folderPath -ItemType Directory
}
This code snippet checks the value of `$exists` and creates the folder only if it is `$`.
Example Script
Here is a complete script that incorporates both checking for the folder’s existence and creating it if necessary:
powershell
$folderPath = “C:\ExampleFolder”
if (-not (Test-Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory
Write-Host “Folder created at $folderPath”
} else {
Write-Host “Folder already exists at $folderPath”
}
This script provides feedback on whether the folder was created or if it already exists, enhancing the user experience.
Error Handling
When working with file system operations, it is essential to include error handling to manage any unexpected issues that may arise. PowerShell provides the `try` and `catch` blocks to handle exceptions gracefully.
Here’s an example of how to implement error handling in the folder creation process:
powershell
try {
if (-not (Test-Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory -ErrorAction Stop
Write-Host “Folder created at $folderPath”
} else {
Write-Host “Folder already exists at $folderPath”
}
} catch {
Write-Host “An error occurred: $_”
}
In this snippet, the `-ErrorAction Stop` parameter ensures that any error during the folder creation will trigger the `catch` block, allowing you to handle it appropriately.
Best Practices
When creating folders in PowerShell, consider the following best practices:
- Use Full Paths: Always use absolute paths to avoid confusion with relative paths.
- Check Permissions: Ensure that your script has the necessary permissions to create folders in the target location.
- Log Actions: Maintain a log of folder creation actions for auditing and troubleshooting purposes.
- Avoid Hardcoding Paths: Use variables or configuration files to make your scripts more flexible.
Best Practice | Description |
---|---|
Use Full Paths | Always specify the full path to prevent ambiguity. |
Check Permissions | Ensure appropriate permissions are in place before execution. |
Log Actions | Keep a record of operations for easier debugging. |
Avoid Hardcoding Paths | Utilize variables for better script adaptability. |
Using PowerShell to Create a Folder if It Does Not Exist
PowerShell provides a straightforward way to manage files and folders. The `New-Item` cmdlet can be utilized to create a folder, but it is essential to check for its existence beforehand to avoid errors. Below are the steps and examples to accomplish this task effectively.
Checking Folder Existence
Before creating a folder, use the `Test-Path` cmdlet to determine if the folder already exists. This cmdlet returns a Boolean value indicating the existence of the specified path.
powershell
$folderPath = “C:\Example\NewFolder”
$exists = Test-Path $folderPath
if (-not $exists) {
New-Item -Path $folderPath -ItemType Directory
}
In this example:
- `$folderPath` is the path where you wish to create the new folder.
- `$exists` checks if the folder already exists.
- The `New-Item` cmdlet creates the folder only if it does not exist.
Creating a Function for Reusability
For enhanced efficiency, you can encapsulate the logic within a function. This allows you to reuse the code as needed without repeating yourself.
powershell
function Create-FolderIfNotExists {
param (
[string]$folderPath
)
if (-not (Test-Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory
Write-Host “Folder created: $folderPath”
} else {
Write-Host “Folder already exists: $folderPath”
}
}
# Usage
Create-FolderIfNotExists -folderPath “C:\Example\NewFolder”
This function:
- Accepts a parameter for the folder path.
- Utilizes `Test-Path` to check for existence.
- Creates the folder if it does not exist and provides feedback.
Handling Errors
While the above script works in most scenarios, incorporating error handling can enhance robustness, especially when dealing with permissions or invalid paths.
powershell
function Create-FolderIfNotExists {
param (
[string]$folderPath
)
try {
if (-not (Test-Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory -ErrorAction Stop
Write-Host “Folder created: $folderPath”
} else {
Write-Host “Folder already exists: $folderPath”
}
} catch {
Write-Host “Error creating folder: $_”
}
}
# Usage
Create-FolderIfNotExists -folderPath “C:\Example\NewFolder”
In this version:
- A `try-catch` block captures any errors during folder creation.
- The `-ErrorAction Stop` parameter ensures that the cmdlet generates an error that can be caught by the `catch` block.
Example of Creating Multiple Folders
If you need to create multiple folders, you can enhance your function to accept an array of folder paths.
powershell
function Create-MultipleFoldersIfNotExist {
param (
[string[]]$folderPaths
)
foreach ($folderPath in $folderPaths) {
Create-FolderIfNotExists -folderPath $folderPath
}
}
# Usage
$foldersToCreate = @(“C:\Example\Folder1”, “C:\Example\Folder2”, “C:\Example\Folder3”)
Create-MultipleFoldersIfNotExist -folderPaths $foldersToCreate
This approach:
- Iterates through each folder path.
- Calls the `Create-FolderIfNotExists` function for each path.
- Allows for bulk folder creation with minimal code duplication.
Implementing these PowerShell scripts enables efficient management of folder creation while maintaining system integrity by ensuring folders are not duplicated.
Expert Insights on Using PowerShell to Create Folders
Jessica Lin (Senior Systems Administrator, Tech Solutions Inc.). “Using PowerShell to create a folder only if it does not exist is a best practice for system administrators. It prevents unnecessary errors in scripts and maintains a clean directory structure. The command ‘New-Item -ItemType Directory -Path “C:\YourFolder” -Force’ is particularly effective, as it ensures that the folder is created without overwriting existing data.”
Michael Tran (DevOps Engineer, Cloud Innovators). “Incorporating conditional checks in your PowerShell scripts can significantly enhance automation workflows. The ‘if (-not (Test-Path “C:\YourFolder”)) { New-Item -ItemType Directory -Path “C:\YourFolder” }’ command is a robust method to ensure that folders are only created when needed, thus optimizing resource usage and script efficiency.”
Linda Garcia (IT Consultant, Future Tech Advisors). “PowerShell provides a powerful way to manage file systems, and creating folders conditionally is a common requirement. I recommend using the ‘Try-Catch’ block along with ‘Test-Path’ to handle exceptions gracefully. This approach not only creates folders but also improves error handling in your scripts, making them more reliable.”
Frequently Asked Questions (FAQs)
How can I create a folder in PowerShell if it does not already exist?
You can use the `New-Item` cmdlet with the `-Force` parameter. The command is: `New-Item -Path “C:\Path\To\Folder” -ItemType Directory -Force`. This will create the folder if it does not exist without throwing an error if it does.
What is the syntax for checking if a folder exists in PowerShell?
Use the `Test-Path` cmdlet to check for the existence of a folder. The syntax is: `Test-Path “C:\Path\To\Folder”`. This command returns `True` if the folder exists and “ otherwise.
Can I create multiple folders at once using PowerShell?
Yes, you can create multiple folders by using a loop or by providing an array of paths. For example:
powershell
$folders = “C:\Folder1”, “C:\Folder2”, “C:\Folder3”
foreach ($folder in $folders) {
if (-not (Test-Path $folder)) {
New-Item -Path $folder -ItemType Directory
}
}
Is there a one-liner command to create a folder if it does not exist?
Yes, you can use a one-liner command: `if (-not (Test-Path “C:\Path\To\Folder”)) { New-Item -Path “C:\Path\To\Folder” -ItemType Directory }`. This checks for existence and creates the folder if necessary.
What happens if I try to create a folder that already exists in PowerShell?
If you use `New-Item` without the `-Force` parameter on an existing folder, PowerShell will throw an error indicating that the item already exists. Using `-Force` suppresses this error.
Can I specify permissions when creating a folder in PowerShell?
PowerShell does not directly allow setting permissions during folder creation with `New-Item`. However, you can set permissions afterward using the `Set-Acl` cmdlet to modify the access control list of the newly created folder.
In summary, the process of creating a folder in PowerShell if it does not already exist is a straightforward yet essential task for system administrators and users alike. Utilizing the `New-Item` cmdlet in conjunction with the `Test-Path` cmdlet allows for efficient folder management by ensuring that duplicate folders are not created. This approach not only streamlines workflows but also helps maintain an organized file structure.
Key insights from the discussion highlight the importance of error handling and validation when working with file systems. Incorporating conditional statements can enhance scripts by providing feedback or logging actions taken, which is particularly useful in automated scripts. Furthermore, understanding the syntax and parameters of PowerShell commands can significantly improve productivity and reduce the likelihood of errors.
Ultimately, mastering the ability to create folders conditionally in PowerShell is a valuable skill that contributes to better system management and automation. By leveraging these techniques, users can ensure their environments remain tidy and efficient, paving the way for more complex scripting tasks in the future.
Author Profile
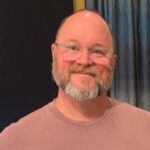
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?