How Can You Create a File in JavaScript? A Step-by-Step Guide
Creating files in JavaScript is a powerful capability that opens up a world of possibilities for developers, whether they’re building web applications, server-side scripts, or even desktop applications using frameworks like Electron. In an era where data management and user interaction are paramount, understanding how to generate and manipulate files programmatically can significantly enhance user experience and functionality. This article will guide you through the essential techniques and methods for creating files in JavaScript, empowering you to take your projects to the next level.
At its core, the ability to create files in JavaScript involves leveraging various APIs and libraries that facilitate file generation and manipulation. From the browser’s built-in capabilities to Node.js’s robust file system module, developers have a range of tools at their disposal. Whether you’re looking to generate text files, JSON data, or even complex binary formats, JavaScript provides the means to do so seamlessly.
Moreover, the process of file creation can be tailored to suit different environments, such as client-side applications where user interaction is key, or server-side applications that handle data storage and retrieval. Understanding the nuances of these environments will not only enhance your coding skills but also enable you to create more dynamic and responsive applications. As we delve deeper into the methods and best practices for file creation in JavaScript, you’ll discover
Creating a File in JavaScript Using the File System API
To create a file in JavaScript, particularly in a Node.js environment, the File System API (fs) is a powerful tool. This API provides methods to interact with the file system, allowing you to create, read, update, and delete files.
To begin, ensure you have Node.js installed on your machine. You can create a file using the following steps:
- **Import the File System Module**: First, you need to require the fs module in your JavaScript file.
“`javascript
const fs = require(‘fs’);
“`
- **Create a New File**: Use the `fs.writeFile()` method to create a new file. This method takes the file path, data to write, and a callback function.
“`javascript
fs.writeFile(‘example.txt’, ‘Hello, World!’, (err) => {
if (err) throw err;
console.log(‘File has been created!’);
});
“`
This snippet will create a file named `example.txt` with the content “Hello, World!”. If the file already exists, it will be overwritten.
Creating Files in the Browser Using Blob and URL.createObjectURL
In a browser context, JavaScript does not have direct access to the file system for security reasons. However, you can create files using the Blob object and the `URL.createObjectURL()` method. This is particularly useful for generating files that users can download.
- Create a Blob Object: First, create a Blob containing the data you want to store in the file.
“`javascript
const data = new Blob([“Hello, World!”], { type: ‘text/plain’ });
“`
- Generate a URL for the Blob: Use the `URL.createObjectURL()` method to create a URL for the Blob.
“`javascript
const url = URL.createObjectURL(data);
“`
- Create a Download Link: Finally, create a link element that allows users to download the file.
“`javascript
const a = document.createElement(‘a’);
a.href = url;
a.download = ‘example.txt’;
a.textContent = ‘Download example.txt’;
document.body.appendChild(a);
“`
This will create a link on your webpage that users can click to download the generated text file.
Comparison of Node.js File Creation and Browser File Generation
Feature | Node.js File System API | Browser Blob/URL API |
---|---|---|
Direct File System Access | Yes | No |
File Overwrite Option | Yes | No (new file each time) |
Callback for Completion | Yes | N/A |
Download Prompt | N/A | Yes |
Use Cases | Server-side applications | Client-side applications |
The table above highlights the key differences between file creation methods in Node.js and the browser. Each method has its unique use cases, allowing developers to choose the appropriate approach based on the environment they are working in.
Creating a File in JavaScript Using the File API
The File API provides a set of methods and properties that allow you to create and manipulate files in a web environment. To create a file, you typically work with the Blob object, which represents a file-like object of immutable, raw data.
Using Blob and URL.createObjectURL
To create a file, you can utilize the Blob constructor along with the URL.createObjectURL method. Here’s how you can do this:
“`javascript
// Create a new Blob object containing text data
const data = new Blob([“Hello, World!”], { type: ‘text/plain’ });
// Create a URL for the Blob
const url = URL.createObjectURL(data);
// Create an anchor element and set its href to the Blob URL
const a = document.createElement(‘a’);
a.href = url;
a.download = ‘hello.txt’; // Specify the file name
// Append the anchor to the body
document.body.appendChild(a);
// Programmatically click the anchor to trigger the download
a.click();
// Clean up by revoking the Blob URL
URL.revokeObjectURL(url);
“`
In this example, a text file named `hello.txt` is created and automatically downloaded when the script is executed.
Creating a File with User Input
To allow users to create files based on their input, you can modify the previous example to include an input field. Here’s a demonstration:
“`html
“`
In this code, a `
Considerations for File Creation
When creating files in JavaScript, consider the following aspects:
- Browser Compatibility: Ensure the File API is supported in the target browsers.
- File Size Limitations: Be aware of limitations for large files; avoid excessive memory usage.
- Security Concerns: Respect user privacy and file handling permissions, especially when working with sensitive data.
Additional Libraries and Frameworks
For more advanced file handling, consider using libraries such as:
Library | Description |
---|---|
FileSaver.js | Simplifies file saving on the client-side. |
JSZip | Allows for creating and manipulating ZIP files. |
PouchDB | Provides a NoSQL database that can manage file data. |
These tools can enhance your ability to manage files and improve user experience in your applications.
Expert Insights on Creating Files in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating files in JavaScript can be efficiently handled using the File System API in Node.js, which allows developers to read and write files seamlessly. This API provides a robust set of methods that can be utilized for various file operations, making it essential for server-side applications.”
James Liu (Web Development Instructor, Code Academy). “For client-side applications, developers can utilize the Blob and URL.createObjectURL methods to create files dynamically in the browser. This approach is particularly useful for generating downloadable content on-the-fly, enhancing user experience without requiring server interaction.”
Sarah Thompson (JavaScript Framework Specialist, Dev Solutions). “Understanding the context in which you need to create files is crucial. For instance, while the browser environment limits direct file system access, leveraging libraries like FileSaver.js can simplify the process of saving files generated in the client-side JavaScript, ensuring compatibility across various browsers.”
Frequently Asked Questions (FAQs)
How can I create a file in JavaScript?
You can create a file in JavaScript using the Blob API. By creating a Blob object and using the URL.createObjectURL method, you can generate a downloadable link for the file.
What is the Blob API in JavaScript?
The Blob API allows you to create immutable raw binary data. It is commonly used to handle file-like objects in web applications, enabling the creation of files directly in the browser.
Can I save a file directly to the user’s file system using JavaScript?
No, JavaScript running in the browser cannot directly save files to the user’s file system due to security restrictions. Instead, you can prompt the user to download a file using a link with a Blob URL.
What is the syntax to create a Blob in JavaScript?
The syntax to create a Blob is: `new Blob([data], { type: ‘mime/type’ });` where `data` can be a string or an array of data, and `type` specifies the MIME type of the file.
How do I trigger a download of a file created in JavaScript?
To trigger a download, create an anchor element (``), set its `href` attribute to the Blob URL created with `URL.createObjectURL(blob)`, and programmatically click the anchor element.
Is it possible to create text files using JavaScript?
Yes, you can create text files in JavaScript by creating a Blob with text data and specifying the MIME type as ‘text/plain’. This allows users to download the text file easily.
In summary, creating a file in JavaScript can be accomplished using various methods depending on the environment in which the code is executed. For web applications, the File API and Blob objects are instrumental in generating files dynamically in the browser. This approach allows developers to create text files, images, and other formats that users can download directly. Additionally, the use of libraries like FileSaver.js can simplify the process of saving files on the client side.
In server-side JavaScript, particularly with Node.js, the ‘fs’ (file system) module provides robust capabilities for file creation and manipulation. This enables developers to read, write, and manage files on the server, facilitating tasks such as logging, data storage, and configuration management. Understanding the differences between client-side and server-side file handling is crucial for effective application development.
Key takeaways include the importance of selecting the appropriate method based on the specific use case, whether it be for client-side applications or server-side environments. Additionally, developers should be aware of the security implications and user permissions associated with file creation, particularly in web applications. Overall, mastering file creation in JavaScript enhances a developer’s ability to build interactive and functional applications.
Author Profile
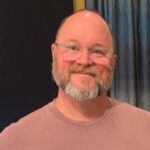
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?