How Can You Comment Out an Entire Section in Python Effectively?
In the world of programming, clarity and organization are paramount. Whether you’re working on a small script or a large-scale application, the ability to manage your code effectively can make a significant difference in both development and debugging. One essential skill every Python programmer should master is how to comment out sections of code. This technique not only helps in documenting your thought process but also allows you to temporarily disable code during testing and iteration. In this article, we will explore the various methods for commenting out whole sections in Python, ensuring your code remains clean and comprehensible.
Commenting out code is a fundamental practice that enhances readability and maintainability. By strategically using comments, developers can provide context for their code, making it easier for themselves and others to understand the logic behind their decisions. In Python, there are several ways to achieve this, each with its own advantages depending on the situation. Understanding these methods will empower you to streamline your coding process and improve collaboration with fellow developers.
As we delve deeper into the topic, we will examine the different techniques available for commenting out blocks of code in Python. From single-line comments to multi-line solutions, you will learn how to effectively manage your codebase, making it easier to navigate and modify as needed. Whether you’re a novice programmer or an
Using Triple Quotes for Multi-line Comments
In Python, one common method to comment out an entire section of code is by utilizing triple quotes. Although primarily used for multi-line strings, triple quotes can effectively serve as comments when not assigned to any variable. This approach allows developers to include lengthy explanations or temporarily disable blocks of code without affecting the overall functionality of the program.
To comment out a section using triple quotes, you can follow this syntax:
“`python
“””
This is a multi-line comment.
You can add as many lines as needed.
“””
“`
Remember that while this method is simple and convenient, it is essential to ensure that the triple-quoted string does not inadvertently become part of any string processing logic.
Using Hash Symbols for Single-line Comments
For shorter comments or single lines, the hash symbol (“) is the standard method in Python. It effectively turns everything after it on the same line into a comment. To comment out multiple lines, you can place a hash symbol at the beginning of each line. Here’s how you can do it:
“`python
This is a comment
This is another comment
And yet another one
“`
While this method is straightforward, it may become cumbersome for larger blocks of text. However, it is beneficial for brief notes or explanations directly related to specific lines of code.
Combining Hash Symbols and Triple Quotes
In scenarios where you want to comment out a large block of code that may include smaller comments, you can combine the two methods. Use triple quotes for the larger sections and hash symbols for inline comments. This approach enhances clarity and maintains readability.
Example:
“`python
“””
This block of code is temporarily disabled
It includes various functionalities
“””
print(“This line will not execute”)
print(“This will also not execute”)
“`
Best Practices for Commenting in Python
When commenting out code, it is crucial to follow best practices to maintain readability and clarity. Here are some guidelines to consider:
- Be Descriptive: Provide enough context in comments for others (or yourself) to understand the purpose of the code.
- Avoid Over-commenting: Excessive comments can clutter code. Aim for clarity in code itself and use comments to clarify complex logic.
- Keep Comments Updated: Ensure comments remain relevant as code changes; outdated comments can lead to confusion.
Commenting Techniques Comparison
Here’s a comparison table summarizing the various commenting techniques in Python:
Technique | Usage | Best For |
---|---|---|
Triple Quotes | Multi-line comments | Long explanations or disabling code blocks |
Hash Symbols | Single-line comments | Short notes and inline comments |
Combination | Both triple quotes and hash symbols | Clarity in complex sections |
Methods for Commenting Out Code in Python
In Python, there are several methods to comment out sections of code. The choice of method often depends on the length of the code being commented and personal or team preferences.
Single-Line Comments
Single-line comments in Python are created using the hash symbol (“). Everything following the “ on that line will be ignored by the Python interpreter. This method is suitable for commenting out short lines or individual statements.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a greeting
“`
Multi-Line Comments Using Triple Quotes
For longer sections of code, Python does not have a built-in multi-line comment syntax like some other programming languages. However, a common practice is to use triple quotes (`”’` or `”””`). While triple quotes are intended for multi-line strings, they can also serve as comments when not assigned to a variable.
Example:
“`python
”’
This is a multi-line comment.
It can span several lines.
”’
print(“Hello, World!”)
“`
This approach has the advantage of being visually clear, as it neatly encapsulates the commented section. However, it is essential to note that using triple quotes this way will create a string object that is not used, which may slightly impact performance in large scripts.
Using the “ Symbol for Block Comments
To comment out larger blocks of code while maintaining readability, you can use the “ symbol at the beginning of each line. This method is straightforward, but it may be cumbersome for extensive sections.
Example:
“`python
print(“This line is commented out.”)
print(“This line is also commented out.”)
print(“This line will not execute.”)
“`
Integrated Development Environment (IDE) Features
Most modern IDEs and code editors offer features to comment out selected lines of code quickly. This functionality enhances productivity by allowing developers to toggle comments without manually typing symbols.
Common shortcuts include:
IDE/Editor | Comment Shortcut | Uncomment Shortcut |
---|---|---|
PyCharm | Ctrl + / | Ctrl + / |
Visual Studio Code | Ctrl + / | Ctrl + / |
Jupyter Notebook | Ctrl + / | Ctrl + / |
Atom | Ctrl + / | Ctrl + / |
Using these shortcuts can save time, especially when working with large codebases or during debugging sessions.
Best Practices for Commenting
When commenting out sections of code, consider the following best practices:
- Clarity: Ensure comments are clear and relevant to the code being commented out.
- Documentation: Use comments to explain why certain code is being disabled, especially if it may be revisited later.
- Clean-Up: Regularly review and remove any comments that are no longer necessary to maintain code readability.
By adhering to these practices, developers can enhance the maintainability and clarity of their code.
Expert Insights on Commenting Out Code Sections in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out a whole section in Python can be efficiently achieved using triple quotes. This method allows developers to temporarily disable blocks of code without affecting the overall structure, making debugging and testing much more manageable.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “While the conventional way to comment out code in Python is to use the hash symbol (), for larger sections, utilizing triple quotes is a cleaner and more effective approach. It not only improves readability but also helps in maintaining code clarity during collaborative projects.”
Linda Zhang (Python Educator and Author, LearnPythonToday). “For beginners, understanding how to comment out code is crucial. Using triple quotes to comment out entire sections can prevent confusion, especially when dealing with multiline comments. It is a best practice that enhances the learning experience for new programmers.”
Frequently Asked Questions (FAQs)
How do I comment out a whole section in Python?
You can comment out a whole section in Python by using triple quotes (`”’` or `”””`). Enclose the code you want to comment out within these quotes, and it will be treated as a string, effectively ignoring it during execution.
Can I use the hash symbol () to comment out multiple lines?
While you can use the hash symbol to comment out multiple lines, it requires placing a “ at the beginning of each line. This method is less efficient for large blocks of code compared to using triple quotes.
Are there any limitations to using triple quotes for comments?
Yes, using triple quotes for comments can lead to unintended consequences if the enclosed text is interpreted as a string. This could affect code readability and may inadvertently alter the behavior of the program if not used carefully.
What is the best practice for commenting code in Python?
The best practice is to use comments judiciously to explain complex logic or provide context. Use inline comments for specific lines and block comments (with triple quotes or multiple “ symbols) for larger sections, ensuring clarity and maintainability.
Can I uncomment a section of code quickly in an IDE?
Most Integrated Development Environments (IDEs) offer shortcuts to uncomment code quickly. Typically, you can select the commented section and use a specific keyboard shortcut (like `Ctrl + /` in many IDEs) to toggle comments on and off.
Is there a difference between comments and docstrings in Python?
Yes, comments are used to explain code and are ignored during execution, while docstrings are a type of comment used to document functions, classes, or modules. Docstrings are accessible at runtime and can be retrieved using the `help()` function or by accessing the `__doc__` attribute.
Commenting out a whole section of code in Python can be achieved through a few different methods, each with its own advantages. The most common approach is to use the hash symbol () at the beginning of each line you wish to comment out. This method is straightforward and works well for short blocks of code. However, for larger sections, manually adding a hash to each line can be cumbersome.
An alternative method involves using triple quotes (”’ or “””) to create a multi-line string. While this is not a traditional comment, it effectively ignores the enclosed code when not assigned to a variable. This technique is particularly useful for temporarily disabling larger blocks of code without altering each line individually. However, it is important to note that this method is not technically a comment and could lead to unintended consequences if the string is inadvertently assigned or executed.
In summary, understanding how to comment out sections of code in Python is essential for effective coding practices. Utilizing the hash symbol for single-line comments and triple quotes for larger blocks provides flexibility in managing code visibility. Developers should choose the method that best suits their needs, keeping in mind the implications of each approach. Proper commenting enhances code readability and maintainability, which are crucial for collaborative projects and long-term code
Author Profile
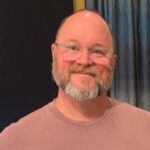
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?