How Can You Effectively Write a Python Script?
How To Write In Python Script: A Beginner’s Guide to Coding with Clarity
In the ever-evolving landscape of technology, programming has become an essential skill that empowers individuals to create, innovate, and solve complex problems. Among the myriad of programming languages available, Python stands out for its simplicity and versatility, making it an ideal choice for both beginners and seasoned developers alike. Whether you’re looking to automate mundane tasks, develop web applications, or dive into data science, learning how to write in Python script is your gateway to unlocking a world of possibilities.
Writing in Python is not just about mastering syntax; it’s about embracing a mindset that prioritizes clarity and efficiency. With its readable code and straightforward structure, Python allows you to express your ideas in a way that is both intuitive and powerful. This article will guide you through the fundamental concepts of writing Python scripts, covering everything from setting up your environment to understanding the core principles of coding. As you embark on this journey, you’ll discover how to harness the power of Python to bring your ideas to life, paving the way for endless opportunities in the digital realm.
Prepare to dive into the essentials of Python scripting, where you’ll learn to craft code that is not only functional but also elegant. Whether you’re a complete novice or looking to
Writing Your First Python Script
To start writing a Python script, you need a text editor or an Integrated Development Environment (IDE). Popular choices include Visual Studio Code, PyCharm, and even simple editors like Notepad or Sublime Text. The choice of editor may depend on your comfort level and the complexity of the projects you intend to undertake.
Begin by creating a new file with a `.py` extension. This signals to the Python interpreter that the file contains Python code. For instance, you might name your file `hello_world.py`. In this file, you can write your first line of code:
“`python
print(“Hello, World!”)
“`
When you execute this script, it will output “Hello, World!” to the console, demonstrating the basic structure of a Python script.
Understanding Python Syntax
Python has a clear and readable syntax, which contributes to its popularity among both beginners and seasoned developers. Key elements of Python syntax include:
- Indentation: Instead of braces or keywords, Python uses indentation to define blocks of code. This is critical in control structures such as loops and functions.
- Comments: Use the “ symbol to add comments, which the interpreter ignores. Comments are essential for documenting your code.
Example of indentation and comments:
“`python
This is a comment
def greet(name):
print(“Hello, ” + name) Greeting the user
“`
Using Variables and Data Types
Variables in Python are created by simply assigning a value to a name. Python supports various data types, including integers, floats, strings, and booleans. Here are some common data types and their usage:
Data Type | Description | Example |
---|---|---|
Integer | Whole numbers | x = 10 |
Float | Decimal numbers | y = 3.14 |
String | Text data | name = “Alice” |
Boolean | True or values | is_active = True |
Variable names should be descriptive and can include letters, numbers, and underscores, but cannot start with a number.
Control Structures: If Statements and Loops
Control structures allow you to dictate the flow of your Python script. The most common include `if` statements and loops.
**If Statements**: These help execute code based on conditions. An example is shown below:
“`python
age = 18
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
Loops: Loops enable you to repeat code. The `for` loop is used to iterate over a sequence, while the `while` loop continues until a condition is met.
Example of a `for` loop:
“`python
for i in range(5):
print(i)
“`
Example of a `while` loop:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
These structures form the backbone of controlling the execution flow in Python scripts, allowing for dynamic and responsive applications.
Understanding Python Syntax
Python syntax is designed to be clean and easy to read, which is essential for writing efficient scripts. Here are some fundamental aspects of Python syntax:
- Indentation: Python uses indentation to define blocks of code. Unlike other languages that use braces, the indentation level indicates the grouping of statements.
- Comments: Use the “ symbol for single-line comments. Multi-line comments can be created using triple quotes (`”’` or `”””`).
- Variables: Assign values to variables without declaring their type, as Python is dynamically typed. For example:
“`python
x = 10
name = “Alice”
“`
Creating a Python Script
To create a Python script, follow these steps:
- Choose an Editor: Use any text editor or an Integrated Development Environment (IDE) like PyCharm, Visual Studio Code, or Jupyter Notebook.
- Save Your File: Use the `.py` extension for your script. For example, `my_script.py`.
- Write Code: Begin writing your Python code, utilizing functions, loops, and conditionals as needed.
Basic Python Constructs
Understanding basic constructs is vital for effective scripting. Below are common constructs in Python:
– **Variables and Data Types**:
- Integers: `x = 5`
- Floats: `pi = 3.14`
- Strings: `greeting = “Hello, World!”`
- Lists: `numbers = [1, 2, 3]`
- Dictionaries: `person = {“name”: “Alice”, “age”: 30}`
– **Control Structures**:
– **If Statements**:
“`python
if x > 10:
print(“x is greater than 10”)
“`
- For Loops:
“`python
for number in numbers:
print(number)
“`
- While Loops:
“`python
while x < 10:
x += 1
```
Defining Functions
Functions are reusable blocks of code that perform specific tasks. Define a function using the `def` keyword:
“`python
def greet(name):
return f”Hello, {name}!”
“`
You can call the function with:
“`python
print(greet(“Alice”))
“`
Modules and Libraries
Python supports modules and libraries, which allow you to extend functionality. Import modules using the `import` statement:
- Standard Library:
- `import math`
- `import random`
- Third-Party Libraries:
- Install with `pip install package_name`
- Import in your script using the same `import` statement.
Error Handling
Error handling is crucial in scripting. Use `try` and `except` blocks to manage exceptions:
“`python
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero.”)
“`
Running a Python Script
To execute your Python script, use the command line:
- Open your command line interface.
- Navigate to the directory containing your script.
- Run the script with the command:
“`
python my_script.py
“`
This will execute the script, displaying any output in the command line window.
Best Practices for Writing Python Scripts
Follow these best practices to enhance code quality:
- Use clear and descriptive variable names.
- Keep functions focused on a single task.
- Write modular code; separate concerns into different functions or modules.
- Document your code with comments and docstrings.
- Follow PEP 8 guidelines for style consistency.
By adhering to these principles, you can write effective, maintainable Python scripts.
Expert Insights on Writing Python Scripts
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When writing Python scripts, clarity and simplicity should be your guiding principles. Utilizing meaningful variable names and modular functions not only enhances readability but also facilitates easier debugging and maintenance.”
Michael Johnson (Lead Data Scientist, Data Insights Group). “Incorporating libraries such as NumPy and Pandas can significantly streamline your Python scripts, especially when handling data manipulation tasks. Understanding the strengths of these libraries will allow you to write more efficient and powerful scripts.”
Sarah Patel (Python Instructor, Code Academy). “Practice is essential when learning how to write in Python. Start with small projects and gradually increase complexity. Engaging with the community through forums or coding challenges can also provide valuable feedback and enhance your skills.”
Frequently Asked Questions (FAQs)
How do I start writing a Python script?
To start writing a Python script, open a text editor or an Integrated Development Environment (IDE) such as PyCharm or VSCode. Create a new file with a `.py` extension, and begin writing your Python code.
What are the basic components of a Python script?
A basic Python script typically includes variables, data types, control structures (like loops and conditionals), functions, and modules. These components work together to perform tasks and manage data.
How can I run a Python script?
You can run a Python script by opening a command line interface, navigating to the directory containing the script, and executing the command `python script_name.py`, replacing `script_name.py` with your actual file name.
What is the purpose of comments in a Python script?
Comments in a Python script are used to explain code, making it easier to understand for others and for your future self. They are ignored by the Python interpreter and are created using the “ symbol for single-line comments or triple quotes for multi-line comments.
How do I handle errors in a Python script?
Error handling in Python can be accomplished using `try` and `except` blocks. This allows you to catch exceptions and handle them gracefully, preventing the script from crashing and providing informative feedback.
What libraries should I consider using in my Python scripts?
Common libraries to consider include NumPy for numerical operations, pandas for data manipulation, requests for handling HTTP requests, and matplotlib for data visualization. The choice of libraries depends on the specific requirements of your project.
Writing in Python script involves understanding the syntax and structure of the language, which is known for its readability and simplicity. To effectively write a Python script, one must first familiarize themselves with the basic components such as variables, data types, control structures, functions, and modules. Each of these elements plays a crucial role in crafting efficient and functional scripts that can automate tasks, process data, or perform complex calculations.
Additionally, leveraging Python’s extensive libraries and frameworks can significantly enhance the capabilities of your scripts. Libraries like NumPy for numerical computations, Pandas for data manipulation, and Flask for web development provide powerful tools that can save time and effort. Understanding how to import and utilize these libraries is essential for writing advanced Python scripts that meet specific needs.
Moreover, following best practices such as writing clear comments, adhering to PEP 8 style guidelines, and structuring code logically will improve the maintainability and readability of your scripts. Utilizing version control systems like Git can also facilitate collaboration and tracking changes in your code over time. By integrating these practices, you can ensure that your Python scripts are not only functional but also robust and easy to understand for yourself and others.
Author Profile
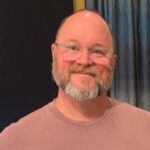
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?