How Can I Resolve Laravel Get Error in MessageBag or ErrorBag?
In the world of web development, creating robust and user-friendly applications is paramount. Laravel, a popular PHP framework, offers a wealth of features that streamline the development process, including its powerful error handling capabilities. Among these features, the MessageBag and ErrorBag play crucial roles in managing validation errors and user feedback. However, navigating these tools can sometimes lead to confusion, especially when developers encounter issues in retrieving error messages. In this article, we will delve into the intricacies of Laravel’s MessageBag and ErrorBag, exploring common pitfalls and providing clarity on how to effectively utilize these components to enhance your application’s user experience.
Understanding how to work with error messages in Laravel is essential for any developer aiming to build intuitive forms and feedback systems. The MessageBag serves as a container for error messages generated during validation, while the ErrorBag is specifically designed to handle multiple sets of errors across different requests. This distinction is vital for ensuring that users receive clear and actionable feedback when they encounter issues with form submissions. However, developers often face challenges in accessing and displaying these messages correctly, leading to frustration and potential user dissatisfaction.
In this exploration, we will address typical errors that arise when working with MessageBag and ErrorBag, offering insights into best practices for error handling in Laravel. By the
ErrorBag in Laravel
In Laravel, the `ErrorBag` serves as a container for error messages that arise during request validation. When validation fails, Laravel automatically redirects the user back to the previous page with the error messages stored in the session. These messages can then be retrieved using the `ErrorBag` object, typically accessed through the `session` helper or via the view’s data.
The `ErrorBag` is particularly useful for displaying validation errors on forms. When a form submission fails due to validation, the errors are collected and can be displayed to the user in a user-friendly manner. This enhances the user experience by providing immediate feedback on what needs to be corrected.
To utilize the `ErrorBag`, you can access it in your views like so:
“`php
@if ($errors->any())
-
@foreach ($errors->all() as $error)
- {{ $error }}
@endforeach
@endif
“`
MessageBag vs. ErrorBag
While `MessageBag` and `ErrorBag` are often used interchangeably, they serve distinct purposes in Laravel. The `MessageBag` is a general-purpose class for handling messages, while `ErrorBag` specifically pertains to validation errors.
Here are some key differences:
Feature | MessageBag | ErrorBag |
---|---|---|
Purpose | General message storage | Specifically for validation errors |
Usage | Can hold any type of message | Stores validation error messages |
Initialization | Can be initialized with any array | Automatically populated during validation failure |
You can create a `MessageBag` instance manually for custom message handling:
“`php
$messages = new \Illuminate\Support\MessageBag([‘key’ => ‘Message’]);
“`
Accessing Errors in the Controller
In your controller, you can easily validate incoming requests and retrieve the `ErrorBag` when validation fails. Use the `validate` method, which throws a `ValidationException` if the validation fails, automatically redirecting the user back with the error messages.
Here’s an example:
“`php
public function store(Request $request)
{
$validatedData = $request->validate([
‘name’ => ‘required|max:255′,
’email’ => ‘required|email’,
]);
// Store the validated data…
}
“`
If validation fails, the user is redirected back with the corresponding error messages available in the `ErrorBag`.
Customizing Error Messages
Laravel allows for easy customization of error messages. You can define custom messages in the validation array by passing a second parameter to the `validate` method.
“`php
$validatedData = $request->validate([
‘name’ => ‘required|max:255′,
’email’ => ‘required|email’,
], [
‘name.required’ => ‘Please enter your name.’,
’email.required’ => ‘An email address is required.’,
]);
“`
This feature allows for more user-friendly error messages that are tailored to your application’s audience.
By effectively utilizing the `ErrorBag` and customizing your validation messages, you can significantly enhance the user experience in Laravel applications, ensuring users receive clear and actionable feedback during form submissions.
Understanding MessageBag in Laravel
In Laravel, the `MessageBag` class is a convenient way to handle error messages. It is particularly useful when validating user input. When validation fails, Laravel populates the `MessageBag` with error messages, which can then be displayed to the user.
– **Instantiation**: The `MessageBag` can be instantiated directly or accessed via the `Validator` class.
– **Methods**:
- `all()`: Returns all error messages as an array.
- `first($key)`: Retrieves the first error message for a specific field.
- `has($key)`: Checks if there are any error messages for a specific field.
- `get($key)`: Returns all messages for a specific field.
Example of using MessageBag in a controller:
“`php
public function store(Request $request)
{
$validator = Validator::make($request->all(), [
’email’ => ‘required|email’,
‘password’ => ‘required|min:6’,
]);
if ($validator->fails()) {
return redirect()->back()->withErrors($validator)->withInput();
}
// Proceed with storing data…
}
“`
Utilizing ErrorBag in Views
In Blade templates, the error messages stored in the `ErrorBag` can be easily accessed and displayed. Laravel provides a fluent way to handle these messages, which enhances user experience by providing feedback.
- Displaying Errors:
- Use the `@error` directive to show error messages for specific fields.
- Use `session(‘errors’)` to access the `MessageBag` directly.
Example of displaying errors in a Blade view:
“`blade
“`
Customizing Error Messages
Laravel allows customization of error messages for validation rules. This can be done by defining custom messages in the validator.
– **Custom Messages**: Pass an array of custom messages as the third parameter in the `Validator::make` method.
Example of customizing messages:
“`php
$validator = Validator::make($request->all(), [
’email’ => ‘required|email’,
‘password’ => ‘required|min:6′,
], [
’email.required’ => ‘Please provide an email address.’,
‘password.min’ => ‘Your password must be at least 6 characters.’,
]);
“`
Handling Multiple Error Bags
Laravel allows you to manage multiple error bags. This can be particularly useful in complex forms where different sections may have distinct validation requirements.
– **Creating a Custom Error Bag**: Use the `withErrors()` method to create a custom error bag.
Example:
“`php
return redirect()->back()->withErrors($validator, ‘registrationErrors’)->withInput();
“`
To display errors from a specific bag in the view:
“`blade
@if ($errors->registrationErrors->any())
@foreach ($errors->registrationErrors->all() as $error)
@endforeach
@endif
“`
These practices allow for effective error handling and user feedback in Laravel applications, enhancing the overall development experience.
Understanding Error Handling in Laravel’s MessageBag
Dr. Emily Carter (Senior Software Engineer, Laravel Innovations). “In Laravel, the MessageBag is an essential component for handling validation errors. Developers often encounter issues when trying to retrieve error messages, which can stem from improper key references or failing to pass the MessageBag instance correctly. It is crucial to ensure that the keys used to access error messages match those defined in the validation rules.”
James Thompson (Lead Developer, CodeCraft Solutions). “A common pitfall when working with Laravel’s error bag is the misunderstanding of how to access the errors after a validation failure. Utilizing the `withErrors()` method correctly is vital, as it allows you to pass the errors to the session. Developers should also familiarize themselves with the `has()` and `first()` methods to efficiently check for and retrieve specific error messages.”
Linda Nguyen (Web Application Architect, TechSavvy). “When dealing with error messages in Laravel, it is important to remember that the MessageBag is designed to be flexible. However, developers often overlook the importance of checking for empty error bags before attempting to display messages. Implementing conditional checks can prevent unnecessary errors and enhance the user experience by providing clear feedback.”
Frequently Asked Questions (FAQs)
What is the purpose of the MessageBag in Laravel?
The MessageBag in Laravel is used to store and manage error messages, typically generated during form validation. It provides a convenient way to retrieve and display these messages in your application.
How can I access error messages stored in the MessageBag?
You can access error messages by using the `errors` variable in your views. For example, you can use `{{ $errors->first(‘field_name’) }}` to get the first error message for a specific field.
What is the difference between ErrorBag and MessageBag in Laravel?
The ErrorBag is a specific instance of the MessageBag that is used to handle validation errors. While both serve to store messages, ErrorBag is primarily focused on validation-related messages, whereas MessageBag can handle any type of message.
How do I check if there are any errors in the MessageBag?
You can check for errors by using the `any()` method on the MessageBag instance. For example, `if ($errors->any()) { // handle errors }` will evaluate to true if there are any error messages present.
Can I customize the error messages in the MessageBag?
Yes, you can customize error messages in Laravel by defining them in the `validation.php` language file or directly in the validation rules when using the `Validator` facade.
How do I clear error messages from the MessageBag?
You can clear error messages by using the `flush()` method on the MessageBag instance. This will remove all messages currently stored in the bag, allowing you to start fresh for the next validation.
In Laravel, the error handling mechanism is primarily facilitated through the use of the MessageBag class, which is designed to store and manage error messages. When validation fails, Laravel automatically populates the error bag with relevant messages that can be easily accessed and displayed in views. This functionality is crucial for providing users with immediate feedback regarding their input, ensuring a smooth user experience.
One of the key features of Laravel’s error handling is the ability to retrieve error messages for specific fields. Developers can access the error bag using the `errors()` helper function within their views. This allows for targeted display of messages associated with particular input fields, enhancing clarity and guiding users to correct their mistakes. Additionally, the error bag supports various methods for checking the existence of errors and retrieving messages, making it versatile and easy to use.
Overall, understanding how to effectively utilize Laravel’s MessageBag and error handling features is essential for any developer working with the framework. By leveraging these tools, developers can create more robust applications that not only validate user input but also provide meaningful feedback, ultimately leading to improved user satisfaction and reduced frustration.
Author Profile
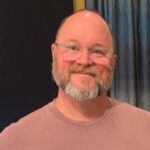
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?