How Can You Fix the Npm Err Maximum Call Stack Size Exceeded Error?
### Introduction
If you’ve ever encountered the dreaded “Npm Err Maximum Call Stack Size Exceeded” error while working on your JavaScript projects, you’re not alone. This perplexing message can halt your development process and leave you scratching your head, wondering what went wrong. As Node Package Manager (npm) continues to be a cornerstone for managing packages in the JavaScript ecosystem, understanding the nuances of its error messages is crucial for developers of all skill levels. In this article, we will unravel the mystery behind this specific error, exploring its common causes and practical solutions to help you get back on track.
### Overview
The “Maximum Call Stack Size Exceeded” error typically arises when a function calls itself recursively without a proper base case, leading to an overflow of the call stack. However, in the context of npm, this error can manifest due to various reasons, including circular dependencies in your project or issues with specific package configurations. As you dive deeper into the intricacies of npm, you’ll discover that understanding the underlying mechanics of your code and the packages you use is essential for effective debugging.
Navigating this error can be frustrating, especially when it disrupts your workflow. Fortunately, with the right knowledge and tools at your disposal, you can identify the root cause and implement effective
Npm Err Maximum Call Stack Size Exceeded: Understanding the Error
The “Maximum Call Stack Size Exceeded” error in npm typically indicates a recursion issue or an infinite loop in your code or in the dependencies you are trying to install. This can occur during various operations, such as installing packages, running scripts, or when the Node.js process exceeds the call stack limit due to excessive function calls.
Common causes of this error include:
- Cyclic Dependencies: When two or more modules depend on each other, leading to an infinite loop during resolution.
- Large Data Structures: Passing large objects or arrays into recursive functions without proper termination conditions can lead to stack overflow.
- Inefficient Code: Inefficient algorithms or poorly structured code can create unnecessary function calls.
Debugging Steps
To resolve this error, consider the following debugging steps:
- Check for Cyclic Dependencies:
- Use tools like `npm ls` to visualize the dependency tree and identify any cycles.
- Review Recursive Functions:
- Inspect your code for recursive functions and ensure that they have correct base cases to terminate properly.
- Increase Stack Size:
- You can temporarily increase the stack size by using the `–stack-size` option when running your Node.js application, though this is not a permanent solution.
- Clear npm Cache:
- Sometimes, the issue may stem from cached packages. Clear the npm cache using the following command:
bash
npm cache clean –force
- Update npm and Node.js:
- Ensure you are using the latest stable versions of npm and Node.js, as updates may resolve underlying bugs.
Example of a Recursive Function Issue
Consider the following example of a recursive function that could lead to a “Maximum Call Stack Size Exceeded” error:
javascript
function recursiveFunction() {
return recursiveFunction(); // This will call itself indefinitely.
}
In this case, the function lacks a base case to stop recursion, leading to stack overflow. A corrected version would include a base case:
javascript
function recursiveFunction(count) {
if (count <= 0) return; // Base case to terminate recursion.
recursiveFunction(count - 1); // Recursive call with decrement.
}
Preventive Measures
To avoid encountering the “Maximum Call Stack Size Exceeded” error in the future, consider implementing the following practices:
- Code Review: Regularly review code to identify potential recursion problems before they become issues.
- Testing: Write unit tests that specifically check for edge cases, including deep recursion.
- Dependency Management: Utilize tools like `npm audit` to manage and understand dependencies better.
Common Solutions Table
Solution | Description |
---|---|
Check Cyclic Dependencies | Use `npm ls` to identify cycles in the dependency tree. |
Review Recursive Functions | Ensure all recursive functions have proper base cases. |
Clear npm Cache | Run `npm cache clean –force` to clear corrupted cache. |
Update npm and Node.js | Ensure you are using the latest versions of Node.js and npm. |
Increase Stack Size | Use `–stack-size` option for temporary resolution. |
Understanding the Error
The “Maximum Call Stack Size Exceeded” error in npm (Node Package Manager) often indicates that the JavaScript engine has hit its recursion limit. This situation typically arises from:
- Infinite loops in code.
- Deeply nested function calls.
- Circular dependencies in module imports.
When the call stack exceeds its limit, the program cannot continue executing, resulting in this error. Understanding the context in which this error occurs is crucial for troubleshooting.
Common Causes
Several scenarios can lead to encountering this error while using npm:
- Circular Dependencies: When two or more modules import each other, leading to an infinite loop of imports.
- Recursive Function Calls: Functions that call themselves without a proper exit condition can quickly consume stack space.
- Large Data Structures: Operations on very large arrays or objects may also trigger this error if they involve deep iterations or recursive processing.
Troubleshooting Steps
To resolve the “Maximum Call Stack Size Exceeded” error, follow these troubleshooting steps:
- Check for Circular Dependencies:
- Use tools such as `madge` or `dependency-cruiser` to identify circular dependencies within your project.
- Refactor the code to eliminate circular imports.
- Review Recursive Functions:
- Ensure that all recursive functions have a defined base case to prevent infinite recursion.
- Limit the depth of recursion where feasible.
- Optimize Data Structures:
- If processing large data structures, consider iterative approaches instead of recursive ones.
- Use algorithms that minimize stack usage, such as tail recursion optimization.
- Increase Stack Size (last resort):
- You can increase the stack size limit by starting Node.js with the `–stack-size` flag. For example:
bash
node –stack-size=16000 your_script.js
Preventative Measures
To avoid encountering this error in the future, consider the following best practices:
- Code Reviews: Regularly conduct code reviews to catch potential infinite loops and recursion issues before they become problematic.
- Modular Design: Maintain a modular design to reduce the likelihood of circular dependencies.
- Limit Recursion Depth: Implement safeguards that limit the depth of recursive calls in critical areas of your application.
- Testing: Implement unit tests that can help identify stack overflow issues during development.
Debugging Techniques
When you encounter this error, the following debugging techniques can help identify the root cause:
- Console Logging: Insert logging statements to trace function calls and identify where the stack overflow occurs.
- Node.js Debugger: Use the built-in Node.js debugger or third-party tools like Chrome DevTools to step through your code.
- Error Stack Trace: Analyze the stack trace provided by the error message to pinpoint the location of the problem.
Addressing the “Maximum Call Stack Size Exceeded” error involves understanding its causes, implementing effective troubleshooting steps, and applying preventative measures to ensure robust application development. By following the outlined strategies, developers can enhance their code quality and avoid similar issues in the future.
Expert Insights on Npm Err Maximum Call Stack Size Exceeded
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Maximum Call Stack Size Exceeded’ error typically indicates that a function is calling itself recursively without a proper base case. This can lead to stack overflow, especially in complex applications. Developers should carefully analyze their recursive functions and consider implementing iterative solutions where feasible.”
James Liu (Lead Developer, Open Source Community). “In my experience, this error often arises from circular dependencies in module imports. It is crucial to maintain a clear architecture in your codebase to avoid such pitfalls. Using tools like dependency graph analyzers can help identify and resolve these circular references before they escalate into runtime errors.”
Sarah Thompson (Technical Writer, CodeCraft Magazine). “When encountering the ‘Maximum Call Stack Size Exceeded’ error, it is essential to review the stack trace provided by npm. This trace can offer insights into which functions are involved in the overflow, allowing developers to pinpoint the exact location of the issue and implement effective debugging strategies.”
Frequently Asked Questions (FAQs)
What does “Maximum Call Stack Size Exceeded” mean in NPM?
The “Maximum Call Stack Size Exceeded” error indicates that a function has called itself recursively too many times, exhausting the call stack. This typically occurs due to infinite loops or excessive recursion in JavaScript code.
What are common causes of the “Maximum Call Stack Size Exceeded” error?
Common causes include circular dependencies in module imports, recursive function calls without a proper base case, or large data structures being processed in a way that leads to excessive recursion depth.
How can I troubleshoot this error in my NPM project?
Start by examining the stack trace provided in the error message to identify the source of the recursion. Check for circular dependencies in your modules and ensure that recursive functions have appropriate termination conditions.
Can this error occur due to issues in third-party packages?
Yes, third-party packages can introduce circular dependencies or poorly implemented recursive functions that may lead to this error. Review the documentation or issues of the package for known problems and solutions.
What steps can I take to prevent this error in my code?
To prevent this error, ensure that recursive functions have a clear base case, avoid circular dependencies by structuring your modules carefully, and consider using iterative approaches instead of recursion for large datasets.
Is there a way to increase the call stack size in Node.js?
While it is technically possible to increase the call stack size using the `–stack-size` flag when starting Node.js, this is not a recommended solution. It is better to refactor your code to avoid deep recursion or excessive function calls.
The error message “npm ERR! Maximum call stack size exceeded” typically indicates that a recursive function or a deeply nested structure has exceeded the JavaScript engine’s call stack limit. This issue often arises during the installation of packages or when executing scripts in Node.js, particularly when there are circular dependencies or an excessive number of nested callbacks. Understanding the underlying causes of this error is crucial for developers to effectively troubleshoot and resolve it.
To address this error, developers should first examine their package.json file for any circular dependencies or misconfigurations that could lead to infinite loops during package resolution. Additionally, clearing the npm cache and updating npm to the latest version can help mitigate potential issues. It is also advisable to review the project’s structure and dependencies to ensure that they are optimized and do not introduce unnecessary complexity.
In summary, encountering the “npm ERR! Maximum call stack size exceeded” error can be frustrating, but it serves as a valuable reminder to maintain clean and efficient code practices. By proactively managing dependencies and regularly updating tools, developers can minimize the risk of running into this error and enhance the overall stability of their Node.js applications.
Author Profile
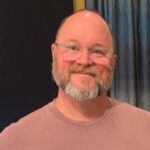
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?