How Can You Easily Print a List in Python?
How To Print A List In Python
In the world of programming, Python stands out as a versatile and user-friendly language that caters to both beginners and seasoned developers alike. One of the fundamental tasks in any programming journey is learning how to effectively display information. Lists, a core data structure in Python, allow you to store multiple items in a single variable, making them indispensable for managing collections of data. Whether you’re working on a simple project or a complex application, knowing how to print a list in Python is an essential skill that can enhance your coding efficiency and clarity.
Printing a list in Python is more than just a straightforward command; it opens the door to a variety of methods and formatting options that can help you present your data in meaningful ways. From displaying raw data to customizing the output for better readability, understanding the nuances of list printing can significantly improve how you communicate information through your code. As you delve into this topic, you’ll discover not only the basic techniques but also some advanced strategies that can elevate your programming prowess.
As we explore the various ways to print lists in Python, you’ll learn about the built-in functions and methods that make this task simple yet powerful. We’ll also touch on best practices for formatting and presenting your lists, ensuring that your output is not only functional but
Using the Print Function
The most straightforward way to print a list in Python is by using the built-in `print()` function. When you pass a list to this function, it converts the list into a string representation and displays it in the console.
“`python
my_list = [1, 2, 3, 4, 5]
print(my_list)
“`
This will output:
“`
[1, 2, 3, 4, 5]
“`
While this method is effective for displaying the entire list, it may not be suitable for all scenarios, especially if you need a more formatted output.
Iterating Through a List
If you require more control over how the elements are displayed, you can iterate through the list using a loop. This method allows you to format each element individually.
“`python
for item in my_list:
print(item)
“`
This will produce the following output:
“`
1
2
3
4
5
“`
By iterating through the list, you can also customize the output further. For example, you can add separators or additional formatting.
Joining List Elements into a String
When you want to print list elements as a single string, the `join()` method is particularly useful. This method concatenates the elements of the list with a specified separator.
“`python
string_list = [‘apple’, ‘banana’, ‘cherry’]
print(“, “.join(string_list))
“`
The output will be:
“`
apple, banana, cherry
“`
This approach is especially handy for creating readable strings from lists of strings.
Formatted Printing with f-Strings
For more complex formatting, Python’s f-strings (available from Python 3.6 onwards) allow you to embed expressions inside string literals. This can be useful when you want to display additional context along with the list elements.
“`python
numbers = [1, 2, 3]
print(f”The numbers are: {‘, ‘.join(map(str, numbers))}.”)
“`
The result will be:
“`
The numbers are: 1, 2, 3.
“`
Using the PrettyTable Library
For tabular representations, the `PrettyTable` library can be very effective. It allows you to create visually appealing tables from lists, which can be particularly useful for displaying data in a structured format.
First, you must install the library if it is not already available:
“`bash
pip install prettytable
“`
Then you can use it as follows:
“`python
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = [“Index”, “Value”]
for index, value in enumerate(my_list):
table.add_row([index, value])
print(table)
“`
This will produce a table like the following:
Index | Value |
---|---|
0 | 1 |
1 | 2 |
2 | 3 |
3 | 4 |
4 | 5 |
Using these methods, you can effectively print lists in various formats, depending on your specific needs and preferences.
Using the print() Function
The simplest way to print a list in Python is by utilizing the built-in `print()` function. This function can handle various data types, including lists.
“`python
my_list = [1, 2, 3, 4, 5]
print(my_list)
“`
This code will output:
“`
[1, 2, 3, 4, 5]
“`
The output includes the brackets, indicating that it is a list.
Printing List Elements Individually
If you prefer to print each element on a separate line, you can use a loop. The `for` loop iterates through the list, allowing individual items to be printed.
“`python
for item in my_list:
print(item)
“`
This will produce:
“`
1
2
3
4
5
“`
Joining List Elements into a String
To print list elements as a single string, you can use the `join()` method. This method requires a separator string and is particularly useful for lists of strings.
“`python
string_list = [“apple”, “banana”, “cherry”]
result = “, “.join(string_list)
print(result)
“`
The output will be:
“`
apple, banana, cherry
“`
Formatted Printing with f-strings
For enhanced formatting, especially when dealing with lists of complex items, Python’s f-strings provide a concise way to include list elements within a formatted string.
“`python
numbers = [10, 20, 30]
print(f”The numbers are: {‘, ‘.join(map(str, numbers))}.”)
“`
This will display:
“`
The numbers are: 10, 20, 30.
“`
Using List Comprehensions for Custom Formatting
List comprehensions can also be employed to format or manipulate list items before printing. This approach allows for greater customization.
“`python
squared_numbers = [x**2 for x in my_list]
print(“Squared values:”, ‘, ‘.join(map(str, squared_numbers)))
“`
The output will be:
“`
Squared values: 1, 4, 9, 16, 25
“`
Printing Lists with Enumerate for Indexing
If you need to print both the index and the value of list elements, use the `enumerate()` function. This function returns pairs of indices and values.
“`python
for index, value in enumerate(my_list):
print(f”Index {index}: {value}”)
“`
This will yield:
“`
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
“`
Summary of Printing Methods
Method | Description |
---|---|
`print()` | Basic printing of the entire list |
`for` loop | Prints each element on a new line |
`join()` method | Combines elements into a single string with a separator |
f-strings | Formats output with embedded expressions |
List comprehensions | Customizes item formatting before printing |
`enumerate()` | Displays index along with values |
These methods provide various ways to print lists in Python, catering to different requirements and preferences.
Expert Insights on Printing Lists in Python
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). “Printing a list in Python is straightforward, but understanding the nuances of list formatting can significantly enhance the readability of your output. Utilizing the `join()` method for string lists or employing list comprehensions can make your printed output more elegant and informative.”
Michael Thompson (Lead Python Developer, CodeCraft Solutions). “When printing lists in Python, it’s crucial to consider the data types within the list. Using the `print()` function directly is effective for simple lists, but for more complex structures, employing the `pprint` module can help in presenting the data in a more structured format.”
Sarah Lee (Data Scientist, Analytics Hub). “For data analysis tasks, printing lists with additional context is vital. Utilizing formatted strings or f-strings in Python can provide clarity when displaying list contents, especially when dealing with large datasets or when integrating with other output formats.”
Frequently Asked Questions (FAQs)
How do I print a simple list in Python?
You can print a simple list in Python using the `print()` function. For example, `print(my_list)` where `my_list` is the variable containing your list.
Can I format the output when printing a list?
Yes, you can format the output by using string formatting methods such as f-strings or the `format()` method. For instance, `print(f”My list: {my_list}”)` or `print(“My list: {}”.format(my_list))` will allow for customized output.
How do I print each item in a list on a new line?
To print each item on a new line, you can use a loop. For example, `for item in my_list: print(item)` will iterate through the list and print each item individually.
Is it possible to print a list with a specific separator?
Yes, you can use the `join()` method to print a list with a specific separator. For example, `print(“, “.join(my_list))` will print the items in the list separated by commas.
What if my list contains non-string elements?
If your list contains non-string elements, you need to convert them to strings before printing. You can do this using a list comprehension: `print(“, “.join(str(item) for item in my_list))`.
Can I print a list in reverse order?
Yes, you can print a list in reverse order by using slicing or the `reversed()` function. For example, `print(my_list[::-1])` or `print(list(reversed(my_list)))` will display the list elements in reverse.
In summary, printing a list in Python is a straightforward process that can be accomplished using various methods. The most common approach is to use the built-in `print()` function, which outputs the entire list in a readable format. Additionally, Python provides flexibility through list comprehensions and the `join()` method, allowing for customized formatting of list elements when printed. Understanding these methods is essential for effective data presentation in Python programming.
Moreover, developers can enhance their output by utilizing loops to print each element of the list individually, which can be particularly useful for lists containing complex data structures. This approach not only improves readability but also allows for additional formatting options, such as adding separators or labels. Leveraging these techniques can significantly improve the clarity of the output, making it easier for users to interpret the information presented.
Ultimately, mastering the various ways to print a list in Python contributes to better coding practices and enhances the overall user experience. By understanding the nuances of list printing, programmers can effectively communicate data and insights, which is a fundamental skill in data analysis and software development. As such, incorporating these techniques into your programming toolkit will undoubtedly lead to more efficient and effective code.
Author Profile
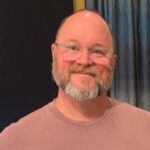
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?