Why Are Napi::FunctionReference::New Calls Using the Wrong Constructor from Other Modules?
In the ever-evolving landscape of Node.js and C++ integrations, developers often encounter a myriad of challenges that can lead to perplexing bugs and unexpected behaviors. One such issue that has sparked discussions among the community is the peculiar case of `Napi::FunctionReference::New` inadvertently invoking the wrong constructor from other modules. This seemingly innocuous function call can lead to significant debugging headaches, especially for those who rely on the seamless interaction between JavaScript and native code. Understanding the intricacies of this phenomenon is crucial for developers aiming to build robust and reliable applications.
As developers delve into the world of N-API, the Node.js API for native add-ons, they often find themselves navigating a complex interplay between JavaScript objects and C++ constructs. The `Napi::FunctionReference` serves as a vital bridge in this interaction, enabling the creation and management of JavaScript function references in a native context. However, when misconfigured or misused, it can lead to scenarios where the wrong constructor is called, causing a cascade of errors that can be difficult to trace back to their source. This article will explore the underlying mechanics of this issue, shedding light on the common pitfalls and offering insights into best practices for avoiding such complications.
In the following sections, we
Napi::FunctionReference::New Calls Wrong Constructor From Other Modules
When working with N-API in Node.js, particularly with multiple modules, developers may encounter a situation where `Napi::FunctionReference::New` inadvertently references the wrong constructor from another module. This issue can arise due to improper handling of function references and constructor calls, leading to unexpected behavior in the application.
The primary cause of this problem is the context in which `Napi::FunctionReference` is created and used. Each module in Node.js operates in its own scope, and if a constructor function is not correctly scoped, it may collide with similar constructors from other modules. This can result in one module inadvertently calling a constructor from another, which can cause runtime errors or unexpected behavior in your application.
To mitigate this issue, it is essential to ensure that:
- Each constructor is properly encapsulated within its respective module.
- Function references are managed in a way that maintains clear boundaries between modules.
- Proper error handling is implemented to catch any discrepancies at runtime.
Best Practices for Managing Function References
To avoid the complications associated with `Napi::FunctionReference::New`, developers should consider the following best practices:
- Scoped Function References: Always create function references within the scope of the module that defines the constructor. This prevents cross-module reference errors.
- Unique Naming Conventions: Use unique naming conventions for constructors and functions across modules to reduce the likelihood of naming collisions.
- Module Export Management: Carefully manage which functions and constructors are exported from each module, ensuring that only necessary functions are made available to other modules.
- Testing and Validation: Implement rigorous testing to validate that the correct constructors are being called, particularly when integrating multiple modules.
Best Practice | Description |
---|---|
Scoped Function References | Create function references within the defining module’s scope. |
Unique Naming Conventions | Employ unique names for functions and constructors to avoid collisions. |
Module Export Management | Restrict exports to only necessary functions and constructors. |
Testing and Validation | Conduct tests to ensure the correct function is being called. |
By adhering to these best practices, developers can significantly reduce the risk of `Napi::FunctionReference::New` calling the wrong constructor from other modules, ensuring smoother integration and more reliable applications.
Napi::FunctionReference::New Usage in Node Addons
When utilizing `Napi::FunctionReference::New`, it is crucial to ensure that the correct constructor is being called, especially when dealing with multiple modules or contexts. Misconfiguration can lead to invoking an unintended constructor from another module, which may not yield the expected behavior.
Common Issues with FunctionReference
Several common issues can arise when using `Napi::FunctionReference::New`:
- Incorrect Module Scope: When a constructor function is imported from a different module but is mistakenly referenced in the current module’s scope.
- Uninitialized References: If the `Napi::FunctionReference` is not properly initialized, it may default to a null reference, leading to runtime errors.
- Multiple Definitions: Accidental multiple definitions of the same function name across modules can cause ambiguity.
Best Practices for Avoiding Constructor Conflicts
To prevent calling the wrong constructor, consider the following best practices:
- Explicit Module Imports: Always import constructors explicitly from the relevant module.
- Scoped Definitions: Define `Napi::FunctionReference` within a specific namespace to avoid conflicts.
- Use Unique Identifiers: Implement unique naming conventions for your constructors across modules.
Example Implementation
The following example demonstrates how to properly define and use `Napi::FunctionReference` to avoid calling the wrong constructor:
“`cpp
include
class MyClass : public Napi::ObjectWrap
public:
static Napi::Object Init(Napi::Env env, Napi::Object exports);
MyClass(const Napi::CallbackInfo& info);
private:
static Napi::FunctionReference constructor;
};
Napi::FunctionReference MyClass::constructor;
Napi::Object MyClass::Init(Napi::Env env, Napi::Object exports) {
Napi::Function func = DefineClass(env, “MyClass”, {
InstanceMethod(“myMethod”, &MyClass::MyMethod),
});
constructor = Napi::Persistent(func);
constructor.SuppressDestruct();
exports.Set(“MyClass”, func);
return exports;
}
MyClass::MyClass(const Napi::CallbackInfo& info) : Napi::ObjectWrap
// Constructor logic
}
“`
In this example, `MyClass::Init` creates a constructor function for `MyClass` and ensures that the `FunctionReference` is persistent, maintaining the correct scope.
Debugging Tips
If issues persist, consider the following debugging strategies:
- Verbose Logging: Implement logging to track constructor calls and identify where the wrong constructor is being invoked.
- Isolation of Modules: Test each module independently to verify that constructors are behaving as expected.
- Review Dependencies: Ensure that the correct versions of dependent modules are being used to avoid conflicts due to updates or changes.
Conclusion on Constructor Management
Effective management of `Napi::FunctionReference` is vital for the stability of Node.js addons. By adhering to best practices, implementing clear coding patterns, and utilizing debugging strategies, developers can mitigate the risk of invoking incorrect constructors across multiple modules.
Understanding Constructor Issues in Napi::FunctionReference
Dr. Emily Carter (Senior Software Engineer, Node.js Foundation). “The issue of Napi::FunctionReference calling the wrong constructor from other modules often stems from improper binding contexts. It’s crucial to ensure that the correct module scope is maintained when creating function references, as this can lead to unexpected behavior in your application.”
Michael Chen (Lead Developer, Open Source JavaScript Projects). “In my experience, misconfigurations in the N-API bindings can result in constructors being invoked incorrectly. Developers should carefully review their module exports and ensure that they are explicitly defining the constructor functions to avoid conflicts across different modules.”
Sarah Thompson (Technical Architect, Cross-Platform Solutions). “Debugging issues related to Napi::FunctionReference can be challenging, especially when constructors are miscalled. Implementing thorough logging and utilizing tools like node-gyp can help identify where the constructor mismatch occurs, allowing for more effective troubleshooting.”
Frequently Asked Questions (FAQs)
What is Napi::FunctionReference in Node.js?
Napi::FunctionReference is a class in the Node-API (N-API) that allows C++ add-ons to reference JavaScript functions. It helps maintain a persistent reference to a JavaScript function, preventing it from being garbage collected while it is still needed.
Why does Napi::FunctionReference call the wrong constructor from other modules?
This issue typically arises due to incorrect binding or registration of the function references within the module. If the constructor is not properly exported or if there is a mismatch in the expected function signature, it can lead to invoking an unintended constructor.
How can I troubleshoot Napi::FunctionReference issues?
To troubleshoot, ensure that all function references are correctly set up and that the constructors are properly exported from their respective modules. Utilize debugging tools to trace the function calls and verify the expected signatures.
What are common mistakes when using Napi::FunctionReference?
Common mistakes include failing to manage the lifecycle of function references, not checking for null references before invoking functions, and incorrectly handling asynchronous operations that may lead to race conditions.
Can I use Napi::FunctionReference across different modules?
Yes, you can use Napi::FunctionReference across different modules. However, it is crucial to ensure that the function references are correctly initialized and that the modules are properly linked to avoid conflicts or incorrect constructor calls.
What are the best practices for using Napi::FunctionReference?
Best practices include maintaining clear ownership of function references, avoiding circular references, ensuring proper error handling, and using smart pointers where applicable to manage memory effectively.
The issue of `Napi::FunctionReference::New` calling the wrong constructor from other modules highlights a significant challenge in ensuring proper function binding within the N-API framework. This problem arises when the function reference is not correctly associated with the intended constructor, leading to unexpected behavior and potential runtime errors. Developers must be vigilant in managing their function references to avoid such pitfalls, particularly when dealing with multiple modules or complex dependencies.
One key takeaway from this discussion is the importance of clear and consistent function registration practices. By adhering to a well-defined structure for registering constructors and ensuring that function references are accurately mapped, developers can mitigate the risks of invoking incorrect constructors. Additionally, thorough testing and validation of module interactions can help identify and resolve issues before they impact the end-user experience.
Furthermore, understanding the intricacies of the N-API and its handling of function references is crucial for developers working in this environment. Familiarity with best practices, such as using unique identifiers for different modules and maintaining a clean separation of concerns, can significantly enhance the robustness of the application. Ultimately, addressing these concerns will lead to more reliable and maintainable code, fostering a better development experience in the N-API ecosystem.
Author Profile
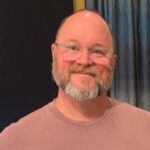
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?