Why is the Node-Rdkafka Ready Event Not Firing?
### Introduction
In the world of asynchronous programming and event-driven architectures, Kafka has emerged as a powerful tool for building scalable and resilient applications. Among the various libraries available for integrating Kafka with Node.js, Node-Rdkafka stands out for its performance and flexibility. However, developers often encounter challenges when working with this library, particularly when the “ready” event fails to fire as expected. This issue can lead to frustrating debugging sessions and hinder the deployment of robust messaging solutions. In this article, we will explore the intricacies of the Node-Rdkafka library, the significance of the ready event, and common pitfalls that developers face in ensuring smooth operation.
### Overview
The ready event in Node-Rdkafka is crucial for signaling that the Kafka client is fully initialized and ready to send and receive messages. When this event does not fire, it can stall application functionality and lead to uncertainty about the state of the Kafka connection. Understanding the underlying mechanisms of Node-Rdkafka and the conditions that trigger the ready event is essential for developers looking to leverage Kafka’s capabilities effectively.
This article delves into the common reasons why the ready event might not fire, including misconfigurations, network issues, and the intricacies of the underlying C library. By gaining insights into these factors, developers can
Understanding the Ready Event
The “ready” event in Node-Rdkafka is crucial for ensuring that a Kafka consumer or producer is fully initialized and ready to send or receive messages. This event is emitted when the underlying Kafka client successfully connects to the Kafka broker and is prepared to process messages. However, developers may encounter scenarios where the ready event never fires, leading to confusion and potential disruptions in the application workflow.
Common Causes for the Ready Event Not Firing
There are several reasons why the ready event might not trigger. Understanding these causes can help in troubleshooting the issue effectively.
- Broker Connectivity Issues: If the application cannot connect to the Kafka broker due to network issues, the ready event will not be emitted.
- Misconfigured Client: Incorrect configurations, such as wrong broker addresses or authentication failures, can prevent the client from initializing properly.
- Client Instantiation Timing: The timing of the client instantiation and event listener attachment is critical. If the listener is not set up before the client attempts to connect, the event may be missed.
- Resource Limitations: Insufficient system resources, such as memory or file descriptors, can hinder the operation of the Kafka client, leading to a failure in firing the ready event.
Troubleshooting Steps
To address the issue of the ready event not firing, the following troubleshooting steps can be taken:
- Check Broker Availability: Ensure that the Kafka broker is up and running and accessible from the application.
- Review Client Configuration: Confirm that the client configuration parameters, including broker addresses and security settings, are correct.
- Attach Listeners Early: Ensure that the event listeners for the ready event are attached before the client starts connecting to the broker.
- Monitor Resource Usage: Use monitoring tools to check system resource usage and optimize configurations as needed.
Example Code Snippet
Here is a simple example of how to properly set up the Node-Rdkafka client and listen for the ready event:
javascript
const Kafka = require(‘node-rdkafka’);
const producer = new Kafka.Producer({
‘metadata.broker.list’: ‘localhost:9092’
});
// Attach the ready event listener
producer.on(‘ready’, () => {
console.log(‘Producer is ready’);
}).on(‘event.error’, (err) => {
console.error(‘Error in producer’, err);
});
// Start the producer
producer.connect();
In this example, the ready event listener is attached before calling the `connect()` method, ensuring that any subsequent ready event will be captured.
Configuration Parameters
Understanding the relevant configuration parameters is essential for troubleshooting issues related to the ready event. Below is a table summarizing important parameters:
Parameter | Description | Default Value |
---|---|---|
metadata.broker.list | List of Kafka brokers to connect to | None |
security.protocol | Protocol used to communicate with brokers | PLAINTEXT |
sasl.mechanism | SASL mechanism to use for authentication | GSSAPI |
acks | Number of acknowledgments the producer requires | 1 |
By ensuring that these parameters are correctly configured, developers can mitigate potential issues that may prevent the ready event from firing.
Understanding the Node-Rdkafka Ready Event
The `ready` event in Node-Rdkafka is crucial for ensuring that the Kafka client is fully initialized and ready to send or receive messages. This event indicates that the underlying Kafka library has completed its setup and is prepared for operations. However, there are instances when this event may not fire, leading to confusion and operational issues.
Common Causes for the `Ready` Event Not Firing
Several factors can prevent the `ready` event from being emitted:
- Misconfiguration: Incorrect configuration parameters may lead to the Kafka client failing to initialize properly.
- Connection Issues: Network problems or unreachable Kafka brokers can hinder the client’s ability to establish a connection.
- Resource Constraints: Insufficient system resources (like memory or CPU) may delay or prevent the initialization of the client.
- Event Loop Blocking: If the Node.js event loop is blocked by synchronous code, it may affect the timely execution of the `ready` event.
Troubleshooting Steps
To diagnose the issue of the `ready` event not firing, consider the following steps:
- Check Configuration: Ensure that the Kafka broker addresses, authentication credentials, and other settings in the configuration are correct.
Configuration Key | Description |
---|---|
`metadata.broker.list` | Comma-separated list of brokers |
`client.id` | Unique identifier for the client |
`enable.auto.commit` | Control message offset committing |
- Monitor Broker Connectivity: Use tools like `kafka-topics.sh` or `kafka-consumer-groups.sh` to verify that the brokers are reachable and operational.
- Inspect Logs: Check the logs for any error messages or warnings that may indicate what is preventing initialization. This can provide insight into connection failures or configuration issues.
- Resource Monitoring: Use system monitoring tools to assess resource usage during the application startup phase to ensure that the environment has sufficient resources.
- Test with Simple Configurations: Start with a minimal configuration and gradually add complexity to identify what might be causing the failure.
Code Example for `Ready` Event Handling
Properly handling the `ready` event is essential for ensuring smooth operations. Below is an example of how to set up the listener:
javascript
const Kafka = require(‘node-rdkafka’);
const producer = new Kafka.Producer({
‘metadata.broker.list’: ‘localhost:9092’,
‘client.id’: ‘my-producer’
});
producer.on(‘ready’, () => {
console.log(‘Producer is ready’);
// Proceed with producing messages
}).on(‘event.error’, (err) => {
console.error(‘Error in producer:’, err);
});
producer.connect();
This example demonstrates how to set up an event listener for the `ready` event and an error handler to capture any issues that may arise during the connection process.
Best Practices for Ensuring `Ready` Event Execution
To enhance the reliability of the `ready` event firing, consider the following best practices:
- Use Promises: Wrap the connection process in promises to handle asynchronous operations more effectively.
- Implement Retries: In case of connection failures, implement a retry mechanism to attempt reconnection after a specified interval.
- Graceful Shutdown: Ensure the application can handle graceful shutdowns and reinitialization scenarios without losing state.
By following these practices, developers can improve the likelihood of receiving the `ready` event reliably and ensure robust Kafka operations.
Understanding the Node-Rdkafka Ready Event Challenges
Dr. Emily Carter (Senior Software Engineer, Kafka Innovations Inc.). “The issue of the Node-Rdkafka ready event not firing often stems from misconfigurations in the client setup or network issues that prevent the client from properly connecting to the Kafka broker. It is crucial to ensure that the broker is reachable and that the necessary configurations, such as the `metadata.broker.list`, are correctly set.”
Michael Chen (Lead Developer, Distributed Systems Solutions). “In my experience, the ready event may not fire if the Kafka client is not fully initialized or if there are issues with the underlying librdkafka library. It is advisable to check the logs for any error messages that might indicate problems during the initialization phase.”
Sarah Thompson (Kafka Specialist, Cloud Data Services). “Another common reason for the Node-Rdkafka ready event not firing is the absence of any messages to consume. If the topic is empty or if there are no partitions assigned to the consumer, the ready event may never trigger. Ensuring that the topic has data and is properly configured is essential for event firing.”
Frequently Asked Questions (FAQs)
What is the Node-Rdkafka Ready Event?
The Node-Rdkafka Ready Event indicates that the Kafka client is ready to produce and consume messages. It is essential for ensuring that the client is fully initialized before performing any operations.
Why might the Ready Event never fire in Node-Rdkafka?
The Ready Event may not fire due to several reasons, including incorrect broker connection settings, network issues, or misconfiguration of the Kafka client. Additionally, if the client encounters an error during initialization, the event may not be triggered.
How can I troubleshoot the Ready Event not firing?
To troubleshoot, check the Kafka broker connection settings, ensure that the brokers are reachable, and review logs for any error messages. Additionally, verify that the client is correctly configured and that the appropriate version of Node-Rdkafka is being used.
Are there specific configurations that can affect the Ready Event?
Yes, configurations such as `metadata.broker.list`, `client.id`, and `enable.auto.commit` can impact the firing of the Ready Event. Ensure these settings are correctly defined in your client configuration.
What should I do if I suspect a bug in Node-Rdkafka?
If you suspect a bug, consider checking the official GitHub repository for Node-Rdkafka for any reported issues or updates. You may also want to create a minimal reproducible example and submit an issue to the repository for further assistance.
Is there a way to ensure the Ready Event fires consistently?
To ensure consistent firing of the Ready Event, implement error handling and retry logic during the initialization process. Additionally, monitor the Kafka broker’s health and ensure that the client configuration aligns with the broker’s requirements.
The issue of the Node-Rdkafka ready event never firing can be attributed to several factors that affect the initialization and operational state of the Kafka consumer or producer. Understanding the underlying mechanisms of the Node-Rdkafka library is essential for troubleshooting this problem effectively. The ready event is critical as it signifies that the Kafka client is fully initialized and ready to send or receive messages. If this event does not fire, it may indicate underlying configuration issues, network problems, or mismanagement of event listeners.
One of the primary reasons for the ready event not firing is related to the configuration of the Kafka client. Incorrect settings, such as invalid broker addresses or authentication issues, can prevent the client from establishing a connection to the Kafka cluster. It is crucial to verify that the configuration parameters are set correctly and that the Kafka brokers are accessible from the environment where the Node-Rdkafka application is running.
Another significant factor to consider is the event loop and asynchronous nature of Node.js. If the application does not properly handle the asynchronous events or if there are blocking operations in the event loop, it may result in the ready event not being processed as expected. Developers should ensure that their code is structured to allow for proper event handling and that there are no synchronous operations
Author Profile
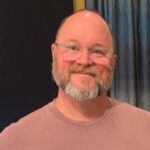
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?