How Can You Exclude Test Files from Your Webpack Build When Using Esbuild?
In the ever-evolving landscape of web development, optimizing your build process is essential for maintaining efficiency and performance. As developers increasingly turn to modern tools like Webpack and esbuild, understanding how to streamline your workflow becomes paramount. One common challenge many face is the need to exclude test files from the production build, which can bloat the final bundle and slow down application performance. In this article, we’ll explore effective strategies to seamlessly exclude test files when using Webpack alongside esbuild, ensuring that your final output is clean, efficient, and tailored to your production needs.
When working on a project, it’s not uncommon to have a variety of files that serve different purposes, including test files that are crucial during development but unnecessary in production. By learning how to configure your build tools to exclude these files, you can significantly reduce the size of your application bundle and enhance load times. This not only improves user experience but also optimizes resource usage on the server side.
In the following sections, we will delve into the practical steps and configurations needed to achieve this exclusion effectively. Whether you are a seasoned developer or just starting with Webpack and esbuild, our guide will provide you with the insights and techniques to refine your build process, ensuring that your application is both performant
Understanding Esbuild Configuration
To effectively exclude test files from a Webpack build using Esbuild, it is crucial to understand how both tools interact and how to configure Esbuild appropriately. Esbuild is a fast JavaScript bundler and minifier, which can be integrated into a Webpack workflow for optimization purposes.
The exclusion of test files can be managed by leveraging Esbuild’s configuration options. This involves specifying patterns that match the file paths of the test files you wish to exclude.
Configuring Esbuild with Webpack
Integrating Esbuild with Webpack requires certain configurations to ensure that the build process is efficient and that test files are excluded. Below are the steps to set up Esbuild in your Webpack configuration.
- Install Esbuild: Ensure that Esbuild is installed as a dev dependency in your project.
“`bash
npm install –save-dev esbuild
“`
- Modify Webpack Configuration: Update your Webpack configuration file (`webpack.config.js`) to include Esbuild as a loader. Here is an example configuration:
“`javascript
const path = require(‘path’);
const EsbuildPlugin = require(‘esbuild-loader’).EsbuildPlugin;
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /__tests__/,
use: {
loader: ‘esbuild-loader’,
options: {
loader: ‘js’,
target: ‘es2015’, // Specify the ECMAScript target
},
},
},
],
},
plugins: [
new EsbuildPlugin(),
],
};
“`
In this configuration, the `exclude` option uses a regular expression to match any files in the `__tests__` directory, effectively omitting them from the build process.
Using Patterns to Exclude Multiple Test Files
When excluding test files, you may want to use patterns that can match various naming conventions or directory structures. Below are some common patterns to consider:
- Exclude all files in `__tests__` directories:
- `/__tests__/`
- Exclude files with a `.test.js` suffix:
- `/.*\.test\.js$/`
- Exclude files with a `.spec.js` suffix:
- `/.*\.spec\.js$/`
You can combine these patterns using the logical OR operator (`|`):
“`javascript
exclude: /(__tests__|.*\.test\.js|.*\.spec\.js)/
“`
Example of Exclusion Configuration
Here is a complete example of a Webpack configuration that excludes both directory and file-based test patterns:
“`javascript
const path = require(‘path’);
const EsbuildPlugin = require(‘esbuild-loader’).EsbuildPlugin;
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /(__tests__|.*\.test\.js|.*\.spec\.js)/,
use: {
loader: ‘esbuild-loader’,
options: {
loader: ‘js’,
target: ‘es2015’,
},
},
},
],
},
plugins: [
new EsbuildPlugin(),
],
};
“`
This configuration efficiently excludes test files during the build process, enabling a cleaner and faster build output.
Table of Excluded File Patterns
Pattern Type | Example Pattern | Description |
---|---|---|
Directory Exclusion | /__tests__/ | Excludes all files in any __tests__ directory |
File Suffix Exclusion | /.*\.test\.js$/ | Excludes files ending with .test.js |
File Suffix Exclusion | /.*\.spec\.js$/ | Excludes files ending with .spec.js |
Configuring Webpack to Exclude Test Files
To exclude test files from your Webpack build while using Esbuild, you need to adjust your Webpack configuration. This typically involves modifying the `module.rules` section to utilize a regular expression that matches your test files.
Here’s how to configure it:
- Install Required Packages
Ensure you have Webpack and Esbuild set up in your project. If not, install them using npm or yarn:
“`bash
npm install –save-dev webpack webpack-cli esbuild-loader
“`
- Modify Webpack Configuration
Open your `webpack.config.js` file and add a rule to exclude test files. You can use the following configuration as a guide:
“`javascript
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /__tests__|\.spec\.js$/, // Exclude test files
use: {
loader: ‘esbuild-loader’,
options: {
loader: ‘jsx’, // Use ‘jsx’ for React files
target: ‘es2015’ // Specify target environment
}
}
}
]
},
resolve: {
extensions: [‘.js’, ‘.jsx’]
}
};
“`
In this configuration:
- The `exclude` property uses a regular expression to match directories or file names that should be excluded from the build process. Adjust the regex pattern based on your file organization.
Utilizing Esbuild Plugin with Webpack
If you prefer a more integrated approach with additional features, consider using the Esbuild plugin for Webpack. This allows for more streamlined configuration and can further optimize your build process.
- Install Esbuild Plugin
Install the Esbuild plugin for Webpack:
“`bash
npm install –save-dev esbuild-webpack-plugin
“`
- Update Webpack Configuration with Esbuild Plugin
Modify your `webpack.config.js` to incorporate the plugin:
“`javascript
const path = require(‘path’);
const EsbuildPlugin = require(‘esbuild-webpack-plugin’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /__tests__|\.spec\.js$/, // Exclude test files
use: ‘esbuild-loader’
}
]
},
plugins: [
new EsbuildPlugin()
],
resolve: {
extensions: [‘.js’, ‘.jsx’]
}
};
“`
This setup allows you to leverage the power of Esbuild while maintaining a clear separation from your test files.
Verifying Exclusion of Test Files
After configuring your Webpack setup, it’s essential to verify that test files are indeed excluded from your build. Here are a few methods to confirm this:
- Build Output Inspection: Run the Webpack build command and inspect the output files in the `dist` directory to ensure no test files are included.
- Console Logging: Add logging within your Webpack configuration to output included files during the build process. This can help you trace which files are being processed.
- Unit Tests: If applicable, create unit tests that depend on the presence of certain files. If your test files are excluded correctly, these tests should fail to reference them.
Implementing these configurations will help you effectively exclude test files from your Webpack build when using Esbuild, ensuring a cleaner and more efficient development process.
Strategies for Excluding Test Files in Webpack Builds with Esbuild
Jessica Tran (Senior Frontend Developer, CodeCraft Solutions). “When configuring Webpack to work with Esbuild, it is crucial to utilize the `exclude` option in your Webpack configuration. This allows you to specify patterns that match your test files, ensuring they are not included in the production build. A common approach is to use a regex pattern that targets files ending in `.test.js` or `.spec.js`.”
Michael Chen (Build Tooling Specialist, DevOps Innovations). “To effectively exclude test files from your Webpack build process while using Esbuild, consider setting up a custom Webpack plugin or modifying the entry points. This ensures that only the necessary application files are bundled, improving build performance and reducing the final bundle size.”
Laura Kim (Software Engineer, Agile Tech). “Incorporating Esbuild with Webpack provides a streamlined build process. However, to exclude test files, you should also configure your testing framework to run independently of your build process. This separation of concerns not only enhances maintainability but also allows for more efficient testing workflows.”
Frequently Asked Questions (FAQs)
How can I exclude test files from a Webpack build using Esbuild?
To exclude test files from a Webpack build using Esbuild, you can utilize the `exclude` option in your Webpack configuration. Specify a glob pattern that matches your test files, such as `/*.test.js` or `/*.spec.js`, in the `exclude` array of your module rules.
What is the purpose of excluding test files in a Webpack build?
Excluding test files in a Webpack build reduces the bundle size and improves build performance. It ensures that only necessary files are included in the final output, which is especially important for production environments.
Can I use a different approach to exclude test files in Esbuild?
Yes, you can also use the `–exclude` flag when running Esbuild from the command line. This allows you to specify patterns for files you want to exclude, including test files, directly during the build process.
Is it possible to conditionally exclude test files based on the environment?
Yes, you can conditionally exclude test files by checking the environment variable in your Webpack configuration. For example, you can set up a condition that excludes test files only when the environment is set to production.
What are the common patterns used to identify test files for exclusion?
Common patterns for identifying test files include `/*.test.js`, `/*.spec.js`, and any files located in a `__tests__` directory. Adjust these patterns based on your project’s file structure and naming conventions.
Does excluding test files affect source maps in the build?
Excluding test files does not typically affect source maps for the remaining code. However, if you have source maps generated for test files, those will not be included in the final build, potentially simplifying debugging for production code.
In the context of configuring a Webpack build to exclude test files while using esbuild, it is essential to understand the integration of both tools effectively. Webpack serves as a powerful module bundler that can be tailored to manage various file types and assets, while esbuild is renowned for its speed and efficiency in transpiling and bundling JavaScript and TypeScript files. By strategically setting up your Webpack configuration, you can optimize the build process and ensure that test files do not interfere with the production build.
One effective method to exclude test files is to utilize the `exclude` option within the Webpack configuration. This allows developers to specify patterns or directories that should be ignored during the build process. For instance, by excluding directories like `__tests__` or files ending with `.test.js`, you can streamline your build output and focus on the necessary production files. Additionally, leveraging esbuild’s capabilities can further enhance the performance of your build, as it can handle large codebases with remarkable speed.
Key takeaways from this discussion include the importance of maintaining a clean build environment by excluding unnecessary files, such as tests, from production builds. This practice not only improves build times but also reduces the potential for errors and ensures that
Author Profile
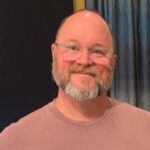
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?