How Can You Effectively Cast Variables in Python?
How To Cast In Python: A Comprehensive Guide
In the world of programming, data types are the building blocks of any application, and Python is no exception. As a dynamically typed language, Python allows developers to work with various data types seamlessly. However, there are times when you may need to convert one data type to another—this process is known as “casting.” Whether you’re manipulating strings, integers, floats, or even complex data structures, understanding how to cast in Python is essential for writing efficient and error-free code.
Casting is not just a technical necessity; it’s a fundamental skill that enhances your programming capabilities. By mastering this concept, you can ensure that your code behaves as expected, especially when performing operations that involve different data types. For instance, when you need to concatenate a string with a number, or when you want to convert user input into a specific format, knowing how to cast effectively can save you from frustrating bugs and unexpected results.
In this article, we will explore the various methods of casting in Python, including built-in functions and best practices. We’ll delve into the nuances of type conversion, highlighting common pitfalls and providing practical examples to illustrate the concepts. Whether you’re a beginner looking to solidify your understanding or an experienced programmer seeking to refine
Understanding Type Casting
Type casting in Python refers to the conversion of one data type to another. Python supports several built-in functions that facilitate this process, allowing for seamless data manipulation across various types. The most commonly used casting functions include `int()`, `float()`, and `str()`.
- int(): Converts a value to an integer.
- float(): Converts a value to a float.
- str(): Converts a value to a string.
Each of these functions can handle different types of inputs and will raise errors if the input cannot be converted. For example:
python
x = int(3.5) # Converts float to integer
y = float(“4.2”) # Converts string to float
z = str(100) # Converts integer to string
Automatic Type Casting
Python also performs automatic type casting in certain scenarios, particularly when performing operations involving mixed data types. In such cases, Python will promote the data type of the operands to avoid data loss. For example, adding an integer to a float will result in a float:
python
a = 5 # int
b = 2.5 # float
result = a + b # result will be of type float
This automatic casting helps maintain data integrity during arithmetic operations and simplifies coding.
Explicit Type Casting
Explicit type casting is when the programmer specifies the type conversion. It is crucial when you want to ensure that the data is in the correct format for processing. Here’s how to perform explicit casting:
- From String to Integer:
python
num_str = “10”
num_int = int(num_str) # num_int will be 10
- From Integer to Float:
python
num_int = 5
num_float = float(num_int) # num_float will be 5.0
- From Float to String:
python
num_float = 3.14
num_str = str(num_float) # num_str will be “3.14”
Common Type Casting Errors
Type casting can lead to various errors if not handled correctly. Below are some common issues and their causes:
Error Type | Description |
---|---|
ValueError | Raised when a conversion fails, e.g., `int(“abc”)`. |
TypeError | Occurs when an operation is applied to an inappropriate type, e.g., adding a string to an integer. |
To mitigate these errors, it is essential to validate the input before attempting to cast it. Utilizing `try` and `except` blocks can also help in gracefully handling exceptions.
Practical Examples
Here are some practical examples of type casting in Python:
python
# Example 1: Converting user input
user_input = input(“Enter a number: “)
try:
number = float(user_input) # Converts user input to float
print(f”You entered: {number}”)
except ValueError:
print(“Invalid input! Please enter a number.”)
# Example 2: List of mixed types
mixed_list = [1, 2.5, “3”, “4.8”]
converted_list = [float(i) for i in mixed_list] # Convert all elements to float
print(converted_list) # Output: [1.0, 2.5, 3.0, 4.8]
By understanding and utilizing type casting effectively, Python developers can ensure their programs handle data types more robustly and efficiently.
Type Casting in Python
Type casting in Python refers to the conversion of one data type into another. This capability allows developers to manipulate data more effectively, ensuring the appropriate type is used for various operations. Python supports several built-in functions for type casting, which are straightforward and flexible.
Built-in Type Casting Functions
Python provides several built-in functions to facilitate type casting:
- int(): Converts a value to an integer.
- float(): Converts a value to a floating-point number.
- str(): Converts a value to a string.
- list(): Converts an iterable (like a string or a tuple) to a list.
- tuple(): Converts an iterable into a tuple.
- dict(): Converts a sequence of key-value pairs into a dictionary.
Examples of Type Casting
Here are some practical examples demonstrating how to use these functions in Python:
python
# Converting a string to an integer
string_num = “42”
int_num = int(string_num)
# Converting an integer to a float
int_value = 7
float_value = float(int_value)
# Converting a float to a string
float_number = 3.14
string_float = str(float_number)
# Converting a string to a list
string_list = “Hello”
list_from_string = list(string_list)
# Converting a tuple to a list
tuple_data = (1, 2, 3)
list_from_tuple = list(tuple_data)
# Converting a list of pairs to a dictionary
pairs = [(“a”, 1), (“b”, 2)]
dictionary_from_pairs = dict(pairs)
Type Casting and Error Handling
When performing type casting, be mindful of potential errors that may arise, especially when converting between incompatible types. Below are common error scenarios:
- ValueError: Raised when trying to convert a string that does not represent a valid number to an integer or float.
Example:
python
invalid_string = “abc”
try:
int_value = int(invalid_string)
except ValueError as e:
print(f”Error: {e}”)
- TypeError: Raised when an operation is applied to an object of inappropriate type.
Type Casting with Collections
Type casting can also be applied to collections, such as lists and tuples. Here’s how to convert between different types of collections:
Original Type | Function Used | Resulting Type |
---|---|---|
List | tuple() | Tuple |
Tuple | list() | List |
String | list() | List of chars |
List of tuples | dict() | Dictionary |
Example of converting a list of tuples to a dictionary:
python
list_of_tuples = [(‘key1’, ‘value1’), (‘key2’, ‘value2’)]
converted_dict = dict(list_of_tuples)
Type Casting
Understanding type casting in Python is essential for efficient data manipulation. By utilizing the built-in functions and being aware of potential errors, developers can perform type conversions seamlessly, ensuring proper data handling in their applications.
Expert Insights on Casting in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Casting in Python is a fundamental concept that allows developers to convert data types, ensuring that operations are performed correctly. Understanding how to use functions like `int()`, `float()`, and `str()` effectively can greatly enhance code efficiency and readability.”
Michael Chen (Data Scientist, Analytics Solutions). “In Python, casting is not just about changing data types; it is also about maintaining data integrity. When working with data from various sources, proper casting helps prevent errors that could arise from type mismatches, especially in data analysis and machine learning applications.”
Laura Thompson (Python Developer, CodeCraft Academy). “Mastering casting in Python is essential for any programmer. It allows for seamless integration of different data types and is particularly useful when dealing with user input, ensuring that the data is in the expected format before processing it further.”
Frequently Asked Questions (FAQs)
What does “casting” mean in Python?
Casting in Python refers to the conversion of one data type into another. This is often necessary when performing operations that require compatible types, such as converting a string to an integer.
How do I cast a string to an integer in Python?
To cast a string to an integer, use the `int()` function. For example, `int(“123”)` converts the string “123” into the integer 123.
Can I cast a float to an integer in Python?
Yes, you can cast a float to an integer using the `int()` function. This will truncate the decimal portion. For example, `int(3.14)` results in `3`.
How can I cast an integer to a string in Python?
To cast an integer to a string, use the `str()` function. For example, `str(456)` converts the integer 456 into the string “456”.
Is it possible to cast a list to a tuple in Python?
Yes, you can cast a list to a tuple using the `tuple()` function. For instance, `tuple([1, 2, 3])` converts the list `[1, 2, 3]` into the tuple `(1, 2, 3)`.
What happens if I try to cast incompatible types in Python?
If you attempt to cast incompatible types, Python will raise a `ValueError`. For example, trying to convert the string “abc” to an integer using `int(“abc”)` will result in an error.
In summary, casting in Python is a fundamental concept that allows developers to convert one data type to another. This process is essential for ensuring that operations involving different types of data can be performed seamlessly. Python provides built-in functions such as `int()`, `float()`, and `str()` to facilitate these conversions, making it easier to manipulate and work with various data types in a dynamic manner.
Moreover, understanding how to cast data types effectively can enhance code readability and maintainability. By explicitly converting types, developers can avoid common pitfalls associated with type mismatches, which can lead to runtime errors or unintended behavior in programs. This practice not only improves the robustness of the code but also assists in debugging and optimizing performance.
mastering the art of casting in Python is crucial for any programmer looking to write efficient and error-free code. By leveraging the available casting functions and recognizing when type conversions are necessary, developers can significantly improve their coding proficiency and the overall quality of their applications.
Author Profile
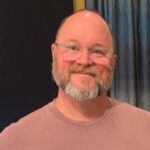
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?