How Can You Save a List to a File Using PowerShell?
In the world of system administration and automation, PowerShell stands out as a powerful tool that enables users to streamline tasks and manage resources efficiently. One common yet essential task is saving data to a file, which can be crucial for logging, reporting, or simply storing information for future reference. Whether you’re a seasoned IT professional or a newcomer to scripting, mastering the art of saving lists to files in PowerShell can significantly enhance your productivity and efficiency.
This article delves into the various methods available in PowerShell for saving lists to files, providing you with the knowledge to handle data effectively. From simple arrays to complex objects, PowerShell offers a range of options to capture and store your data in formats that suit your needs. We will explore the syntax and commands that facilitate this process, ensuring you can easily implement these techniques in your own scripts.
By the end of this guide, you will not only understand the fundamental concepts behind saving lists to files in PowerShell but also gain practical insights that can be applied in real-world scenarios. Whether you’re looking to create logs, export data for analysis, or just keep a record of your scripts’ outputs, the strategies discussed here will empower you to take control of your data management tasks with confidence.
Saving a List to a Text File
In PowerShell, saving a list to a text file can be accomplished with the `Out-File` cmdlet or by using redirection operators. This allows users to export data from variables or commands directly into a file for later use or analysis.
To save a list of items stored in a variable to a text file, you can use the following command:
“`powershell
$list = “Item1”, “Item2”, “Item3”
$list | Out-File -FilePath “C:\path\to\your\file.txt”
“`
Alternatively, the same operation can be performed using the redirection operator:
“`powershell
$list > “C:\path\to\your\file.txt”
“`
Both methods will create a text file containing each item on a new line.
Saving a List in CSV Format
When working with data that requires a structured format, saving as CSV (Comma-Separated Values) is often more advantageous. This format is commonly used for data manipulation and can be easily imported into spreadsheet applications.
To save a list as a CSV file, the `Export-Csv` cmdlet is utilized:
“`powershell
$list = @(
[PSCustomObject]@{ Name = “Item1”; Value = 10 },
[PSCustomObject]@{ Name = “Item2”; Value = 20 }
)
$list | Export-Csv -Path “C:\path\to\your\file.csv” -NoTypeInformation
“`
Using `-NoTypeInformation` ensures that unnecessary type information is not included in the CSV file. The resulting CSV will look like this:
Name | Value |
---|---|
Item1 | 10 |
Item2 | 20 |
Appending to Existing Files
If you need to add additional data to an existing file without overwriting it, you can use the `-Append` parameter with the `Out-File` cmdlet:
“`powershell
$newItems = “Item4”, “Item5”
$newItems | Out-File -FilePath “C:\path\to\your\file.txt” -Append
“`
This command ensures that the new items are added to the end of the file rather than replacing its current contents.
Saving a List of Objects
When dealing with more complex data structures, such as lists of objects, you might want to export them in a way that preserves their properties. This can be done using `Export-Csv` or `ConvertTo-Json` for JSON format.
To save a list of objects to a JSON file, use:
“`powershell
$objects = @(
[PSCustomObject]@{ Name = “Item1”; Value = 10 },
[PSCustomObject]@{ Name = “Item2”; Value = 20 }
)
$objects | ConvertTo-Json | Set-Content -Path “C:\path\to\your\file.json”
“`
This will create a JSON file that accurately represents the list of objects, which can be useful for APIs and data interchange.
Understanding how to save lists to files in PowerShell is crucial for effective data management and automation. By utilizing the appropriate cmdlets and file formats, users can ensure their data is stored efficiently and is easily accessible for future use.
Saving Data to a File in PowerShell
PowerShell provides several methods to save a list or collection of data to a file. Below are some common techniques to achieve this, including exporting to CSV, text files, and more.
Exporting to CSV Files
CSV (Comma-Separated Values) files are widely used for storing tabular data. PowerShell’s `Export-Csv` cmdlet allows users to easily export data into a CSV format.
Basic Syntax:
“`powershell
$Data | Export-Csv -Path “C:\path\to\file.csv” -NoTypeInformation
“`
Example:
“`powershell
$Users = Get-ADUser -Filter * | Select-Object Name, EmailAddress
$Users | Export-Csv -Path “C:\Users\ExportedUsers.csv” -NoTypeInformation
“`
Key Parameters:
- `-Path`: Specifies the file path where the CSV will be saved.
- `-NoTypeInformation`: Prevents the type information from being included in the first line of the CSV.
Saving to Text Files
For simpler data storage, PowerShell can output data directly to text files using the `Out-File` cmdlet or the redirection operator (`>`).
**Using Out-File**:
“`powershell
$Data | Out-File -FilePath “C:\path\to\file.txt”
“`
**Using Redirection Operator**:
“`powershell
$Data > “C:\path\to\file.txt”
“`
Example:
“`powershell
$Processes = Get-Process
$Processes | Out-File -FilePath “C:\Processes.txt”
“`
Saving Lists with Format-Table
To format data neatly before saving, `Format-Table` can be combined with `Out-File` to control how the output is structured.
Example:
“`powershell
$Services = Get-Service | Format-Table -Property Name, Status
$Services | Out-File -FilePath “C:\Services.txt”
“`
Appending to Existing Files
To append data to an existing file rather than overwrite it, use the `-Append` parameter with `Out-File` or the `>>` operator.
**Example**:
“`powershell
$NewData = “This is a new line.”
$NewData | Out-File -FilePath “C:\path\to\file.txt” -Append
“`
**Using Redirection**:
“`powershell
$NewData >> “C:\path\to\file.txt”
“`
Using JSON Format
PowerShell also supports exporting data in JSON format, which is useful for web applications and data interchange.
Basic Syntax:
“`powershell
$Data | ConvertTo-Json | Set-Content -Path “C:\path\to\file.json”
“`
Example:
“`powershell
$Config = @{Name=”Sample”; Version=1.0}
$Config | ConvertTo-Json | Set-Content -Path “C:\Config.json”
“`
Key Cmdlets:
- `ConvertTo-Json`: Converts PowerShell objects to JSON format.
- `Set-Content`: Writes content to a file.
Batch Saving with ForEach-Object
When dealing with multiple items or datasets, `ForEach-Object` can be used to iterate through collections and save each item individually.
Example:
“`powershell
$Items = Get-ChildItem
$Items | ForEach-Object { $_.Name | Out-File -FilePath “C:\ItemsList.txt” -Append }
“`
This approach is effective for logging or creating files based on each item in a collection.
Expert Insights on Saving Lists to Files in PowerShell
“Jessica Turner (Senior Systems Administrator, Tech Solutions Inc.). PowerShell provides a straightforward way to save lists to files using cmdlets like Export-Csv or Out-File. These tools not only facilitate the export of data but also allow for easy manipulation of the output format, making it essential for system administrators to master.”
“Michael Chen (PowerShell Specialist, Cloud Innovations). When saving a list to a file in PowerShell, it is crucial to consider the file format that best suits your needs. For structured data, CSV is often preferred, while plain text files are useful for simpler lists. Understanding these nuances can significantly enhance data management practices.”
“Linda Patel (IT Consultant, Future Tech Advisors). Utilizing PowerShell to save lists not only streamlines workflows but also integrates seamlessly with other automation tasks. By leveraging scripts to automate this process, IT professionals can ensure consistency and reliability in data handling across various applications.”
Frequently Asked Questions (FAQs)
How can I save a list to a file in PowerShell?
You can save a list to a file in PowerShell using the `Out-File` cmdlet or the `Set-Content` cmdlet. For example, to save a list of items, you can use:
“`powershell
$list = “Item1”, “Item2”, “Item3”
$list | Out-File -FilePath “C:\path\to\your\file.txt”
“`
What is the difference between Out-File and Set-Content in PowerShell?
`Out-File` is primarily used for formatting output for display, while `Set-Content` is used to write or replace content in a file. Use `Out-File` for formatted output and `Set-Content` for direct file writing.
Can I append to a file instead of overwriting it in PowerShell?
Yes, you can append to a file using the `-Append` parameter with `Out-File` or `Add-Content`. For example:
“`powershell
“NewItem” | Add-Content -Path “C:\path\to\your\file.txt”
“`
What file formats can I save my list in using PowerShell?
You can save your list in various formats, including plain text (.txt), CSV (.csv), and JSON (.json). The format depends on the cmdlet used, such as `Export-Csv` for CSV files.
How do I save a list of objects to a CSV file in PowerShell?
To save a list of objects to a CSV file, use the `Export-Csv` cmdlet. For example:
“`powershell
$list = @(
[PSCustomObject]@{Name=”Item1″; Value=1},
[PSCustomObject]@{Name=”Item2″; Value=2}
)
$list | Export-Csv -Path “C:\path\to\your\file.csv” -NoTypeInformation
“`
Is it possible to save a list in a specific encoding format in PowerShell?
Yes, you can specify the encoding format when saving a file using the `-Encoding` parameter. For example:
“`powershell
$list | Out-File -FilePath “C:\path\to\your\file.txt”
In summary, saving a list to a file using PowerShell is a straightforward process that can be accomplished through various methods. The most common approaches include utilizing cmdlets such as `Out-File`, `Set-Content`, and `Export-Csv`, each serving different purposes depending on the desired output format. Users can choose to save data in plain text, CSV, or other formats, making PowerShell a versatile tool for data management and automation.
Additionally, understanding the syntax and options available for these cmdlets is crucial for effective file handling. For instance, using the `-Append` parameter with `Out-File` allows users to add to existing files without overwriting them. Similarly, `Export-Csv` is particularly useful for exporting structured data into a format that can be easily imported into spreadsheet applications, thereby enhancing data usability.
Ultimately, leveraging PowerShell to save lists to files not only streamlines workflows but also enhances data organization and accessibility. By mastering these techniques, users can efficiently manage their data, automate repetitive tasks, and improve overall productivity within their computing environment.
Author Profile
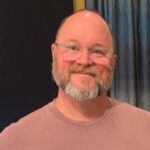
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?