How Can You Use Regex to Ensure At Least One Capital Letter in Your Strings?
In the digital age, where security and data integrity are paramount, the importance of strong passwords cannot be overstated. As we navigate through countless online platforms, the need for robust authentication methods has led to the development of various password policies. One essential aspect of these policies is the requirement for at least one capital letter in a password. But how do we ensure that our passwords meet these criteria? Enter the world of regular expressions, or regex, a powerful tool that can help us validate our passwords with precision and efficiency. In this article, we will explore the intricacies of crafting a regex pattern that enforces the inclusion of at least one capital letter, empowering you to enhance your security practices.
Regular expressions are a sequence of characters that form a search pattern, primarily used for string matching within texts. They provide a flexible and efficient way to validate user input, making them invaluable in programming and data processing. When it comes to password validation, regex can be employed to ensure that users adhere to specific requirements, such as including uppercase letters, lowercase letters, numbers, and special characters. By understanding how to construct a regex pattern that checks for at least one capital letter, developers and security professionals can significantly improve the robustness of their authentication systems.
In this article, we will delve into the syntax
Understanding the At Least One Capital Letter Regex
Regular expressions (regex) are powerful tools used for pattern matching within strings. When validating passwords, usernames, or other text inputs, ensuring at least one capital letter is often a requirement. The regex pattern to match a string containing at least one uppercase letter is straightforward yet effective.
The basic regex pattern for this purpose is:
“`
(?=.*[A-Z])
“`
This expression can be broken down into its components:
- `(?=…)`: This is a positive lookahead assertion that checks for the presence of a specified pattern without consuming characters.
- `.*`: This part matches any character (except for line terminators) zero or more times.
- `[A-Z]`: This character class matches any uppercase letter from A to Z.
Practical Examples
To illustrate how this regex works, consider the following examples:
String | Matches? |
---|---|
hello | No |
Hello | Yes |
hello World | Yes |
1234 | No |
test1234 | No |
Test1234 | Yes |
aBc | Yes |
alllowercase | No |
Combining with Other Requirements
In many scenarios, you may want to enforce additional criteria, such as requiring at least one lowercase letter, one numeric digit, and a minimum length. A more complex regex pattern could look like this:
“`
^(?=.*[a-z])(?=.*[A-Z])(?=.*\d).{8,}$
“`
This pattern enforces the following rules:
- `^`: Asserts the start of the string.
- `(?=.*[a-z])`: Ensures at least one lowercase letter.
- `(?=.*[A-Z])`: Ensures at least one uppercase letter.
- `(?=.*\d)`: Ensures at least one digit.
- `.{8,}`: Requires a minimum length of 8 characters.
- `$`: Asserts the end of the string.
Use Cases
Utilizing a regex for validating capital letters is particularly useful in various applications, including:
- Password validation: Strengthening security by enforcing complexity.
- User registration forms: Ensuring usernames meet specific criteria.
- Data entry fields: Validating input to meet business rules.
Conclusion
Incorporating regex patterns to check for at least one capital letter can significantly enhance data integrity and security in applications. By understanding and applying these expressions effectively, developers can create robust validation mechanisms.
Understanding At Least One Capital Letter Regex
Regular expressions (regex) are powerful tools used for pattern matching and validation in strings. The requirement to check for at least one capital letter in a string can be efficiently achieved using regex syntax.
Regex Pattern Explanation
To create a regex pattern that ensures at least one uppercase letter is present in a string, the following components can be utilized:
- Character Class: `[A-Z]` identifies any uppercase letter from A to Z.
- Quantifier: `+` specifies that the preceding element must occur at least once.
Combining these elements, the regex pattern becomes:
“`
(?=.*[A-Z])
“`
This pattern utilizes a positive lookahead assertion, ensuring that there is at least one capital letter anywhere in the string.
Implementation Examples
Here are various programming languages showcasing how to use this regex pattern:
Language | Code Example |
---|---|
Python |
import re def has_capital_letter(string): return bool(re.search(r'(?=.*[A-Z])', string)) |
JavaScript |
function hasCapitalLetter(string) { return /(?=.*[A-Z])/.test(string); } |
Java |
import java.util.regex.*; public class Main { public static boolean hasCapitalLetter(String string) { return Pattern.compile("(?=.*[A-Z])").matcher(string).find(); } } |
PHP |
function hasCapitalLetter($string) { return preg_match('/(?=.*[A-Z])/', $string); } |
Common Use Cases
Regex patterns for capital letter validation are frequently employed in various scenarios:
- Password Validation: Ensuring that users create strong passwords that include at least one uppercase letter.
- Form Validation: Validating user inputs in web forms to comply with specific formatting requirements.
- Data Processing: Scrubbing or validating data to ensure consistency and compliance with business rules.
Testing and Validation
When implementing regex for capital letter checks, it’s essential to test various scenarios to confirm accuracy. Consider the following test cases:
Test Case | Input | Expected Result |
---|---|---|
Only lowercase letters | `abcdefg` | |
Mixed case | `abcDfg` | True |
All uppercase | `ABCDEFG` | True |
Empty string | “ | |
Special characters | `abc!@D` | True |
Utilizing unit tests or regex test tools can help ensure the regex performs as expected across different strings.
Performance Considerations
While regex is a powerful tool, it can become inefficient with complex patterns or very large datasets. It is recommended to:
- Optimize patterns by reducing unnecessary complexity.
- Use non-greedy quantifiers where applicable.
- Test performance with large inputs to avoid slowdowns in applications.
By adhering to these guidelines, the implementation of regex for validating at least one capital letter can be both effective and efficient.
Understanding Regex for Capital Letter Validation
Dr. Emily Carter (Senior Software Engineer, CodeSecure Solutions). “Incorporating a regex pattern that ensures at least one capital letter is essential for validating user inputs, especially in password creation. This not only enhances security but also promotes better practices in user authentication.”
Michael Chen (Lead Data Scientist, DataGuard Analytics). “Using regex to enforce the presence of at least one capital letter in strings is a fundamental step in data validation. It helps prevent common errors and ensures that the data adheres to expected formats, which is crucial in data integrity.”
Sarah Thompson (Cybersecurity Consultant, SecureNet Advisors). “From a cybersecurity perspective, implementing a regex that checks for at least one uppercase letter can significantly reduce the risk of weak passwords. This simple rule is a vital component of a robust password policy.”
Frequently Asked Questions (FAQs)
What is the purpose of an At Least One Capital Letter Regex?
The At Least One Capital Letter Regex is designed to validate strings, ensuring that at least one uppercase letter is present. This is commonly used in password validation and input forms to enhance security.
How can I create an At Least One Capital Letter Regex pattern?
A simple regex pattern for this requirement is `^(?=.*[A-Z]).+$`. This pattern asserts that the string contains at least one uppercase letter anywhere in the text.
Can the At Least One Capital Letter Regex be combined with other validation rules?
Yes, the At Least One Capital Letter Regex can be combined with other rules, such as requiring lowercase letters, numbers, or special characters, by using additional assertions within the regex pattern.
What programming languages support At Least One Capital Letter Regex?
Most programming languages, including Python, Java, JavaScript, and PHP, support regex patterns, allowing for the implementation of the At Least One Capital Letter Regex for string validation.
Are there any common pitfalls when using At Least One Capital Letter Regex?
Common pitfalls include not anchoring the regex properly, which may lead to unexpected matches, and failing to account for cases where additional criteria may conflict with the presence of a capital letter.
How can I test my At Least One Capital Letter Regex?
You can test your regex using online regex testers or integrated development environments (IDEs) that support regex functionality. Input various test strings to ensure the regex behaves as expected under different scenarios.
In summary, the use of regular expressions (regex) to enforce the presence of at least one capital letter in a string is a common requirement in various programming and data validation scenarios. This regex pattern typically takes the form of `(?=.*[A-Z])`, which utilizes a positive lookahead assertion to ensure that at least one uppercase letter exists within the input. By implementing such a pattern, developers can enhance the security and integrity of user inputs, particularly in contexts like password validation and form submissions.
Furthermore, understanding regex not only aids in the validation of strings but also empowers developers to create more robust applications. Regex patterns can be tailored to meet specific criteria, such as length, character types, and more, allowing for comprehensive input validation. This flexibility is crucial in preventing common vulnerabilities associated with improper input handling, thereby improving overall application security.
Key takeaways include the importance of regex in ensuring data integrity and the necessity of incorporating at least one capital letter in user-generated content. Developers should familiarize themselves with regex syntax and best practices to effectively implement these patterns. By doing so, they can significantly reduce the risk of errors and enhance user experience through clearer validation feedback.
Author Profile
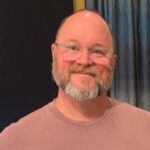
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?