How Can You Use PowerShell to Remove Duplicates From an Array?
In the world of scripting and automation, PowerShell stands out as a powerful tool for managing and manipulating data. One common challenge that many developers and system administrators face is dealing with duplicate entries in arrays. Whether you’re processing logs, filtering user data, or managing configurations, the presence of duplicates can lead to inefficiencies and inaccuracies. Understanding how to effectively remove duplicates from arrays in PowerShell not only streamlines your scripts but also enhances the overall performance of your tasks.
Removing duplicates from an array in PowerShell is a fundamental skill that can simplify your data management processes. PowerShell offers a variety of methods and cmdlets that allow users to efficiently filter out redundant entries, ensuring that the data you work with is clean and reliable. From leveraging built-in functions to utilizing custom scripts, there are several approaches to tackle this challenge, each with its own advantages and use cases.
As we delve deeper into this topic, we will explore the various techniques available in PowerShell for removing duplicates from arrays. Whether you’re a seasoned PowerShell user or just starting out, mastering these methods will empower you to handle data more effectively, paving the way for more robust automation solutions. Get ready to enhance your scripting skills and take control of your data management tasks!
Using `Select-Object` to Remove Duplicates
One of the most straightforward methods to remove duplicates from an array in PowerShell is by utilizing the `Select-Object` cmdlet. This cmdlet allows for the selection of unique objects from a collection, making it an effective tool for deduplication.
To implement this, you can use the `-Unique` parameter. Here’s a basic example:
“`powershell
$array = 1, 2, 2, 3, 4, 4, 5
$uniqueArray = $array | Select-Object -Unique
“`
In this example, `$uniqueArray` will contain the values `1, 2, 3, 4, 5`, effectively removing duplicates.
Using HashSet for Performance
For larger datasets, leveraging a `HashSet` can provide improved performance due to its underlying data structure, which is optimized for uniqueness checks. Here’s how you can utilize a `HashSet` in PowerShell to remove duplicates:
“`powershell
$array = 1, 2, 2, 3, 4, 4, 5
$hashSet = New-Object System.Collections.Generic.HashSet[int]
$array | ForEach-Object { $hashSet.Add($_) }
$uniqueArray = $hashSet.ToArray()
“`
This method ensures that only unique items are added to the `HashSet`, and the final array can be retrieved using the `ToArray()` method.
Utilizing `Group-Object` for Complex Structures
When dealing with arrays of complex objects, `Group-Object` can be beneficial. This cmdlet groups objects based on specified properties and allows you to select unique items based on those properties.
Consider an array of objects as follows:
“`powershell
$people = @(
[PSCustomObject]@{ Name = “Alice”; Age = 30 },
[PSCustomObject]@{ Name = “Bob”; Age = 25 },
[PSCustomObject]@{ Name = “Alice”; Age = 30 },
[PSCustomObject]@{ Name = “Charlie”; Age = 35 }
)
$uniquePeople = $people | Group-Object -Property Name | ForEach-Object { $_.Group[0] }
“`
This script groups the objects by the `Name` property and selects the first occurrence from each group, resulting in an array of unique names.
Comparison of Methods
The following table summarizes the methods discussed for removing duplicates from arrays in PowerShell:
Method | Description | Performance | Use Case |
---|---|---|---|
Select-Object | Simple deduplication using the -Unique parameter. | Moderate | Small to medium arrays. |
HashSet | Utilizes a HashSet for efficient uniqueness checks. | High | Large arrays with many duplicates. |
Group-Object | Groups objects by properties to retrieve unique entries. | Moderate | Complex objects or when specific properties matter. |
Each method has its advantages and is suitable for different scenarios. Selecting the right approach will depend on the specific requirements of your data and performance considerations.
Removing Duplicates from an Array in PowerShell
To effectively remove duplicates from an array in PowerShell, various methods can be employed, each with its own set of advantages. The most common approaches include using the `Sort-Object` cmdlet, leveraging hash tables, and utilizing the `Select-Object` cmdlet.
Using Sort-Object
The `Sort-Object` cmdlet can be used in combination with the `-Unique` parameter to eliminate duplicates from an array. This method is straightforward and efficient for most use cases.
“`powershell
$array = 1, 2, 2, 3, 4, 4, 5
$uniqueArray = $array | Sort-Object -Unique
“`
- Input Array: `1, 2, 2, 3, 4, 4, 5`
- Output Array: `1, 2, 3, 4, 5`
Using Hash Tables
Another effective method is to use a hash table to store unique values. This approach is particularly useful for larger datasets where performance is critical, as hash tables provide faster lookups.
“`powershell
$array = 1, 2, 2, 3, 4, 4, 5
$hashTable = @{}
foreach ($item in $array) {
$hashTable[$item] = $null
}
$uniqueArray = $hashTable.Keys
“`
- Input Array: `1, 2, 2, 3, 4, 4, 5`
- Output Array: `1, 2, 3, 4, 5`
Using Select-Object
The `Select-Object` cmdlet can also be applied to remove duplicates by using the `-Unique` parameter. This method is particularly useful when dealing with complex objects or when specific properties need to be considered.
“`powershell
$array = @(
[PSCustomObject]@{ Name = ‘Alice’; Age = 30 },
[PSCustomObject]@{ Name = ‘Bob’; Age = 25 },
[PSCustomObject]@{ Name = ‘Alice’; Age = 30 }
)
$uniqueArray = $array | Select-Object -Unique
“`
- Input Array: Contains duplicate custom objects.
- Output Array: Unique custom objects based on all properties.
Performance Considerations
When choosing a method to remove duplicates from an array, consider the following factors:
Method | Performance | Use Case |
---|---|---|
Sort-Object | Moderate | General use, small to medium arrays |
Hash Table | Fast | Large datasets requiring high performance |
Select-Object | Moderate | Complex objects with specific properties |
Selecting the appropriate method is crucial depending on the size of the array and the complexity of the objects involved. Each method provides a robust solution for removing duplicates, allowing for flexibility based on specific requirements.
Expert Insights on Removing Duplicates from Arrays in PowerShell
Jessica Lin (Senior Software Developer, Tech Innovations Inc.). “When working with arrays in PowerShell, utilizing the `Select-Object -Unique` method is one of the most efficient ways to remove duplicates. This approach not only simplifies the code but also enhances performance when handling large datasets.”
Mark Thompson (PowerShell Automation Specialist, SysAdmin Weekly). “In my experience, combining the `Sort-Object` cmdlet with `Get-Unique` can be particularly useful for ensuring that duplicates are removed in a sorted manner. This method is especially beneficial when the order of items is not a priority but uniqueness is essential.”
Dr. Emily Carter (Data Scientist, Analytics Hub). “For complex data structures, such as arrays of objects, leveraging LINQ-like functionality in PowerShell can be advantageous. Using `Group-Object` followed by `Select-Object` allows for precise control over which properties to evaluate for uniqueness, making it a powerful technique in data processing.”
Frequently Asked Questions (FAQs)
How can I remove duplicates from an array in PowerShell?
You can remove duplicates from an array in PowerShell by using the `Select-Object` cmdlet with the `-Unique` parameter. For example: `$array = 1, 2, 2, 3; $uniqueArray = $array | Select-Object -Unique`.
Is there a method to remove duplicates without affecting the original array?
Yes, you can create a new array that contains only unique values without modifying the original array. Use the same `Select-Object -Unique` method and assign the result to a new variable.
Can I remove duplicates from an array of objects in PowerShell?
Yes, you can remove duplicates from an array of objects by specifying a property to compare. For example: `$uniqueObjects = $objects | Select-Object -Property PropertyName -Unique`.
What is the difference between using `Sort-Object` and `Select-Object -Unique`?
`Sort-Object` sorts the array and can remove duplicates if used in conjunction with `Select-Object -Unique`. However, `Select-Object -Unique` directly filters out duplicates without sorting, preserving the original order.
Are there any performance considerations when removing duplicates from large arrays in PowerShell?
Yes, performance may vary depending on the method used. The `Select-Object -Unique` method is generally efficient, but for very large arrays, consider using hash tables or LINQ-like approaches for improved performance.
Can I use LINQ-style queries to remove duplicates in PowerShell?
Yes, you can use LINQ-style queries by leveraging the `System.Linq` namespace. This allows for more complex operations, including filtering and grouping, to remove duplicates from arrays.
In summary, removing duplicates from an array in PowerShell is a straightforward task that can be accomplished using various methods. The most common approach is to utilize the `Select-Object` cmdlet with the `-Unique` parameter, which effectively filters out duplicate values from an array. This method is not only efficient but also easy to implement for users of all skill levels.
Another useful technique involves leveraging the `Get-Unique` cmdlet, which can be particularly beneficial when working with sorted data. Additionally, utilizing hash tables or the `Group-Object` cmdlet can provide more advanced solutions for managing duplicates, especially in complex data structures. Understanding these different methods allows users to choose the most appropriate solution based on their specific requirements.
Key takeaways include the importance of selecting the right method based on the context of the data and the desired outcome. PowerShell provides flexible options for handling duplicates, making it a powerful tool for data manipulation. By mastering these techniques, users can enhance their scripting capabilities and improve the efficiency of their data processing tasks.
Author Profile
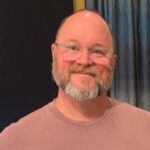
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?