How Can You Easily Retrieve All Products From the Cart in Magento 2?
In the dynamic world of e-commerce, understanding customer behavior is paramount for optimizing sales strategies and enhancing the shopping experience. For Magento 2 users, one of the key aspects of this understanding lies in effectively managing the shopping cart. The ability to retrieve all products from a user’s cart not only aids in inventory management but also opens doors to personalized marketing tactics and improved customer engagement. Whether you’re a developer looking to refine your Magento store or a business owner aiming to leverage data for better decision-making, mastering the process of extracting cart information is essential.
When it comes to Magento 2, the platform offers robust tools and features that allow for seamless interaction with the shopping cart. By accessing the cart’s contents, you can gain valuable insights into customer preferences, identify trends, and even tailor promotions to boost conversion rates. This capability is especially critical in a competitive landscape where every detail can influence a buyer’s journey.
In this article, we will delve into the methods and best practices for retrieving all products from the cart in Magento 2. We’ll explore the underlying architecture that supports these functionalities, as well as provide practical examples that can enhance your e-commerce operations. Whether you’re troubleshooting issues or looking to implement new features, understanding how to effectively manage cart data will empower you
Accessing the Cart in Magento 2
To retrieve all products from the cart in Magento 2, you first need to access the cart object. Magento provides a structured way to interact with the cart through its service contracts and repositories. The cart is typically accessed through the `Quote` model, which represents the shopping cart for a customer.
You can access the cart using dependency injection in your custom module or through a controller. Here’s how you can do it in a custom class:
“`php
use Magento\Checkout\Model\Cart as CheckoutCart;
class YourClassName
{
protected $checkoutCart;
public function __construct(CheckoutCart $checkoutCart)
{
$this->checkoutCart = $checkoutCart;
}
public function getCartItems()
{
return $this->checkoutCart->getQuote()->getAllItems();
}
}
“`
This code snippet demonstrates how to inject the `CheckoutCart` class and retrieve all items from the cart.
Retrieving Product Data from the Cart
Once you have access to the cart items, you can extract detailed information about each product. The `getAllItems()` method returns an array of `QuoteItem` objects, which contain various attributes of the products added to the cart.
To extract and display product data, you can loop through the items and access their properties. Here’s an example:
“`php
$cartItems = $this->getCartItems();
foreach ($cartItems as $item) {
echo ‘Product ID: ‘ . $item->getProductId() . ‘
‘;
echo ‘Name: ‘ . $item->getName() . ‘
‘;
echo ‘Quantity: ‘ . $item->getQty() . ‘
‘;
echo ‘Price: ‘ . $item->getPrice() . ‘
‘;
}
“`
Data Structure of Cart Items
The properties of each `QuoteItem` can include but are not limited to:
- Product ID
- Name
- Quantity
- Price
- SKU
- Weight
- Options (custom options)
Below is a table summarizing key attributes of a cart item:
Attribute | Description |
---|---|
Product ID | Unique identifier for the product. |
Name | The name of the product as it appears on the site. |
Quantity | The number of units of the product in the cart. |
Price | The price of the product at the time of addition to the cart. |
SKU | Stock Keeping Unit, a unique identifier for inventory. |
Weight | The weight of the product, important for shipping calculations. |
Options | Any custom options selected by the customer for the product. |
By leveraging these methods and properties, developers can effectively manage and display the contents of the shopping cart within Magento 2, ensuring a smooth user experience.
Magento 2 Get All Products From Cart
To retrieve all products from the cart in Magento 2, you can utilize the `\Magento\Checkout\Model\Session` class, which provides access to the current customer’s cart. Here’s how to implement this functionality in a custom module or script.
Step-by-Step Implementation
- **Inject the Checkout Session**: First, you need to inject the `Checkout\Session` class into your custom class or controller.
“`php
use Magento\Checkout\Model\Session as CheckoutSession;
class YourClass
{
protected $checkoutSession;
public function __construct(CheckoutSession $checkoutSession)
{
$this->checkoutSession = $checkoutSession;
}
}
“`
- **Get the Cart Items**: Once you have access to the checkout session, you can retrieve the cart items by calling the `getQuote()` method.
“`php
$quote = $this->checkoutSession->getQuote();
$items = $quote->getAllItems();
“`
- **Extract Product Data**: Loop through the cart items to extract relevant product information such as ID, name, and quantity.
“`php
foreach ($items as $item) {
$productId = $item->getProductId();
$productName = $item->getName();
$qty = $item->getQty();
// Further processing or storage of product information
}
“`
Complete Example
Here’s a complete example that combines the above steps. This script can be included in a custom block or controller.
“`php
namespace Vendor\Module\Controller\Cart;
use Magento\Checkout\Model\Session as CheckoutSession;
use Magento\Framework\App\Action\Action;
use Magento\Framework\App\Action\Context;
class GetCartItems extends Action
{
protected $checkoutSession;
public function __construct(Context $context, CheckoutSession $checkoutSession)
{
parent::__construct($context);
$this->checkoutSession = $checkoutSession;
}
public function execute()
{
$quote = $this->checkoutSession->getQuote();
$items = $quote->getAllItems();
$cartItems = [];
foreach ($items as $item) {
$cartItems[] = [
‘product_id’ => $item->getProductId(),
‘product_name’ => $item->getName(),
‘quantity’ => $item->getQty(),
];
}
// Output or return the cart items as needed
return $this->jsonResponse($cartItems);
}
protected function jsonResponse($data)
{
$this->getResponse()->representJson(json_encode($data));
}
}
“`
Additional Considerations
- Handling Configurable Products: If you are working with configurable products, you may want to retrieve the associated child product IDs and attributes.
- Performance Optimization: When dealing with large carts, consider implementing pagination or limiting the number of items processed to maintain performance.
- Error Handling: Implement error handling to manage cases where the quote might not exist or other exceptions that could arise during the retrieval process.
- Testing: Ensure thorough testing of your implementation across different scenarios, including empty carts, different product types, and user sessions.
By following these steps, you can efficiently retrieve and manipulate cart items in Magento 2.
Expert Insights on Retrieving All Products from Magento 2 Cart
Jessica Tran (E-commerce Solutions Architect, TechCommerce Inc.). “To effectively retrieve all products from a Magento 2 cart, developers should leverage the `\Magento\Checkout\Model\Cart` class. This class provides a straightforward method to access the cart items, ensuring that you can manipulate and display product data efficiently.”
Michael Chen (Magento Certified Developer, EcomExperts). “Utilizing the `getQuote()->getAllItems()` method is essential for obtaining a comprehensive list of products in the cart. This approach guarantees that you capture all relevant details, including product attributes, which can be crucial for custom functionalities.”
Sarah Patel (Senior Magento Consultant, Digital Commerce Solutions). “When working with Magento 2, it is important to consider performance implications when retrieving cart data. Implementing caching strategies and optimizing database queries can significantly enhance the efficiency of fetching all products from the cart.”
Frequently Asked Questions (FAQs)
How can I retrieve all products from the cart in Magento 2?
To retrieve all products from the cart in Magento 2, you can use the `\Magento\Checkout\Model\Cart` class. By calling the `getQuote()` method, you can access the cart’s quote and then use `getAllItems()` to get the list of all products in the cart.
What is the difference between a cart and a quote in Magento 2?
In Magento 2, a cart refers to the user’s current selection of products for purchase, while a quote represents the cart’s underlying data structure that contains information about the items, pricing, and customer details. The quote is persisted in the database, allowing for session management.
Can I get product details along with the cart items in Magento 2?
Yes, you can retrieve product details along with the cart items by loading each product’s data using the product IDs obtained from the cart items. Utilize the `\Magento\Catalog\Model\ProductRepository` to fetch detailed information about each product.
Is there a way to filter products in the cart based on specific criteria?
Yes, you can filter products in the cart by iterating through the items returned by `getAllItems()` and applying your specific criteria, such as SKU, price, or product type, to create a filtered list.
How do I access the cart items programmatically in Magento 2?
To access the cart items programmatically, you can inject the `\Magento\Checkout\Model\Cart` class into your custom class or controller and then call the `getQuote()->getAllItems()` method to retrieve the items.
What happens if the cart is empty when trying to retrieve products?
If the cart is empty, calling `getAllItems()` will return an empty array. It is essential to check if the cart contains items before attempting to process them to avoid errors in your code.
In summary, retrieving all products from the cart in Magento 2 is an essential task for developers and store owners who wish to manage the shopping experience effectively. Magento 2 provides a robust framework that allows for easy access to cart contents through its API and built-in classes. By utilizing the `\Magento\Checkout\Model\Cart` class, developers can programmatically obtain a list of all items currently in the customer’s cart, facilitating various functionalities such as inventory checks, promotional offers, and personalized marketing strategies.
Moreover, understanding the structure of cart items is crucial for effective data handling. Each item in the cart contains vital information such as product ID, quantity, price, and options. This enables developers to create tailored experiences based on the products customers are interested in. Additionally, leveraging Magento’s event-driven architecture can help in tracking cart changes and responding to customer behavior in real-time, enhancing overall user engagement.
Key takeaways include the importance of utilizing Magento 2’s built-in methods for accessing cart data, which not only simplifies the process but also ensures compatibility with future updates. Furthermore, developers should consider implementing proper error handling and data validation to maintain a seamless shopping experience. Overall, a thorough understanding of how to get all products from the cart
Author Profile
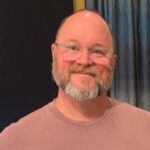
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?