How Can You Easily Convert XLSX to CSV Using JavaScript?
In today’s data-driven world, the ability to seamlessly convert files from one format to another is essential for efficient data management and analysis. Among the various file types, Excel spreadsheets (XLSX) and Comma-Separated Values (CSV) files stand out as two of the most commonly used formats. While XLSX files are rich with features, including formulas, charts, and multiple sheets, CSV files offer a simpler, more universally compatible way to handle data, especially for applications that require straightforward data manipulation. This article delves into the process of converting XLSX files to CSV using JavaScript, a powerful tool that can streamline this task for developers and data analysts alike.
The conversion process from XLSX to CSV is not just a matter of changing file extensions; it involves transforming structured data into a plain text format that can be easily read and processed by various applications. JavaScript provides several libraries and methods that simplify this conversion, allowing users to handle large datasets efficiently. Understanding the nuances of this conversion is crucial for anyone looking to integrate data from Excel into web applications or databases, where CSV is often the preferred format due to its simplicity and compatibility.
As we explore the techniques and tools available for converting XLSX to CSV in JavaScript, you’ll discover how to leverage libraries like SheetJS
Libraries for Converting XLSX to CSV in JavaScript
To facilitate the conversion of XLSX files to CSV format in JavaScript, several libraries are available that simplify this process. These libraries allow developers to easily read Excel files and convert their contents into a more universally accepted format like CSV. Below are some popular libraries:
- SheetJS (xlsx): This is one of the most widely used libraries for processing Excel files in JavaScript. It supports reading and writing various spreadsheet formats, including XLSX and CSV.
- Papa Parse: While primarily a CSV parsing library, it can be used in conjunction with other libraries to handle CSV outputs generated from XLSX files.
- ExcelJS: This library supports reading, manipulating, and writing Excel files and can easily export them to CSV format.
Each of these libraries has its own strengths, and the choice may depend on specific project requirements.
Using SheetJS to Convert XLSX to CSV
SheetJS provides a straightforward approach to convert XLSX files to CSV. Below is a step-by-step guide on how to accomplish this.
- Install the Library: You can install SheetJS via npm:
“`bash
npm install xlsx
“`
- Read the XLSX File: You can read the XLSX file using the `readFile` method.
- Convert to CSV: Utilize the `sheet_to_csv` method to convert the worksheet to CSV format.
Here’s a sample code snippet demonstrating these steps:
“`javascript
const XLSX = require(‘xlsx’);
function convertXlsxToCsv(filePath) {
// Read the XLSX file
const workbook = XLSX.readFile(filePath);
// Assuming the first sheet is the one we want to convert
const firstSheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[firstSheetName];
// Convert to CSV
const csv = XLSX.utils.sheet_to_csv(worksheet);
return csv;
}
// Example usage
const csvData = convertXlsxToCsv(‘example.xlsx’);
console.log(csvData);
“`
Handling Large XLSX Files
When dealing with large XLSX files, memory management becomes crucial. Here are some tips to efficiently convert large files to CSV:
- Stream the Data: Instead of loading the entire file into memory, consider streaming the data. Libraries like `xlsx-stream-reader` can be beneficial in this regard.
- Chunking: Process the file in chunks, allowing you to convert portions of the file sequentially.
- Use Workers: Offload heavy processing to Web Workers to keep the UI responsive.
Example of CSV Output
When the conversion is complete, the output will typically be structured as follows:
Column 1 | Column 2 | Column 3 |
---|---|---|
Data 1 | Data 2 | Data 3 |
Data 4 | Data 5 | Data 6 |
The resulting CSV will maintain a similar structure, where each row corresponds to a row in the XLSX file, and each column is separated by a comma. This format is widely used for data interchange, making it an ideal choice for exporting data.
By leveraging these libraries and techniques, developers can efficiently convert XLSX files to CSV format, catering to various application needs.
Libraries for Conversion
Several JavaScript libraries facilitate the conversion of XLSX files to CSV format. The most prominent ones include:
- SheetJS (xlsx): This library is widely used for reading and writing spreadsheet files in various formats, including XLSX and CSV.
- PapaParse: While primarily a CSV parser, it can be combined with other libraries to handle XLSX files.
- ExcelJS: This library allows for reading, manipulating, and writing Excel files but may require additional steps for CSV conversion.
Using SheetJS for XLSX to CSV Conversion
SheetJS offers a straightforward approach for converting XLSX files to CSV. Follow these steps to implement the conversion:
- Install SheetJS:
Use npm or include the library via a CDN in your HTML file.
“`bash
npm install xlsx
“`
- Read the XLSX File:
Use the `readFile` method to load the XLSX file.
“`javascript
const XLSX = require(‘xlsx’);
const workbook = XLSX.readFile(‘path/to/file.xlsx’);
“`
- Convert to CSV:
Access the desired sheet and convert it to CSV format.
“`javascript
const sheetName = workbook.SheetNames[0];
const sheet = workbook.Sheets[sheetName];
const csvData = XLSX.utils.sheet_to_csv(sheet);
“`
- Save the CSV File:
Use the `fs` module to write the CSV data to a file.
“`javascript
const fs = require(‘fs’);
fs.writeFileSync(‘output.csv’, csvData);
“`
Handling Multiple Sheets
If your XLSX file contains multiple sheets, you may need to iterate over each sheet for conversion. Here’s how:
“`javascript
const sheets = workbook.SheetNames;
sheets.forEach(sheetName => {
const sheet = workbook.Sheets[sheetName];
const csvData = XLSX.utils.sheet_to_csv(sheet);
fs.writeFileSync(`${sheetName}.csv`, csvData);
});
“`
Considerations for Data Formats
When converting XLSX to CSV, be aware of potential data format issues:
- Date Formats: Ensure that dates are in a recognizable format in CSV.
- Formulas: Only the result of the formula is exported; formulas themselves are not retained.
- Special Characters: Handle any special characters or encodings that may not translate well into CSV.
Error Handling
Implement error handling to manage issues during the conversion process. Example:
“`javascript
try {
const workbook = XLSX.readFile(‘path/to/file.xlsx’);
// Conversion logic here
} catch (error) {
console.error(‘Error reading the XLSX file:’, error);
}
“`
Performance Considerations
For larger XLSX files, performance may degrade. To optimize:
- Stream Processing: Use streaming options provided by libraries to handle large files without loading everything into memory.
- Chunking: Consider processing the file in chunks if the data set is significantly large.
Testing the Implementation
After implementing the conversion, thoroughly test with various XLSX files to ensure compatibility and data integrity. Utilize unit tests to validate that the conversion maintains the expected structure and format.
Example Code Snippet
Here is a complete example of converting an XLSX file to CSV:
“`javascript
const XLSX = require(‘xlsx’);
const fs = require(‘fs’);
try {
const workbook = XLSX.readFile(‘path/to/file.xlsx’);
workbook.SheetNames.forEach(sheetName => {
const sheet = workbook.Sheets[sheetName];
const csvData = XLSX.utils.sheet_to_csv(sheet);
fs.writeFileSync(`${sheetName}.csv`, csvData);
});
} catch (error) {
console.error(‘Error during conversion:’, error);
}
“`
Expert Insights on Converting XLSX to CSV Using JavaScript
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Converting XLSX files to CSV format using JavaScript is increasingly essential for data manipulation and analysis. Libraries like SheetJS provide robust solutions that allow developers to handle complex spreadsheets efficiently, ensuring that data integrity is maintained during the conversion process.”
Mark Thompson (Software Engineer, Data Solutions Group). “Utilizing JavaScript for file conversion tasks, such as XLSX to CSV, is not only efficient but also allows for seamless integration into web applications. The asynchronous nature of JavaScript enhances performance, especially when dealing with large datasets, making it a preferred choice for developers.”
Linda Huang (Technical Writer, Coding for Everyone). “When converting XLSX to CSV in JavaScript, it is crucial to consider the potential loss of formatting and data types. Developers should implement thorough testing to ensure that the resulting CSV files meet the necessary requirements for downstream applications, particularly in data analytics.”
Frequently Asked Questions (FAQs)
What is the process to convert XLSX to CSV using JavaScript?
To convert XLSX to CSV in JavaScript, you can use libraries such as SheetJS (xlsx). Load the XLSX file, parse its contents, and then convert the data to CSV format using the library’s built-in functions.
Are there any libraries available for converting XLSX to CSV in JavaScript?
Yes, popular libraries include SheetJS (xlsx), PapaParse, and ExcelJS. These libraries provide functionalities to read, manipulate, and convert spreadsheet data efficiently.
Can I convert XLSX to CSV in a Node.js environment?
Yes, you can use libraries like SheetJS in a Node.js environment. Install the library via npm, read the XLSX file, and then convert it to CSV using the appropriate methods provided by the library.
What are the limitations of converting XLSX to CSV?
CSV format does not support multiple sheets, formulas, or rich formatting. Only the raw data from the active sheet will be converted, and any non-text data may be lost in the conversion process.
How do I handle large XLSX files during conversion?
When dealing with large XLSX files, consider using streaming methods provided by libraries like SheetJS. This approach minimizes memory usage by processing data in chunks rather than loading the entire file into memory at once.
Is it possible to customize the CSV output during conversion?
Yes, many libraries allow customization of the CSV output. You can specify delimiters, quote characters, and even filter which rows or columns to include in the final CSV file.
Converting XLSX files to CSV format using JavaScript is a common task for developers who need to handle spreadsheet data in web applications. The process typically involves utilizing libraries such as SheetJS (xlsx) that facilitate reading and writing various spreadsheet formats. These libraries provide robust functionality, allowing users to easily parse XLSX files and export the data into CSV format with minimal code.
One of the key advantages of converting XLSX to CSV is the simplification of data handling. CSV files are lightweight and can be easily processed by various programming languages and tools, making them an ideal choice for data interchange. Additionally, CSV files are plain text, which allows for easier debugging and manual editing compared to the binary format of XLSX files.
Moreover, when implementing the conversion in JavaScript, developers can leverage asynchronous operations to enhance performance, especially when dealing with large datasets. Using features such as Promises or async/await syntax can ensure that the application remains responsive while processing files. Overall, understanding the conversion process and the tools available is essential for effective data management in JavaScript applications.
Author Profile
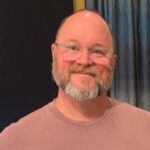
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?