How Can You Compile C Programs on Linux Like a Pro?
Compiling C code on Linux is a fundamental skill for programmers and developers alike, opening the door to a world of software development and system programming. Whether you’re a seasoned coder looking to brush up on your skills or a novice eager to dive into the intricacies of C, understanding how to effectively compile your code is crucial. The Linux environment, known for its powerful command-line interface and robust development tools, provides an ideal platform for compiling C programs. In this article, we will explore the essential steps and best practices for compiling C on Linux, empowering you to turn your code into executable programs with confidence.
To begin, it’s important to grasp the basic concepts of the compilation process. Compiling C code involves transforming human-readable source code into machine code that a computer can execute. This process typically consists of several stages, including preprocessing, compiling, assembling, and linking. Each of these stages plays a vital role in ensuring that your program runs smoothly and efficiently on the Linux operating system.
As we delve deeper into the topic, we’ll also discuss the tools and commands commonly used in the Linux environment for compiling C code. From the widely-used GCC (GNU Compiler Collection) to various flags that can optimize your compilation process, understanding these elements will enhance your coding experience. Whether
Setting Up Your Development Environment
To compile C programs on Linux, you first need to ensure that your development environment is properly set up. This includes installing the necessary tools and libraries. The most common compiler used for C programming on Linux is GCC (GNU Compiler Collection).
- To install GCC, you can use your distribution’s package manager. For example:
- On Ubuntu or Debian-based systems:
“`bash
sudo apt update
sudo apt install build-essential
“`
- On Fedora:
“`bash
sudo dnf install gcc gcc-c++
“`
- On Arch Linux:
“`bash
sudo pacman -S base-devel
“`
After installation, verify that GCC is installed correctly by checking the version:
“`bash
gcc –version
“`
Writing Your First C Program
Once your development environment is ready, you can start writing a simple C program. Use any text editor of your choice (like `nano`, `vim`, or `gedit`) to create a new file. For example, you might create a file named `hello.c`:
“`c
include
int main() {
printf(“Hello, World!\n”);
return 0;
}
“`
Save the file and ensure it is in a directory where you want to compile it.
Compiling the C Program
To compile your C program, navigate to the directory containing your source file in the terminal. Use the `gcc` command followed by the filename to compile your program:
“`bash
gcc hello.c -o hello
“`
In this command:
- `hello.c` is the source file.
- `-o hello` specifies the name of the output executable file. If omitted, the default name will be `a.out`.
Running the Compiled Program
After compilation, you can run the program by executing the output file. Use the following command:
“`bash
./hello
“`
This command runs the compiled C program, and you should see the output:
“`
Hello, World!
“`
Handling Compilation Errors
Compilation errors may occur if there are issues in your code. GCC will provide error messages that indicate the line number and type of error. Here are common types of errors:
- Syntax Errors: Mistakes in the syntax of the code.
- Type Errors: Using incompatible data types.
- Linker Errors: Issues related to missing libraries or functions.
Error Type | Description | Example |
---|---|---|
Syntax Error | Incorrect syntax in the code. | Missing semicolon at the end of a statement. |
Type Error | Using a variable of one type in a context where another type is expected. | Assigning a string to an integer variable. |
Linker Error | Missing libraries or unresolved references. | Using a function from an external library without linking it. |
Review the error messages carefully, correct the identified issues in your code, and try compiling again.
Installing a C Compiler
To compile C programs on Linux, you need to have a C compiler installed. The most common compiler is GCC (GNU Compiler Collection). Follow these steps to install it:
- Debian/Ubuntu:
“`bash
sudo apt update
sudo apt install build-essential
“`
- Fedora:
“`bash
sudo dnf groupinstall “Development Tools”
“`
- Arch Linux:
“`bash
sudo pacman -S base-devel
“`
These commands will install GCC along with other essential development tools.
Writing a Simple C Program
Create a simple C program using any text editor. For example, using `nano`:
“`bash
nano hello.c
“`
Insert the following code:
“`c
include
int main() {
printf(“Hello, World!\n”);
return 0;
}
“`
Save and exit the editor (in `nano`, press `CTRL + X`, then `Y` to confirm and `Enter`).
Compiling the C Program
To compile the program, use the `gcc` command followed by the source file name and the `-o` flag to specify the output file name.
“`bash
gcc hello.c -o hello
“`
This command compiles `hello.c` and produces an executable named `hello`.
Running the Compiled Program
After compilation, you can run the executable by prefixing it with `./`:
“`bash
./hello
“`
You should see the output:
“`
Hello, World!
“`
Common GCC Options
GCC offers a variety of options to control the compilation process. Here are some commonly used ones:
Option | Description |
---|---|
`-Wall` | Enables all compiler’s warning messages |
`-g` | Generates debug information |
`-O2` | Enables optimization for speed |
`-std=c11` | Specifies the C standard to use (e.g., C11, C99) |
`-I |
Adds a directory to the list of directories to be searched for header files |
`-L |
Adds a directory to the list of directories to be searched for libraries |
Debugging C Programs
When you compile with the `-g` option, you can use `gdb` (GNU Debugger) for debugging:
- Compile your program with debugging information:
“`bash
gcc -g hello.c -o hello
“`
- Start `gdb` with the executable:
“`bash
gdb ./hello
“`
- Use commands within `gdb`, such as:
- `run`: Executes the program.
- `break
`: Sets a breakpoint at the specified line. - `next`: Executes the next line of code.
- `print
`: Displays the value of a variable.
These steps and tools will enhance your ability to compile and debug C programs effectively on a Linux system.
Expert Insights on Compiling C on Linux
Dr. Emily Carter (Senior Software Engineer, Open Source Initiative). “Compiling C on Linux is a fundamental skill for developers. Utilizing the GCC (GNU Compiler Collection) is essential, as it provides a robust environment for compiling C programs efficiently and effectively.”
Mark Thompson (Linux Systems Administrator, Tech Solutions Corp). “Understanding the command line is crucial when compiling C on Linux. Mastering commands like ‘gcc’ along with proper flags can significantly enhance your programming workflow and debugging process.”
Linda Zhang (Professor of Computer Science, University of Technology). “The process of compiling C on Linux not only involves the use of compilers but also an understanding of linking libraries and managing dependencies, which are critical for successful program execution.”
Frequently Asked Questions (FAQs)
How do I install a C compiler on Linux?
To install a C compiler on Linux, you can use the package manager specific to your distribution. For example, on Ubuntu, you can run `sudo apt install build-essential`, which installs GCC (GNU Compiler Collection) along with other essential tools.
What command is used to compile a C program?
The command to compile a C program using GCC is `gcc filename.c -o outputname`. This command compiles the source file `filename.c` and generates an executable named `outputname`.
How can I compile multiple C source files at once?
To compile multiple C source files, list them all in the `gcc` command, like this: `gcc file1.c file2.c -o outputname`. This command compiles both `file1.c` and `file2.c` into a single executable.
What are common flags used with the GCC compiler?
Common flags include `-Wall` to enable all warnings, `-g` to include debugging information, and `-O2` for optimization. For example, `gcc -Wall -g filename.c -o outputname` enables warnings and debugging.
How do I run a compiled C program in Linux?
To run a compiled C program, use the command `./outputname`, where `outputname` is the name of the executable generated during compilation. Ensure you are in the directory where the executable is located.
What should I do if I encounter compilation errors?
If you encounter compilation errors, carefully read the error messages provided by the compiler. They typically indicate the line number and type of error. Correct the code as needed and recompile the program.
compiling C programs on Linux involves a systematic approach that begins with writing the source code and ends with executing the compiled binary. The primary tool for this process is the GCC (GNU Compiler Collection), which provides a robust and versatile environment for compiling C code. Users can utilize various command-line options to customize the compilation process, such as specifying optimization levels, including libraries, and generating debugging information.
Additionally, understanding the structure of a typical C program and the compilation workflow is crucial. This includes pre-processing, compilation, assembly, and linking stages. Each stage serves a specific purpose and contributes to transforming human-readable C code into machine-executable binaries. Familiarity with these stages helps in troubleshooting compilation errors and optimizing the build process.
Furthermore, leveraging Makefiles can significantly streamline the compilation of larger projects. Makefiles automate the build process, manage dependencies, and provide a clear structure for compiling multiple source files. This not only saves time but also reduces the likelihood of errors during compilation. Overall, mastering the compilation of C on Linux equips developers with essential skills for software development in a Unix-like environment.
Author Profile
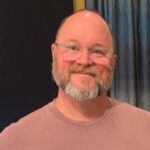
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?