How Can You Make a Variable Global in Java?
In the world of Java programming, managing variable scope is crucial for building efficient and maintainable applications. As developers dive into the intricacies of the language, they often encounter the need to share data across different parts of their code. This is where the concept of global variables comes into play. But what does it truly mean to make a variable global in Java, and how can it enhance your coding practices? In this article, we will unravel the mystery of global variables, exploring their significance, implementation strategies, and the best practices to ensure your code remains clean and efficient.
Understanding how to make a variable global involves grasping the nuances of Java’s variable scope and accessibility. Unlike some programming languages that allow true global variables, Java operates within a more structured framework, where variables are typically confined to their respective classes or methods. However, by utilizing specific techniques, developers can effectively achieve a similar outcome, allowing variables to be accessed from various locations in their code. This not only simplifies data management but also fosters better collaboration between different components of an application.
As we delve deeper into the topic, we will explore various methods for creating global-like variables in Java, including the use of static variables, singleton patterns, and other design principles. Each approach comes with its own set of advantages and considerations
Understanding Scope in Java
In Java, the concept of scope is crucial when dealing with variables. Scope determines where a variable can be accessed within the code. Variables can be categorized into different scopes:
- Local Variables: Defined within a method or block and accessible only within that method or block.
- Instance Variables: Associated with an instance of a class and can be accessed by all methods within the class.
- Static Variables: Belong to the class rather than any particular object and are shared among all instances.
To make a variable global in Java, you typically use static variables or instance variables, depending on the desired accessibility.
Using Static Variables
Static variables are declared with the `static` keyword. They are shared among all instances of a class, making them a suitable option for global variables.
“`java
public class GlobalVariableExample {
static int globalVar = 0; // Static variable
public static void increment() {
globalVar++;
}
public static void display() {
System.out.println(“Global Variable: ” + globalVar);
}
}
“`
In this example, `globalVar` can be accessed and modified from any static method in the class. Static variables can also be accessed using the class name:
“`java
GlobalVariableExample.globalVar = 10;
“`
Using Instance Variables
Instance variables can also serve as global variables, but they are tied to a specific instance of a class. This means you need to create an object of that class to access the variable.
“`java
public class GlobalVariableInstance {
int globalVar = 0; // Instance variable
public void increment() {
globalVar++;
}
public void display() {
System.out.println(“Global Variable: ” + globalVar);
}
}
“`
To use the instance variable globally, you would do the following:
“`java
GlobalVariableInstance instance = new GlobalVariableInstance();
instance.globalVar = 5;
“`
Comparison of Static and Instance Variables
The choice between static and instance variables depends on the specific requirements of your application. Below is a table summarizing key differences:
Feature | Static Variable | Instance Variable |
---|---|---|
Declaration | static | No static keyword |
Memory Location | Class level | Object level |
Accessibility | Shared across instances | Unique to each instance |
Use Case | Common data for all objects | Data specific to an object |
In summary, understanding the differences between static and instance variables is essential when deciding how to implement global variables in your Java applications.
Understanding Variable Scope in Java
In Java, the scope of a variable determines where the variable can be accessed or modified. There are several types of scopes:
- Local Variables: Declared within a method or block, accessible only within that method or block.
- Instance Variables: Declared within a class but outside any method, associated with an instance of the class.
- Static Variables: Declared with the `static` keyword, belong to the class rather than any instance, accessible from static methods.
To make a variable global, it typically needs to be accessible across different classes or methods. This is often achieved using instance or static variables.
Making a Variable Global Using Instance Variables
To declare a variable as global in the context of an object, you define it as an instance variable within a class. This allows the variable to be accessed by any method within that class, and by other classes if appropriately referenced.
Example:
“`java
public class MyClass {
// Instance variable
private int globalVariable;
public MyClass(int value) {
this.globalVariable = value; // Initialize the variable
}
public void displayValue() {
System.out.println(“Global Variable: ” + globalVariable);
}
}
“`
In this example, `globalVariable` is accessible throughout the `MyClass` methods.
Making a Variable Global Using Static Variables
Static variables are shared across all instances of a class, making them globally accessible if the class is referenced correctly. To declare a static variable:
- Use the `static` keyword.
- Access it directly through the class name or through an instance.
Example:
“`java
public class MyClass {
// Static variable
static int globalStaticVariable;
public static void setGlobalStaticVariable(int value) {
globalStaticVariable = value; // Set the static variable
}
public static void displayStaticValue() {
System.out.println(“Global Static Variable: ” + globalStaticVariable);
}
}
“`
You can access `globalStaticVariable` using `MyClass.globalStaticVariable` or via `MyClass.displayStaticValue()`.
Accessing Global Variables Across Classes
To access a global variable from another class, ensure the variable is declared as public or use getter methods.
Example of accessing a static variable:
“`java
public class AnotherClass {
public void accessGlobal() {
MyClass.setGlobalStaticVariable(10);
MyClass.displayStaticValue(); // Output: Global Static Variable: 10
}
}
“`
In this case, `AnotherClass` accesses `globalStaticVariable` through `MyClass`.
Best Practices for Global Variables
When working with global variables, consider these best practices:
- Use access modifiers: Control access with `private`, `protected`, or `public`.
- Avoid unnecessary global variables: Limit global variables to enhance code maintainability and reduce complexity.
- Prefer encapsulation: Use getter and setter methods to manage access to global variables.
- Document usage: Clearly comment on the purpose and usage of global variables to aid future developers.
Conclusion on Variable Scope Management
Understanding how to manage variable scope effectively allows for cleaner, more maintainable Java code. Utilizing instance and static variables appropriately can facilitate global access while ensuring proper encapsulation. Following best practices promotes better design and reduces potential issues related to variable management.
Expert Insights on Making Variables Global in Java
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “To make a variable global in Java, one must declare it as a static variable within a class. This allows the variable to be accessed across all instances of that class, effectively making it global within the scope of the application.”
Michael Chen (Software Architect, CodeCraft Solutions). “Utilizing static variables is a common approach to achieve global access in Java. However, developers should be cautious about thread safety and potential side effects when multiple threads access these global variables concurrently.”
Sarah Patel (Java Instructor, Advanced Programming Academy). “While making a variable global can simplify access, it is crucial to consider design principles. Overusing global variables can lead to tightly coupled code, which is harder to maintain. Instead, consider using dependency injection or singleton patterns for better design.”
Frequently Asked Questions (FAQs)
How do I declare a global variable in Java?
To declare a global variable in Java, you need to define it as a static variable inside a class but outside any method. This makes it accessible to all methods within that class.
What is the difference between a global variable and a local variable in Java?
A global variable is defined at the class level and can be accessed by all methods within that class, while a local variable is defined within a method and can only be accessed within that method’s scope.
Can I access a global variable from another class in Java?
Yes, you can access a global variable from another class if it is declared as public and static. You would access it using the class name followed by the variable name.
What are the best practices for using global variables in Java?
Best practices include minimizing the use of global variables to avoid tight coupling, using them only when necessary, and ensuring they are encapsulated properly to maintain data integrity.
How can I make a variable global across multiple classes in Java?
To make a variable global across multiple classes, declare it as public static in a common class. Other classes can then access it using the class name.
Are there any drawbacks to using global variables in Java?
Yes, global variables can lead to code that is difficult to maintain and debug, as they can be modified from various places in the code, potentially leading to unpredictable behavior.
In Java, making a variable global typically involves defining it as a class-level variable, also known as an instance variable or a static variable, depending on the scope required. By declaring a variable outside of any methods, it becomes accessible to all methods within the class, thus achieving a global-like behavior within that class. If a variable needs to be shared across multiple instances of a class, it can be declared as static, which allows it to be accessed without creating an instance of the class.
Another important aspect of global variables in Java is the use of access modifiers. By utilizing public, private, or protected keywords, developers can control the visibility of these variables. Public variables can be accessed from any other class, while private variables are restricted to the defining class. This encapsulation is crucial for maintaining data integrity and adhering to object-oriented principles.
while Java does not support true global variables in the same way some other programming languages do, developers can achieve similar functionality through class-level variables and appropriate access modifiers. Understanding how to declare and manage these variables effectively is essential for writing clean, maintainable, and efficient Java code.
Author Profile
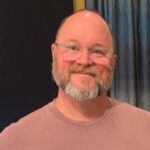
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?