How Can You Use VBA to Group Emails by Field in Outlook?
In the fast-paced world of business communication, managing emails efficiently can make all the difference. For many professionals, Microsoft Outlook stands as a cornerstone tool, enabling seamless correspondence and organization. But what if you could take your email management to the next level? Enter VBA (Visual Basic for Applications), a powerful programming language that allows users to automate tasks and customize Outlook to fit their unique workflows. One of the most effective techniques in VBA is the ability to group emails by specific fields, transforming the way you view and interact with your inbox.
Grouping emails by fields such as sender, date, or subject not only streamlines your workflow but also enhances your productivity. Imagine sifting through hundreds of emails with ease, finding exactly what you need without the frustration of endless scrolling. With VBA, you can create custom scripts that enable this functionality, allowing you to tailor your Outlook experience to your personal or organizational needs. This article will explore the ins and outs of using VBA to group emails effectively, providing you with the tools to harness the full potential of Outlook.
As we delve into the intricacies of VBA and its application in Outlook, you’ll discover how to implement grouping strategies that can save you time and effort. Whether you’re a seasoned programmer or a novice looking to enhance your email management skills
Understanding Grouping in VBA for Outlook
Grouping data in Outlook using VBA can significantly enhance your ability to manage and analyze emails, contacts, calendar items, and tasks. By leveraging the power of grouping, you can organize items based on specific fields such as categories, dates, or sender addresses. This can help streamline workflows and improve productivity.
To implement grouping, you can utilize the `GroupBy` method in VBA. This allows you to sort and categorize items effectively. The general syntax for grouping items in a collection is as follows:
“`vba
Items.GroupBy(Field)
“`
Where `Field` can be any property of the items you wish to group by. Common fields include:
- `ReceivedTime`
- `Subject`
- `SenderName`
- `Categories`
Example of Grouping Emails by Sender
To illustrate how to group emails by sender, consider the following example. This VBA code retrieves all emails from the Inbox and groups them by the sender’s name:
“`vba
Sub GroupEmailsBySender()
Dim olApp As Outlook.Application
Dim olNs As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Dim olItems As Outlook.Items
Dim olMail As Outlook.MailItem
Dim groupedMails As Collection
Dim sender As String
Dim i As Long
Set olApp = New Outlook.Application
Set olNs = olApp.GetNamespace(“MAPI”)
Set olFolder = olNs.GetDefaultFolder(olFolderInbox)
Set olItems = olFolder.Items
Set groupedMails = New Collection
On Error Resume Next
For Each olMail In olItems
If TypeOf olMail Is Outlook.MailItem Then
sender = olMail.SenderName
groupedMails.Add olMail, sender
End If
Next olMail
On Error GoTo 0
‘ Display the grouped emails
For i = 1 To groupedMails.Count
Debug.Print groupedMails(i).SenderName & “: ” & groupedMails(i).Subject
Next i
End Sub
“`
This code will output the grouped emails in the Immediate Window, allowing you to see which emails are from the same sender.
Grouping Calendar Items by Date
Another common grouping scenario is to group calendar items by date. This can be done similarly by using the `Start` property of the AppointmentItem. Here’s how you can achieve this:
“`vba
Sub GroupCalendarByDate()
Dim olApp As Outlook.Application
Dim olNs As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Dim olItems As Outlook.Items
Dim olAppt As Outlook.AppointmentItem
Dim groupedAppointments As Collection
Dim appointmentDate As String
Set olApp = New Outlook.Application
Set olNs = olApp.GetNamespace(“MAPI”)
Set olFolder = olNs.GetDefaultFolder(olFolderCalendar)
Set olItems = olFolder.Items
Set groupedAppointments = New Collection
On Error Resume Next
For Each olAppt In olItems
If TypeOf olAppt Is Outlook.AppointmentItem Then
appointmentDate = Format(olAppt.Start, “dd-mm-yyyy”)
groupedAppointments.Add olAppt, appointmentDate
End If
Next olAppt
On Error GoTo 0
‘ Display the grouped appointments
For Each appointment In groupedAppointments
Debug.Print appointment.Start & “: ” & appointment.Subject
Next appointment
End Sub
“`
This code will group calendar items by their start date, outputting the subject of each appointment.
Benefits of Grouping Items in Outlook
Grouping items in Outlook offers several advantages:
- Improved Organization: By categorizing items, users can quickly locate specific emails, appointments, or tasks.
- Enhanced Analysis: Grouping allows for better data analysis, as users can identify trends and patterns based on grouped criteria.
- Increased Productivity: With organized information, users can manage their time and tasks more efficiently.
Field | Description |
---|---|
ReceivedTime | Groups items based on when they were received. |
SenderName | Groups emails by the sender’s name. |
Categories | Groups items based on assigned categories. |
Utilizing grouping in VBA for Outlook can lead to a more streamlined and efficient approach to managing your daily tasks and communications.
Using VBA to Group Outlook Items by Field
When working with Outlook items using VBA, grouping by specific fields can help organize data effectively. Below are the steps and examples to achieve this.
Setting Up the Outlook Application
To manipulate Outlook items, first, you need to establish a connection with the Outlook application. The following code snippet demonstrates how to do this:
“`vba
Dim olApp As Outlook.Application
Dim olNamespace As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Set olApp = New Outlook.Application
Set olNamespace = olApp.GetNamespace(“MAPI”)
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
“`
Grouping Items by a Specific Field
You can group Outlook items such as emails, tasks, or calendar events by various fields, such as subject, sender, or due date. Here’s an example of grouping emails by the sender:
“`vba
Dim olItem As Object
Dim olItems As Outlook.Items
Dim senderDict As Object
Dim sender As String
Set senderDict = CreateObject(“Scripting.Dictionary”)
Set olItems = olFolder.Items
For Each olItem In olItems
If TypeOf olItem Is Outlook.MailItem Then
sender = olItem.SenderName
If Not senderDict.Exists(sender) Then
senderDict.Add sender, 1
Else
senderDict(sender) = senderDict(sender) + 1
End If
End If
Next olItem
“`
Outputting Grouped Data
To display the grouped data, you can use a simple loop to iterate through the dictionary and output the results. The following code example shows how to print the grouped data to the Immediate Window in the VBA editor:
“`vba
Dim key As Variant
For Each key In senderDict.Keys
Debug.Print key & “: ” & senderDict(key) & ” emails”
Next key
“`
Grouping by Multiple Fields
For more complex grouping, such as grouping emails by both sender and date, you can create a nested dictionary structure. Here’s an example:
“`vba
Dim dateDict As Object
Dim emailDate As String
Set dateDict = CreateObject(“Scripting.Dictionary”)
For Each olItem In olItems
If TypeOf olItem Is Outlook.MailItem Then
sender = olItem.SenderName
emailDate = Format(olItem.SentOn, “yyyy-mm-dd”)
If Not dateDict.Exists(sender) Then
dateDict.Add sender, CreateObject(“Scripting.Dictionary”)
End If
If Not dateDict(sender).Exists(emailDate) Then
dateDict(sender).Add emailDate, 1
Else
dateDict(sender)(emailDate) = dateDict(sender)(emailDate) + 1
End If
End If
Next olItem
“`
Displaying Multi-Level Grouped Data
To display the grouped data by sender and date, use the following code snippet:
“`vba
Dim senderKey As Variant, dateKey As Variant
For Each senderKey In dateDict.Keys
Debug.Print senderKey & “:”
For Each dateKey In dateDict(senderKey).Keys
Debug.Print ” ” & dateKey & “: ” & dateDict(senderKey)(dateKey) & ” emails”
Next dateKey
Next senderKey
“`
Handling Errors
Implement error handling to ensure that your VBA code executes smoothly. Use the following structure:
“`vba
On Error Resume Next
‘ Your code here
If Err.Number <> 0 Then
Debug.Print “Error: ” & Err.Description
End If
On Error GoTo 0
“`
This approach helps catch issues without stopping the entire script execution.
Expert Insights on VBA Outlook Grouping Techniques
Dr. Emily Carter (Senior Software Developer, Tech Innovations Inc.). “Utilizing VBA to group Outlook items by field can significantly enhance data organization. By leveraging the built-in properties of Outlook objects, developers can create custom views that allow users to quickly access relevant information, improving overall productivity.”
Michael Chen (IT Consultant, Business Solutions Group). “When grouping Outlook items with VBA, it is crucial to understand the underlying data structure. Properly identifying the fields to group by can lead to more meaningful insights and facilitate better decision-making processes within teams.”
Sarah Thompson (Microsoft Office Specialist, Productivity Experts). “Implementing grouping in Outlook through VBA not only streamlines email management but also enhances collaboration. By automating the grouping process, users can focus on high-priority tasks without getting bogged down by clutter.”
Frequently Asked Questions (FAQs)
What does “Group By Field” mean in VBA for Outlook?
“Group By Field” in VBA for Outlook refers to the process of organizing items in a collection based on a specific property or field, such as date, sender, or subject. This allows for better data management and analysis.
How can I implement “Group By Field” in a VBA script for Outlook?
To implement “Group By Field,” you can use the `Items` collection of a folder and apply a sorting mechanism followed by a loop that checks the value of the specified field. You can then categorize items accordingly.
Can I group emails by multiple fields in VBA for Outlook?
Yes, you can group emails by multiple fields by first sorting the collection by the primary field and then iterating through the items to group them by the secondary field within the primary group.
What are some common fields to group by in Outlook VBA?
Common fields to group by include `ReceivedTime`, `SenderName`, `Subject`, and `Categories`. These fields provide meaningful ways to categorize and analyze email data.
Are there any performance considerations when grouping items in Outlook VBA?
Yes, performance can be impacted by the size of the collection being processed. It is advisable to limit the number of items being grouped or to filter the collection before applying the grouping logic to enhance efficiency.
Is it possible to export grouped data from Outlook using VBA?
Yes, you can export grouped data by iterating through the grouped items and writing the results to an external file, such as Excel or a CSV file. This allows for further analysis and reporting outside of Outlook.
In summary, utilizing VBA in Outlook to group items by specific fields can significantly enhance the organization and management of emails, calendar events, and other items. By leveraging the capabilities of VBA, users can automate the grouping process, allowing for more efficient data handling and improved visibility of related items. This functionality is particularly beneficial for users who deal with large volumes of information and require a streamlined approach to access and analyze their data.
Key takeaways from the discussion include the importance of understanding the Outlook object model, as it serves as the foundation for effective VBA scripting. Familiarity with the various properties and methods associated with items in Outlook is crucial for successfully implementing grouping features. Additionally, users should be aware of the different types of fields available for grouping, such as categories, dates, and custom properties, to tailor their scripts to meet specific needs.
Moreover, it is essential to consider performance implications when grouping large datasets. Efficient coding practices, such as minimizing the number of iterations and utilizing built-in Outlook functions, can lead to faster execution times and a better user experience. Overall, mastering VBA for Outlook grouping can empower users to create customized solutions that enhance productivity and facilitate better information management.
Author Profile
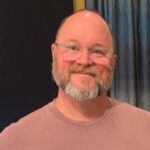
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?