How Can You Effectively Remove Leading Zeros in SQL?
### Introduction
In the world of data management and manipulation, SQL (Structured Query Language) stands as a cornerstone for interacting with databases. One common challenge that developers and data analysts often face is the presence of leading zeros in numeric fields. While these zeros may serve a purpose in certain contexts, they can complicate data processing, sorting, and reporting. Whether you’re dealing with identification numbers, product codes, or any other numerical data, knowing how to effectively remove leading zeros in SQL can streamline your operations and enhance data integrity.
Understanding how to manipulate strings and numbers in SQL is essential for anyone working with databases. Leading zeros can inadvertently alter the way data is interpreted, leading to potential discrepancies in analysis and reporting. For instance, a product code like “00123” may be treated as a string rather than a number, affecting calculations and comparisons. SQL provides various functions and methods to address this issue, allowing users to transform their data into a more usable format.
In this article, we will explore the techniques and best practices for removing leading zeros in SQL. From simple string manipulation functions to more complex queries, we’ll cover the tools at your disposal to ensure that your data is clean, accurate, and ready for analysis. Whether you’re a seasoned SQL expert or just starting your journey
Understanding Leading Zeros
Leading zeros are the zeros that precede the first non-zero digit in a number. While they can be significant in certain contexts, such as in numerical identifiers like ZIP codes or account numbers, they often pose challenges in data processing and storage, particularly in SQL databases. For instance, when working with numeric data types, leading zeros are typically disregarded, which can lead to data inconsistencies when converting from string types.
Removing Leading Zeros from Strings
To remove leading zeros from a string in SQL, you can use several methods depending on the SQL database being used. The most common approach involves using string functions. Below are some SQL statements that demonstrate how to achieve this.
- Using `CAST` or `CONVERT`: This method converts the string to an integer, effectively removing any leading zeros.
sql
SELECT CAST(‘000123’ AS INT) AS NoLeadingZeros;
- Using `LTRIM` with `REPLACE`: This is useful when you want to maintain the data type as a string.
sql
SELECT LTRIM(REPLACE(‘000123’, ‘0’, ‘ ‘)) AS NoLeadingZeros;
- Using Regular Expressions: Some SQL databases like PostgreSQL and MySQL support regular expression functions.
sql
SELECT REGEXP_REPLACE(‘000123’, ‘^0+’, ”) AS NoLeadingZeros;
Performance Considerations
When removing leading zeros, performance can be a concern, especially with large datasets. Consider the following tips to optimize performance:
- Avoid unnecessary conversions: Converting data types can be resource-intensive. Ensure you only convert when necessary.
- Indexing: If removing leading zeros is part of a regular query pattern, consider indexing the columns after transformation for faster retrieval.
- Batch processing: Process data in batches to minimize locking and improve performance.
Example Table of Leading Zeros Removal
Below is an example table demonstrating the removal of leading zeros from different input strings.
Original String | Processed Value |
---|---|
‘000001234’ | 1234 |
‘0000’ | 0 |
‘0123’ | 123 |
‘000ABCDE’ | ABCDE |
This table highlights how different leading zero scenarios are handled, illustrating the effectiveness of the methods discussed.
Best Practices
when dealing with leading zeros in SQL, selecting the appropriate method for removal is crucial. Consider the context of your data and the intended use to choose the best approach, ensuring both accuracy and performance in your database operations.
Using CAST and CONVERT Functions
One effective way to remove leading zeros from a string representation of a number in SQL is by using the `CAST` or `CONVERT` functions. These functions allow you to convert a string to an integer, which inherently removes any leading zeros.
Example Syntax:
sql
SELECT CAST(your_column AS INT) AS NoLeadingZeros
FROM your_table;
OR
sql
SELECT CONVERT(INT, your_column) AS NoLeadingZeros
FROM your_table;
Considerations:
- Ensure that the column contains valid numeric strings; otherwise, a conversion error may occur.
- The resulting integer will lose any decimal places if present.
Using the REPLACE Function
If the data type is not numeric and you want to retain it as a string, you can use the `REPLACE` function combined with a pattern match to eliminate leading zeros.
Example Syntax:
sql
SELECT REPLACE(your_column, ‘0’, ”) AS NoLeadingZeros
FROM your_table
WHERE your_column LIKE ‘0%’;
This method will replace all occurrences of ‘0’ in the string. However, it is important to note that this will remove all zeros, not just leading ones. Therefore, a more precise approach is needed.
Using SUBSTRING and PATINDEX Functions
To specifically target and remove only leading zeros while preserving zeros elsewhere in the string, the `SUBSTRING` function can be utilized alongside `PATINDEX`.
Example Syntax:
sql
SELECT
SUBSTRING(your_column, PATINDEX(‘%[^0]%’, your_column + ‘X’), LEN(your_column)) AS NoLeadingZeros
FROM your_table;
Explanation:
- `PATINDEX(‘%[^0]%’, your_column + ‘X’)` finds the position of the first non-zero character.
- `SUBSTRING` then extracts the substring starting from that position to the end of the string.
Handling NULL and Empty Values
When performing these transformations, it is crucial to handle potential `NULL` or empty string values to avoid unexpected results.
Example Syntax:
sql
SELECT
CASE
WHEN your_column IS NULL OR your_column = ” THEN your_column
ELSE SUBSTRING(your_column, PATINDEX(‘%[^0]%’, your_column + ‘X’), LEN(your_column))
END AS NoLeadingZeros
FROM your_table;
This `CASE` statement checks for `NULL` or empty values before applying the transformation, ensuring that the output remains consistent and avoids errors.
Performance Considerations
When processing large datasets, performance can be impacted by string manipulation functions. Here are a few tips to optimize performance:
- Indexing: Ensure that the column being transformed is properly indexed to speed up the search operations.
- Batch Processing: Process data in smaller batches if applicable, to minimize memory usage and lock contention.
- Testing: Always test performance on a representative dataset to evaluate the efficiency of your chosen method before deployment in production environments.
Implementing these techniques will enable you to effectively remove leading zeros in SQL while maintaining data integrity and performance. Choose the method that best fits your data type and requirements to achieve the desired results.
Expert Insights on Removing Leading Zeros in SQL
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Removing leading zeros in SQL is essential when dealing with numeric data types. It not only optimizes storage but also enhances performance during data retrieval operations. However, one must ensure that the data type conversion does not lead to loss of information, especially in cases where leading zeros are significant, such as in ZIP codes or account numbers.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “In SQL, utilizing functions like TRIM or CAST can effectively remove leading zeros. However, it is crucial to understand the context of the data. For instance, in financial applications, leading zeros might be relevant for formatting purposes. Therefore, a thorough analysis of data requirements is necessary before implementing such transformations.”
Laura Simmons (Data Quality Analyst, Insight Analytics). “When removing leading zeros, one must consider the implications on data integrity and validation. Implementing checks and balances, such as ensuring that the data remains in a valid format post-transformation, is vital. Additionally, documenting any changes made to the data structure helps maintain clarity for future data handling.”
Frequently Asked Questions (FAQs)
What is the purpose of removing leading zeros in SQL?
Removing leading zeros in SQL is often necessary for formatting purposes, data normalization, or to ensure that numeric comparisons and calculations are accurate. Leading zeros can cause issues when storing or processing data as integers.
How can I remove leading zeros from a string in SQL?
You can use the `CAST` or `CONVERT` functions to convert a string to an integer, which automatically removes leading zeros. For example: `SELECT CAST(your_column AS INT) FROM your_table;`.
Is there a function in SQL specifically for removing leading zeros?
While there is no built-in function dedicated solely to removing leading zeros, you can achieve this using string manipulation functions such as `LTRIM` combined with `REPLACE` or by converting to an integer type.
Can I remove leading zeros from numeric data types in SQL?
Numeric data types do not store leading zeros; therefore, when you insert a number with leading zeros into a numeric column, the zeros are automatically removed.
What happens if I try to remove leading zeros from a column with mixed data types?
If you attempt to remove leading zeros from a column with mixed data types, you may encounter errors or unexpected results. It is advisable to ensure that the column is consistently formatted as a string before applying any transformation.
Are there performance considerations when removing leading zeros in SQL?
Yes, performance can be affected, especially on large datasets. Using functions to manipulate strings can lead to slower query performance. It is best to handle such operations carefully, possibly during data entry or preprocessing stages.
Removing leading zeros in SQL is a common requirement when dealing with numeric data stored as strings. The presence of leading zeros can lead to confusion in data interpretation and can affect sorting and comparisons. Various SQL functions and methods can be utilized to eliminate these leading zeros effectively, depending on the specific SQL dialect in use, such as SQL Server, MySQL, or Oracle.
One of the primary methods to remove leading zeros involves converting the string to an integer type, which inherently discards any leading zeros. For instance, using the `CAST` or `CONVERT` functions can transform the string representation of a number into an integer, thereby removing any unnecessary leading zeros. Another approach is to use string manipulation functions, such as `LTRIM`, in combination with `REPLACE` or `SUBSTRING`, to specifically target and remove leading zeros without altering the numeric value.
It is essential to consider the implications of removing leading zeros, especially in cases where the original format is significant, such as in identifiers or codes. In such scenarios, it may be more appropriate to retain the leading zeros for consistency and clarity. Additionally, understanding the context of the data and the desired output format will guide the choice of method for removing leading zeros.
Author Profile
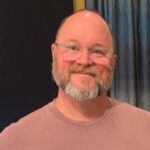
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?