Why Does My ESP32 FreeRTOS Task Time Out and How Can I Fix It?
In the world of embedded systems, the ESP32 microcontroller stands out as a powerful and versatile platform, particularly when paired with FreeRTOS for real-time task management. However, developers often encounter challenges that can lead to task timeouts, which can disrupt the smooth operation of their applications. Understanding the intricacies of task management in FreeRTOS is crucial for optimizing performance and ensuring that your ESP32 projects run seamlessly. In this article, we will delve into the common pitfalls associated with task timeouts, exploring their causes and offering insights into effective solutions.
Task timeouts in FreeRTOS can stem from various factors, including resource contention, improper task prioritization, and inadequate handling of inter-task communication. These issues can lead to unexpected behavior in your applications, such as tasks failing to execute as planned or the system becoming unresponsive. By recognizing the signs of task timeouts and understanding their underlying causes, developers can take proactive measures to mitigate these challenges.
Moreover, addressing task timeouts not only enhances the reliability of your ESP32 projects but also improves overall system efficiency. With a solid grasp of FreeRTOS task management principles, you can fine-tune your applications, ensuring that each task executes within its designated timeframe. Join us as we explore practical strategies and best
Understanding Task Timeouts in FreeRTOS on ESP32
When working with FreeRTOS on the ESP32 platform, developers may encounter situations where tasks appear to timeout. This can occur due to various factors affecting the scheduling and execution of tasks. Understanding these factors is critical for optimizing performance and ensuring that tasks operate as intended.
Tasks in FreeRTOS are designed to be preemptive, allowing the scheduler to manage multiple tasks efficiently. However, if a task is blocked or takes too long to execute, it can lead to timeouts. Key reasons for task timeouts include:
- Blocking Calls: Using functions that block the execution of a task (e.g., waiting for a semaphore, queue, or event group) without proper timeout handling.
- Priority Inversion: Lower-priority tasks may be preventing higher-priority tasks from executing, leading to unexpected behavior.
- Stack Overflow: Insufficient stack size allocated for the task may cause it to crash or become unresponsive.
- Resource Contention: Multiple tasks competing for the same resources can lead to delays and timeouts.
Strategies to Prevent Task Timeouts
To mitigate the risk of task timeouts in FreeRTOS on the ESP32, several strategies can be implemented:
- Use Timeouts: When waiting for resources, always specify a timeout. This prevents tasks from being indefinitely blocked.
- Adjust Task Priorities: Ensure that tasks are assigned appropriate priorities based on their criticality and the expected workload. This helps avoid priority inversion.
- Monitor Stack Usage: Use FreeRTOS functions like `uxTaskGetStackHighWaterMark()` to monitor stack usage and adjust stack sizes as needed.
- Implement Watchdog Timers: Use hardware or software watchdog timers to reset tasks that become unresponsive.
Issue | Possible Solution |
---|---|
Blocking Calls | Implement timeouts on blocking operations. |
Priority Inversion | Use priority inheritance mechanisms. |
Stack Overflow | Increase stack size and monitor stack usage. |
Resource Contention | Optimize resource management and task synchronization. |
Debugging Task Timeouts
When diagnosing task timeouts, it is essential to gather relevant information to pinpoint the cause. Consider the following debugging practices:
- Enable FreeRTOS Trace Hooks: By enabling trace hooks, you can gain insights into task execution and identify where timeouts occur.
- Analyze Task States: Use FreeRTOS API functions like `vTaskGetInfo()` to analyze the state of tasks and determine if they are in a blocked state.
- Check System Load: Monitor the overall system load to ensure that the CPU is not overwhelmed with tasks, which may lead to timeouts.
By employing these strategies and debugging methods, developers can effectively manage task execution within FreeRTOS on the ESP32, minimizing the impact of timeouts and optimizing system performance.
Understanding FreeRTOS Task Timeouts
In FreeRTOS, tasks can experience timeouts due to various reasons. A timeout typically occurs when a task waits for a resource or an event that does not occur within a specified timeframe. Recognizing and addressing these timeouts is crucial for maintaining system performance and reliability.
Common Causes of Task Timeouts
Several factors can lead to task timeouts in an ESP32 FreeRTOS environment:
- Resource Contention: Multiple tasks competing for the same resource can lead to delays.
- Blocking Calls: Using blocking functions without appropriate timeout handling can result in indefinite waits.
- High System Load: When the CPU is overloaded, tasks may not get scheduled in a timely manner.
- Incorrect Priorities: Tasks with low priority may be starved for CPU time if higher-priority tasks are always running.
- Deadlocks: Improper management of mutexes or semaphores may result in a deadlock situation, where tasks are waiting indefinitely.
Configuring Timeouts
FreeRTOS provides several mechanisms for configuring timeouts. Key parameters to consider include:
Parameter | Description |
---|---|
`configUSE_PREEMPTION` | Enables preemption to allow higher-priority tasks to run. |
`configTICK_RATE_HZ` | Sets the tick rate, affecting timing and scheduling. |
`pdMS_TO_TICKS(ms)` | Converts milliseconds to ticks for timeout configurations. |
`xSemaphoreTake()` | Can specify timeout when attempting to take a semaphore. |
Adjusting these parameters can help mitigate timeout issues and improve task responsiveness.
Handling Task Timeouts
Implementing robust timeout handling mechanisms is essential. Strategies include:
- Using Timeouts: When calling `xSemaphoreTake()` or similar functions, always specify a timeout.
- Periodic Checkpoints: Tasks should periodically check whether they should continue waiting or abort on timeout.
- Error Handling: Implement error handling routines that respond to timeout events, allowing for graceful recovery.
- Logging: Maintain logs for timeout occurrences to diagnose and address underlying issues.
Best Practices for Task Management
To minimize task timeouts, adhere to the following best practices:
- Task Prioritization: Design tasks with appropriate priorities based on their urgency and importance.
- Resource Management: Carefully manage shared resources to prevent contention and deadlocks.
- Efficient Code: Optimize task code to reduce execution time and improve response rates.
- Testing and Profiling: Regularly test and profile tasks to identify performance bottlenecks.
Debugging Task Timeouts
Debugging task timeouts can be challenging. Utilize the following techniques:
- FreeRTOS Trace Tools: Use built-in trace tools to visualize task execution and identify where timeouts occur.
- Serial Output: Implement serial logging to capture task states and timeout events for analysis.
- Watchdog Timers: Use watchdog timers to detect and reset tasks that exceed expected execution time.
By addressing the underlying causes of task timeouts and implementing these strategies, developers can enhance the stability and performance of their ESP32 FreeRTOS applications.
Expert Insights on ESP32 FreeRTOS Task Timeouts
Dr. Emily Chen (Embedded Systems Researcher, Tech Innovations Lab). “When dealing with task timeouts in ESP32 FreeRTOS, it is crucial to ensure that the task priorities are appropriately set. A lower priority task may not get the CPU time it needs, leading to unexpected timeouts. Properly managing task scheduling and ensuring that higher priority tasks do not starve lower ones is essential for reliable operation.”
Mark Thompson (IoT Solutions Architect, SmartTech Solutions). “One common issue that developers face with ESP32 FreeRTOS is the handling of blocking operations within tasks. If a task is waiting on a resource that is not being released, it can lead to timeouts. Implementing proper timeout mechanisms and ensuring non-blocking calls wherever possible can help mitigate this risk significantly.”
Linda Garcia (Firmware Engineer, NextGen Electronics). “Debugging task timeouts in FreeRTOS on the ESP32 can be challenging. Utilizing the FreeRTOS trace tools can provide insights into task states and help identify bottlenecks. Additionally, reviewing the stack sizes allocated to each task is vital, as insufficient stack space can lead to unpredictable behavior and timeouts.”
Frequently Asked Questions (FAQs)
What causes an ESP32 FreeRTOS task to time out?
Task timeouts in ESP32 FreeRTOS typically occur due to blocking operations, such as waiting for a semaphore or a queue that never receives data. Additionally, insufficient stack size or priority inversion can also lead to timeouts.
How can I debug a FreeRTOS task timeout on ESP32?
To debug a task timeout, utilize the FreeRTOS trace facility to monitor task states. Check the task’s stack size and ensure that it is adequate. Review the code for any blocking calls and ensure proper synchronization mechanisms are in place.
What are the default timeout values for FreeRTOS tasks on ESP32?
The default timeout values for FreeRTOS tasks on ESP32 can vary based on the specific API used, such as `xSemaphoreTake()`, which typically has a timeout value defined in ticks. Developers should refer to the FreeRTOS documentation for specific defaults.
Can task priorities affect timeout behavior in FreeRTOS on ESP32?
Yes, task priorities significantly influence timeout behavior. Higher priority tasks can preempt lower priority tasks, potentially leading to starvation of lower priority tasks and causing timeouts if they are not scheduled in a timely manner.
How can I prevent task timeouts in my ESP32 FreeRTOS application?
To prevent task timeouts, ensure that tasks are designed to yield control regularly, avoid long blocking operations, and implement proper error handling for synchronization primitives. Additionally, consider adjusting task priorities and stack sizes as needed.
What should I do if a task keeps timing out despite proper configuration?
If a task continues to time out, review the overall system load and resource availability. Analyze the task’s logic for potential deadlocks or infinite loops. Consider profiling the application to identify bottlenecks or performance issues that may contribute to timeouts.
In summary, the issue of ESP32 FreeRTOS task timeouts is a critical consideration for developers working with real-time applications on the ESP32 platform. FreeRTOS is designed to manage multiple tasks efficiently, but improper configuration or resource management can lead to tasks timing out. This can result in unexpected behavior and degraded system performance. Understanding the underlying causes of task timeouts is essential for effective debugging and optimization.
Key factors contributing to task timeouts include insufficient stack size, priority inversion, and blocking operations that exceed the allotted time. Developers should ensure that each task has an appropriate stack size and that task priorities are set correctly to prevent lower-priority tasks from blocking higher-priority ones. Additionally, careful management of delays and blocking calls is necessary to avoid exceeding timeout thresholds.
To mitigate timeout issues, developers can implement strategies such as using watchdog timers, optimizing task execution time, and employing non-blocking methods where possible. Monitoring system performance and utilizing FreeRTOS features like task notifications can also enhance responsiveness and reliability. By addressing these aspects, developers can create robust applications that leverage the full capabilities of the ESP32 without encountering task timeout problems.
Author Profile
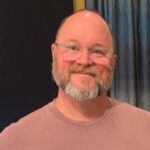
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?