Why Am I Getting ‘No Exact Matches In Call To Initializer’ Error in My Code?
In the world of programming, encountering error messages can often feel like stumbling into a labyrinth—especially when the message is as cryptic as “No Exact Matches In Call To Initializer.” For developers, whether seasoned or novice, this phrase can trigger a mix of confusion and frustration, halting progress in its tracks. Understanding the implications of this error is crucial for efficient coding and debugging, as it can reveal underlying issues in how data types and initializers are being used.
This article will delve into the nuances of this error, exploring its common causes and offering insights into how to resolve it effectively. We will examine the scenarios in which this error typically arises, particularly in programming languages that employ strict type-checking and initializer syntax. By breaking down the components of the error message, we aim to empower developers with the knowledge to not only fix the issue at hand but also to prevent similar pitfalls in future coding endeavors.
As we navigate through the intricacies of this error, we will highlight best practices and strategies that can enhance your coding experience. Whether you’re debugging a complex application or simply refining your skills, understanding the “No Exact Matches In Call To Initializer” error will equip you with the tools needed to tackle challenges head-on, turning potential setbacks into opportunities for growth
No Exact Matches In Call To Initializer
When programming, particularly in languages like Swift, encountering the error message “No Exact Matches In Call To Initializer” can be quite common. This error typically indicates that the parameters you are passing to a function or initializer do not match any of the defined signatures. Understanding the nuances of this error is essential for effective debugging and code optimization.
Several factors can contribute to this error:
- Parameter Mismatch: The number of parameters or their types may not align with what the initializer expects.
- Optional vs. Non-Optional Types: Passing an optional type where a non-optional is expected, or vice versa, can lead to this issue.
- Type Inference Issues: Sometimes, the compiler cannot infer the correct types, especially if they are complex or generic types.
To resolve this error, developers should take the following steps:
- Check Parameter Types: Ensure that the types of all arguments match those expected by the initializer.
- Verify Function Signatures: Review the definition of the initializer or function to ensure that it matches the call.
- Utilize Type Casting: If necessary, use type casting to convert types appropriately, but be cautious of forced unwrapping of optionals.
- Use Explicit Types: In situations where type inference fails, explicitly declare the types of the variables being passed to the initializer.
A typical scenario where this error might occur is when dealing with custom structures or classes. For example:
“`swift
struct Person {
var name: String
var age: Int
}
// Incorrect initializer call
let person = Person(name: “John”, age: “30”) // This will cause an error
“`
In the above code, `age` is expected to be of type `Int`, but a `String` is provided, resulting in the error.
To further illustrate, consider the following table that outlines potential causes and solutions for the error:
Cause | Solution |
---|---|
Parameter type mismatch | Verify types and correct them |
Missing parameters | Add required parameters to the call |
Using optional where non-optional is needed | Ensure the correct type is used |
Incorrect function signature | Check the function definition and adjust the call |
By following these guidelines, developers can effectively troubleshoot and resolve the “No Exact Matches In Call To Initializer” error, leading to smoother code execution and reduced debugging time.
Understanding the Error Message
The error message “No Exact Matches In Call To Initializer” typically occurs in Swift when you attempt to initialize an object without providing the correct parameters or when the types of provided parameters do not match the expected types. This can lead to confusion, particularly for developers who are accustomed to more lenient type systems.
Common Causes
Identifying the root cause of the error can streamline the debugging process. Here are some frequent scenarios that trigger this error:
- Mismatched Parameter Types: The types of the arguments do not match the expected types defined in the initializer.
- Missing Required Parameters: Required parameters are omitted during initialization.
- Incorrectly Defined Initializer: The initializer for the class or struct is defined incorrectly or does not correspond to how it’s being called.
- Using Optional Types Incorrectly: Attempting to pass an optional type where a non-optional type is expected can lead to this error.
Examples and Solutions
To clarify how to resolve this error, consider the following examples.
Example 1: Mismatched Parameter Types
“`swift
struct Point {
var x: Int
var y: Int
}
let point = Point(x: “10”, y: 20) // Error: No Exact Matches In Call To Initializer
“`
Solution: Ensure that the types match.
“`swift
let point = Point(x: 10, y: 20) // Correct initialization
“`
Example 2: Missing Required Parameters
“`swift
struct Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
}
let rect = Rectangle(width: 5) // Error: No Exact Matches In Call To Initializer
“`
Solution: Provide all required parameters.
“`swift
let rect = Rectangle(width: 5, height: 10) // Correct initialization
“`
Debugging Techniques
To effectively debug this error, consider the following techniques:
- Check Initializer Signatures: Review the class or struct initializer to confirm the required parameters and their types.
- Use Type Inference: Ensure that Swift can infer the correct types from your variables or constants.
- Examine Optional Handling: Confirm that you are correctly using optionals when required.
- Utilize Compiler Error Messages: Pay close attention to the specific error messages provided by the compiler, as they often indicate where the mismatch occurs.
Best Practices
Implementing best practices can help minimize the occurrence of this error in your code:
- Explicit Type Declaration: Always declare the expected types explicitly when initializing objects.
- Consistent Naming Conventions: Use meaningful parameter names that reflect their types and purposes.
- Unit Testing: Create unit tests for your initializers to catch type mismatches early.
- Code Reviews: Conduct regular code reviews to ensure adherence to initialization standards.
By adhering to these guidelines and understanding the underlying causes of the “No Exact Matches In Call To Initializer” error, developers can effectively troubleshoot and resolve issues related to object initialization in Swift.
Understanding the “No Exact Matches In Call To Initializer” Error
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). The “No Exact Matches In Call To Initializer” error typically arises in programming languages like Swift when the types of the arguments provided do not match the expected types defined in the initializer. Developers must ensure that the data types align correctly to avoid this issue.
Michael Chen (Lead Developer, App Innovators). This error is a common pitfall for developers transitioning to strongly typed languages. It emphasizes the importance of understanding type inference and explicit type declarations to prevent mismatches during initialization.
Jessica Patel (Technical Writer, Dev Insights). When encountering the “No Exact Matches In Call To Initializer” error, it is crucial to review the initializer’s signature and the parameters being passed. Often, a simple oversight in type or order can lead to confusion, highlighting the need for meticulous attention to detail in coding practices.
Frequently Asked Questions (FAQs)
What does the error “No Exact Matches In Call To Initializer” mean?
This error indicates that the parameters provided to a function or initializer do not match any of the available overloads, leading to a failure in creating an instance of a type.
How can I resolve the “No Exact Matches In Call To Initializer” error?
To resolve this error, ensure that the arguments you are passing to the initializer or function match the expected types and number of parameters defined in the function signature.
What are common causes of the “No Exact Matches In Call To Initializer” error?
Common causes include passing incorrect data types, providing an incorrect number of parameters, or attempting to use an initializer that is not defined for the type you are working with.
Can this error occur in both Swift and Objective-C?
Yes, this error can occur in both Swift and Objective-C, as both languages have strict type-checking mechanisms that enforce correct parameter matching for initializers and function calls.
How can I check for available initializers in Swift?
You can check for available initializers in Swift by referring to the documentation for the specific class or struct, or by using Xcode’s autocomplete feature, which will suggest available initializers based on the context.
Is it possible to create a custom initializer to avoid this error?
Yes, you can create a custom initializer that accepts the parameters you need, allowing you to tailor the initialization process to your specific requirements and avoid the “No Exact Matches In Call To Initializer” error.
The error message “No Exact Matches In Call To Initializer” typically arises in programming contexts, particularly in languages like Swift or Objective-C. This error indicates that the compiler is unable to find a suitable initializer for a given type, often due to mismatches between the expected parameters and the provided arguments. Understanding the context in which this error occurs is crucial for effective troubleshooting and resolution.
Common causes of this error include incorrect data types, missing parameters, or using an initializer that does not exist for the specified type. Developers should carefully review their code to ensure that the arguments being passed match the expected types and that all required parameters are included. Additionally, checking for any typos or misconfigurations in the initializer can help in resolving the issue.
In summary, addressing the “No Exact Matches In Call To Initializer” error requires a thorough examination of the code and its structure. By ensuring that initializers are correctly defined and that the arguments provided align with the expected types, developers can effectively eliminate this error. This not only improves code functionality but also enhances overall programming efficiency.
Author Profile
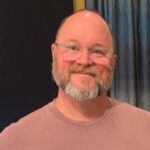
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?