How Can You Effectively Run a Python Script in Python?
Introduction
In the world of programming, Python stands out as a versatile and user-friendly language that has captured the hearts of both beginners and seasoned developers alike. Whether you’re automating mundane tasks, analyzing data, or developing complex applications, knowing how to run a Python script is an essential skill that can unlock a multitude of possibilities. As you embark on your journey with Python, understanding the various methods to execute your scripts will not only enhance your coding efficiency but also empower you to bring your ideas to life with ease.
Running a Python script may seem straightforward, but it encompasses a range of techniques that cater to different environments and use cases. From utilizing the command line interface to leveraging integrated development environments (IDEs), each method offers unique advantages that can streamline your workflow. Additionally, understanding how to manage script execution in different contexts—such as within virtual environments or as part of larger applications—can significantly impact your development experience.
As we delve deeper into this topic, we will explore the fundamental approaches to executing Python scripts, providing you with the knowledge and tools necessary to harness the full potential of this powerful programming language. Whether you’re looking to run simple scripts or integrate them into more complex systems, this guide will serve as your roadmap to mastering the art of Python script execution.
Running a Python Script from the Command Line
To execute a Python script from the command line, follow these steps:
- Open your command line interface (Command Prompt on Windows, Terminal on macOS and Linux).
- Navigate to the directory where your Python script is located using the `cd` command. For example:
cd path\to\your\script
- Run the script using the Python interpreter by typing:
python script_name.py
or, if you are using Python 3 and the default `python` command points to Python 2, you may need to specify:
python3 script_name.py
Make sure to replace `script_name.py` with the actual name of your Python file. If your script has executable permissions (on Unix-like systems), you can also run it directly by prefixing it with `./`:
./script_name.py
Executing a Python Script within Another Python Script
You can run one Python script from another by using the `import` statement or the `exec` function. Here’s how to do it:
- Using import: This method is suitable for reusable code modules. For example:
python
import script_name
This will execute the top-level code in `script_name.py`.
- Using exec: This method is more dynamic and can be used to execute scripts contained in a string or file:
python
with open(‘script_name.py’) as file:
exec(file.read())
Be cautious when using `exec`, as it can execute arbitrary code and potentially lead to security risks.
Running Python Scripts in Integrated Development Environments (IDEs)
Most IDEs provide straightforward methods to run Python scripts:
- PyCharm:
- Open your script in the editor.
- Right-click anywhere in the editor and select “Run ‘script_name'”.
- VS Code:
- Open the script.
- Press `F5` to run the script or use the Run button in the top right corner.
- Jupyter Notebook:
- Create a new cell.
- Type the command:
python
%run script_name.py
- Execute the cell.
Common Errors When Running Python Scripts
While executing Python scripts, you may encounter several common errors. Here’s a table summarizing these errors and their potential solutions:
Error Type | Description | Solution |
---|---|---|
SyntaxError | Occurs when Python encounters incorrect syntax. | Check for typos, missing colons, or mismatched parentheses. |
FileNotFoundError | Triggered when the specified script file cannot be found. | Verify the file path and filename are correct. |
IndentationError | Happens when indentation is inconsistent. | Ensure consistent use of tabs or spaces for indentation. |
NameError | Occurs when a variable is referenced before assignment. | Check if all variables are defined before they are used. |
By understanding these methods and common pitfalls, you can effectively run Python scripts in various environments.
Running Python Scripts from the Command Line
To execute a Python script from the command line, follow these steps:
- Open the Command Line Interface: This can be done by searching for Command Prompt on Windows or Terminal on macOS/Linux.
- Navigate to the Script’s Directory: Use the `cd` command to change directories to where your Python script is located. For example:
bash
cd path/to/your/script
- Run the Script: Execute the script by typing the following command:
bash
python script_name.py
Replace `script_name.py` with the actual name of your Python file. If you have multiple versions of Python installed, you might need to use `python3` instead.
Executing Python Scripts within an IDE
Integrated Development Environments (IDEs) offer tools for executing scripts directly. Here’s how to run a Python script in popular IDEs:
- PyCharm:
- Open your project.
- Right-click on the script file in the Project pane.
- Select “Run ‘script_name’”.
- Visual Studio Code:
- Open the script file.
- Click on the green play button in the top-right corner or use the shortcut `Ctrl + F5`.
- Jupyter Notebook:
- Open a notebook.
- Write your Python code in a cell.
- Press `Shift + Enter` to execute the cell.
Using Python’s Interactive Mode
Python can also be run in an interactive mode, which is useful for testing snippets of code:
- Open the Command Line: Just like before, open your command line interface.
- Start Python: Type `python` or `python3` and press Enter. You will enter the interactive shell.
- Type Your Code: Enter your Python commands directly into the shell. For example:
python
print(“Hello, World!”)
- Exit Interactive Mode: Type `exit()` or press `Ctrl + Z` (Windows) or `Ctrl + D` (macOS/Linux).
Scheduling Python Scripts
To run Python scripts at specific times, consider using scheduling tools:
- Windows Task Scheduler:
- Open Task Scheduler and create a new task.
- Set the trigger and action to run your Python script using the command line.
- Cron Jobs on Linux/macOS:
- Open the terminal and type `crontab -e` to edit your cron jobs.
- Add a line in the format:
bash
- * * * * python /path/to/your/script.py
- Adjust the asterisks to set the desired schedule.
Using Python’s Built-in `exec` Function
Python allows you to run scripts programmatically within another script using the `exec` function:
python
with open(‘script_name.py’) as file:
exec(file.read())
This method can be useful for dynamically executing code or scripts.
Running Python Scripts from a Text Editor
Some text editors provide built-in functionality to run Python scripts directly:
– **Sublime Text**:
- Install the SublimeREPL package.
- Open the script and select Tools > SublimeREPL > Python.
- Atom:
- Install the script package.
- Open your script and press `Ctrl + Shift + B` to run it.
This facilitates quick testing and development without switching between applications.
Expert Insights on Running Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively run a Python script, one must ensure that the Python interpreter is properly installed on their system. Utilizing the command line interface, users can execute scripts by navigating to the script’s directory and entering ‘python script_name.py’. This method is fundamental for both beginners and seasoned developers.”
Mark Thompson (Lead Python Developer, CodeCrafters). “For running Python scripts efficiently, I recommend using virtual environments. This practice not only isolates dependencies but also allows for seamless execution of scripts across different projects. The command ‘python -m venv env_name’ creates a new environment, which can then be activated to run your scripts without conflicts.”
Sarah Lee (Python Educator, LearnPython.org). “Understanding how to run a Python script is crucial for anyone learning the language. Beyond the command line, utilizing integrated development environments (IDEs) like PyCharm or VSCode can enhance the experience. These tools provide built-in features that simplify script execution and debugging, making them ideal for learners.”
Frequently Asked Questions (FAQs)
How do I run a Python script from the command line?
To run a Python script from the command line, open your terminal or command prompt, navigate to the directory containing the script, and type `python script_name.py`, replacing `script_name.py` with the actual name of your script.
Can I run a Python script in an integrated development environment (IDE)?
Yes, you can run a Python script in an IDE such as PyCharm, Visual Studio Code, or Jupyter Notebook. Simply open the script in the IDE and look for a “Run” option or use a designated shortcut (often F5 or Shift + Enter).
What is the difference between running a script with ‘python’ and ‘python3’?
The command `python` typically refers to Python 2.x, while `python3` explicitly refers to Python 3.x. Use `python3` to ensure compatibility with scripts written for Python 3.
How can I run a Python script in the background on a Unix-like system?
To run a Python script in the background on a Unix-like system, append an ampersand (`&`) to the command. For example, use `python script_name.py &` to execute the script without blocking the terminal.
Is it possible to run a Python script automatically at startup?
Yes, you can configure your operating system to run a Python script at startup. On Windows, you can place a shortcut to the script in the Startup folder. On Linux, you can add it to the crontab or use systemd services.
How do I pass command-line arguments to a Python script?
You can pass command-line arguments to a Python script by including them after the script name in the command line. Access these arguments within the script using the `sys.argv` list from the `sys` module.
In summary, running a Python script in Python involves several straightforward methods that cater to different user needs and environments. Users can execute scripts directly from the command line, through integrated development environments (IDEs), or by utilizing text editors that support Python execution. Each method offers unique advantages, such as ease of use, debugging capabilities, and project management features, which can enhance the overall development experience.
Additionally, understanding how to run Python scripts effectively can significantly streamline the coding process. It is essential to be familiar with the command-line interface, as it provides a direct way to execute scripts and manage Python environments. Furthermore, leveraging IDEs and text editors can improve productivity through features like syntax highlighting, code completion, and version control integration.
Ultimately, the choice of method for running Python scripts depends on the user’s specific requirements and preferences. By exploring various execution techniques, users can optimize their workflow, reduce errors, and enhance their programming skills. This knowledge is fundamental for anyone looking to develop robust Python applications or engage in data analysis and automation tasks.
Author Profile
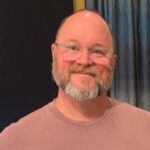
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?