How Can You Use Python If Statements on One Line for Efficient Coding?
:
In the world of programming, efficiency and readability are paramount, especially when it comes to writing clean and concise code. Python, known for its elegant syntax and simplicity, offers developers a variety of ways to express their logic. One particularly interesting aspect is the ability to condense conditional statements into a single line, allowing for more streamlined code. Whether you’re a seasoned developer looking to refine your skills or a beginner eager to grasp the nuances of Python, mastering the art of writing an `if` statement on one line can significantly enhance your coding prowess.
In this article, we will explore the concept of writing Python `if` statements in a single line, a technique that not only saves space but also maintains clarity. This approach is particularly useful in scenarios where you want to execute simple conditions without cluttering your code with multiple lines. By leveraging Python’s unique syntax, you can create elegant solutions that are both functional and aesthetically pleasing.
We’ll delve into practical examples and best practices, highlighting the scenarios where one-liners can be effectively employed. As we navigate through the intricacies of this technique, you’ll discover how to balance brevity with readability, ensuring that your code remains accessible to others (and to yourself in the future). Prepare to unlock a new level of efficiency in your
Single-Line If Statements
In Python, you can use a single-line if statement to simplify code where only a simple conditional action is necessary. This syntactical construct enhances readability and conciseness, making it suitable for situations where you want to execute a single expression based on a condition.
The general syntax for a single-line if statement is as follows:
“`python
x = 5
result = “Greater than 2” if x > 2 else “Not greater than 2”
“`
In this example, the expression evaluates `x > 2`. If true, `result` is assigned “Greater than 2”; otherwise, it is assigned “Not greater than 2”. This format is often referred to as the ternary operator.
Using Single-Line If Statements Effectively
When using single-line if statements, consider the following:
- Clarity: Ensure that the condition and expressions are clear and straightforward.
- Complexity: Avoid nesting multiple conditions in a single line, as this can lead to reduced readability.
- Use Cases: Best suited for simple conditions where the intent is easily understood.
Here are a few scenarios where single-line if statements can be advantageous:
- Assigning a value based on a condition.
- Returning a value from a function based on a simple check.
- Filtering data in list comprehensions.
Examples of Single-Line If Statements
Below are some practical examples demonstrating single-line if statements in various contexts:
“`python
Assigning a value based on a condition
status = “Adult” if age >= 18 else “Minor”
Returning a value in a function
def check_even(num):
return “Even” if num % 2 == 0 else “Odd”
Filtering a list using list comprehension
numbers = [1, 2, 3, 4, 5]
even_numbers = [n for n in numbers if n % 2 == 0]
“`
Limitations of Single-Line If Statements
While single-line if statements can be useful, they do have limitations. Here are some points to consider:
- Readability: Overusing single-line statements can make code harder to read, especially for complex conditions.
- Debugging Difficulty: Debugging can become more challenging when logic is condensed into a single line.
To illustrate when to use single-line if statements versus traditional multi-line statements, consider the following table:
Situation | Single-Line Example | Multi-Line Example |
---|---|---|
Simple assignment | x = “Pass” if score >= 50 else “Fail” |
x = “Fail” if score >= 50: x = “Pass” |
Function return | return “Positive” if num > 0 else “Negative” |
if num > 0: return “Positive” else: return “Negative” |
In summary, single-line if statements are a powerful feature in Python that can lead to cleaner and more efficient code when used judiciously. Balancing their use with clarity and maintainability is crucial for effective programming.
Using Conditional Expressions in Python
In Python, you can use a conditional expression, also known as a ternary operator, to write an `if` statement in a single line. This is particularly useful for simple assignments or return statements. The syntax is as follows:
“`python
value_if_true if condition else value_if_
“`
Example of Conditional Expressions
Here’s a practical example that demonstrates how to use a conditional expression:
“`python
age = 20
status = “Adult” if age >= 18 else “Minor”
“`
In this example, the variable `status` will be assigned “Adult” if `age` is 18 or older; otherwise, it will be assigned “Minor”.
Use Cases for One-Line If Statements
One-line `if` statements are best suited for:
- Simple assignments
- Return statements in functions
- Inline condition checks within list comprehensions
- Short and clear conditional logic
List Comprehensions with Conditional Logic
You can also incorporate one-line `if` statements within list comprehensions. This allows for filtering or modifying elements in a list based on a condition. Here’s how it works:
“`python
numbers = [1, 2, 3, 4, 5]
squared_even = [x**2 for x in numbers if x % 2 == 0]
“`
In this snippet, `squared_even` will contain the squares of even numbers from the `numbers` list.
Using `and` and `or` for Conditional Logic
For even more concise expressions, you can utilize logical operators to combine conditions. Here’s how:
- Using `and`:
“`python
result = “Valid” if age >= 18 and age < 65 else "Invalid"
```
- Using `or`:
“`python
status = “Minor” if age < 18 else "Adult" if age < 65 else "Senior"
```
Limitations of One-Line If Statements
While one-line `if` statements can enhance readability for simple conditions, they can become unwieldy and difficult to read if overly complex. It’s important to maintain clarity in your code.
Best Practices
- Keep it simple: Use one-liners for straightforward logic.
- Readability: If the condition is complex, consider using traditional multi-line `if` statements.
- Consistency: Maintain a consistent style throughout your codebase to improve maintainability.
By leveraging one-line `if` statements judiciously, you can write more concise and elegant Python code, enhancing both its functionality and readability.
Expert Insights on Python One-Liner If Statements
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Using one-liner if statements in Python can greatly enhance code readability when applied judiciously. However, developers must be cautious not to sacrifice clarity for brevity, especially in complex conditions.”
James Liu (Python Developer and Author, CodeCraft Publications). “One-liners can be a powerful tool in Python, particularly for simple conditional assignments. They allow for concise expressions that can streamline code, but it’s essential to ensure that they remain understandable to others who may read the code later.”
Sarah Thompson (Lead Data Scientist, Insight Analytics). “In data science applications, one-liner if statements can simplify data manipulation tasks significantly. Yet, it is crucial to maintain a balance between compactness and the potential for introducing bugs due to overly complex expressions.”
Frequently Asked Questions (FAQs)
What is a one-liner if statement in Python?
A one-liner if statement in Python, often referred to as a conditional expression or ternary operator, allows for a concise way to write simple conditional logic in a single line. The syntax is `value_if_true if condition else value_if_`.
How do I use a one-liner if statement in a list comprehension?
In a list comprehension, a one-liner if statement can be used to filter or modify elements. The syntax is `[expression if condition else alternative for item in iterable]`, which allows for conditional processing of each item.
Can I use multiple conditions in a one-liner if statement?
Yes, you can use multiple conditions in a one-liner if statement by chaining them with logical operators. For example: `value_if_true if condition1 else value_if_second_true if condition2 else value_if_`.
What are the limitations of using one-liner if statements?
One-liner if statements can reduce readability, especially with complex conditions or multiple nested expressions. It is advisable to use them for simple conditions to maintain code clarity and avoid confusion.
Is it possible to use one-liner if statements with functions?
Yes, one-liner if statements can be used within function calls to determine arguments based on conditions. For example: `function(arg_if_true if condition else arg_if_)` allows for dynamic argument selection.
Are one-liner if statements a good practice in Python?
One-liner if statements can enhance code brevity, but they should be used judiciously. For complex logic, traditional multi-line if statements are often preferred to ensure clarity and maintainability.
In Python, the ability to write conditional statements on one line is a feature that enhances code readability and conciseness. This is primarily achieved through the use of the ternary conditional operator, which allows developers to express simple if-else conditions succinctly. The syntax follows the format: `value_if_true if condition else value_if_`. This approach not only reduces the number of lines of code but also makes it easier to understand the logic at a glance when dealing with straightforward conditions.
Moreover, utilizing one-liners for conditional statements can be particularly beneficial in scenarios where brevity is essential, such as in list comprehensions or lambda functions. However, while this feature can streamline code, it is crucial to maintain clarity. Overusing one-liners for complex conditions can lead to code that is difficult to read and maintain. Therefore, developers should exercise discretion when opting for this style, ensuring that the code remains understandable to others who may work with it in the future.
In summary, Python’s capability to implement if statements on one line serves as a powerful tool for developers aiming for concise and efficient code. The key takeaway is to balance brevity with clarity, using one-liners judiciously to enhance the overall quality of the code
Author Profile
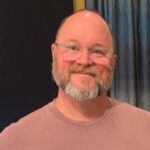
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?