How Can You Print Quotes in Python Like a Pro?
In the world of programming, the simplest tasks often hold the key to mastering a language. One such fundamental skill in Python is the ability to print quotes—an essential technique that not only enhances your coding repertoire but also enriches your understanding of how strings and outputs work. Whether you’re a beginner eager to learn the ropes or an experienced coder brushing up on the basics, knowing how to effectively print quotes can serve as a stepping stone to more complex programming concepts.
Printing quotes in Python is more than just displaying text on the screen; it’s about understanding how to manipulate strings, manage formatting, and utilize escape characters. From single quotes to double quotes, and even triple quotes for multi-line strings, each variation serves a unique purpose and can be leveraged in different scenarios. This article will guide you through the nuances of printing quotes, ensuring you grasp the underlying principles while also providing practical examples to solidify your learning.
As you delve deeper into the intricacies of Python’s print function, you’ll discover how to seamlessly integrate quotes within your code, manage potential pitfalls, and even explore advanced formatting options. Whether you’re aiming to create user-friendly outputs or simply want to impress your peers with your coding finesse, mastering the art of printing quotes is an essential skill that will enhance your programming
Using Print Function to Display Quotes
In Python, the primary method for outputting text to the console is through the `print()` function. To display quotes, you can directly include them within the parentheses of the `print()` function. Here are some examples of how to correctly print quotes:
- To print a single quote:
“`python
print(‘This is a single quote: \’ ‘)
“`
- To print a double quote:
“`python
print(“This is a double quote: \” “)
“`
- To print a string that contains both single and double quotes:
“`python
print(“She said, ‘Hello!’ and I replied, \”Hi!\””)
“`
Escaping Quotes
If your string contains the same type of quote as the delimiters, you need to escape them using a backslash (`\`). This ensures Python interprets them correctly rather than ending the string. Here’s how it works:
- Printing a string with an embedded single quote:
“`python
print(‘It\’s a sunny day!’)
“`
- Printing a string with an embedded double quote:
“`python
print(“He said, \”Python is great!\””)
“`
Using Triple Quotes
Another way to print strings containing quotes is by using triple quotes. Triple quotes can be either single or double quotes, and they allow for multi-line strings as well.
- Using triple single quotes:
“`python
print(”’This is a multi-line string.
It can include ‘single’ and “double” quotes without escaping.”’)
“`
- Using triple double quotes:
“`python
print(“””This is another multi-line string.
It can also include ‘single’ and “double” quotes effortlessly.”””)
“`
Formatted Strings
Python also supports formatted strings, which can be particularly useful for dynamically including quotes in output. You can use f-strings (formatted string literals) introduced in Python 3.6.
Example:
“`python
name = “Alice”
print(f”{name} said, ‘Hello, World!'”)
“`
Table of Quotes Printing Methods
The following table summarizes the methods discussed for printing quotes in Python:
Method | Description | Example |
---|---|---|
Basic Print | Directly print quotes. | print(“Hello, World!”) |
Escaping Quotes | Use backslash to escape quotes. | print(‘It\’s a test.’) |
Triple Quotes | Use triple quotes for multi-line or embedded quotes. | print(”’She said, “Hello!””’) |
Formatted Strings | Include variables in strings. | print(f”Name: {name}”) |
These methods provide flexibility in how quotes are presented in the output, allowing for a variety of formatting options based on your specific needs.
Using Print Function to Output Quotes
In Python, the `print()` function is the primary method for displaying output to the console, including quotes. The syntax for the `print()` function is straightforward, and it accepts one or more arguments, which can be strings, numbers, or other objects.
To print quotes, you can use single quotes, double quotes, or triple quotes, depending on your needs. Here are the methods to print quotes:
Single and Double Quotes
You can use single (`’`) or double (`”`) quotes interchangeably in Python. When you want to include quotes within your output, you must ensure they do not conflict with the string delimiters.
- Single Quotes Example:
“`python
print(‘Hello, “World!”‘)
“`
- Double Quotes Example:
“`python
print(“Hello, ‘World!'”)
“`
In the examples above, the quotes within the string do not interfere with the outer quotes.
Escaping Quotes
If you need to include the same type of quotes as the delimiters, you can escape them using a backslash (`\`). This allows you to include both types of quotes without causing a syntax error.
- Escaping Single Quotes:
“`python
print(‘It\’s a beautiful day!’)
“`
- Escaping Double Quotes:
“`python
print(“She said, \”Hello!\””)
“`
Triple Quotes for Multi-line Strings
Triple quotes (`”’` or `”””`) are particularly useful for multi-line strings or when you want to include both single and double quotes without escaping.
- Example of Triple Quotes:
“`python
print(“””This is a string that spans
multiple lines, and it can contain both ‘single’ and “double” quotes.”””)
“`
Formatted Strings
Python also provides f-strings (formatted string literals) for easier string formatting. This is particularly useful when you want to include variables within your quotes.
- Using f-Strings:
“`python
name = “Alice”
print(f”Hello, {name}. It’s a pleasure to meet you!”)
“`
Using the String Format Method
Another method for including quotes and formatting strings is using the `str.format()` method. This allows for greater control over the output.
- Example with str.format():
“`python
name = “Bob”
print(“Hello, {}. It’s great to see you!”.format(name))
“`
Summary Table of Quote Usage
Method | Syntax Example | Description |
---|---|---|
Single Quotes | `print(‘Hello, “World!”‘)` | Outputs double quotes inside single quotes. |
Double Quotes | `print(“Hello, ‘World!'”)` | Outputs single quotes inside double quotes. |
Escaped Quotes | `print(‘It\’s a day!’)` | Uses backslash to escape quotes. |
Triple Quotes | `print(“””Multi-line string.”””)` | Supports multi-line and both quote types. |
f-Strings | `print(f”Hello, {name}”)` | Allows variable interpolation. |
str.format() | `print(“Hello, {}”.format(name))` | Formats strings using placeholders. |
Utilizing these various methods, you can effectively print quotes in Python, ensuring clarity and precision in your output.
Expert Insights on Printing Quotes in Python
Dr. Emily Carter (Senior Software Engineer, CodeCrafters Inc.). “Printing quotes in Python is a fundamental skill that every programmer should master. Utilizing the print function effectively allows for clear communication of output, which is crucial for debugging and user interaction.”
Michael Chen (Python Instructor, Tech Academy). “Understanding how to print quotes in Python not only enhances your coding proficiency but also helps in learning about string manipulation. Using single or double quotes appropriately can prevent syntax errors and improve code readability.”
Laura Simmons (Lead Developer, Innovative Solutions). “When printing quotes in Python, it’s essential to be aware of escape characters. Mastering this concept allows developers to include quotes within strings without causing interruptions in the code execution.”
Frequently Asked Questions (FAQs)
How do I print a simple string in Python?
You can print a simple string in Python using the `print()` function. For example, `print(“Hello, World!”)` will display the text on the screen.
What is the syntax for printing variables in Python?
To print variables in Python, you can use the `print()` function along with the variable name. For instance, if you have a variable `name = “Alice”`, you can print it using `print(name)`.
How can I print multiple items in one line?
You can print multiple items in one line by separating them with commas in the `print()` function. For example, `print(“Hello,”, “World!”)` will output `Hello, World!`.
What is the difference between single quotes and double quotes in Python?
In Python, single quotes (`’`) and double quotes (`”`) are functionally equivalent for defining strings. You can use either, but it’s important to be consistent and to use one type to enclose the other when including quotes within the string.
How can I include special characters or quotes in my printed output?
You can include special characters or quotes in your printed output by using escape sequences. For example, to print a double quote, use `\”`, like this: `print(“He said, \”Hello!\””)`.
Can I format strings while printing in Python?
Yes, you can format strings while printing in Python using f-strings (formatted string literals) or the `format()` method. For example, `name = “Alice”; print(f”Hello, {name}!”)` will output `Hello, Alice!`.
In summary, printing quotes in Python is a straightforward process that can be achieved using various methods. The most common approach involves using the print() function, which can display strings containing quotes directly. It is essential to understand how to handle different types of quotes, such as single quotes (‘ ‘) and double quotes (” “), to avoid syntax errors. Additionally, using escape characters, such as the backslash (\), allows for the inclusion of quotes within strings without disrupting the code structure.
Another useful technique is the use of triple quotes (”’ ”’ or “”” “””) for multi-line strings or when the string itself contains both single and double quotes. This flexibility not only enhances readability but also simplifies the code when dealing with complex string outputs. Furthermore, incorporating formatted string literals (f-strings) can improve the clarity and efficiency of printing quotes alongside variable content.
Ultimately, mastering these techniques for printing quotes in Python is crucial for effective programming. Understanding the nuances of string handling in Python will facilitate better communication of information through print statements. As you continue to explore Python, these foundational skills will serve as building blocks for more advanced programming concepts.
Author Profile
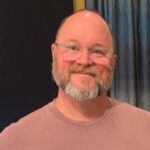
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?