How Can You Achieve Complex Tasks in Python with Just One Line of Code?
In the world of programming, efficiency and elegance often go hand in hand, and Python is a language that embodies this philosophy. One of the most intriguing aspects of Python is its ability to condense complex operations into concise, readable statements. The phrase “If In One Line Python” captures the essence of this capability, inviting developers to explore how they can streamline their code while maintaining clarity. Whether you’re a seasoned programmer or a curious beginner, understanding how to leverage one-liners can significantly enhance your coding prowess and improve the overall performance of your applications.
Python’s syntax is designed to be intuitive, allowing users to express ideas with minimal code. This simplicity opens the door to powerful one-liners that can execute conditional logic without the need for verbose structures. Imagine being able to implement decision-making processes in your code using just a single line, making your scripts not only shorter but also easier to read and maintain. This approach not only saves time during development but also reduces the likelihood of errors, as fewer lines of code often lead to fewer bugs.
As we delve deeper into the art of crafting one-liners in Python, we will explore various techniques and best practices that can transform your coding style. From leveraging conditional expressions to utilizing built-in functions, the potential for creating efficient
Using If Statements in One Line
Python allows for concise expressions of conditional logic through the use of ternary conditional operators. This can be particularly useful when you want to write succinct code without compromising clarity. The syntax for a one-liner if statement in Python is as follows:
“`python
value_if_true if condition else value_if_
“`
This structure provides a clear and efficient way to assign a value based on a condition. Here’s an example:
“`python
result = “Even” if number % 2 == 0 else “Odd”
“`
In this case, the variable `result` will be assigned “Even” if `number` is even, and “Odd” otherwise.
Practical Examples
One-liner if statements can streamline your code in various scenarios. Below are some practical examples:
- Assigning Values: Assign a value based on a condition.
“`python
status = “Active” if user.is_active else “Inactive”
“`
- Conditional Output: Print a message based on a condition.
“`python
print(“Welcome back!” if user.is_logged_in else “Please log in.”)
“`
- List Comprehensions: Create a list with conditional logic.
“`python
even_numbers = [num for num in numbers if num % 2 == 0]
“`
Considerations for Readability
While one-liner if statements can enhance brevity, they can also impact code readability. It is essential to balance conciseness with clarity. Here are some guidelines:
- Simplicity: Use one-liners for simple conditions. If the logic is complex, consider a traditional multi-line if statement.
- Commenting: Include comments for clarity if the one-liner is not immediately understandable.
- Consistent Style: Maintain consistency across your codebase to avoid confusion.
Comparison of One-liner vs. Multi-line If Statements
The following table illustrates the differences between one-liner if statements and traditional multi-line if statements:
Aspect | One-liner If Statement | Multi-line If Statement |
---|---|---|
Length | Shorter, more concise | Longer, more verbose |
Readability | Generally more readable | |
Use Case | Simple conditions | Complex logic |
Performance | Similar performance | Similar performance |
while one-liner if statements offer a streamlined approach to conditional logic in Python, careful consideration of readability and complexity is vital to maintain code quality. Use them judiciously to enhance your coding efficiency while ensuring clarity for anyone who may read your code in the future.
Using Conditional Expressions
In Python, conditional expressions allow you to perform an if-else operation in a single line. This is particularly useful for simple decisions within list comprehensions or when assigning variables based on conditions. The syntax is as follows:
“`python
x if condition else y
“`
This expression evaluates `condition`. If true, it returns `x`; otherwise, it returns `y`.
Example:
“`python
result = “Even” if number % 2 == 0 else “Odd”
“`
List Comprehensions with Conditional Logic
List comprehensions can incorporate if statements to filter or modify the elements of a list in a concise manner. The syntax is:
“`python
[expression for item in iterable if condition]
“`
Example:
“`python
squares = [x**2 for x in range(10) if x % 2 == 0]
“`
This generates a list of squares for even numbers between 0 and 9.
Lambda Functions for One-Line Definitions
Lambda functions in Python are small anonymous functions defined using the `lambda` keyword. They can encapsulate simple logic succinctly.
Syntax:
“`python
lambda arguments: expression
“`
Example:
“`python
add = lambda a, b: a + b
result = add(5, 3) Returns 8
“`
Lambda functions are often used with functions like `map()`, `filter()`, and `reduce()`.
One-Liner for Looping and Accumulating Values
Python allows for the aggregation of values using a single line with the `sum()` function combined with a generator expression.
Example:
“`python
total = sum(x for x in range(1, 101) if x % 2 != 0)
“`
This calculates the sum of all odd numbers from 1 to 100.
Using the `any()` and `all()` Functions
The `any()` and `all()` functions provide a way to evaluate iterables for conditions in a single line.
**Example:**
“`python
has_even = any(x % 2 == 0 for x in my_list)
all_positive = all(x > 0 for x in my_list)
“`
- `any()` returns `True` if at least one element is true.
- `all()` returns `True` only if all elements are true.
Dictionary Comprehensions
Similar to list comprehensions, dictionary comprehensions provide a way to create dictionaries in a single line.
Syntax:
“`python
{key_expression: value_expression for item in iterable if condition}
“`
Example:
“`python
squared_dict = {x: x**2 for x in range(5)}
“`
This generates a dictionary where keys are numbers and values are their squares.
Chaining Functions for Concise Operations
You can chain functions together to execute multiple operations in one line. This is particularly effective with methods that return values.
Example:
“`python
result = my_list.sort(); my_list.reverse()
“`
This modifies `my_list` by first sorting it and then reversing it, all in one line.
Exception Handling in One Line
While exception handling is typically verbose, you can use a one-liner for simple cases with a conditional expression.
Example:
“`python
value = safe_function() if safe_condition else default_value
“`
This ensures that `value` is assigned based on the success of `safe_function()` or defaults to `default_value`.
Expert Insights on Python’s One-Liner Capabilities
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Python’s ability to condense complex operations into a single line enhances code readability and efficiency, making it a favorite among developers who prioritize clean and maintainable code.”
Marcus Lee (Data Scientist, Analytics Hub). “One-liners in Python can significantly speed up data manipulation tasks. However, it’s crucial to balance brevity with clarity to ensure that the code remains understandable for future maintenance.”
Sarah Thompson (Python Educator, Code Academy). “Teaching Python one-liners can empower new programmers to think creatively about problem-solving, but instructors should emphasize the importance of writing clear, well-structured code alongside these concise expressions.”
Frequently Asked Questions (FAQs)
What does “If In One Line” mean in Python?
The phrase “If In One Line” refers to the use of a single line of code to implement conditional statements in Python, typically using a ternary operator or a concise syntax.
How can I write an if statement in one line in Python?
You can use the syntax `value_if_true if condition else value_if_` to write an if statement in one line. This allows for a compact representation of conditional logic.
Can I use “if” statements in list comprehensions in Python?
Yes, you can include “if” statements in list comprehensions, allowing you to filter items based on a condition while generating a new list. The syntax is `[expression for item in iterable if condition]`.
What are the benefits of using one-liner if statements in Python?
One-liner if statements enhance code readability and conciseness, making it easier to express simple conditions without the overhead of multiple lines of code.
Are there any drawbacks to using one-liner if statements?
One-liner if statements can reduce code clarity when overused or applied to complex conditions, making it harder to understand the logic at a glance. It’s important to balance brevity with readability.
Can I use multiple conditions in a one-liner if statement?
Yes, you can chain multiple conditions using logical operators (and, or) within a one-liner if statement, but ensure that the expression remains clear and comprehensible.
In the realm of programming, particularly with Python, the phrase “if in one line” refers to the ability to condense conditional statements into a single line of code. This approach is often achieved through the use of the ternary conditional operator, which allows developers to streamline their code, enhancing readability and efficiency. By utilizing this technique, programmers can express simple conditional logic succinctly, making their code more elegant and easier to maintain.
One of the key takeaways from the discussion surrounding this topic is the balance between brevity and clarity. While writing conditions in a single line can reduce the amount of code and improve visual organization, it is crucial to ensure that the resulting code remains understandable. Overly complex one-liners can lead to confusion and hinder maintainability, which is counterproductive to the goals of clean coding practices.
Ultimately, mastering the use of one-liners in Python can significantly improve a developer’s coding style. However, it is essential to apply this technique judiciously, ensuring that it serves to enhance the overall quality of the code rather than detract from it. By maintaining a focus on clarity and simplicity, programmers can leverage the power of concise conditional expressions to write more effective and efficient Python code.
Author Profile
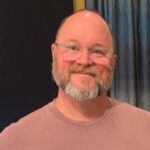
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?