How Can You Effectively Comment Out a Section in Python?
In the world of programming, clarity and organization are paramount. As you delve into Python, one of the most popular and versatile programming languages, you may find yourself needing to manage your code effectively. One essential skill every Python programmer should master is the ability to comment out sections of code. Whether you’re debugging, collaborating with others, or simply trying to make your code more readable, knowing how to comment out a section can save you time and frustration. In this article, we will explore the techniques and best practices for commenting out code in Python, ensuring that your projects remain clean and comprehensible.
Commenting out code is a fundamental practice that allows developers to temporarily disable certain lines without deleting them. This can be particularly useful when testing different parts of your code or when you want to leave notes for yourself or your teammates. In Python, there are specific conventions for adding comments, and understanding these will enhance your coding workflow. We’ll discuss the various methods available, from single-line comments to multi-line options, and how they can be effectively utilized to streamline your coding process.
Moreover, commenting is not just about making your code easier to read; it’s also about fostering collaboration and communication among developers. By learning the best practices for commenting out sections in Python, you’ll
Commenting in Python
In Python, commenting is an essential practice that helps in documenting code and making it more understandable. There are primarily two methods to comment in Python: single-line comments and multi-line comments.
Single-Line Comments
Single-line comments in Python are initiated with the “ symbol. Any text following this symbol on the same line will be treated as a comment and ignored during execution. This is useful for brief explanations or notes.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a message
“`
Multi-Line Comments
To comment out multiple lines in Python, there are two common approaches:
- Using Triple Quotes: Python allows the use of triple quotes (`”’` or `”””`) for multi-line strings, which can effectively act as multi-line comments when not assigned to a variable. Although not strictly a comment syntax, this method is widely used.
Example:
“`python
”’
This is a multi-line comment.
It spans several lines.
”’
print(“Hello, World!”)
“`
- Using Multiple Single-Line Comments: Another method is to use the “ symbol at the beginning of each line that you wish to comment out.
Example:
“`python
This is the first line of a multi-line comment
This is the second line
This is the third line
print(“Hello, World!”)
“`
Best Practices for Commenting
Effective commenting enhances code readability and maintainability. Here are some best practices:
- Be Clear and Concise: Comments should be straightforward and directly related to the code they describe.
- Avoid Obvious Comments: Refrain from stating the obvious; instead, focus on the intent or purpose of the code.
- Update Comments: Ensure that comments are maintained and updated as the code changes. Outdated comments can lead to confusion.
Comparison of Commenting Methods
The following table summarizes the differences between the two primary methods of multi-line commenting:
Method | Syntax | Use Case |
---|---|---|
Triple Quotes | ”’ or “”” | Good for longer explanations or temporarily disabling blocks of code. |
Multiple Single-Line Comments | Ideal for quick, inline comments and clarity on specific lines. |
By following these guidelines and understanding the different methods available, programmers can effectively comment out sections of code in Python, improving both documentation and collaboration within development teams.
Commenting Out Single Lines
In Python, you can comment out a single line of code by using the hash symbol (“). This symbol indicates that everything following it on that line is a comment and will not be executed.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This part won’t affect the output
“`
Commenting Out Multiple Lines
To comment out multiple lines in Python, you can use consecutive hash symbols for each line. Alternatively, you can use triple quotes (`”’` or `”””`) to create a multi-line string that is not assigned to any variable. However, be cautious, as this method does not technically create comments but rather a string that will not be used.
Using hash symbols:
“`python
This is the first line of a comment
This is the second line of a comment
print(“This will execute.”)
“`
Using triple quotes:
“`python
”’
This is a multi-line string that acts as a comment.
It won’t be executed.
”’
print(“Only this line executes.”)
“`
Best Practices for Commenting
When commenting out sections of code, consider the following best practices to ensure clarity and maintainability:
- Be Clear and Concise: Write comments that explain why the code exists, not just what it does.
- Avoid Obvious Comments: Do not comment on code that is self-explanatory.
- Keep Comments Updated: Ensure comments reflect the current state of the code after any modifications.
- Use Comments Sparingly: Over-commenting can clutter the code. Only comment when necessary.
Use Cases for Commenting Out Code
Commenting out code can be particularly useful in various situations, such as:
Use Case | Description |
---|---|
Debugging | Temporarily disable sections of code to isolate issues. |
Experimentation | Test different approaches without permanently deleting code. |
Collaboration | Provide explanations for sections that may need clarification for team members. |
Code Refactoring | Mark sections that require future improvement without removing them. |
Tools and IDE Support
Many Integrated Development Environments (IDEs) and code editors provide shortcuts for commenting out code:
IDE/Editor | Shortcut for Commenting Out Code |
---|---|
PyCharm | Ctrl + / (Windows/Linux), Cmd + / (Mac) |
Visual Studio Code | Ctrl + / (Windows/Linux), Cmd + / (Mac) |
Jupyter Notebook | Ctrl + / (Windows/Linux), Cmd + / (Mac) |
Atom | Ctrl + / (Windows/Linux), Cmd + / (Mac) |
Utilizing these shortcuts can significantly enhance your efficiency when working with code.
Expert Insights on Commenting Out Sections in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out sections of code in Python is essential for debugging and maintaining code clarity. Utilizing the hash symbol () allows developers to easily disable specific lines or blocks without deleting them, which is crucial during the development process.”
James Liu (Python Developer, Open Source Advocate). “For larger blocks of code, using triple quotes (”’ or “””) can be an effective way to comment out multiple lines. This method not only enhances readability but also allows for quick toggling of code sections during testing.”
Sarah Thompson (Lead Instructor, Python Programming Bootcamp). “It’s important for beginners to understand the significance of commenting out code. It not only helps in isolating errors but also serves as a form of documentation, making it easier for others to understand the developer’s thought process.”
Frequently Asked Questions (FAQs)
How do I comment out a single line in Python?
To comment out a single line in Python, use the hash symbol (“) at the beginning of the line. Everything following the “ on that line will be treated as a comment.
Can I comment out multiple lines in Python?
Yes, you can comment out multiple lines by placing a “ at the beginning of each line. Alternatively, you can use triple quotes (`”’` or `”””`) to create a multi-line string that is not assigned to any variable, effectively acting as a comment.
What is the purpose of commenting out code in Python?
Commenting out code helps in debugging and testing by allowing developers to disable specific sections of code without deleting them. It also aids in making the code more understandable for others by providing explanations.
Are there any best practices for commenting in Python?
Best practices include writing clear and concise comments that explain the purpose of the code, avoiding obvious comments, and maintaining comments as the code evolves to ensure they remain relevant.
Can I use comments in Python scripts to improve code readability?
Yes, well-placed comments can significantly enhance code readability by providing context and explanations for complex logic, making it easier for others (or yourself) to understand the code later.
Is there a difference between comments and docstrings in Python?
Yes, comments are used to explain code and are not executed, while docstrings are special strings used to document modules, classes, and functions. Docstrings can be accessed programmatically and are typically enclosed in triple quotes.
In Python, commenting out a section of code is a crucial practice that enhances code readability and maintainability. The primary method for commenting in Python involves using the hash symbol () for single-line comments. For multi-line comments, developers typically use triple quotes (”’ or “””) to enclose the block of text. This approach allows for clear documentation of code sections, making it easier for others (or oneself) to understand the purpose and functionality of the code during future reviews or modifications.
Another important aspect to consider is the role of comments in debugging and development. By commenting out sections of code, developers can isolate issues and test specific functionalities without permanently removing code. This practice is invaluable during the development process, as it allows for iterative testing and refinement of algorithms while preserving the original code structure.
In summary, effectively utilizing comments in Python not only aids in clarifying the intent of the code but also facilitates smoother collaboration among developers. By adopting a consistent commenting strategy, programmers can ensure their code is accessible and comprehensible, ultimately leading to more efficient programming practices and improved project outcomes.
Author Profile
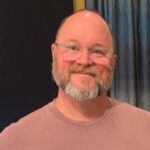
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?