How Can You Undo Actions in Python Programming?
In the world of programming, mistakes are an inevitable part of the learning process. Whether you’re a seasoned developer or just starting your journey with Python, the ability to efficiently undo actions can save you time, frustration, and countless hours of debugging. Imagine writing a complex function only to realize you’ve made a critical error halfway through. Instead of starting from scratch, what if you could seamlessly revert to a previous state? This article explores the various methods and best practices for implementing an “undo” functionality in Python, empowering you to code with confidence and creativity.
When it comes to undoing actions in Python, there are several strategies you can employ, each suited to different scenarios. From leveraging built-in data structures to utilizing external libraries, Python offers a versatile toolkit for managing changes in your code. Understanding these methods not only enhances your programming skills but also equips you with the knowledge to handle errors gracefully, ensuring your projects remain on track.
As we delve deeper into the topic, we will explore practical examples and techniques that demonstrate how to implement undo functionality effectively. Whether you’re developing a simple script or a complex application, mastering the art of undoing actions will elevate your coding experience and help you navigate the challenges of programming with ease. Get ready to unlock the secrets of Python’s undo capabilities
Understanding Undo Mechanisms in Python
In Python, implementing an undo functionality is not straightforward, as the language does not provide a built-in mechanism for this. However, developers can create their own undo features by managing the state of an application effectively. The concept is primarily about tracking changes and allowing users to revert to a previous state.
Using Stacks for Undo Functionality
One common approach to implement an undo feature is through the use of a stack data structure. Stacks operate on a Last In, First Out (LIFO) principle, making them ideal for keeping track of changes that need to be undone.
- Push: When a change is made, the current state is pushed onto the stack.
- Pop: When an undo action is triggered, the most recent state is popped off the stack, reverting the application to that state.
Here is a simple implementation of undo functionality using a stack:
“`python
class UndoStack:
def __init__(self):
self.stack = []
def push(self, state):
self.stack.append(state)
def pop(self):
if self.stack:
return self.stack.pop()
return None
“`
Example of Undo Functionality
To illustrate the use of the `UndoStack`, consider a text editor application. Each time the user makes a change, the current text can be stored in the stack. Here’s a basic example:
“`python
class TextEditor:
def __init__(self):
self.text = “”
self.undo_stack = UndoStack()
def type(self, new_text):
self.undo_stack.push(self.text)
self.text += new_text
def undo(self):
previous_text = self.undo_stack.pop()
if previous_text is not None:
self.text = previous_text
“`
In this example, every time text is typed, the current state is saved. When the user calls `undo()`, the text reverts to the last saved state.
Implementing Redo Functionality
To enhance the user experience, implementing a redo functionality in conjunction with undo is advisable. This can be achieved by maintaining a second stack, often referred to as a redo stack.
- Undo Action: When an undo is performed, the state is not only removed from the undo stack but also pushed onto the redo stack.
- Redo Action: When a redo action is triggered, the state is popped from the redo stack and pushed back onto the undo stack.
Here’s how you can modify the `TextEditor` class to include redo functionality:
“`python
class TextEditor:
def __init__(self):
self.text = “”
self.undo_stack = UndoStack()
self.redo_stack = UndoStack()
def type(self, new_text):
self.undo_stack.push(self.text)
self.redo_stack = UndoStack() Clear redo stack
self.text += new_text
def undo(self):
previous_text = self.undo_stack.pop()
if previous_text is not None:
self.redo_stack.push(self.text)
self.text = previous_text
def redo(self):
next_text = self.redo_stack.pop()
if next_text is not None:
self.undo_stack.push(self.text)
self.text = next_text
“`
Advantages of Using Stacks for Undo/Redo
The stack-based approach for implementing undo and redo functionalities has several advantages:
Advantage | Description |
---|---|
Simplicity | Stacks are easy to understand and implement, making them suitable for managing state changes. |
Efficiency | Push and pop operations are O(1), ensuring that undo and redo actions are performed quickly. |
Flexibility | Stacks can be easily expanded to handle more complex scenarios, such as multi-level undo. |
This structured approach not only provides a clear method for implementing undo functionality in Python but also enhances the interactivity and user experience of applications.
Understanding Undo Functionality in Python
In Python, the concept of “undo” is not natively implemented in the same way it may be in other programming environments. However, there are various strategies and libraries available to facilitate undo functionality in applications.
Implementing Undo Functionality
To create an undo feature, one must typically maintain a history of actions. This can be achieved through several approaches:
- Stack Data Structure: Utilizing a stack to store the previous states or commands allows for easy reversal of actions.
- Command Pattern: This design pattern encapsulates all necessary information to perform an action or undo it, providing a flexible way to handle commands.
- State Management: Storing the state of an object at certain points allows for restoring to previous states.
Example: Using a Stack for Undo
Here is a simplified example of how to implement an undo functionality using a stack:
“`python
class UndoManager:
def __init__(self):
self.history = []
def do_action(self, action):
self.history.append(action)
print(f”Performed action: {action}”)
def undo(self):
if self.history:
action = self.history.pop()
print(f”Undid action: {action}”)
else:
print(“No actions to undo.”)
Usage
manager = UndoManager()
manager.do_action(“Action 1”)
manager.do_action(“Action 2”)
manager.undo() Outputs: Undid action: Action 2
manager.undo() Outputs: Undid action: Action 1
manager.undo() Outputs: No actions to undo.
“`
Advanced Undo with Command Pattern
The Command pattern enhances the functionality by creating command objects that can be executed and undone. Below is a basic structure:
“`python
class Command:
def execute(self):
pass
def undo(self):
pass
class ConcreteCommand(Command):
def __init__(self, receiver):
self.receiver = receiver
def execute(self):
self.receiver.action()
def undo(self):
self.receiver.undo_action()
class Receiver:
def action(self):
print(“Action performed.”)
def undo_action(self):
print(“Action undone.”)
class Invoker:
def __init__(self):
self.commands = []
def execute_command(self, command):
command.execute()
self.commands.append(command)
def undo_command(self):
if self.commands:
command = self.commands.pop()
command.undo()
Usage
receiver = Receiver()
command = ConcreteCommand(receiver)
invoker = Invoker()
invoker.execute_command(command) Outputs: Action performed.
invoker.undo_command() Outputs: Action undone.
“`
Using Libraries for Undo Functionality
For more complex applications, leveraging existing libraries can be beneficial. Some popular libraries include:
Library | Description |
---|---|
`PyQt` | Provides undo/redo functionality in GUI apps. |
`Tkinter` | Basic support for undo in canvas and text widgets. |
`Pygame` | Implementing custom undo for game states. |
These libraries often come with built-in mechanisms to handle undo operations efficiently, allowing developers to focus on the application logic rather than the underlying implementation details.
By employing stacks, the Command pattern, or third-party libraries, developers can effectively implement undo functionality in Python applications, enhancing user experience and providing a robust solution for action management.
Expert Insights on Implementing Undo Functionality in Python
Dr. Emily Carter (Software Architect, CodeCraft Solutions). “Implementing an undo function in Python typically involves maintaining a history stack that records changes. This allows developers to revert to previous states effectively. Utilizing data structures like lists or custom classes can streamline this process, ensuring that the undo functionality is both efficient and easy to implement.”
Michael Chen (Lead Developer, PyTech Innovations). “In Python, the concept of undo can be realized through command pattern implementations. By encapsulating actions as objects, developers can easily manage and reverse operations. This approach not only enhances code readability but also promotes scalability in applications that require complex undo functionalities.”
Sarah Thompson (Senior Python Developer, Data Solutions Inc.). “An effective undo mechanism in Python applications often leverages the observer pattern. By notifying observers of changes, developers can track modifications and allow users to revert actions seamlessly. This design pattern is particularly useful in GUI applications where user interactions are frequent.”
Frequently Asked Questions (FAQs)
How can I implement an undo feature in a Python application?
To implement an undo feature in Python, you can use a stack data structure to keep track of actions. Each time an action is performed, push it onto the stack. When an undo is requested, pop the last action from the stack and revert the changes accordingly.
Is there a built-in undo function in Python?
Python does not have a built-in undo function. However, you can create your own undo functionality by managing the state of your application and using data structures like stacks or lists to track changes.
What libraries can help implement undo functionality in Python?
Libraries such as `tkinter` for GUI applications or `pygame` for game development can help implement undo functionality. Additionally, you can use design patterns like Command or Memento, which are useful for managing undo operations.
Can I use decorators to create an undo feature in Python?
Yes, decorators can be used to wrap functions and track their state before execution. By storing the state before a function call, you can revert to that state when an undo operation is triggered.
How do I handle multiple levels of undo in Python?
To handle multiple levels of undo, maintain a stack for actions and a separate stack for states. Each time an action is performed, push the current state onto the state stack. On undo, pop from both stacks to revert to the previous state.
What are some common challenges when implementing undo in Python?
Common challenges include managing complex states, ensuring performance with large data sets, and handling concurrent modifications. It is essential to design a robust system that efficiently tracks changes without introducing significant overhead.
In Python, the concept of “undo” is not a built-in feature like in some other programming languages or applications. However, developers can implement undo functionality through various strategies. One common approach is to use data structures such as stacks to keep track of changes made to data. By pushing each state onto the stack, you can easily revert to a previous state by popping the last change off the stack.
Another method to achieve undo functionality is through the use of version control systems or libraries that manage state changes. These systems can track modifications over time, allowing users to revert to earlier versions of data or configurations. Additionally, certain frameworks and libraries may offer built-in support for undo operations, which can simplify the implementation process.
It is essential to consider the specific requirements of your application when implementing undo functionality. Factors such as the complexity of the data, the frequency of changes, and the user experience should guide the design choices. By carefully planning and utilizing appropriate data structures or libraries, developers can create an effective undo feature that enhances the usability of their applications.
Author Profile
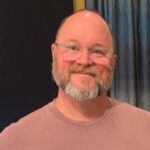
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?