What Does the Colon Symbol Mean in Python Programming?
In the world of Python programming, the colon (`:`) is more than just a punctuation mark; it serves as a pivotal symbol that dictates the structure and flow of code. Whether you’re a seasoned developer or a newcomer to the language, understanding the role of the colon is essential for writing clean, efficient, and functional Python code. From defining functions and loops to creating conditional statements, the colon acts as a gateway that signals the beginning of a new block of code, guiding the interpreter and the programmer alike.
At its core, the colon is a syntax element that helps to delineate the boundaries of various code structures. When you encounter a colon in Python, it indicates that what follows is a block of code that belongs to a specific statement, such as an `if` condition, a `for` loop, or a function definition. This unique functionality not only enhances readability but also enforces a level of organization that is crucial for effective programming. The colon’s role in Python is a testament to the language’s design philosophy, which emphasizes simplicity and clarity.
As you delve deeper into the nuances of Python programming, you’ll discover that the colon is integral to mastering control flow and code organization. Its presence is a signal to the interpreter that it’s time to expect an indented block of code
Understanding the Role of Colons in Python
In Python, the colon (`:`) serves several critical functions that are essential for the structure and readability of the code. It is primarily used to indicate the start of an indented block of code. This is especially prominent in control flow statements, function definitions, and class definitions. Below are the main contexts in which colons are used:
- Control Flow Statements: In conditional statements such as `if`, `elif`, and `else`, the colon signals the beginning of the block that will execute if the condition is met.
- Loops: The colon is also used in loops (`for`, `while`) to denote the start of the block of code that will repeat.
- Function Definitions: When defining a function, the colon indicates the start of the function body.
- Class Definitions: Similarly, when creating a class, the colon marks the beginning of the class body.
- Dictionaries: In dictionary literals, the colon separates keys from their associated values.
Here’s a brief overview of these uses:
Context | Example | Description |
---|---|---|
Control Flow | if x > 0: | Starts a block of code that executes if the condition is true. |
Loop | for i in range(5): | Begins a block of code that will iterate over a sequence. |
Function Definition | def my_function(): | Indicates the start of a function body. |
Class Definition | class MyClass: | Denotes the start of a class body. |
Dictionary | {‘key’: ‘value’} | Separates the key from its value in a dictionary. |
Each use of the colon not only enhances the clarity of the code but also enforces Python’s indentation-based block structure. This design choice eliminates the need for braces or other delimiters, making Python code often more readable and visually appealing.
Furthermore, the consistent use of colons throughout Python code helps in maintaining a clean syntax. For instance, the following code snippets illustrate the various uses of colons in different programming constructs:
“`python
Control Flow
if temperature > 100:
print(“It’s too hot!”)
Loop
for number in range(5):
print(number)
Function Definition
def greet(name):
print(f”Hello, {name}!”)
Class Definition
class Animal:
def speak(self):
print(“Animal sound”)
“`
In each of these examples, the colon plays a pivotal role in defining where a block of code begins, which is crucial for maintaining the flow and structure of the program. Understanding the significance of the colon in Python is key to writing clear and effective code.
Understanding the Role of Colon in Python
In Python, the colon (`:`) is a syntactical element that serves several important functions within the language’s structure. It is primarily used to denote the start of an indented block of code, which is essential for defining control structures, function definitions, and class declarations.
Key Uses of Colon in Python
The colon is utilized in various contexts, including:
- Function Definitions: It marks the beginning of the function body.
“`python
def my_function():
print(“Hello, World!”)
“`
- Conditional Statements: It precedes the block of code that executes if the condition is true.
“`python
if condition:
do_something()
“`
- Loops: It indicates the start of the loop body.
“`python
for item in iterable:
process(item)
“`
- Class Definitions: It denotes the beginning of the class body.
“`python
class MyClass:
def method(self):
pass
“`
- Exception Handling: It is used in try-except blocks to separate the try block from the except block.
“`python
try:
risky_operation()
except SomeException:
handle_exception()
“`
Colons in Data Structures
Colons also appear in data structures, particularly in dictionaries and slices:
- Dictionaries: In key-value pairs, the colon separates the key from the value.
“`python
my_dict = {“key”: “value”}
“`
- Slicing: In list slicing, colons define the start and end indices.
“`python
my_list = [0, 1, 2, 3, 4]
sliced = my_list[1:3] Returns [1, 2]
“`
Syntax Rules Involving Colons
When using colons in Python, several syntax rules must be followed:
Context | Usage Example | Description |
---|---|---|
Function Definition | `def func_name():` | Begins the function body. |
Control Statements | `if condition:` | Starts the block to execute if the condition is true. |
Loop Statements | `while condition:` | Initiates the loop body. |
Class Definition | `class ClassName:` | Starts the class body. |
Dictionary Definition | `my_dict = {key: value}` | Separates keys from values. |
Slicing | `my_list[start:end]` | Specifies the slice of the list. |
Conclusion on Colons in Python Syntax
The colon is a fundamental component of Python syntax, influencing the structure and readability of code. Its proper use is critical for defining code blocks and data structures, ensuring clarity and functionality in Python programming.
Understanding the Role of the Colon in Python Programming
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). The colon in Python serves as a critical syntactical element that indicates the start of an indented block of code. It is essential for defining constructs such as functions, loops, and conditional statements, thereby enhancing code readability and structure.
Michael Thompson (Lead Python Developer, Tech Solutions Group). In Python, the colon is not merely a punctuation mark; it signifies the beginning of a new scope. This is particularly important in defining functions and control flow statements, as it delineates where the block of code associated with that statement begins, ensuring proper execution.
Sarah Johnson (Python Programming Instructor, LearnCode Academy). Understanding the function of the colon in Python is vital for any programmer. It indicates that the following indented lines belong to the preceding statement, which is crucial for maintaining the logical structure of the code and preventing errors in execution.
Frequently Asked Questions (FAQs)
What does a colon signify in Python?
The colon in Python is used to indicate the start of an indented block of code. It is commonly found in control structures such as if statements, loops, and function definitions.
How is a colon used in function definitions?
In function definitions, a colon is placed at the end of the function header to denote the beginning of the function body, which must be indented.
What role does a colon play in conditional statements?
In conditional statements, the colon follows the condition and precedes the block of code that executes if the condition evaluates to true.
Can you provide an example of using a colon in a loop?
Certainly. In a for loop, the colon is placed after the loop declaration, such as `for i in range(5):`, indicating that the following indented lines are part of the loop’s body.
Is a colon required in Python syntax?
Yes, a colon is mandatory in specific constructs like function definitions, loops, and conditional statements. Omitting it will result in a syntax error.
Are there any other contexts where a colon is used in Python?
Yes, colons are also used in dictionary key-value pairs and in slicing operations for lists and strings, specifying the start and end indices.
The colon in Python serves multiple critical functions that are essential for the language’s syntax and structure. Primarily, it is used to indicate the beginning of an indented block of code, which is fundamental in defining control structures such as loops and conditionals. For instance, after an `if`, `for`, or `while` statement, a colon signifies that the subsequent lines will be part of the block that executes based on the condition or iteration specified. This use of indentation, paired with the colon, is a distinctive feature of Python that enhances readability and enforces a clean coding style.
Additionally, colons are utilized in function definitions and class declarations, marking the start of the function body or class attributes. This consistency in usage reinforces the importance of colons in Python’s object-oriented programming paradigm. Furthermore, colons are also employed in dictionary definitions and slicing operations, showcasing their versatility across different contexts within the language.
In summary, the colon is a fundamental aspect of Python’s syntax that plays a crucial role in defining the structure and flow of code. Understanding its various applications is essential for effective programming in Python. By recognizing the significance of the colon, developers can write cleaner, more efficient code while adhering to Python’s stylistic conventions
Author Profile
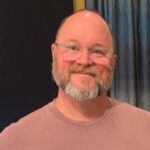
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?