How Can You Easily Create a CSV File in Python?
In the world of data management and analysis, CSV (Comma-Separated Values) files have become a staple format for storing and exchanging information. Whether you’re a data analyst, a software developer, or simply someone looking to organize your data efficiently, mastering the art of creating CSV files in Python is an invaluable skill. With its user-friendly syntax and powerful libraries, Python makes it easier than ever to generate, manipulate, and export data in this widely-used format.
Creating a CSV file in Python not only streamlines your workflow but also enhances data interoperability across various platforms and applications. This versatile format can handle everything from simple lists to complex datasets, making it an essential tool for anyone working with data. In this article, we will explore the fundamental techniques for generating CSV files, including the use of built-in libraries and best practices to ensure your data is well-structured and easily accessible.
As we delve deeper, you’ll discover the straightforward methods to create CSV files, the nuances of handling different data types, and tips for ensuring your files are both readable and efficient. Whether you’re looking to automate data exports or simply want to keep your information organized, understanding how to create CSV files in Python will empower you to take control of your data and enhance your productivity. Get ready to unlock the potential of
Using the CSV Module
The most straightforward way to create a CSV file in Python is by utilizing the built-in `csv` module. This module provides functionality to both read from and write to CSV files, making it an essential tool for data manipulation.
To create a CSV file, you can follow these steps:
- Import the CSV module: This allows you to access the functions provided by the module.
- Open a file in write mode: You need to specify the file name and the mode in which you want to open the file.
- Create a CSV writer object: This object will handle the writing of data to the file.
- Write data to the CSV file: You can write data as a list or as rows.
Here is an example code snippet that illustrates this process:
python
import csv
# Data to be written to the CSV file
data = [
[‘Name’, ‘Age’, ‘City’],
[‘Alice’, 30, ‘New York’],
[‘Bob’, 25, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]
]
# Creating a CSV file
with open(‘people.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
In the example above, the `newline=”` argument is used to prevent additional blank lines in the output on certain platforms.
Writing Dictionaries to CSV
Another common scenario involves writing dictionaries to a CSV file, which can be accomplished using the `csv.DictWriter` class. This approach allows you to specify the header row based on the keys of the dictionaries.
Here’s how to do it:
- Define the fieldnames: These will be the keys used in your dictionaries.
- Create a DictWriter object: This will allow writing dictionaries directly to the CSV file.
- Write the header and the data: Use the `writeheader()` method to write the fieldnames, followed by writing each dictionary.
Here’s an example:
python
import csv
# List of dictionaries to be written to the CSV file
data = [
{‘Name’: ‘Alice’, ‘Age’: 30, ‘City’: ‘New York’},
{‘Name’: ‘Bob’, ‘Age’: 25, ‘City’: ‘Los Angeles’},
{‘Name’: ‘Charlie’, ‘Age’: 35, ‘City’: ‘Chicago’}
]
# Creating a CSV file
with open(‘people_dict.csv’, mode=’w’, newline=”) as file:
fieldnames = [‘Name’, ‘Age’, ‘City’]
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader() # Write the header
writer.writerows(data) # Write the data
Handling Different Delimiters
CSV files typically use commas as delimiters, but there are cases where other delimiters, such as semicolons or tabs, are needed. The `csv` module allows you to specify a different delimiter by passing the `delimiter` parameter when creating a `csv.writer` or `csv.DictWriter` object.
Example for a semicolon delimiter:
python
import csv
data = [
[‘Name’, ‘Age’, ‘City’],
[‘Alice’, 30, ‘New York’],
[‘Bob’, 25, ‘Los Angeles’]
]
with open(‘people_semicolon.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file, delimiter=’;’)
writer.writerows(data)
Example Data in a Table Format
To illustrate the data structure used in the examples, consider the following table:
Name | Age | City |
---|---|---|
Alice | 30 | New York |
Bob | 25 | Los Angeles |
Charlie | 35 | Chicago |
By following these guidelines, you can effectively create and manipulate CSV files in Python to suit various data management needs.
Creating a CSV File Using Python’s Built-in CSV Module
Python provides a built-in `csv` module that simplifies the process of creating and reading CSV files. This module facilitates handling CSV data and ensures proper formatting.
To create a CSV file, follow these steps:
- Import the CSV Module: Begin by importing the `csv` module.
- Open a File for Writing: Use the `open()` function to create a new CSV file or overwrite an existing one.
- Create a CSV Writer Object: Utilize `csv.writer()` to create a writer object that will handle the writing of data to the file.
- Write Data: Use methods such as `writerow()` or `writerows()` to write single or multiple rows of data.
Here’s a code example demonstrating these steps:
python
import csv
# Data to be written
data = [
[‘Name’, ‘Age’, ‘City’],
[‘Alice’, 30, ‘New York’],
[‘Bob’, 25, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]
]
# Creating a CSV file
with open(‘people.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
Customizing CSV Output
When creating CSV files, you might want to customize the output format. The `csv.writer()` function allows for various configurations:
- Delimiter: Change the default comma delimiter to another character (e.g., semicolon).
- Quote Character: Specify how to handle quoting of strings.
- Line Terminator: Adjust how lines are terminated in the file.
Example of customizing the delimiter:
python
with open(‘people_semicolon.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file, delimiter=’;’)
writer.writerows(data)
Writing Dictionaries to a CSV File
When dealing with more complex data structures, such as dictionaries, the `csv.DictWriter` class is particularly useful. It allows you to write rows as dictionaries, mapping keys to column names.
To write a dictionary to a CSV file:
- Create a Dictionary: Prepare your data in dictionary format.
- Define Fieldnames: Specify the order of columns.
- Use DictWriter: Instantiate `csv.DictWriter` and write the header and rows.
Example:
python
data_dict = [
{‘Name’: ‘Alice’, ‘Age’: 30, ‘City’: ‘New York’},
{‘Name’: ‘Bob’, ‘Age’: 25, ‘City’: ‘Los Angeles’},
{‘Name’: ‘Charlie’, ‘Age’: 35, ‘City’: ‘Chicago’}
]
with open(‘people_dict.csv’, mode=’w’, newline=”) as file:
fieldnames = [‘Name’, ‘Age’, ‘City’]
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(data_dict)
Handling Special Characters
When writing CSV files, special characters (e.g., commas, quotes) can interfere with the CSV format. The `csv` module handles these cases by enclosing fields containing special characters in quotes.
If you want to ensure proper handling of special characters, you can set the quoting parameter in the `csv.writer()` or `csv.DictWriter()`:
python
writer = csv.writer(file, quoting=csv.QUOTE_MINIMAL)
This ensures that only fields that contain special characters are quoted, maintaining clean output.
Creating CSV files in Python is a straightforward process using the `csv` module. By customizing delimiters and utilizing `DictWriter`, you can effectively manage various data formats and structures.
Expert Insights on Creating CSV Files in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Creating CSV files in Python is a fundamental skill for any data professional. Utilizing the built-in `csv` module allows for efficient data handling and ensures compatibility with various data analysis tools. It is essential to understand how to write both headers and rows correctly to maintain data integrity.”
Michael Chen (Software Engineer, Data Solutions Group). “When generating CSV files in Python, leveraging the `pandas` library can significantly simplify the process. It provides powerful data manipulation capabilities and allows for seamless export of DataFrames to CSV format, making it a preferred choice for many developers working with large datasets.”
Sarah Patel (Python Developer, CodeCraft Academy). “For beginners, understanding the nuances of CSV file creation in Python is crucial. It is important to handle exceptions and ensure proper encoding to avoid common pitfalls such as data loss or corruption. A thorough grasp of these concepts lays a solid foundation for more advanced data processing tasks.”
Frequently Asked Questions (FAQs)
How do I create a CSV file in Python?
To create a CSV file in Python, you can use the built-in `csv` module. First, open a file in write mode using `open()`, then create a `csv.writer` object and use the `writerow()` or `writerows()` methods to write data to the file.
What is the purpose of the `csv` module in Python?
The `csv` module in Python provides functionality to read from and write to CSV files. It simplifies the process of handling comma-separated values and allows for easy data manipulation and storage.
Can I write a list of dictionaries to a CSV file in Python?
Yes, you can write a list of dictionaries to a CSV file using the `csv.DictWriter` class. This allows you to specify the fieldnames and write each dictionary as a row in the CSV file.
What is the difference between `writerow()` and `writerows()` in Python’s `csv` module?
The `writerow()` method writes a single row to the CSV file, while `writerows()` writes multiple rows at once. Use `writerows()` when you have a list of iterable items to write.
How can I specify a different delimiter when creating a CSV file?
You can specify a different delimiter by passing the `delimiter` parameter to the `csv.writer()` or `csv.DictWriter()` constructor. For example, use `delimiter=’;’` to use a semicolon instead of a comma.
Is it possible to append data to an existing CSV file in Python?
Yes, you can append data to an existing CSV file by opening the file in append mode (`’a’`) instead of write mode (`’w’`). This allows you to add new rows without overwriting the existing content.
Creating a CSV file in Python is a straightforward process that leverages the built-in capabilities of the language, particularly through the use of the `csv` module. This module provides functionality for both reading from and writing to CSV files, making it a versatile tool for data manipulation. By utilizing the `csv.writer` class, users can easily format their data into a structured CSV format, which is widely used for data storage and exchange.
One of the key insights from the discussion is the importance of understanding the structure of CSV files. A CSV file consists of rows and columns, where each row represents a record and each column represents a field within that record. When creating a CSV file, it is essential to ensure that the data is organized correctly to maintain clarity and usability. Additionally, handling special characters, such as commas within the data, requires careful attention to prevent data corruption.
Another significant takeaway is the flexibility provided by Python in terms of data types and sources. Python can easily convert various data structures, such as lists and dictionaries, into CSV format. This adaptability allows for seamless integration with other data sources, such as databases or APIs, making Python a powerful tool for data processing tasks. Overall, mastering the creation of CSV files in Python
Author Profile
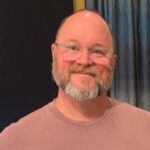
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?