How Can You Run a PowerShell Script From Another PowerShell Script?
In the world of automation and system administration, PowerShell stands out as a powerful scripting language that allows users to manage and configure systems with remarkable efficiency. One of the most compelling features of PowerShell is its ability to run scripts from within other scripts, enabling a modular approach to automation tasks. This capability not only enhances code reusability but also streamlines complex workflows, making it easier for administrators and developers to maintain and scale their scripts. Whether you’re orchestrating a series of tasks or simply want to keep your code organized, understanding how to run a PowerShell script from another script is an essential skill that can elevate your scripting game.
When you invoke one PowerShell script from another, you unlock a plethora of possibilities for task automation. This technique allows you to compartmentalize your code, breaking down larger scripts into manageable segments that can be executed independently or in sequence. By leveraging this functionality, you can create a library of reusable scripts that can be called upon as needed, significantly reducing redundancy and improving maintainability.
Moreover, running scripts from other scripts can help you handle complex scenarios where different scripts perform distinct functions but need to work together seamlessly. This approach not only saves time but also minimizes the potential for errors, as each script can be tested and debugged
Executing a Script from Another Script
To run a PowerShell script from within another script, you can utilize the `&` (call operator) or the `Start-Process` cmdlet. The choice between these methods typically depends on whether you want to run the script in the same session or as a new process.
Using the call operator `&` executes the script in the current session, allowing for the direct use of any variables or functions defined in the parent script. The syntax is straightforward:
“`powershell
& “Path\To\YourScript.ps1”
“`
Alternatively, if you need the script to run in a separate process, which is beneficial for isolation or when dealing with different execution policies, you can use `Start-Process`. This method is particularly useful when you want to pass arguments to the script:
“`powershell
Start-Process powershell -ArgumentList “-File ‘Path\To\YourScript.ps1′”
“`
Passing Arguments Between Scripts
When invoking another script, you might want to pass parameters to it. This can be accomplished by defining parameters in the called script and specifying them in the calling script. Here’s how you can do this:
In the child script (`YourScript.ps1`):
“`powershell
param(
[string]$Name,
[int]$Age
)
Write-Output “Name: $Name, Age: $Age”
“`
In the parent script:
“`powershell
& “Path\To\YourScript.ps1” -Name “John Doe” -Age 30
“`
This approach allows for dynamic input, making your scripts more flexible and reusable.
Using the `Invoke-Expression` Cmdlet
Another method to execute a script from another script is through `Invoke-Expression`. This cmdlet evaluates a string as a command. While powerful, it should be used with caution due to potential security risks associated with executing arbitrary strings.
Example of using `Invoke-Expression`:
“`powershell
$scriptPath = “Path\To\YourScript.ps1”
Invoke-Expression “& ‘$scriptPath'”
“`
Considerations for Script Execution
When running scripts from another script, consider the following factors:
- Execution Policy: Ensure that the execution policy allows for script execution. You may need to set the policy to `RemoteSigned` or `Unrestricted`.
- Script Location: Use full paths to avoid errors related to relative paths.
- Error Handling: Implement error handling to manage failures gracefully. You can use `try-catch` blocks.
Method | Session Type | Use Case |
---|---|---|
Call Operator (&) | Same Session | Access to parent script variables |
Start-Process | New Process | Isolation, different execution policies |
Invoke-Expression | Same Session | Dynamic command execution |
By understanding these methods and considerations, you can effectively manage the execution of PowerShell scripts from one another, enhancing modularity and maintainability in your scripting practices.
Invoking PowerShell Scripts
To run a PowerShell script from another PowerShell script, you can use the `&` (call operator) or the `Start-Process` cmdlet. Both methods enable you to execute a script located at a specific path.
Using the Call Operator
The call operator is the most straightforward method to invoke another script. Here’s the syntax:
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
This command runs the specified script in the current PowerShell session. If the script requires parameters, you can pass them directly after the script path:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
Using Start-Process
`Start-Process` allows for more control over how the script is executed, including running it in a new window. The basic syntax is:
“`powershell
Start-Process powershell -ArgumentList “-File C:\Path\To\YourScript.ps1”
“`
This command launches a new PowerShell process to run the script. For scripts that require parameters, include them in the `-ArgumentList` parameter:
“`powershell
Start-Process powershell -ArgumentList “-File C:\Path\To\YourScript.ps1 -Param1 Value1 -Param2 Value2”
“`
Handling Execution Policies
When executing scripts, you may encounter restrictions based on PowerShell’s execution policy. To check the current execution policy, use:
“`powershell
Get-ExecutionPolicy
“`
Common execution policies include:
Policy Name | Description |
---|---|
Restricted | No scripts can be run. |
AllSigned | Only scripts signed by a trusted publisher can be run. |
RemoteSigned | Scripts created locally can run; remote scripts must be signed. |
Unrestricted | All scripts can run. |
To change the execution policy, use:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Make sure to run PowerShell as an administrator if changing the policy.
Passing Variables Between Scripts
To share variables between scripts, you can define them in the calling script and pass them as parameters to the called script. For example:
In the calling script:
“`powershell
$myVar = “Hello”
& “C:\Path\To\YourScript.ps1” -Message $myVar
“`
In `YourScript.ps1`:
“`powershell
param(
[string]$Message
)
Write-Output $Message
“`
This method allows for dynamic data sharing, enhancing the modularity of your scripts.
Handling Output and Errors
You can capture output and errors from a called script by using the following techniques:
- Capturing Output:
“`powershell
$output = & “C:\Path\To\YourScript.ps1”
“`
- Handling Errors:
To handle errors gracefully, use `try` and `catch` blocks:
“`powershell
try {
& “C:\Path\To\YourScript.ps1”
} catch {
Write-Error “An error occurred: $_”
}
“`
This approach ensures that your primary script can continue running or exit gracefully based on the success or failure of the called script.
Implementing these methods allows for efficient execution of PowerShell scripts from other scripts, enhancing automation and script organization.
Expert Insights on Running PowerShell Scripts from Another Script
Dr. Emily Carter (Senior PowerShell Developer, Tech Innovations Inc.). “When executing a PowerShell script from another script, it is essential to utilize the `&` call operator or the `Start-Process` cmdlet. This ensures that the script runs in the correct context and allows for better error handling and output management.”
Michael Thompson (IT Automation Specialist, FutureTech Solutions). “Using the `Invoke-Expression` cmdlet can be a powerful way to run scripts dynamically. However, one must be cautious with this approach due to potential security risks associated with executing arbitrary code. Always validate input when using this method.”
Sarah Mitchell (PowerShell Scripting Expert, ScriptSavvy). “For modular script design, consider using functions and dot-sourcing. This allows you to load a script into the current session, making its functions available for immediate use without creating a new process, which can enhance performance and maintainability.”
Frequently Asked Questions (FAQs)
How can I run a PowerShell script from another PowerShell script?
You can run a PowerShell script from another script by using the `&` (call) operator followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What is the difference between using the call operator and dot-sourcing?
The call operator (`&`) runs the script in a new scope, meaning variables defined in the called script do not affect the calling script. Dot-sourcing (`. “C:\Path\To\YourScript.ps1″`) runs the script in the current scope, allowing variables and functions to persist.
Can I pass parameters to a PowerShell script when calling it from another script?
Yes, you can pass parameters by including them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What happens if the called script encounters an error?
If the called script encounters an error, it will terminate unless error handling is implemented. You can use `try` and `catch` blocks to manage errors effectively.
Is it possible to run scripts located on remote machines?
Yes, you can run scripts on remote machines using PowerShell Remoting. Use the `Invoke-Command` cmdlet with the `-ScriptBlock` parameter to execute scripts remotely.
Do I need special permissions to run scripts from another script?
Yes, you may need appropriate execution policies set in PowerShell. Use `Set-ExecutionPolicy` to adjust the policy, ensuring your scripts are permitted to run.
In summary, executing a PowerShell script from another PowerShell script is a straightforward process that can significantly enhance the modularity and reusability of code. By utilizing the `&` (call) operator or the `Invoke-Expression` cmdlet, users can seamlessly run external scripts, allowing for more organized and efficient scripting practices. This capability is particularly useful in complex automation tasks where breaking down scripts into smaller, manageable components can lead to improved clarity and maintainability.
Moreover, it is essential to consider the context in which the scripts are executed. The execution policy settings in PowerShell can affect whether scripts can run, so users should ensure that the appropriate policies are configured. Additionally, passing parameters between scripts can further enhance functionality, enabling scripts to share data and control flow effectively. Understanding how to manage these elements is crucial for anyone looking to leverage PowerShell for automation.
Ultimately, mastering the technique of running one PowerShell script from another not only streamlines workflows but also empowers users to build more sophisticated automation solutions. By embracing modular scripting, users can create a library of reusable scripts that can be easily maintained and updated, fostering a more efficient development environment. This strategic approach to scripting is invaluable for both novice and experienced PowerShell users
Author Profile
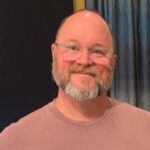
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?