How Can You Execute a PowerShell Script from a Batch File?
In the world of Windows automation, the ability to seamlessly integrate different scripting languages can significantly enhance productivity and streamline workflows. One such powerful combination is executing PowerShell scripts from batch files. This synergy allows users to harness the simplicity of batch files while tapping into the advanced capabilities of PowerShell, creating a robust solution for automating repetitive tasks, managing system configurations, and executing complex commands with ease. Whether you’re a seasoned IT professional or a curious newcomer, understanding how to execute PowerShell scripts from batch files can open up a realm of possibilities for efficient task management.
At its core, executing PowerShell scripts from batch files involves a straightforward process that bridges the gap between two powerful scripting environments. Batch files, with their traditional command-line interface, provide a familiar platform for executing commands, while PowerShell brings a modern, object-oriented approach to scripting. This combination not only enhances the functionality of your scripts but also allows for greater flexibility in handling various tasks, from file manipulation to system administration.
As we delve deeper into this topic, you will discover the essential techniques for invoking PowerShell scripts within batch files, along with best practices for error handling and script management. By mastering this integration, you can elevate your automation strategies and unlock the full potential of your Windows environment, making your scripting endeavors more efficient
Understanding PowerShell Execution Policies
PowerShell execution policies govern the conditions under which PowerShell scripts can run on a system. It is crucial to set these policies appropriately when executing scripts from a batch file to avoid permission issues. The four primary execution policies include:
- Restricted: No scripts can be run. This is the default setting.
- AllSigned: Only scripts signed by a trusted publisher can be run.
- RemoteSigned: Scripts created locally can run, but those downloaded from the internet must be signed by a trusted publisher.
- Unrestricted: All scripts can run, but will prompt the user for confirmation when executing downloaded scripts.
To check the current execution policy, you can use the following command in PowerShell:
“`powershell
Get-ExecutionPolicy
“`
To change the execution policy, use:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Be cautious when changing execution policies, as they can expose the system to security risks if set incorrectly.
Creating a Batch File to Execute PowerShell Scripts
To execute a PowerShell script from a batch file, you need to use the `powershell` command followed by the script path. Here’s a basic structure:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1”
“`
In this example, the `-ExecutionPolicy Bypass` parameter allows the script to run without being blocked by the execution policy, which is useful for scripts that need to be executed in environments with strict policies.
Example of a Batch File
Below is a simple example of a batch file that executes a PowerShell script:
“`batch
@echo off
REM Change directory to where the script is located
cd “C:\Scripts”
REM Execute the PowerShell script
powershell -ExecutionPolicy Bypass -File “ExampleScript.ps1”
“`
This batch file changes the directory to `C:\Scripts`, then runs `ExampleScript.ps1` using PowerShell.
Handling Script Parameters
If your PowerShell script requires parameters, you can pass them directly from the batch file. Here’s how to do it:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 “Value2”
“`
In this example, `-Param1` and `-Param2` are parameters that `YourScript.ps1` expects.
Testing and Debugging
When executing PowerShell scripts from batch files, consider implementing logging and error handling to streamline debugging. Here’s a simple way to log output:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” > “C:\Path\To\log.txt” 2>&1
“`
This command redirects both standard output and error messages to a log file, allowing for easier troubleshooting.
Parameter | Description |
---|---|
-ExecutionPolicy | Sets the execution policy for the session. |
-File | Specifies the script file to execute. |
2>&1 | Redirects error output to standard output. |
By following these guidelines, you can effectively execute PowerShell scripts from batch files while managing execution policies and handling parameters appropriately.
Executing a PowerShell Script from a Batch File
To execute a PowerShell script from a batch file, you can use the `powershell.exe` command with specific parameters. This method allows you to seamlessly integrate PowerShell functionality into your batch processing workflows.
Basic Syntax
The basic command structure to run a PowerShell script from a batch file is as follows:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1”
“`
Explanation of Parameters
- `-ExecutionPolicy Bypass`: This parameter temporarily bypasses the execution policy, allowing the script to run without restrictions. This is particularly useful for scripts that might not be signed.
- `-File`: This parameter indicates that you are specifying a script file to execute.
Creating the Batch File
- Open a text editor (e.g., Notepad).
- Enter the PowerShell command using the syntax provided.
- Save the file with a `.bat` extension, for example, `runScript.bat`.
Example Batch File
“`batch
@echo off
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
pause
“`
- `@echo off`: This command prevents the commands from being displayed in the command prompt.
- `pause`: This command keeps the command prompt window open until a key is pressed, allowing the user to see any output or error messages.
Handling Script Parameters
If your PowerShell script requires parameters, you can pass them directly in the batch file. Modify the command as follows:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
Example with Parameters
“`batch
@echo off
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1” -InputFile “C:\Data\Input.txt” -OutputFile “C:\Data\Output.txt”
pause
“`
Common Issues and Troubleshooting
When executing PowerShell scripts from batch files, you may encounter several common issues:
Issue | Description | Solution |
---|---|---|
Script not found | The specified path to the script is incorrect. | Verify the script path is correct. |
Execution policy error | The script is blocked by the execution policy. | Use `-ExecutionPolicy Bypass` in the command. |
Permissions issues | The script may not have permission to run. | Run the batch file as an administrator. |
Output not visible | The command prompt closes immediately after execution. | Include a `pause` command to view output. |
Running PowerShell Commands Directly
If you prefer to run PowerShell commands directly from the batch file without a separate script, use the following syntax:
“`batch
powershell.exe -ExecutionPolicy Bypass -Command “Your-PowerShell-Command”
“`
Example of Direct Command Execution
“`batch
@echo off
powershell.exe -ExecutionPolicy Bypass -Command “Get-Process | Where-Object { $_.CPU -gt 100 }”
pause
“`
This command retrieves processes that are using more than 100 CPU units.
Best Practices
- Use full paths: Always specify full paths for scripts and files to avoid path resolution issues.
- Error handling: Incorporate error handling in PowerShell scripts to manage exceptions gracefully.
- Testing: Test the batch file and PowerShell scripts in a safe environment before deploying them in production.
Expert Insights on Executing PowerShell Scripts from Batch Files
James Carter (Senior Systems Administrator, Tech Solutions Inc.). “Executing PowerShell scripts from batch files is a powerful method for automating tasks in Windows environments. It allows system administrators to leverage the capabilities of PowerShell while maintaining the simplicity of batch scripting, which is essential for legacy systems.”
Linda Chen (IT Security Consultant, CyberSafe Networks). “When integrating PowerShell scripts within batch files, it is crucial to consider security implications. Ensuring that scripts are signed and that execution policies are properly configured can help mitigate risks associated with running scripts in an automated fashion.”
Robert Ellis (DevOps Engineer, Cloud Innovations). “Batch files can serve as a great entry point for executing PowerShell scripts, especially in CI/CD pipelines. The key is to ensure that the environment is set up correctly, with the necessary permissions and paths configured to avoid execution errors.”
Frequently Asked Questions (FAQs)
How can I execute a PowerShell script from a batch file?
To execute a PowerShell script from a batch file, use the following command: `powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1″`. This command bypasses the execution policy and runs the specified PowerShell script.
What does the ExecutionPolicy parameter do?
The ExecutionPolicy parameter determines the level of security applied to PowerShell scripts. By using `Bypass`, you allow the script to run without restrictions, which is useful for automation but should be used cautiously.
Can I pass arguments to a PowerShell script from a batch file?
Yes, you can pass arguments by appending them after the script path in the command. For example: `powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” -Argument1 Value1 -Argument2 Value2`.
What should I do if the PowerShell script does not execute?
If the script does not execute, check the following: ensure the script path is correct, verify that the script is not blocked by Windows, and confirm that your batch file has the necessary permissions to run PowerShell.
Is it possible to run a PowerShell script silently from a batch file?
Yes, to run a PowerShell script silently, you can use the `-WindowStyle Hidden` parameter in your command, like this: `powershell -ExecutionPolicy Bypass -WindowStyle Hidden -File “C:\Path\To\YourScript.ps1″`.
What are the security implications of executing PowerShell scripts from batch files?
Executing PowerShell scripts from batch files can pose security risks, especially when using `Bypass` for the ExecutionPolicy. Ensure that the scripts are from trusted sources and consider implementing additional security measures, such as code signing.
Executing a PowerShell script from a batch file is a straightforward process that enhances the capabilities of batch scripting by integrating the powerful features of PowerShell. This approach allows users to leverage the advanced functionalities of PowerShell while maintaining the simplicity of batch files. By using the appropriate command syntax, users can seamlessly call PowerShell scripts, enabling automation and task management across various Windows environments.
One of the primary methods to execute a PowerShell script from a batch file involves using the `powershell.exe` command followed by the `-File` parameter, which specifies the path to the script. This method ensures that the script runs in the PowerShell environment, allowing for access to all the cmdlets and functionalities that PowerShell offers. Additionally, users can pass parameters to the script, enhancing its flexibility and reusability.
Another important consideration is the execution policy of PowerShell, which may restrict the running of scripts. Users must ensure that the execution policy is set appropriately, using commands such as `Set-ExecutionPolicy`, to allow scripts to run without hindrance. This aspect is crucial for maintaining security while enabling script execution.
In summary, executing PowerShell scripts from batch files provides a powerful tool for automation and system management.
Author Profile
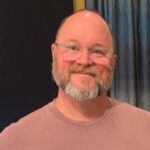
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?