Why Must Every Derived Table Have Its Own Alias in SQL?
In the intricate world of SQL and database management, the nuances of writing efficient queries can often make the difference between a well-performing application and a sluggish one. One such nuance that every developer must grasp is the importance of using aliases for derived tables. While the concept may seem trivial at first glance, it serves as a foundational principle that enhances both the clarity and functionality of your SQL statements. In this article, we will delve into the critical rule that “Every Derived Table Must Have Its Own Alias,” exploring its significance, common pitfalls, and best practices to ensure your queries are not only syntactically correct but also optimized for performance.
Derived tables, often created using subqueries in the FROM clause, allow for powerful data manipulation and retrieval. However, without a unique alias, these temporary result sets can lead to confusion and errors in your SQL statements. The requirement for an alias is not merely a stylistic choice; it is a necessary component that enables the SQL engine to accurately reference and manage these ephemeral tables. By understanding the rationale behind this rule, developers can avoid common mistakes that may arise from neglecting to assign an alias, ultimately leading to cleaner and more maintainable code.
As we navigate through the nuances of derived tables and their aliases, we will also highlight the implications of this
Understanding Derived Tables
Derived tables are temporary result sets created within a SQL statement, often used in complex queries to simplify the retrieval of data. They can be thought of as subqueries or inline views that provide a means to encapsulate a query and treat it as a table.
A critical rule when working with derived tables is that each must have its own alias. This requirement is essential for several reasons:
- Clarity: Assigning an alias makes it clear to the reader what the derived table represents within the broader query context.
- Reference: The alias allows for easy reference to the derived table in the main query, facilitating better organization and readability.
- Avoiding Ambiguity: In cases where multiple derived tables are used, aliases prevent confusion by clearly distinguishing each result set.
Alias Requirement in SQL
When a derived table is defined, it is necessary to provide an alias immediately after the derived table’s definition. The syntax for creating a derived table with an alias typically looks like this:
“`sql
SELECT column1, column2
FROM (SELECT columnA, columnB FROM table_name WHERE condition) AS alias_name
“`
In the example above, `alias_name` serves as the identifier for the derived table generated by the inner query.
Example of Derived Tables with Aliases
Consider the following SQL example that demonstrates the use of a derived table with an alias:
“`sql
SELECT dt.employee_id, dt.total_sales
FROM (
SELECT employee_id, SUM(sales) AS total_sales
FROM sales_data
GROUP BY employee_id
) AS dt
WHERE dt.total_sales > 10000;
“`
In this query:
- The inner query computes total sales per employee and is aliased as `dt`.
- The outer query references `dt` to filter employees with total sales exceeding 10,000.
Common Mistakes and Best Practices
When working with derived tables, several common mistakes can arise, particularly regarding alias usage:
- Failure to Provide an Alias: Omitting an alias will result in a syntax error.
- Reusing Aliases: Using the same alias for multiple derived tables within the same query can cause confusion and errors.
- Inconsistent Naming: Choose meaningful and consistent names for aliases to enhance readability.
Best practices include:
- Always provide an alias for every derived table.
- Use descriptive names that reflect the purpose or content of the derived table.
- Maintain a consistent naming convention throughout your SQL queries.
Derived Table Alias | Description |
---|---|
dt | Represents the derived table with total sales per employee. |
sales_summary | A summary of sales data, typically aggregating figures. |
filtered_results | Contains results filtered by specific criteria. |
By adhering to the requirement that every derived table must have its own alias, SQL developers can create clear, maintainable, and effective queries that yield the desired results while avoiding potential pitfalls associated with ambiguity and confusion.
Understanding Derived Tables and Their Aliases
In SQL, a derived table is a temporary result set created within the context of a query. This result set must be given an alias for the database management system (DBMS) to reference it properly. Without an alias, the SQL statement will result in an error.
Importance of Aliases
- Clarity: Aliases make the query more readable, allowing users to understand the context and purpose of the derived table.
- Reference: SQL requires an alias to identify the derived table when performing additional operations such as joins or selections.
- Avoiding Errors: Ensuring that every derived table has an alias helps prevent syntax errors that can occur during query execution.
Syntax for Using Derived Tables
When constructing a SQL query with a derived table, the syntax generally follows this format:
“`sql
SELECT column1, column2
FROM (SELECT column1, column2 FROM table_name WHERE condition) AS alias_name
WHERE additional_condition;
“`
Example of a Derived Table with Alias
Consider a scenario where you want to calculate the average salary from a table named `employees`. The derived table will be aliased for further use:
“`sql
SELECT avg_salaries.department, avg_salaries.avg_salary
FROM (
SELECT department, AVG(salary) AS avg_salary
FROM employees
GROUP BY department
) AS avg_salaries;
“`
Common Mistakes to Avoid
- Omitting the Alias: Forgetting to provide an alias will lead to a syntax error. Always ensure an alias follows the derived table.
- Using Reserved Keywords: Avoid using SQL reserved keywords as aliases without proper quotation, as this can also result in errors.
- Ambiguous Aliases: Choose meaningful and distinct aliases that clearly indicate the content of the derived table.
Best Practices
- Use Descriptive Names: When creating an alias, use a name that reflects the data contained within the derived table. For example, use `sales_summary` instead of just `s`.
- Keep it Short: While clarity is essential, try to keep aliases concise to maintain readability.
- Follow Naming Conventions: Adhere to any established naming conventions within your organization or team for consistency.
Conclusion on Aliases in Derived Tables
The requirement that every derived table must have its own alias is fundamental in SQL. It ensures clarity, prevents errors, and enhances the overall readability of queries. By following best practices in naming and structuring derived tables, developers can write more efficient and understandable SQL code.
The Importance of Aliases in Derived Tables
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Every derived table must have its own alias to ensure clarity and avoid ambiguity in SQL queries. Without an alias, it becomes challenging for both the database engine and the developer to reference the derived table, leading to potential errors and confusion in complex queries.”
Mark Thompson (Senior Data Analyst, Insight Analytics). “Using aliases for derived tables is not just a best practice; it is essential for maintaining readable and maintainable code. When collaborating with teams, clear naming conventions through aliases facilitate better understanding and communication among developers.”
Linda Martinez (SQL Consultant, Data Solutions Group). “In SQL, derived tables without aliases can lead to unexpected results and make debugging significantly more difficult. An alias acts as a reference point, allowing for easier identification of the data being manipulated and ensuring that queries perform as intended.”
Frequently Asked Questions (FAQs)
What is a derived table in SQL?
A derived table is a temporary result set created within a SQL query, typically using a subquery in the FROM clause. It allows for complex queries by encapsulating logic and filtering data before the main query processes it.
Why must every derived table have its own alias?
Every derived table must have its own alias to provide a unique identifier for the result set. This is necessary for referencing the derived table in the outer query and avoiding ambiguity in SQL syntax.
What happens if a derived table does not have an alias?
If a derived table does not have an alias, the SQL query will result in a syntax error. SQL requires that all derived tables be named to ensure clarity and proper execution of the query.
Can you provide an example of a derived table with an alias?
Certainly. An example would be:
“`sql
SELECT * FROM (SELECT employee_id, salary FROM employees WHERE department = ‘Sales’) AS sales_employees;
“`
In this example, the derived table is aliased as `sales_employees`.
Is it possible to use the alias of a derived table in the outer query?
Yes, the alias of a derived table can be used in the outer query to reference the columns within the derived table. This allows for further filtering, aggregation, or joining with other tables.
Are there any exceptions to the rule of needing an alias for derived tables?
No, there are no exceptions. SQL standards mandate that all derived tables must have an alias to ensure that the query is both valid and understandable.
In SQL, a derived table is a temporary result set created within a query, often used in the FROM clause to simplify complex queries. However, it is essential to note that every derived table must have its own alias. This requirement is critical because it allows the database engine to reference the derived table in the outer query, ensuring clarity and preventing ambiguity in the SQL statement.
The necessity for an alias for each derived table enhances the readability and maintainability of SQL code. By providing a clear identifier, developers can easily understand the purpose and context of the derived table within the larger query structure. This practice also aids in debugging, as it allows for straightforward identification of specific components within complex queries.
In summary, adhering to the rule that every derived table must have its own alias is not merely a syntactical requirement but a best practice that promotes clarity and efficiency in SQL programming. By consistently applying this principle, developers can create more organized and understandable SQL queries, ultimately leading to better performance and easier maintenance of database systems.
Author Profile
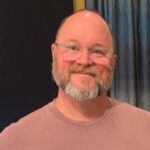
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?