Are Integers Mutable in Python? Unpacking the Truth Behind Python’s Data Types
### Are Integers Mutable In Python?
In the realm of programming, understanding data types and their behaviors is crucial for writing efficient and error-free code. Among the many data types that Python offers, integers hold a special place due to their fundamental role in calculations and data manipulation. However, a common question arises for both novice and experienced programmers alike: Are integers mutable in Python? This seemingly simple query opens the door to a deeper exploration of how Python handles data types, memory management, and the implications of mutability in programming.
To grasp the concept of mutability, one must first understand what it means in the context of programming languages. In Python, data types are categorized as either mutable or immutable, which dictates whether their values can be changed after creation. While mutable types, such as lists and dictionaries, allow modifications to their content, immutable types, like strings and tuples, do not permit alterations. Integers, being a core data type, fall into this classification, but their behavior can often lead to confusion among developers.
As we delve into the intricacies of Python’s handling of integers, we will uncover the implications of their immutability, how it affects performance, and the nuances of memory allocation. This exploration will not only clarify the nature of integers but also enhance your
Understanding Mutability in Python
In Python, mutability refers to the ability of an object to be modified after it has been created. Objects that can be changed are known as mutable objects, while those that cannot be changed are immutable. This distinction is crucial for understanding how different types of objects behave in Python, particularly when it comes to integers.
Characteristics of Integers
Integers in Python are classified as immutable objects. This means that once an integer is created, its value cannot be altered. Any operation that appears to modify an integer actually results in the creation of a new integer object. The original integer remains unchanged, which can lead to some important implications in programming.
- Immutable Behavior:
- When you perform operations like addition, subtraction, or multiplication on integers, Python generates a new integer rather than modifying the existing one.
- For example, executing `x = 5` followed by `x += 2` results in the creation of a new integer object with the value `7`, while the original integer `5` remains unchanged in memory.
Implications of Integer Mutability
The immutability of integers can affect how they are used in various programming scenarios, particularly with regard to performance and memory management. Here are some implications to consider:
- Memory Efficiency:
- Python caches small integers (typically from -5 to 256) for performance optimization. This means that multiple variables can point to the same integer object, reducing memory overhead.
- Identity and Equality:
- Since integers are immutable, two variables can reference the same integer object without any risk of one variable changing its value and affecting the other.
Operation | Resulting Object | Explanation |
---|---|---|
`a = 3` | Integer 3 | `a` references a new integer object. |
`b = a` | Integer 3 | `b` references the same integer object as `a`. |
`a += 1` | Integer 4 | A new integer object is created; `a` now references this new object. |
`b` | Integer 3 | `b` still references the original integer object. |
Integer Mutability
Understanding that integers are immutable is essential for efficient programming in Python. This characteristic influences how variables are assigned and manipulated, and it plays a significant role in memory management and performance optimization.
Understanding Mutability in Python
In Python, mutability refers to the ability of an object to change its state or value after it has been created. Objects in Python can be classified into two categories based on this characteristic: mutable and immutable.
Mutable Objects
Mutable objects can be changed in place, meaning their contents can be altered without creating a new object. Examples include:
- Lists
- Dictionaries
- Sets
Immutable Objects
Immutable objects, on the other hand, cannot be altered once they are created. Any operation that seems to modify an immutable object will actually create a new object. Examples include:
- Strings
- Tuples
- Integers
Are Integers Mutable?
Integers in Python are immutable. This means that once an integer object is created, its value cannot be modified. If you perform an operation that alters its value, Python creates a new integer object instead of modifying the existing one.
Key Characteristics of Integer Mutability
- Creation of New Objects: When an integer is incremented or decremented, a new integer object is created.
- Memory Management: Python uses an internal optimization technique called interning for small integers (typically in the range of -5 to 256). This means that these integers share memory locations to save space.
Example of Integer Immutability
python
a = 5
b = a
a += 1 # a is now 6; a new integer object is created
print(a) # Output: 6
print(b) # Output: 5
In this example, the operation `a += 1` does not change the value of `b`, demonstrating that `a` and `b` are distinct objects after the operation.
Comparison with Mutable Types
To illustrate the difference between mutable and immutable types in Python, consider the following examples:
Type | Mutability | Example Code | Result |
---|---|---|---|
Integer | Immutable | `x = 3; x += 1` | `x` points to a new object (4) |
List | Mutable | `my_list = [1, 2]; my_list.append(3)` | `my_list` is now [1, 2, 3] |
In the list example, the original list is modified in place, demonstrating mutability. In contrast, with integers, a new object is created.
Implications of Integer Immutability
The immutability of integers has several implications for programming in Python:
- Thread Safety: Immutable objects are inherently thread-safe, as their state cannot change. This reduces the risk of bugs in concurrent programming.
- Hashability: Immutable types, including integers, can be used as keys in dictionaries and elements in sets, as their hash value remains constant.
- Performance Optimization: Python can optimize memory usage and performance through techniques like caching and interning for immutable types.
Understanding the mutability of integers is crucial for effective programming in Python, as it influences how variables and data structures are manipulated throughout your code.
Understanding the Mutability of Integers in Python
Dr. Emily Carter (Computer Science Professor, Tech University). “In Python, integers are immutable, meaning that once an integer object is created, its value cannot be changed. Any operation that seems to modify an integer actually creates a new integer object instead.”
Michael Chen (Senior Software Engineer, Code Innovations). “The immutability of integers in Python is a fundamental aspect of the language’s design. This characteristic ensures that integers can be safely used as keys in dictionaries and elements in sets without the risk of unexpected changes.”
Lisa Patel (Python Developer Advocate, Open Source Foundation). “Understanding that integers are immutable is crucial for Python developers. It affects how we manage memory and optimize performance, particularly in applications that handle large datasets or require high computational efficiency.”
Frequently Asked Questions (FAQs)
Are integers mutable in Python?
No, integers are immutable in Python. Once an integer object is created, its value cannot be changed. Any operation that seems to modify an integer actually creates a new integer object.
What does it mean for an object to be mutable or immutable?
Mutable objects can be changed after they are created, allowing for modifications without creating a new object. Immutable objects cannot be altered once created, requiring the creation of a new object for any change.
Can you give examples of mutable and immutable types in Python?
Examples of mutable types include lists, dictionaries, and sets. Immutable types include integers, floats, strings, and tuples.
How does immutability affect performance in Python?
Immutability can enhance performance in certain scenarios, such as when using integers as dictionary keys or in sets, as their hash values remain constant. This can lead to faster lookups and reduced memory overhead.
What happens if I try to change an integer value in Python?
Attempting to change an integer value will result in the creation of a new integer object. The original integer remains unchanged, and the variable will reference the new object instead.
Are there any implications of using immutable objects like integers in programming?
Using immutable objects can lead to safer code, as they prevent accidental changes. This can help avoid bugs related to unintended modifications, especially in concurrent programming scenarios.
In Python, integers are immutable objects, which means that once an integer is created, its value cannot be changed. This immutability is a fundamental characteristic of integers in Python, distinguishing them from mutable data types like lists or dictionaries. When an operation that appears to modify an integer is performed, Python actually creates a new integer object rather than altering the original object.
The implications of integer immutability are significant for memory management and performance. Since integers are immutable, Python can optimize memory usage by reusing existing integer objects for small values. For instance, integers between -5 and 256 are preallocated and shared across the program, which enhances efficiency. This behavior is particularly beneficial in scenarios involving frequent integer operations, as it reduces the overhead of creating new objects.
Understanding the immutability of integers is crucial for Python developers, as it influences how variables and memory are handled. When performing operations that involve integers, developers must be aware that any modification will result in a new object being created. This knowledge helps prevent unintended side effects and enhances code reliability, particularly in complex applications where data integrity is paramount.
Author Profile
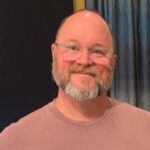
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?