How Can You Effectively Speed Up Your Python Code?
In the ever-evolving world of programming, efficiency is key. As Python continues to gain popularity for its simplicity and versatility, developers often find themselves grappling with performance issues that can slow down their applications. Whether you’re working on a data analysis project, a web application, or a machine learning model, knowing how to speed up your Python code can be the difference between a sluggish experience and a seamless one. In this article, we will explore various strategies and best practices that can help you optimize your Python code, making it faster and more efficient without sacrificing readability.
Python is renowned for its ease of use, but this can sometimes come at the cost of performance. Many developers encounter bottlenecks in their code that can lead to frustrating delays, especially when handling large datasets or complex algorithms. Fortunately, there are numerous techniques available to enhance the speed of your Python programs. From leveraging built-in functions and libraries to employing advanced algorithms and data structures, the possibilities for optimization are vast and varied.
As we delve into the intricacies of Python performance, we will cover essential concepts that every programmer should consider. You’ll learn about the importance of profiling your code to identify slow spots, the benefits of using efficient libraries like NumPy and Pandas, and how to write cleaner, more
Optimize Algorithms and Data Structures
Choosing the right algorithm and data structure is fundamental for speeding up Python code. Efficient algorithms reduce the number of operations and optimize performance. Here are some strategies to consider:
- Use Built-in Functions: Python’s built-in functions are implemented in C and optimized for performance. Utilizing functions like `sum()`, `min()`, and `max()` can lead to significant speed improvements compared to manual implementations.
- Choose Appropriate Data Structures: Selecting the right data structure can dramatically affect performance. For example:
- Use lists for ordered collections.
- Use sets for membership tests.
- Use dictionaries for key-value pairs.
- Algorithm Complexity: Always analyze the time and space complexity of your algorithms. Aim for lower complexity classes (e.g., O(n log n) is better than O(n²)).
Utilize Caching and Memoization
Caching is a technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. This can drastically reduce computation time in scenarios involving repeated calculations.
- Memoization: This is a specific form of caching used for functions. Implement it using decorators, such as `functools.lru_cache`. This method allows Python to cache results based on input parameters.
Example of memoization:
“`python
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
```
Profile Your Code
Profiling is essential for identifying bottlenecks in your Python code. Tools such as cProfile and line_profiler help you understand where your program spends most of its time.
- cProfile: This built-in module provides a way to analyze the performance of your program.
- line_profiler: This tool allows you to measure the time spent on each individual line of code.
Using these profilers will help you pinpoint areas that require optimization.
Reduce the Use of Global Variables
Global variables can slow down your code due to the way Python handles variable scope. Accessing global variables is generally slower than accessing local variables.
- Encapsulate Variables: Where possible, encapsulate variables within functions or classes to enhance performance.
- Limit Global Scope: Minimize the use of global variables to avoid performance penalties associated with scope resolution.
Leverage Multiprocessing and Threading
For CPU-bound tasks, consider using the `multiprocessing` module. This allows you to bypass Python’s Global Interpreter Lock (GIL) by creating separate memory spaces for processes.
- Multiprocessing: Best suited for tasks that require heavy computations. It utilizes multiple CPU cores effectively.
- Threading: More appropriate for I/O-bound tasks, where the program spends time waiting for external resources.
Example of using multiprocessing:
“`python
from multiprocessing import Pool
def square(x):
return x * x
if __name__ == ‘__main__’:
with Pool(processes=4) as pool:
results = pool.map(square, range(10))
“`
Table: Performance Improvement Techniques
Technique | Description | Use Case |
---|---|---|
Built-in Functions | Utilize optimized built-in functions for speed | Data processing |
Memoization | Cache results of expensive function calls | Recursive functions |
Profiling | Analyze code to identify bottlenecks | Performance tuning |
Encapsulate Variables | Limit the use of global variables | General coding practices |
Multiprocessing | Use multiple processes for CPU-bound tasks | Heavy computations |
Optimize Your Algorithms
Choosing the right algorithm can dramatically affect performance. Consider the following points to optimize your algorithms:
- Select Efficient Data Structures: Utilize data structures that enhance speed, such as:
- Lists for ordered collections.
- Sets for membership tests.
- Dictionaries for key-value pairs.
- Algorithm Complexity: Analyze the time complexity of algorithms. Prefer algorithms with lower complexity. For example:
- Use QuickSort (O(n log n)) instead of Bubble Sort (O(n^2)).
- Opt for binary search (O(log n)) over linear search (O(n)).
Utilize Built-in Functions and Libraries
Python’s built-in functions are implemented in C and optimized for performance. Rely on them whenever possible, such as:
- Map and Filter: Use `map()` and `filter()` instead of list comprehensions for large datasets.
- NumPy: For numerical computations, leverage the NumPy library, which is optimized for speed and memory usage.
Function | Description | Performance Benefit |
---|---|---|
`sum()` | Sum items in an iterable | Faster than manual loops |
`sorted()` | Sorts an iterable | Optimized sorting |
`any()` | Check if any element is true in an iterable | Faster short-circuiting |
Profile Your Code
Identifying bottlenecks is crucial for optimization. Use profiling tools to analyze where time is spent in your code:
- cProfile: A built-in module that provides a detailed report of function call times.
- line_profiler: Allows line-by-line analysis for finer granularity.
By profiling, you can focus on optimizing the most time-consuming parts of your code.
Minimize Global Variable Access
Accessing global variables is slower than accessing local variables. To enhance performance:
- Pass Variables: Use function arguments instead of relying on global variables.
- Local Caching: Store frequently accessed global variables in local variables within functions.
Use List Comprehensions Wisely
List comprehensions can be more efficient than traditional loops. However, use them judiciously:
- Simple Transformations: Prefer list comprehensions for straightforward transformations.
- Avoid Complex Conditions: If conditions are too complex, consider using regular loops for readability and maintainability.
Implement Caching Techniques
Caching can significantly reduce redundant calculations. Consider the following methods:
- Memoization: Store the results of expensive function calls and return the cached result when the same inputs occur again.
“`python
def memoize(func):
cache = {}
def wrapper(*args):
if args not in cache:
cache[args] = func(*args)
return cache[args]
return wrapper
“`
- Using `functools.lru_cache`: A built-in decorator to cache function results.
Concurrency and Parallelism
If your application is CPU-bound, consider using concurrency and parallelism techniques:
- Threading: Use the `threading` module for I/O-bound tasks to improve efficiency.
- Multiprocessing: Utilize the `multiprocessing` module to bypass the Global Interpreter Lock (GIL) for CPU-bound tasks.
Method | Best Use Case | Module |
---|---|---|
Threading | I/O-bound tasks | `threading` |
Multiprocessing | CPU-bound tasks | `multiprocessing` |
Optimize Loops
Loop optimization can lead to significant performance improvements:
- Avoid Unnecessary Computations: Move computations outside of loops whenever possible.
- Use Generator Expressions: For large datasets, use generators to reduce memory overhead.
Following these techniques will help streamline Python code, enhancing performance and efficiency.
Strategies for Accelerating Python Performance
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively speed up Python code, leveraging built-in functions and libraries such as NumPy can significantly enhance performance due to their implementation in C, which is much faster than pure Python loops.”
Michael Chen (Data Scientist, Analytics Hub). “Profiling your code using tools like cProfile or line_profiler is crucial. By identifying bottlenecks, you can focus your optimization efforts where they will have the most impact, leading to substantial performance improvements.”
Sarah Patel (Python Performance Consultant, CodeOptima). “Utilizing concurrency and parallelism through libraries such as asyncio or multiprocessing can drastically reduce execution time for I/O-bound or CPU-bound tasks, making your Python applications more efficient.”
Frequently Asked Questions (FAQs)
How can I improve the performance of my Python code?
You can enhance performance by optimizing algorithms, using built-in functions, minimizing the use of global variables, and employing efficient data structures like sets and dictionaries.
What are some common libraries to speed up Python code?
Libraries such as NumPy for numerical computations, Cython for compiling Python to C, and Numba for just-in-time compilation can significantly accelerate execution speed.
Is it beneficial to use multithreading or multiprocessing in Python?
Yes, multithreading can improve I/O-bound tasks, while multiprocessing is effective for CPU-bound tasks. Both approaches can leverage multiple cores to enhance performance.
How does code profiling help in speeding up Python code?
Code profiling identifies bottlenecks in your code, allowing you to focus optimization efforts on the most time-consuming sections, ultimately improving overall performance.
What role does algorithm complexity play in Python performance?
Algorithm complexity directly impacts performance; choosing algorithms with lower time and space complexity can lead to significant speed improvements, especially with large datasets.
Can using a different Python implementation improve speed?
Yes, alternative implementations like PyPy, which features a JIT compiler, can execute Python code faster than the standard CPython, particularly for long-running applications.
In summary, speeding up Python code involves a multifaceted approach that encompasses optimizing algorithms, utilizing efficient data structures, and leveraging built-in functions. By carefully analyzing the time complexity of your code and selecting the most appropriate algorithms for the task at hand, significant performance improvements can be achieved. Additionally, understanding the strengths and weaknesses of various data structures can lead to more efficient memory usage and faster execution times.
Another key strategy for enhancing Python performance is to utilize libraries and tools that are specifically designed for optimization. Libraries such as NumPy and Pandas provide optimized implementations of common operations, allowing for faster computations, especially when dealing with large datasets. Furthermore, employing techniques like Just-In-Time (JIT) compilation through tools like Numba can dramatically reduce execution time for numerically intensive tasks.
Lastly, profiling and benchmarking your code is essential for identifying bottlenecks and understanding where optimizations will have the most impact. By using profiling tools, developers can gain insights into which parts of their code are consuming the most resources, enabling targeted improvements. a combination of algorithmic efficiency, appropriate data structures, the use of optimized libraries, and thorough profiling can significantly enhance the performance of Python code.
Author Profile
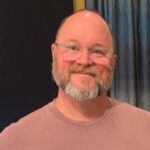
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?