How Can You Effectively Loop Through a List in Python?
In the world of programming, efficiency and elegance are key, especially when it comes to handling data. One of the most fundamental data structures in Python is the list, a versatile tool that allows you to store and manipulate collections of items. Whether you’re working with a simple list of numbers or a complex array of objects, knowing how to loop through a list effectively can significantly enhance your coding prowess. This article will guide you through the various techniques and best practices for iterating over lists in Python, empowering you to write cleaner and more efficient code.
Looping through a list is a common task that every Python programmer encounters, and it can be accomplished in several ways. From the classic `for` loop to more advanced methods such as list comprehensions and the `enumerate` function, each approach has its own strengths and use cases. Understanding these methods not only helps you retrieve data but also allows you to manipulate and transform it in powerful ways.
As we delve into the nuances of list iteration, you’ll discover how to choose the right looping technique for your specific needs, optimize performance, and even handle complex data structures. Whether you’re a beginner looking to grasp the basics or an experienced coder seeking to refine your skills, this exploration of looping through lists in Python is sure to
Using For Loops
A common and straightforward method to loop through a list in Python is by utilizing a `for` loop. This construct allows you to iterate over each element in the list, executing a block of code for every item. The syntax is simple and elegant, making it the preferred choice for many programmers.
Example:
“`python
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
“`
In this snippet, each fruit in the `fruits` list is printed on a new line. The variable `fruit` takes on the value of each element in the list sequentially.
Using While Loops
Another approach to loop through a list is by employing a `while` loop. This method is useful when you need more control over the iteration process, such as modifying the index directly or handling more complex conditions.
Example:
“`python
fruits = [“apple”, “banana”, “cherry”]
index = 0
while index < len(fruits):
print(fruits[index])
index += 1
```
This example utilizes an index variable to track the current position in the list. The loop continues until the index exceeds the length of the list.
List Comprehensions
List comprehensions provide a concise way to create lists by iterating over existing lists. This method is particularly useful for generating new lists by applying an expression to each element.
Example:
“`python
squared_numbers = [x**2 for x in range(10)]
“`
In this case, `squared_numbers` contains the squares of numbers from 0 to 9. List comprehensions can also include conditions:
“`python
even_squares = [x**2 for x in range(10) if x % 2 == 0]
“`
Here, `even_squares` will only include the squares of even numbers.
Iterating with Enumerate
When you need both the index and the value from a list during iteration, the `enumerate()` function is invaluable. It allows you to loop over the list while keeping track of the index automatically.
Example:
“`python
fruits = [“apple”, “banana”, “cherry”]
for index, fruit in enumerate(fruits):
print(f”{index}: {fruit}”)
“`
This will output the index alongside each fruit, making it easier to reference their positions.
Iterating with the Map Function
For functional programming enthusiasts, the `map()` function provides a way to apply a function to all items in a list. This can be an elegant alternative to traditional loops when transforming data.
Example:
“`python
def to_uppercase(fruit):
return fruit.upper()
fruits = [“apple”, “banana”, “cherry”]
uppercase_fruits = list(map(to_uppercase, fruits))
“`
The `uppercase_fruits` list will contain all fruit names in uppercase.
Method | Description | Example |
---|---|---|
For Loop | Standard iteration over list elements. | for fruit in fruits: print(fruit) |
While Loop | Iteration with manual index control. | while index < len(fruits): print(fruits[index]); index += 1 |
List Comprehension | Creating lists based on existing lists. | squared_numbers = [x**2 for x in range(10)] |
Enumerate | Iterate with index and value. | for index, fruit in enumerate(fruits): print(f”{index}: {fruit}”) |
Map Function | Apply a function to list items. | uppercase_fruits = list(map(to_uppercase, fruits)) |
Each method offers unique benefits, and the choice of which to use often depends on the specific use case and the readability of the code.
Different Methods to Loop Through a List in Python
In Python, there are several effective methods to iterate over a list. Each method has its own use case depending on the specific requirements of the task at hand.
Using a Basic For Loop
The most straightforward way to loop through a list is using a `for` loop. This method allows you to access each element in the list sequentially.
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
“`
This code snippet will output:
“`
1
2
3
4
5
“`
Using the Range Function
Another common approach is to use the `range()` function, which provides the index of each element during the iteration.
“`python
my_list = [‘a’, ‘b’, ‘c’, ‘d’]
for i in range(len(my_list)):
print(my_list[i])
“`
This code will print:
“`
a
b
c
d
“`
List Comprehensions
List comprehensions offer a concise way to create lists while iterating over another list. They are particularly useful for transforming data.
“`python
squared_numbers = [x**2 for x in range(10)]
print(squared_numbers)
“`
The output will be:
“`
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
“`
Enumerate Function
Using the `enumerate()` function allows for iteration over a list while keeping track of the index, which can be particularly useful for tracking positions.
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
for index, value in enumerate(my_list):
print(index, value)
“`
This will yield:
“`
0 apple
1 banana
2 cherry
“`
Using While Loop
A `while` loop can also be employed to iterate through a list, although it is less common than the `for` loop.
“`python
my_list = [10, 20, 30, 40]
index = 0
while index < len(my_list):
print(my_list[index])
index += 1
```
Output:
```
10
20
30
40
```
Looping Through Multiple Lists
To loop through multiple lists simultaneously, you can use the `zip()` function, which aggregates elements from each list into tuples.
“`python
list1 = [1, 2, 3]
list2 = [‘one’, ‘two’, ‘three’]
for number, word in zip(list1, list2):
print(number, word)
“`
This will produce:
“`
1 one
2 two
3 three
“`
Using List Iterators
Python provides an iterator for lists, which can be accessed using the `iter()` function.
“`python
my_list = [5, 10, 15]
iterator = iter(my_list)
for item in iterator:
print(item)
“`
The output will be:
“`
5
10
15
“`
Summary of Looping Techniques
Method | Description |
---|---|
Basic For Loop | Iterates directly over elements. |
Range Function | Iterates using indices. |
List Comprehensions | Creates new lists based on existing ones. |
Enumerate | Provides both index and value. |
While Loop | Uses a conditional to iterate. |
Zip | Loops through multiple lists concurrently. |
Iterators | Uses the iterator protocol for lists. |
Understanding these various methods empowers you to choose the most effective looping strategy for your specific scenario in Python programming.
Expert Insights on Looping Through Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Looping through lists in Python is fundamental for data manipulation. Utilizing constructs like for-loops and list comprehensions not only enhances code readability but also optimizes performance in data-heavy applications.”
Michael Chen (Python Developer Advocate, CodeCraft Solutions). “Understanding the nuances of looping through lists is crucial for any Python developer. I recommend mastering both traditional loops and Pythonic approaches, such as the enumerate function, to efficiently handle list indexing and values simultaneously.”
Sarah Johnson (Data Scientist, Analytics Group). “When working with large datasets, the method of looping through lists can significantly impact processing time. Leveraging libraries like NumPy for vectorized operations can often replace explicit loops, resulting in more efficient data processing.”
Frequently Asked Questions (FAQs)
How do I loop through a list in Python?
You can loop through a list in Python using a `for` loop. For example:
“`python
my_list = [1, 2, 3]
for item in my_list:
print(item)
“`
What is the difference between a for loop and a while loop for iterating through a list?
A `for` loop iterates over each element in a list directly, while a `while` loop requires manual index management. The `for` loop is generally simpler and less error-prone for this purpose.
Can I use list comprehensions to loop through a list?
Yes, list comprehensions provide a concise way to create lists by looping through an existing list. For example:
“`python
squared = [x**2 for x in my_list]
“`
How can I access both the index and the value when looping through a list?
You can use the `enumerate()` function to access both the index and the value. For example:
“`python
for index, value in enumerate(my_list):
print(index, value)
“`
Is it possible to loop through a list in reverse order?
Yes, you can loop through a list in reverse order using the `reversed()` function or by slicing the list. For example:
“`python
for item in reversed(my_list):
print(item)
“`
What are some common mistakes to avoid when looping through a list?
Common mistakes include modifying the list while iterating, using incorrect indices, and forgetting to initialize variables. These can lead to unexpected behavior or errors.
In Python, looping through a list is a fundamental operation that allows developers to access and manipulate each element within the list efficiently. Various methods can be employed to achieve this, including the traditional for loop, while loops, and more advanced techniques such as list comprehensions and the use of the built-in map function. Each method offers unique advantages, making it essential for programmers to choose the one that best fits their specific use case.
One of the most common approaches is the for loop, which iterates over each item in the list, providing a straightforward way to access elements. List comprehensions, on the other hand, offer a concise syntax for creating new lists by applying an expression to each element, thus enhancing code readability and efficiency. Additionally, using the enumerate function can be particularly useful when both the index and the value of the elements are needed during iteration.
In summary, understanding how to loop through lists in Python is crucial for effective programming. By leveraging the various looping techniques available, developers can write cleaner, more efficient code. Mastering these methods not only improves productivity but also enhances the overall quality of the code, making it easier to maintain and debug in the long run.
Author Profile
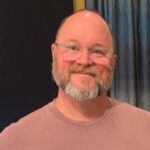
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?