Why Must the Initial Value of a Reference to Non-Const Be an Lvalue?
In the realm of C++ programming, the nuances of variable types and references can often lead to perplexing errors, especially for those venturing into the depths of object-oriented design. One such error that can stump even seasoned developers is the message: “Initial value of reference to non-const must be an lvalue.” This seemingly cryptic warning serves as a crucial reminder of the distinctions between lvalues and rvalues, and how they interact with references in C++. Understanding this concept is not only essential for writing robust code but also for mastering the subtleties of C++’s memory management and performance optimization.
At its core, the error highlights a fundamental principle of C++: references to non-const types must be initialized with lvalues, which are essentially variables that occupy identifiable locations in memory. This requirement ensures that the reference can safely modify the value it points to, maintaining the integrity of the program’s state. Conversely, rvalues, which are temporary objects or literals, cannot be bound to non-const references, as they do not have a persistent address in memory. This distinction is pivotal for developers to grasp, as it directly impacts how they manage data and resources within their applications.
Navigating the intricacies of lvalues and rvalues can be
Understanding Lvalues and Rvalues
In C++, the terms lvalue and rvalue refer to the value categories that specify the memory location and the type of operations that can be performed on them. An lvalue (locator value) represents an object that occupies some identifiable location in memory. This means it has a persistent address, allowing for taking its address, modifying its value, and passing it to functions expecting a reference.
Conversely, an rvalue (read value) is a temporary object that does not occupy a specific memory location; it is often the result of expressions or function calls. Lvalues can appear on the left-hand side of an assignment, while rvalues typically appear on the right-hand side.
To further clarify:
- Lvalue:
- Has an identifiable location in memory.
- Can be assigned a new value.
- Example: Variables, dereferenced pointers.
- Rvalue:
- Does not have a persistent memory address.
- Usually temporary and can’t be assigned to.
- Example: Literals, temporary objects from expressions.
Reference Types in C++
In C++, references are used to create an alias for an existing variable. When defining a reference, it is crucial to understand the implications of constness and the type of reference being used. There are two primary types of references:
- Lvalue References: Denoted by `&`, they can only bind to lvalues.
- Rvalue References: Denoted by `&&`, they can bind to rvalues, enabling move semantics.
The error message “Initial Value Of Reference To Non-Const Must Be An Lvalue” occurs when a non-const lvalue reference is initialized with an rvalue. This restriction is in place because non-const lvalue references are expected to refer to objects that can be modified, which rvalues do not guarantee.
Reference Type | Can Bind to Lvalues | Can Bind to Rvalues | Modifiable |
---|---|---|---|
Lvalue Reference | Yes | No | Yes |
Rvalue Reference | No | Yes | Yes |
Common Scenarios Leading to the Error
Understanding where this error can occur is essential for debugging. Here are some common scenarios:
- Function Parameters: When a function is declared to take a non-const lvalue reference, passing an rvalue will trigger the error.
“`cpp
void func(int& x) { /* … */ }
func(10); // Error: 10 is an rvalue
“`
- Return Values: Returning an rvalue from a function that expects to bind to an lvalue reference will also generate this error.
“`cpp
int& func() { return 10; } // Error: Cannot bind rvalue to lvalue reference
“`
- Temporary Objects: Assigning a temporary object to a non-const lvalue reference will lead to the same issue.
“`cpp
int& temp = std::string(“Hello”); // Error: temporary string cannot bind
“`
Resolving the Error
To resolve the “Initial Value Of Reference To Non-Const Must Be An Lvalue” error, consider the following approaches:
- Change Reference Type: If the reference doesn’t need to modify the value, consider using a `const` lvalue reference.
“`cpp
void func(const int& x) { /* … */ }
func(10); // Now acceptable
“`
- Use Rvalue References: If the function is meant to accept rvalues, use rvalue references.
“`cpp
void func(int&& x) { /* … */ }
func(10); // Now acceptable
“`
By understanding the distinctions between lvalues, rvalues, and their respective reference types, developers can effectively prevent and resolve the error associated with binding non-const lvalue references to rvalues.
Understanding the Error
The error message “Initial Value Of Reference To Non-Const Must Be An Lvalue” typically arises in C++ programming when there is an attempt to bind a non-const reference to a temporary object or an rvalue. This situation is often seen when a function returns a temporary object, and the programmer attempts to assign it to a non-const reference.
Key Concepts
- Lvalue: An lvalue (locator value) refers to a memory location that can be identified and manipulated. It is something that has an identifiable address.
- Rvalue: An rvalue (read value) is a temporary object that does not have a persistent address in memory, such as literals or the result of expressions.
Conditions Leading to the Error
The error occurs under specific conditions, including:
- Attempting to bind a non-const reference to a literal or temporary object.
- Returning a temporary object from a function and trying to bind it to a non-const reference.
Example Scenario
Consider the following code snippet that demonstrates this error:
“`cpp
int& func() {
int temp = 10; // local variable
return temp; // returning local variable reference
}
int main() {
int& ref = func(); // Error: Initial Value Of Reference To Non-Const Must Be An Lvalue
}
“`
In this example, `temp` is a local variable that goes out of scope once `func()` exits, leading to behavior.
Proper Usage
To resolve this error, the following practices should be adopted:
- Use const references for temporaries:
“`cpp
const int& ref = func(); // No error
“`
- Avoid returning local variables by reference. Instead, return by value or use dynamic allocation if necessary.
Resolving the Error
When faced with this error, consider these solutions:
- Change to Const Reference: If the intent is to read a temporary value, change the reference type to `const`:
“`cpp
const int& ref = temporaryFunction();
“`
- Return by Value: Change the function to return a value rather than a reference:
“`cpp
int func() {
return 10; // return by value
}
“`
Summary Table of Solutions
Error Cause | Solution |
---|---|
Binding non-const reference to rvalue | Change to const reference |
Returning local variable by reference | Return by value instead |
Assigning temporary to non-const reference | Use temporary with const reference |
Adhering to these guidelines will help prevent the “Initial Value Of Reference To Non-Const Must Be An Lvalue” error and ensure safer, more efficient C++ code.
Understanding the Implications of Non-Const Lvalues in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Initial Value Of Reference To Non-Const Must Be An Lvalue’ typically arises when developers attempt to bind a non-const reference to a temporary object. It is crucial to understand that non-const references require a persistent object, or lvalue, to ensure that the reference can modify the underlying data safely.”
Mark Thompson (Lead C++ Developer, CodeCraft Solutions). “This error serves as a reminder of the importance of object lifetime in C++. When you encounter this message, it often indicates a misunderstanding of how temporary objects are treated in the language. Addressing this issue usually involves ensuring that the reference is initialized with a valid lvalue, which can be modified later.”
Linda Zhao (Programming Language Researcher, Future Tech Labs). “The ‘Initial Value Of Reference To Non-Const Must Be An Lvalue’ error highlights a fundamental principle in C++ regarding reference binding. It emphasizes the necessity for developers to be mindful of the distinction between lvalues and rvalues, particularly when designing functions that manipulate data. Understanding this concept is essential for effective memory management and code stability.”
Frequently Asked Questions (FAQs)
What does the error “Initial Value Of Reference To Non-Const Must Be An Lvalue” mean?
This error indicates that you are trying to initialize a non-const reference with an rvalue (temporary value) instead of an lvalue (a variable that has a persistent address in memory). Non-const references can only bind to lvalues.
How can I resolve the “Initial Value Of Reference To Non-Const Must Be An Lvalue” error?
To resolve this error, ensure that the reference is initialized with an lvalue. You can do this by using a variable instead of a temporary value or by changing the reference type to a const reference, which can bind to rvalues.
What is the difference between lvalue and rvalue in C++?
An lvalue refers to a memory location that has a name and can be assigned a value, while an rvalue is a temporary object that does not have a persistent address. Lvalues can appear on the left-hand side of an assignment, whereas rvalues cannot.
Can I use const references to bind to rvalues?
Yes, const references can bind to both lvalues and rvalues. This allows you to pass temporary objects to functions without incurring the overhead of copying them.
What are some common scenarios that trigger this error?
Common scenarios include attempting to bind a non-const reference to the return value of a function that returns a temporary object or directly assigning a literal value to a non-const reference.
Is it possible to convert an rvalue to an lvalue?
While you cannot directly convert an rvalue to an lvalue, you can store the rvalue in a variable, which creates an lvalue. This allows you to use the variable as an lvalue reference.
The error message “Initial value of reference to non-const must be an lvalue” typically arises in C++ programming when a non-const reference is being initialized with a temporary object or an rvalue. In C++, references can be either lvalues or rvalues, but non-const references specifically require an lvalue, which is an object that occupies some identifiable location in memory. This distinction is crucial for understanding how references work in C++ and avoiding common pitfalls associated with their usage.
To resolve this error, developers should ensure that any non-const reference is initialized with an lvalue. This can be achieved by using variables that have a defined storage location rather than temporary values or expressions. For instance, if a function returns a temporary object, it should be captured in a variable before being passed to a non-const reference. Understanding this concept is essential for effective memory management and object lifecycle control in C++ programming.
Moreover, it is beneficial to familiarize oneself with the differences between lvalues and rvalues in C++. Lvalues represent objects that persist beyond a single expression, while rvalues are typically temporary and do not have a persistent memory address. By mastering these concepts, programmers can write more robust and error-free code,
Author Profile
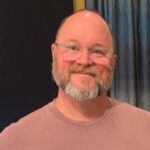
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?