How Can You Effectively Implement Waiting in Python?
In the fast-paced world of programming, efficiency is key, but sometimes, a little patience goes a long way. Whether you’re managing asynchronous tasks, handling user input, or simply pacing your application’s operations, knowing how to effectively implement waiting mechanisms in Python can make a significant difference. This article will guide you through the various techniques available in Python for introducing delays and managing time, ensuring your code runs smoothly and responsively.
When it comes to waiting in Python, there are several methods to consider, each suited for different scenarios. From simple time delays using built-in functions to more complex asynchronous programming techniques, Python offers a robust toolkit for managing time. Understanding these options not only enhances your coding skills but also helps you write more efficient and user-friendly applications.
As we dive deeper into the topic, you’ll discover how to leverage Python’s capabilities to control execution flow, synchronize tasks, and improve overall performance. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, mastering the art of waiting in Python is an essential step in your programming journey.
Using time.sleep()
The simplest way to introduce delays in Python is through the built-in `time` module, specifically using the `sleep()` function. This function halts the execution of the current thread for a specified number of seconds.
“`python
import time
Example of using time.sleep()
print(“Start waiting…”)
time.sleep(5) Wait for 5 seconds
print(“Done waiting!”)
“`
In this example, the program pauses for 5 seconds between the two print statements. The `sleep()` function can take either integer or floating-point values, allowing for precision in timing.
- Syntax: `time.sleep(seconds)`
- Parameters:
- `seconds`: A non-negative float or integer representing the number of seconds to wait.
Using threading.Event()
For more complex scenarios, particularly in multi-threaded environments, the `threading` module provides the `Event` class. This allows you to create a flag that can be used to control the flow of your program based on specific events.
“`python
import threading
event = threading.Event()
def wait_for_event():
print(“Waiting for event…”)
event.wait() Blocks until the event is set
print(“Event occurred!”)
thread = threading.Thread(target=wait_for_event)
thread.start()
Simulate some processing
time.sleep(3) Wait for 3 seconds
event.set() Trigger the event
“`
In this code, the `wait_for_event()` function will block until `event.set()` is called, allowing for synchronization between threads.
- Methods:
- `event.wait(timeout=None)`: Blocks until the flag is true or until the optional timeout occurs.
- `event.set()`: Sets the flag to true, unblocking any waiting threads.
- `event.clear()`: Resets the flag to .
Using asyncio.sleep()
When working with asynchronous code, the `asyncio` module provides the `sleep()` function, which is non-blocking and allows other tasks to run concurrently.
“`python
import asyncio
async def main():
print(“Start async wait…”)
await asyncio.sleep(2) Wait for 2 seconds
print(“Done async wait!”)
asyncio.run(main())
“`
The `await` keyword is used to pause the execution of the coroutine until the `asyncio.sleep()` completes, allowing other tasks to run during the wait time.
- Key Features:
- Non-blocking: Does not pause the entire program, making it suitable for I/O-bound tasks.
- Can be combined with other asyncio functions for better performance.
Method | Blocking | Use Case |
---|---|---|
time.sleep() | Yes | Simple delays in synchronous code |
threading.Event() | No | Thread synchronization |
asyncio.sleep() | No | Asynchronous programming |
In summary, the choice of method for implementing wait times in Python heavily depends on the specific requirements of your application, whether it be simple delays, multi-threaded operations, or asynchronous tasks.
Using time.sleep()
The simplest way to pause execution in Python is by using the `time.sleep()` function. This function allows you to specify the duration of the pause in seconds, including fractions of a second.
Example:
“`python
import time
print(“Start waiting…”)
time.sleep(5) Wait for 5 seconds
print(“Done waiting!”)
“`
Key Features:
- Accepts a single argument: the number of seconds to wait.
- Can be a float for fractional seconds (e.g., `time.sleep(0.5)` for half a second).
Using threading.Event()
For more control over waiting in a multithreaded environment, `threading.Event()` is an effective approach. This allows threads to wait until a specific condition is met.
Example:
“`python
import threading
event = threading.Event()
def wait_for_event():
print(“Waiting for the event to be set…”)
event.wait() Block until the event is set
print(“Event has been set!”)
thread = threading.Thread(target=wait_for_event)
thread.start()
Simulate some processing
time.sleep(3)
event.set() Set the event to unblock the waiting thread
“`
Key Features:
- Allows threads to wait for an event.
- Offers a `set()` method to unblock waiting threads.
- Supports a timeout feature with `wait(timeout)`.
Using asyncio.sleep()
In asynchronous programming, `asyncio.sleep()` provides a non-blocking way to wait. This function is part of the `asyncio` library, which is designed for writing concurrent code using the async/await syntax.
Example:
“`python
import asyncio
async def main():
print(“Start waiting asynchronously…”)
await asyncio.sleep(2) Non-blocking wait for 2 seconds
print(“Done waiting asynchronously!”)
asyncio.run(main())
“`
Key Features:
- Compatible with the async/await syntax.
- Non-blocking, allowing other tasks to run during the wait.
- Ideal for I/O-bound operations where you want to maintain responsiveness.
Using timeit for Timing Execution
If the goal is to measure the time taken by a particular block of code, the `timeit` module is a robust option. This is particularly useful for benchmarking.
Example:
“`python
import timeit
execution_time = timeit.timeit(“time.sleep(1)”, setup=”import time”, number=1)
print(f”Execution time: {execution_time} seconds”)
“`
Key Features:
- Measures execution time of small code snippets.
- Automatically handles setup and teardown code.
- Provides accurate timing, reducing the impact of background processes.
Waiting for User Input
Sometimes, waiting for user input can be a form of waiting in Python. The `input()` function halts execution until the user provides some input.
Example:
“`python
input(“Press Enter to continue…”)
print(“Continuing execution.”)
“`
Key Features:
- Blocks until the user presses Enter.
- Can be customized with a message prompting the user.
Comparison Table of Waiting Methods
Method | Blocking | Non-blocking | Use Case |
---|---|---|---|
time.sleep() | Yes | No | Simple pauses |
threading.Event() | Yes | No | Thread synchronization |
asyncio.sleep() | No | Yes | Asynchronous tasks |
timeit | Yes | N/A | Measuring execution time |
input() | Yes | No | Waiting for user input |
Expert Insights on Implementing Waits in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, implementing wait mechanisms is crucial for managing asynchronous tasks effectively. Utilizing the `asyncio.sleep()` function allows developers to pause execution without blocking the entire program, which is essential for maintaining responsiveness in applications.”
Michael Chen (Lead Developer, Python Development Group). “When working with web scraping or API calls, using `time.sleep()` can be beneficial for rate limiting. However, it’s important to balance wait times to avoid excessive delays that could hinder performance and user experience.”
Sarah Thompson (Python Instructor, Code Academy). “Teaching newcomers to Python, I emphasize the importance of understanding both `time.sleep()` and `threading.Event().wait()`. Each serves different purposes, and knowing when to use them is key to writing efficient and effective Python code.”
Frequently Asked Questions (FAQs)
How do I pause execution in Python?
You can pause execution in Python using the `time.sleep(seconds)` function from the `time` module, where `seconds` is the number of seconds you want the program to wait.
What is the difference between time.sleep() and threading.Event().wait()?
`time.sleep()` halts the execution of the current thread for a specified duration, while `threading.Event().wait()` can pause execution until a certain condition is met or a timeout occurs, allowing for more complex synchronization.
Can I use asyncio to wait in Python?
Yes, you can use `await asyncio.sleep(seconds)` within an asynchronous function to pause execution without blocking the event loop, enabling other tasks to run concurrently.
How do I implement a countdown timer in Python?
You can implement a countdown timer using a loop that decrements a variable and calls `time.sleep(1)` to wait for one second between each decrement, printing the remaining time at each step.
Is there a way to wait for user input in Python?
Yes, you can use the `input()` function to pause execution until the user provides input, effectively waiting for the user to press Enter or type a response.
What happens if I use time.sleep() in a GUI application?
Using `time.sleep()` in a GUI application will freeze the interface during the sleep period. It is recommended to use threading or asynchronous techniques to avoid blocking the main thread and keep the interface responsive.
In Python, waiting or pausing the execution of a program can be accomplished through various methods, each serving distinct purposes depending on the context. The most common approach is using the `time.sleep()` function, which allows developers to specify a duration in seconds for which the program should halt. This is particularly useful for creating delays in scripts, managing timing in animations, or pacing the execution of tasks.
Another method to implement waiting in Python is through asynchronous programming with the `asyncio` library. This approach enables non-blocking waits, allowing other tasks to run concurrently while waiting for a specified condition or event. Using `await asyncio.sleep()` is a typical pattern in asynchronous code, facilitating efficient resource management and responsiveness in applications.
Additionally, waiting can be implemented in the context of threading and multiprocessing, where synchronization mechanisms such as `Event`, `Condition`, or `Semaphore` can be utilized. These constructs allow threads or processes to wait for specific signals or conditions, ensuring that resources are accessed in a controlled manner and preventing race conditions.
In summary, understanding how to effectively wait in Python is crucial for developing robust applications. Whether through simple delays with `time.sleep()`, asynchronous waits with `asyncio`, or synchronization in
Author Profile
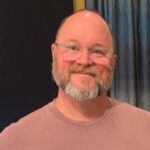
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?