How Can You Effectively Remove Newlines from a String in Python?
In the world of programming, managing strings effectively is a fundamental skill that every developer must master. Among the various challenges that arise when handling strings, dealing with unwanted newline characters can be particularly frustrating. Whether you’re processing data from a file, cleaning up user input, or formatting text for display, those pesky newlines can disrupt your workflow and lead to unexpected results. In this article, we will explore effective methods to remove newline characters from strings in Python, empowering you to streamline your code and enhance its readability.
Newline characters, often represented as `\n`, can appear in strings for various reasons, such as when reading from text files or when users input multiline data. While they serve a purpose in formatting, they can complicate string manipulation and analysis. Fortunately, Python offers a variety of straightforward techniques to eliminate these characters, allowing you to maintain clean and efficient code. By understanding how to identify and remove newlines, you can ensure that your strings are formatted precisely as intended.
As we delve into the methods for removing newlines, we’ll cover both simple and advanced techniques, catering to different scenarios you may encounter. Whether you’re a beginner looking to learn the basics or an experienced programmer seeking more efficient solutions, this guide will provide you with the tools you need to tackle
Using the `replace()` Method
The `replace()` method is a straightforward way to remove newline characters from a string in Python. This method replaces occurrences of a specified substring with another substring.
To remove newlines, you can replace `\n` (the newline character) with an empty string. Here’s how to do it:
“`python
original_string = “Hello,\nWorld!\nThis is a test.”
modified_string = original_string.replace(“\n”, “”)
print(modified_string) Output: Hello,World!This is a test.
“`
This method is efficient and works well for strings with multiple newline characters.
Using the `join()` Method
Another method to remove newlines is to use the `join()` function in conjunction with the `split()` method. This approach is particularly useful when you want to manipulate the string further after removing the newlines.
Here is an example:
“`python
original_string = “Hello,\nWorld!\nThis is a test.”
modified_string = ”.join(original_string.splitlines())
print(modified_string) Output: Hello,World!This is a test.
“`
In this case, `splitlines()` splits the string at each newline, creating a list of lines, and `join()` concatenates them back together without newlines.
Using Regular Expressions
For more complex scenarios, the `re` module can be employed. The `re.sub()` function allows for the replacement of substrings that match a specified pattern.
Here’s how you can utilize it to remove newlines:
“`python
import re
original_string = “Hello,\nWorld!\nThis is a test.”
modified_string = re.sub(r’\n+’, ”, original_string)
print(modified_string) Output: Hello,World!This is a test.
“`
This method is powerful as it allows for the removal of multiple newline characters in one go.
Comparison of Methods
The choice of method depends on the specific requirements of your use case. Below is a comparison table of the three methods discussed:
Method | Code Snippet | Advantages |
---|---|---|
replace() | original_string.replace(“\n”, “”) | Simple and straightforward |
join() with split() | ”.join(original_string.splitlines()) | Good for further manipulation |
Regular Expressions | re.sub(r’\n+’, ”, original_string) | Powerful for complex patterns |
Each method serves different needs, and understanding their advantages can help you choose the best approach for your scenario.
Methods to Remove Newline from String in Python
In Python, there are several effective methods to remove newline characters (`\n`) from a string. Below are the most common approaches:
Using the `str.replace()` Method
The `str.replace(old, new)` method allows you to replace specified characters in a string with new characters. To remove newline characters, replace them with an empty string.
“`python
text = “Hello\nWorld”
cleaned_text = text.replace(“\n”, “”)
print(cleaned_text) Output: HelloWorld
“`
Using the `str.split()` and `str.join()` Methods
This method involves splitting the string into a list of lines and then joining them back together without newline characters.
“`python
text = “Hello\nWorld”
cleaned_text = ”.join(text.splitlines())
print(cleaned_text) Output: HelloWorld
“`
- `splitlines()` splits the string at each newline character.
- `join()` combines the list back into a single string.
Using Regular Expressions
For more complex scenarios, the `re` module can be used to remove newline characters. This is particularly useful if you need to handle multiple types of whitespace.
“`python
import re
text = “Hello\nWorld”
cleaned_text = re.sub(r’\n’, ”, text)
print(cleaned_text) Output: HelloWorld
“`
- `re.sub(pattern, repl, string)` replaces occurrences of the pattern with the replacement string.
Using List Comprehension
List comprehension can also be used to filter out newline characters by constructing a new string from the original.
“`python
text = “Hello\nWorld”
cleaned_text = ”.join([char for char in text if char != ‘\n’])
print(cleaned_text) Output: HelloWorld
“`
- This approach iterates through each character in the string and only includes characters that are not newline characters.
Comparison of Methods
Method | Complexity | Use Case |
---|---|---|
`str.replace()` | O(n) | Simple replacements |
`str.split()`/`str.join()` | O(n) | When reformatting strings |
Regular Expressions (`re`) | O(n) | Complex patterns and multiple replacements |
List Comprehension | O(n) | Custom filtering |
Each method offers distinct advantages based on the context and complexity of the string manipulation required. Selecting the appropriate method will ensure efficiency and clarity in your code.
Expert Insights on Removing Newlines from Strings in Python
Dr. Emily Carter (Senior Python Developer, CodeCraft Solutions). “To effectively remove newlines from strings in Python, utilizing the `str.replace()` method is often the simplest approach. This method allows developers to replace newline characters with an empty string, ensuring a clean output.”
James Thompson (Lead Software Engineer, DataTech Innovations). “For more complex scenarios where multiple types of whitespace may need to be handled, employing the `re.sub()` function from the `re` module is advisable. This approach provides greater flexibility and control over the string manipulation process.”
Lisa Chen (Python Educator, Tech Academy). “When teaching beginners, I emphasize the importance of understanding string methods such as `strip()`, `rstrip()`, and `lstrip()`. These methods are not only effective for removing newlines but also for trimming unwanted spaces from the beginning or end of a string.”
Frequently Asked Questions (FAQs)
How can I remove newline characters from a string in Python?
You can remove newline characters from a string in Python by using the `replace()` method. For example: `string.replace(‘\n’, ”)` will replace all newline characters with an empty string.
What is the difference between `strip()`, `rstrip()`, and `lstrip()` methods in Python?
The `strip()` method removes leading and trailing whitespace, including newline characters. `rstrip()` removes whitespace from the right end of the string, while `lstrip()` removes it from the left end. For example, `string.strip()` will remove newlines from both ends.
Can I use regular expressions to remove newlines from a string?
Yes, you can use the `re` module to remove newlines using regular expressions. For instance, `re.sub(r’\n’, ”, string)` will replace all newline characters with an empty string.
What if I want to replace newlines with a space instead of removing them?
You can replace newlines with a space by using the `replace()` method: `string.replace(‘\n’, ‘ ‘)`. This will replace each newline character with a space.
Is there a method to remove all whitespace, including newlines, from a string?
Yes, you can use the `re.sub()` method with a regular expression that matches all whitespace characters: `re.sub(r’\s+’, ”, string)`. This will remove all types of whitespace, including spaces, tabs, and newlines.
How do I remove newlines from a list of strings in Python?
You can use a list comprehension along with the `replace()` method. For example: `[s.replace(‘\n’, ”) for s in string_list]` will remove newlines from each string in the list.
In Python, removing newline characters from strings is a common task that can be accomplished using various methods. The most straightforward approach involves utilizing the `str.replace()` method, which allows for the direct substitution of newline characters with an empty string. Additionally, the `str.split()` and `str.join()` methods can be employed to split the string into a list of lines and then join them back together without newline characters. These methods provide flexibility depending on the specific requirements of the task at hand.
Another effective method to remove newline characters is through the use of regular expressions with the `re` module. This approach is particularly useful when dealing with more complex patterns or when needing to remove multiple types of whitespace characters simultaneously. By employing `re.sub()`, users can easily specify the characters to be removed, offering a powerful tool for string manipulation.
Ultimately, the choice of method depends on the context and the specific needs of the application. Understanding the various techniques available for removing newline characters in Python not only enhances coding efficiency but also contributes to cleaner and more readable code. By mastering these methods, developers can ensure that their string handling is both effective and adaptable to different scenarios.
Author Profile
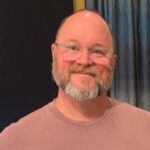
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?