How Can You Efficiently Record, Path, and Retrieve Values in Python’s Nested Data Structures?
In the world of programming, data structures are the backbone of efficient data management and manipulation. Among the myriad of data structures available, nested data structures in Python stand out for their versatility and complexity. These structures, which can hold multiple layers of data, allow developers to create rich and intricate representations of real-world scenarios. Whether you’re organizing a collection of user profiles, managing a multi-dimensional array, or storing hierarchical information, mastering nested data structures is essential for any Python programmer.
Navigating through nested data structures can be both a challenge and an opportunity. Understanding how to effectively record, access, and retrieve values from these structures is crucial for developing robust applications. With Python’s powerful built-in data types like lists, dictionaries, and tuples, you can create sophisticated data arrangements that mirror complex relationships. However, the intricacies of traversing these structures require a solid grasp of indexing, key-value pairs, and the principles of recursion.
As we delve deeper into the world of nested data structures in Python, we will explore practical techniques for recording data, defining paths for retrieval, and extracting values with precision. Whether you’re a seasoned developer or a newcomer to Python, this exploration will equip you with the knowledge and skills to handle nested data structures with confidence and efficiency. Get ready to unlock
Understanding Nested Data Structures
Nested data structures in Python allow for the organization of complex data in a hierarchical manner. This can include combinations of lists, dictionaries, tuples, and sets. Each of these structures can contain others, leading to a flexible and powerful means of data management.
For example, a nested dictionary can be used to represent a record of students where each student has multiple attributes, such as name, age, and grades:
“`python
students = {
‘Alice’: {‘age’: 20, ‘grades’: [85, 90, 78]},
‘Bob’: {‘age’: 22, ‘grades’: [88, 92, 95]},
}
“`
In this structure, each student’s name is a key associated with another dictionary that holds their respective attributes.
Accessing Nested Data
Retrieving values from nested data structures requires specifying the keys and indices that navigate through the layers of the structure. This can be achieved using a combination of indexing and key retrieval.
To access the age of Bob, you would use the following syntax:
“`python
bob_age = students[‘Bob’][‘age’]
“`
For a more complex structure, consider the following:
“`python
company = {
‘HR’: {
’employees’: [‘Alice’, ‘Bob’],
‘departments’: {
‘recruitment’: {‘openings’: 5},
‘payroll’: {‘budget’: 100000}
}
},
‘Engineering’: {
’employees’: [‘Charlie’],
‘projects’: [‘Project X’, ‘Project Y’]
}
}
“`
To retrieve the number of openings in the recruitment department:
“`python
openings = company[‘HR’][‘departments’][‘recruitment’][‘openings’]
“`
Path Retrieval in Nested Structures
When working with deeply nested structures, it is often necessary to retrieve a value by following a specific path through the hierarchy. One effective method is to define a function that takes a list of keys or indices representing the path to the desired value.
Here is a function that demonstrates this concept:
“`python
def get_nested_value(data, path):
for key in path:
data = data[key]
return data
“`
You can use this function to access the number of employees in the HR department as follows:
“`python
hr_employees = get_nested_value(company, [‘HR’, ’employees’])
“`
Example Table of Nested Data Retrieval
The following table illustrates different retrieval scenarios using nested data structures.
Scenario | Path | Code Example | Retrieved Value |
---|---|---|---|
Access Bob’s Age | [‘Bob’, ‘age’] | students[‘Bob’][‘age’] | 22 |
Access Openings in Recruitment | [‘HR’, ‘departments’, ‘recruitment’, ‘openings’] | company[‘HR’][‘departments’][‘recruitment’][‘openings’] | 5 |
Access HR Employees | [‘HR’, ’employees’] | get_nested_value(company, [‘HR’, ’employees’]) | [‘Alice’, ‘Bob’] |
This structured approach to nested data retrieval not only enhances code readability but also minimizes errors associated with accessing deeply nested values.
Understanding Nested Data Structures in Python
Nested data structures in Python allow for the organization of data in complex ways, enabling the storage of multiple levels of information. Common types of nested data structures include:
- Lists within lists
- Dictionaries within dictionaries
- Lists containing dictionaries
- Dictionaries containing lists
Each of these structures can be combined to form more intricate models, allowing for a greater degree of data representation.
Accessing Values in Nested Structures
Accessing values within nested data structures involves specifying a path through the layers of the structure. Here are some examples:
- Lists within lists:
“`python
data = [[1, 2, 3], [4, 5, 6]]
value = data[1][2] Accessing the value 6
“`
- Dictionaries within dictionaries:
“`python
data = {‘outer’: {‘inner’: ‘value’}}
value = data[‘outer’][‘inner’] Accessing ‘value’
“`
- Lists containing dictionaries:
“`python
data = [{‘key1’: ‘value1’}, {‘key2’: ‘value2’}]
value = data[1][‘key2’] Accessing ‘value2’
“`
- Dictionaries containing lists:
“`python
data = {‘key’: [1, 2, 3]}
value = data[‘key’][0] Accessing 1
“`
Retrieving Values with a Function
To abstract the value retrieval process, you can create a generic function. This function will accept the data structure and a path as input parameters. Here is a sample implementation:
“`python
def retrieve_value(data, path):
for key in path:
data = data[key] if isinstance(data, dict) else data[int(key)]
return data
“`
Usage Example:
“`python
nested_data = {
‘a’: [{‘b’: 1}, {‘c’: 2}],
‘d’: {‘e’: 3}
}
value = retrieve_value(nested_data, [‘a’, ‘0’, ‘b’]) Returns 1
value2 = retrieve_value(nested_data, [‘d’, ‘e’]) Returns 3
“`
Best Practices for Working with Nested Structures
When dealing with nested data structures, consider the following best practices:
- Clear Naming Conventions: Use descriptive names for keys and variables to ensure readability.
- Error Handling: Implement error handling to manage cases where keys or indexes do not exist.
- Documentation: Document the structure and expected data types to facilitate understanding and maintenance.
Example of Error Handling:
“`python
def safe_retrieve_value(data, path):
try:
return retrieve_value(data, path)
except (KeyError, IndexError):
return None Or handle the error as appropriate
“`
Performance Considerations
Accessing deeply nested structures can lead to performance issues, especially in large datasets. To mitigate this:
- Flatten Data When Possible: If the data structure allows, consider flattening the data to reduce nesting complexity.
- Use Libraries: For extensive nested data manipulations, consider using libraries like `pandas` or `jsonpath-ng`, which provide optimized methods for data access.
This structured approach to understanding and manipulating nested data in Python enhances both the performance and maintainability of your code.
Expert Insights on Navigating Nested Data Structures in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Understanding nested data structures in Python is crucial for efficient data retrieval. Utilizing libraries like JSON or Pandas can simplify the process of accessing deeply nested values, allowing for more streamlined data manipulation and analysis.”
James Liu (Software Architect, CodeCraft Solutions). “When dealing with nested data structures, defining clear paths for data retrieval is essential. Implementing functions that can traverse these structures recursively can enhance code readability and maintainability, ultimately leading to fewer bugs and easier updates.”
Sarah Thompson (Python Developer, Open Source Community). “The ability to efficiently retrieve values from nested data structures in Python is a skill that can significantly impact performance. Leveraging tools like list comprehensions and dictionary methods can optimize access patterns, making your code not only faster but also more elegant.”
Frequently Asked Questions (FAQs)
What is a nested data structure in Python?
A nested data structure in Python refers to a data structure that contains other data structures as its elements. Common examples include lists of lists, dictionaries containing lists, or dictionaries containing other dictionaries.
How can I record a nested data structure in Python?
To record a nested data structure, you can define it using Python’s built-in data types, such as lists and dictionaries. For instance, a dictionary can hold lists as values, and those lists can contain dictionaries, creating a multi-layered structure.
What is the best way to retrieve a value from a nested data structure?
To retrieve a value from a nested data structure, you can use a combination of indexing and key access. For example, if you have a dictionary containing lists, you would first access the dictionary key, then the list index to get the desired value.
Can you provide an example of accessing a value in a nested dictionary?
Certainly. For a nested dictionary like `data = {‘key1’: {‘subkey1’: ‘value1’}}`, you can access ‘value1’ using `data[‘key1’][‘subkey1’]`.
How do I handle missing keys when retrieving values from a nested structure?
You can handle missing keys using the `get()` method, which allows you to specify a default value if the key does not exist. For example, `data.get(‘key1’, {}).get(‘subkey1’, ‘default_value’)` will return ‘default_value’ if ‘key1’ or ‘subkey1’ is missing.
What libraries can assist with managing nested data structures in Python?
Libraries such as `pandas` and `json` can assist with managing nested data structures. `pandas` provides DataFrames that can handle complex data, while `json` allows for easy manipulation of JSON-like nested structures.
In the realm of Python programming, nested data structures such as dictionaries and lists play a pivotal role in organizing and managing complex data. These structures allow developers to create records that can hold multiple layers of information, making it easier to store related data in a hierarchical format. Understanding how to effectively navigate these nested structures is essential for retrieving values accurately and efficiently.
Retrieving values from nested data structures involves utilizing appropriate methods to access each level of the hierarchy. This often requires a clear understanding of the data’s structure and the paths that lead to the desired data points. By employing techniques such as indexing for lists and key referencing for dictionaries, programmers can extract values seamlessly. Additionally, Python’s built-in functions and libraries can enhance this process, providing robust tools for data manipulation.
Key takeaways from the discussion emphasize the importance of mastering nested data structures for effective data management in Python. Developers should familiarize themselves with various methods of accessing and manipulating these structures to improve code efficiency and readability. Furthermore, leveraging Python’s capabilities can lead to more organized code, ultimately enhancing overall software development practices.
Author Profile
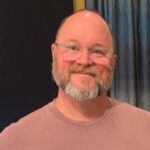
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?