How Does Laravel Handle Database Setup During Installation?
In the ever-evolving landscape of web development, Laravel has emerged as a powerful framework that streamlines the process of building robust applications. One of the pivotal aspects of deploying a Laravel application is its interaction with databases, which can significantly influence performance, scalability, and maintainability. As developers embark on the journey of installing Laravel, understanding the potential database configurations and choices becomes paramount. This article delves into the intricacies of Laravel’s database options during installation, shedding light on how these decisions can shape the future of your application.
When you install Laravel, one of the first considerations is the database you choose to work with. Laravel supports a variety of database systems, including MySQL, PostgreSQL, SQLite, and SQL Server, each offering unique features and benefits. The choice of database can affect not only the functionality of your application but also its performance and ease of use. As you navigate through the installation process, it’s essential to weigh the advantages and disadvantages of each option to ensure that your application is built on a solid foundation.
Moreover, Laravel’s elegant syntax and built-in tools, such as Eloquent ORM, facilitate seamless database interactions, making it easier for developers to manage data relationships and perform complex queries. However, the installation phase is where critical decisions are made
Database Configuration in Laravel
When installing Laravel, one of the crucial steps is configuring the database connection. Laravel supports various database systems, including MySQL, PostgreSQL, SQLite, and SQL Server, allowing developers to choose the one that best fits their project requirements.
To set up the database, you need to modify the `.env` file located in the root of your Laravel project. The following environment variables are essential for database configuration:
- `DB_CONNECTION`: The database driver (e.g., mysql, pgsql, sqlite, sqlsrv).
- `DB_HOST`: The hostname of your database server (e.g., 127.0.0.1 for local development).
- `DB_PORT`: The port number for the database connection (default is 3306 for MySQL).
- `DB_DATABASE`: The name of the database you want to connect to.
- `DB_USERNAME`: The username to access the database.
- `DB_PASSWORD`: The password for the provided username.
Here is an example of how your `.env` file might look for a MySQL connection:
“`plaintext
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
“`
Running Migrations
Once the database configuration is complete, you can leverage Laravel’s migration system to create and modify database tables. Migrations are like version control for your database, allowing you to define the structure of your tables using PHP code.
To run migrations, use the following Artisan command:
“`bash
php artisan migrate
“`
This command executes all pending migrations, creating the corresponding tables in your database. If you need to roll back the last migration, you can use:
“`bash
php artisan migrate:rollback
“`
It’s also possible to refresh all migrations by running:
“`bash
php artisan migrate:refresh
“`
Seeding the Database
In addition to migrations, Laravel offers a seeding feature to populate your database with initial data. This is particularly useful for testing or setting up a development environment with sample data.
To create a new seeder, you can use the Artisan command:
“`bash
php artisan make:seeder UserSeeder
“`
After defining the data you wish to insert in the `run` method of the seeder, execute it using:
“`bash
php artisan db:seed –class=UserSeeder
“`
You can also seed the database automatically after running migrations by adding the `–seed` flag:
“`bash
php artisan migrate –seed
“`
Database Connections Table
The following table outlines the key database connection settings for various database systems supported by Laravel:
Database System | DB_CONNECTION | Default Port |
---|---|---|
MySQL | mysql | 3306 |
PostgreSQL | pgsql | 5432 |
SQLite | sqlite | N/A |
SQL Server | sqlsrv | 1433 |
Understanding these configurations and tools will streamline your database management process within Laravel, enhancing your development efficiency and project organization.
Understanding Laravel’s Database Configuration on Install
Laravel provides a straightforward approach to database configuration during installation. When you set up a new Laravel project, the framework generates an `.env` file that contains essential environment variables, including database settings. These settings define how Laravel connects to your database.
Default Database Configuration
By default, Laravel is configured to use a MySQL database. The configuration parameters in the `.env` file include:
- DB_CONNECTION: The type of database (e.g., `mysql`, `pgsql`, `sqlite`, `sqlsrv`).
- DB_HOST: The hostname of the database server (default is `127.0.0.1` for local development).
- DB_PORT: The port number the database server listens on (default for MySQL is `3306`).
- DB_DATABASE: The name of the database to connect to.
- DB_USERNAME: The username for database authentication.
- DB_PASSWORD: The password for the specified database user.
Example `.env` configuration for MySQL:
“`
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_db
DB_USERNAME=root
DB_PASSWORD=secret
“`
Setting Up the Database
Before running your Laravel application, ensure the database is created and accessible. Follow these steps:
- Create the Database: Use your database management tool (e.g., phpMyAdmin, MySQL Workbench) to create a new database that matches the `DB_DATABASE` value in your `.env` file.
- Set Permissions: Ensure the user specified in `DB_USERNAME` has the necessary privileges to access and modify the database.
Migrations and Schema Management
Laravel’s migration system allows you to define your database schema programmatically. This provides a version control system for your database, ensuring consistent structure across different environments. Key features include:
- Creating Migrations: Use the Artisan command to create a new migration.
“`bash
php artisan make:migration create_users_table
“`
- Running Migrations: Apply migrations to your database with:
“`bash
php artisan migrate
“`
- Rolling Back Migrations: Undo the last batch of migrations using:
“`bash
php artisan migrate:rollback
“`
Database Seeding
Database seeding allows you to populate your database with sample data. This is particularly useful for testing and development. You can create seeders that define how to populate tables with initial data.
- Creating a Seeder: Generate a new seeder with:
“`bash
php artisan make:seeder UsersTableSeeder
“`
- Running Seeders: Execute seeders using:
“`bash
php artisan db:seed
“`
- Combining Migrations and Seeders: You can run migrations followed by seeders in one command:
“`bash
php artisan migrate –seed
“`
Testing Database Connections
To confirm your database connection is correctly configured, you can use Laravel’s built-in commands:
- Tinker: Launch an interactive shell to test database queries:
“`bash
php artisan tinker
“`
- Querying: Use Eloquent or the query builder to test connections directly.
Example using Eloquent:
“`php
use App\Models\User;
$users = User::all();
“`
By following these configurations and practices, you can effectively manage your Laravel application’s database setup and ensure smooth development processes.
Expert Insights on Laravel’s Database Configuration During Installation
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Laravel’s installation process is designed to streamline database configuration, allowing developers to specify their database connection details in the `.env` file. This flexibility is crucial for adapting to various environments, whether local or cloud-based.”
Michael Chen (Lead Developer, Open Source Solutions). “One of the key advantages of Laravel is its ability to manage database migrations seamlessly during installation. This feature ensures that developers can easily set up their database schema and maintain version control, which is essential for collaborative projects.”
Sarah Thompson (Database Architect, Cloud Data Systems). “Understanding Laravel’s database configuration options at installation can significantly impact application performance. Properly defining the database type and connection settings can enhance efficiency and scalability right from the start.”
Frequently Asked Questions (FAQs)
What is Laravel’s default database configuration upon installation?
Laravel, upon installation, defaults to using the SQLite database. However, it can be easily configured to use other databases such as MySQL, PostgreSQL, or SQL Server by modifying the `.env` file.
How do I change the database connection in Laravel?
To change the database connection, update the `DB_CONNECTION` variable in the `.env` file to your desired database type (e.g., `mysql`, `pgsql`, etc.) and ensure the corresponding connection details are provided.
Does Laravel automatically create a database on installation?
No, Laravel does not automatically create a database during installation. The database must be created manually or through migration commands after configuring the database connection.
What should I do if my database connection fails after installation?
If the database connection fails, check the `.env` file for correct database credentials, ensure the database server is running, and verify that the specified database exists.
Can I use Laravel without a database?
Yes, Laravel can be used without a database. You can develop applications with file storage, caching, or other services that do not require a database connection.
How can I set up a database migration in Laravel?
To set up a database migration in Laravel, use the Artisan command `php artisan make:migration create_table_name` to generate a migration file, then define the schema in the `up` method and run `php artisan migrate` to apply the changes.
In summary, the installation of Laravel, a popular PHP framework, often involves the configuration of a database, which is a critical step for any web application development. During the installation process, developers have the option to set up various database systems, including MySQL, PostgreSQL, SQLite, and SQL Server. The choice of database can significantly influence the performance, scalability, and overall architecture of the application. Laravel provides a robust set of tools and features that facilitate seamless database integration and management, ensuring that developers can focus on building their applications rather than wrestling with database complexities.
One of the key takeaways from the discussion is the importance of properly configuring the database during the installation phase. This includes setting up environment variables in the `.env` file, which allows Laravel to connect to the database securely and efficiently. Additionally, understanding the differences between the various database options available can help developers make informed decisions based on their specific project requirements. Utilizing Laravel’s migration and seeding features can further streamline the database setup process, enabling developers to maintain version control over their database schema and populate it with initial data easily.
Furthermore, leveraging Laravel’s Eloquent ORM simplifies database interactions, allowing developers to work with database records as if they were simple PHP objects. This
Author Profile
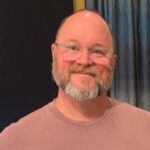
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?