Why Am I Seeing AxiosError: Request Failed With Status Code 400?
In the fast-paced world of web development, where seamless communication between the client and server is paramount, encountering errors can be a frustrating experience. One common issue that developers often face is the dreaded `AxiosError: Request Failed With Status Code 400`. This error, signaling a bad request, can halt progress and leave developers scratching their heads. Understanding the nuances of this error is crucial for anyone looking to build robust applications that rely on API interactions. In this article, we will delve into the intricacies of the 400 status code, exploring its causes, implications, and effective strategies for resolution.
When working with Axios, a popular JavaScript library for making HTTP requests, a status code of 400 indicates that the server could not process the request due to client-side errors. This could stem from various issues, such as malformed request syntax, invalid request parameters, or even missing required fields. As developers, it’s essential to grasp the underlying reasons for this error to ensure smooth communication with APIs and enhance user experience.
Moreover, addressing a 400 error goes beyond merely fixing the immediate issue; it also involves understanding best practices in API design and error handling. By familiarizing ourselves with the common pitfalls that lead to this error, we can not only troubleshoot effectively but also
Understanding Axios and HTTP Status Codes
Axios is a popular JavaScript library used for making HTTP requests from Node.js or the browser. It simplifies the process of handling requests and responses, allowing developers to work with both promises and async/await syntax. One common issue developers encounter while using Axios is the `AxiosError: Request Failed With Status Code 400` error, which indicates a bad request.
A status code of 400 is part of the HTTP response codes and typically signifies that the server cannot process the request due to a client error. This could be due to various reasons, such as malformed request syntax, invalid request message framing, or deceptive request routing.
Common Causes of AxiosError: Request Failed With Status Code 400
When facing a 400 status error with Axios, developers should consider the following potential causes:
- Incorrect URL: The endpoint might be mistyped or incorrectly formatted.
- Missing Required Parameters: The API might expect certain parameters that were not included in the request.
- Invalid Parameter Values: The values provided for parameters may not meet the API’s validation criteria.
- Content-Type Issues: The request may not specify the correct `Content-Type` header required by the server.
- Authentication Issues: Missing or invalid authentication tokens can also lead to a 400 error.
How to Debug Axios 400 Errors
To effectively debug an Axios 400 error, follow these steps:
- **Check the Request URL**: Ensure that the URL is correct and accessible.
- **Inspect Request Payload**: Verify that all required parameters are included and correctly formatted.
- **Review API Documentation**: Cross-reference your request against the API documentation to ensure compliance with its requirements.
- **Examine Headers**: Confirm that the headers sent with the request are appropriate for the API endpoint.
- **Log Error Details**: Use Axios error handling to log the complete error response for further analysis.
Example of how to log Axios errors:
“`javascript
axios.post(‘/api/endpoint’, data)
.then(response => {
console.log(response.data);
})
.catch(error => {
if (error.response) {
console.error(‘Error:’, error.response.data);
console.error(‘Status Code:’, error.response.status);
console.error(‘Headers:’, error.response.headers);
} else {
console.error(‘Error:’, error.message);
}
});
“`
Best Practices for Avoiding 400 Errors
To minimize the occurrence of 400 errors when using Axios, consider implementing the following best practices:
- Validate Input Data: Implement client-side validation to ensure that all input data conforms to expected formats before sending requests.
- Use Try-Catch Blocks: Encapsulate your Axios requests within try-catch blocks to handle errors more gracefully.
- Utilize API Client Libraries: Where available, use official API client libraries that abstract away the intricacies of crafting requests, reducing the likelihood of errors.
Error Handling Strategy
An effective error handling strategy can improve user experience and aid in debugging:
Error Type | Recommended Action |
---|---|
Bad Request (400) | Log error details and inform the user of invalid input. |
Unauthorized (401) | Redirect to login or prompt for credentials. |
Not Found (404) | Display a user-friendly message indicating the resource could not be found. |
Internal Server Error (500) | Log the error for investigation and display a generic error message. |
By following these guidelines, developers can effectively mitigate and resolve issues related to Axios 400 errors, ensuring a smoother user experience and more reliable application performance.
Understanding the 400 Status Code
The 400 status code, commonly referred to as “Bad Request,” indicates that the server cannot process the request due to a client error. This can occur for several reasons, and understanding these reasons is crucial for effective debugging.
- Common Causes:
- Invalid syntax in the request.
- Missing required parameters or headers.
- Incorrect data types or formats.
- Exceeding the request size limits.
Identifying the exact cause requires examining the request closely, including the request URL, headers, and body.
Diagnosing Axios Errors
When using Axios, a popular JavaScript library for making HTTP requests, a 400 error can manifest as an `AxiosError`. This error contains valuable information that can aid in diagnosing the issue.
- Key Properties of AxiosError:
- `message`: Describes the error.
- `response`: Contains the server’s response, including status code and error details.
- `config`: Reflects the configuration of the request made.
- `request`: The request object sent to the server.
To effectively debug an `AxiosError`, inspect the `response` property, particularly the `data` section which may provide insights into what went wrong.
Best Practices for Handling 400 Errors
Implementing best practices can mitigate the occurrence of 400 errors and improve the robustness of your application.
- Validation:
- Validate user input on the client-side before sending requests.
- Ensure all required fields are populated.
- Error Handling:
- Use try-catch blocks to handle potential errors gracefully.
- Provide user feedback when a bad request occurs.
- Logging:
- Log errors on the client side to capture the details of the failed request.
- Monitor server logs for patterns that may indicate systemic issues.
Example of Handling Axios 400 Errors
The following code snippet illustrates how to handle a 400 error in an Axios request:
“`javascript
axios.post(‘/api/endpoint’, { data: ‘example’ })
.then(response => {
console.log(‘Success:’, response.data);
})
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
console.error(‘Error Data:’, error.response.data);
console.error(‘Status Code:’, error.response.status);
console.error(‘Headers:’, error.response.headers);
} else if (error.request) {
// The request was made but no response was received
console.error(‘Request:’, error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.error(‘Error Message:’, error.message);
}
});
“`
This structure allows for comprehensive error handling, ensuring that developers can respond appropriately based on the type of error encountered.
Testing and Debugging Tools
Utilizing tools can simplify the process of diagnosing and fixing 400 errors.
Tool | Purpose |
---|---|
Postman | Test API requests and inspect responses. |
Fiddler | Monitor and analyze HTTP traffic. |
Browser Dev Tools | Debug JavaScript and network requests. |
These tools facilitate deeper insights into HTTP requests, making it easier to spot issues that lead to 400 errors.
Understanding AxiosError: Insights from Software Development Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A 400 status code typically indicates a client-side error, meaning the request sent to the server is malformed or invalid. It is crucial to validate the data being sent in the request before it is dispatched to avoid such errors.”
James Patel (Lead API Developer, Cloud Solutions Corp.). “When encountering an AxiosError with a 400 status code, developers should inspect the request payload and headers. Often, issues arise from incorrect content types or missing required parameters, which can be easily resolved through thorough testing.”
Lisa Nguyen (Full Stack Developer, CodeCraft Labs). “Debugging a 400 error in Axios requires a systematic approach. Utilizing tools like Postman can help simulate requests and pinpoint the exact cause of the error, ensuring that the API is receiving the expected input.”
Frequently Asked Questions (FAQs)
What does “AxiosError: Request Failed With Status Code 400” mean?
This error indicates that the server could not process the request due to a client-side issue, often caused by invalid input or a malformed request.
What are common causes of a 400 status code in Axios?
Common causes include missing required parameters, invalid data types, or incorrect endpoint URLs. Additionally, issues with authentication tokens or headers may also trigger this error.
How can I troubleshoot a 400 error in my Axios request?
To troubleshoot, check the request URL, verify the request method (GET, POST, etc.), ensure that all required parameters are included, and validate the data format being sent.
Can server-side issues cause a 400 status code?
No, a 400 status code specifically indicates a client-side error. Server-side issues typically result in different status codes, such as 500 for internal server errors.
What should I do if I encounter this error while using an API?
Review the API documentation for required parameters and expected formats. Use tools like Postman to test the request independently and identify any discrepancies.
Is there a way to handle Axios errors gracefully in my application?
Yes, implement error handling in your Axios requests using `.catch()` to manage errors effectively. You can log the error details and provide user-friendly messages based on the error type.
The Axios error indicating “Request Failed With Status Code 400” signifies that the server could not process the request due to client-side issues. This typically means that the request sent by the client is malformed or contains invalid parameters. Understanding the nature of a 400 Bad Request error is crucial for developers, as it directly impacts the functionality of web applications and APIs. Properly diagnosing the cause of this error can lead to more efficient debugging and improved user experience.
Key insights into addressing this error include validating the request payload and ensuring that all required fields are correctly formatted and included. Developers should also utilize tools such as Postman or browser developer tools to inspect the request and response details. By doing so, they can identify discrepancies between what is expected by the server and what is being sent from the client. Effective error handling and logging mechanisms can further aid in capturing these issues early in the development process.
In summary, encountering an Axios error with a status code of 400 serves as a reminder of the importance of meticulous request construction and validation. By adopting best practices in API communication and error handling, developers can minimize the occurrence of such errors and enhance the overall reliability of their applications. Continuous learning and adaptation to error responses will ultimately lead to a more
Author Profile
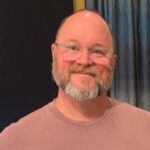
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?