Can You Really Code a Website Using Python? Exploring the Possibilities!
Can You Code A Website In Python?
In the ever-evolving landscape of web development, Python has emerged as a powerful and versatile programming language that transcends its traditional boundaries. While many may associate Python primarily with data science, artificial intelligence, or automation, its capabilities extend far beyond these realms. The question on many aspiring developers’ minds is: can you code a website in Python? The answer is a resounding yes, and the journey to creating dynamic, interactive web applications using this language is both exciting and accessible.
Python offers a plethora of frameworks and libraries designed specifically for web development, making it an ideal choice for both beginners and seasoned developers alike. From the popular Django framework, which provides a robust structure for building complex applications, to Flask, a lightweight option perfect for smaller projects, Python equips developers with the tools they need to bring their ideas to life. This article will explore the various ways you can harness Python to create stunning websites, highlighting its strengths and the resources available to help you succeed.
As we delve deeper into the world of Python web development, you’ll discover not only the technical aspects of coding a website but also the community and ecosystem that supports this vibrant language. Whether you’re looking to build a personal blog, an e-commerce platform, or a sophisticated
Frameworks for Web Development in Python
Python offers a variety of frameworks that simplify the web development process. These frameworks provide pre-built components, tools, and libraries that enable developers to create robust applications efficiently. The most popular frameworks include:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It follows the “batteries-included” philosophy, offering a wide range of features out of the box, including an ORM (Object-Relational Mapping), an admin panel, and authentication mechanisms.
- Flask: A micro-framework that is lightweight and modular, allowing for greater flexibility. Flask is ideal for smaller applications or those that require custom components without the overhead of a larger framework.
- FastAPI: This modern framework is designed for building APIs quickly and efficiently. It leverages Python type hints, providing automatic validation and documentation generation. FastAPI is particularly well-suited for asynchronous programming.
Each framework has its unique strengths, and the choice depends on the project’s requirements and the developer’s familiarity with the tools.
Setting Up a Basic Web Application with Flask
To demonstrate how to code a simple web application in Python, we will use Flask. The steps below outline the process:
- Install Flask: First, ensure that you have Python installed. Then, install Flask using pip:
“`
pip install Flask
“`
- Create a Basic Application: Create a new Python file (e.g., `app.py`) and add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the Application: In your terminal, navigate to the directory containing `app.py` and run:
“`
python app.py
“`
- Access the Application: Open your web browser and go to `http://127.0.0.1:5000/` to see your application in action.
This basic setup illustrates how easy it is to create a web application using Python and Flask.
Database Integration in Python Web Applications
Integrating a database into your Python web application is essential for data persistence. Common choices for databases include:
- SQLite: A lightweight, file-based database suitable for small to medium applications.
- PostgreSQL: A powerful, open-source object-relational database system ideal for larger applications.
- MySQL: A widely-used relational database management system known for its reliability.
Here’s a simple table comparing these databases:
Database | Type | Use Case | Scalability |
---|---|---|---|
SQLite | File-based | Small applications | Low |
PostgreSQL | Relational | Medium to large applications | High |
MySQL | Relational | Web applications | High |
To integrate a database in a Flask application, you can use SQLAlchemy, a powerful ORM that provides a high-level abstraction for database operations. Here’s a brief example of how to set it up:
- Install SQLAlchemy:
“`
pip install Flask-SQLAlchemy
“`
- Configure the Database in your `app.py`:
“`python
from flask_sqlalchemy import SQLAlchemy
app.config[‘SQLALCHEMY_DATABASE_URI’] = ‘sqlite:///site.db’
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(150), nullable=)
db.create_all()
“`
This code sets up a basic user model and creates the necessary database tables. You can now expand your application by adding more models and functionality as needed.
Frameworks for Web Development in Python
Python offers several robust frameworks that facilitate web development, each catering to different needs and complexity levels. Here are some popular frameworks:
- Django: A high-level framework that promotes rapid development and clean, pragmatic design. It includes built-in features such as an ORM, authentication, and an admin panel.
- Flask: A micro-framework that is lightweight and easy to extend. It is ideal for smaller applications or when you want more control over components.
- FastAPI: Designed for building APIs with automatic generation of OpenAPI documentation. It is asynchronous and suitable for high-performance applications.
- Pyramid: A flexible framework that can scale from small applications to large ones. It allows developers to choose the components they need.
Setting Up a Basic Web Application with Flask
To illustrate how to code a website using Python, consider a simple Flask application. Below are the essential steps:
- Install Flask:
“`bash
pip install Flask
“`
- Create a Simple App:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the Application:
Execute the script in your terminal:
“`bash
python your_flask_app.py
“`
- Access the Webpage:
Open your web browser and go to `http://127.0.0.1:5000/` to see the output.
Database Integration
Integrating a database into a Python web application is a common requirement. Django has a built-in ORM, while Flask can use SQLAlchemy or other ORM libraries. Below is a brief overview of setting up SQLAlchemy with Flask:
- Install SQLAlchemy:
“`bash
pip install Flask-SQLAlchemy
“`
- Configure the Database:
“`python
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config[‘SQLALCHEMY_DATABASE_URI’] = ‘sqlite:///test.db’
db = SQLAlchemy(app)
“`
- Create a Model:
“`python
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=)
def __repr__(self):
return f’
“`
- Create the Database:
“`python
with app.app_context():
db.create_all()
“`
Deployment Options
Once the application is developed, deployment is the next crucial step. Common deployment options for Python web applications include:
Deployment Method | Description |
---|---|
Heroku | A cloud platform that supports Python and provides a straightforward deployment process. |
AWS Elastic Beanstalk | A service for deploying and scaling applications automatically, supporting various languages including Python. |
DigitalOcean | Offers scalable compute instances where you can set up your application manually. |
PythonAnywhere | A platform specifically designed for hosting Python applications, providing easy deployment options. |
Utilizing version control systems such as Git and continuous integration/continuous deployment (CI/CD) tools can further streamline the deployment process and improve collaboration.
Best Practices for Python Web Development
To ensure a maintainable and scalable web application, adhere to the following best practices:
- Code Structure: Organize your code using a consistent structure, such as separating routes, models, and services into different modules.
- Environment Management: Use virtual environments to manage dependencies and avoid conflicts.
- Security: Implement security measures, such as input validation, SQL injection prevention, and proper session management.
- Testing: Write unit and integration tests to ensure your application behaves as expected.
- Documentation: Maintain clear documentation for your code and API endpoints to facilitate collaboration and onboarding of new developers.
By following these guidelines, developers can create efficient, secure, and scalable web applications using Python.
Can You Build a Website Using Python? Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Yes, you can code a website in Python using frameworks like Django and Flask. These frameworks provide robust tools for web development, making it easier to manage databases, user authentication, and overall application structure.”
Michael Chen (Lead Web Developer, Creative Solutions Agency). “While Python is not traditionally associated with front-end development, it excels in back-end services. By utilizing Python for server-side logic, developers can create dynamic web applications that interact seamlessly with front-end technologies.”
Sarah Thompson (Full-Stack Developer, Future Tech Labs). “The versatility of Python allows for rapid development of web applications. With libraries like Flask for microservices or Django for full-fledged applications, Python enables developers to create scalable and maintainable websites efficiently.”
Frequently Asked Questions (FAQs)
Can you code a website in Python?
Yes, you can code a website in Python using web frameworks such as Django and Flask. These frameworks provide tools and libraries that simplify the development process.
What are the advantages of using Python for web development?
Python offers readability, simplicity, and a vast ecosystem of libraries. Its frameworks facilitate rapid development, making it a popular choice for both beginners and experienced developers.
Is Python suitable for both frontend and backend development?
Python is primarily used for backend development. For frontend development, technologies like HTML, CSS, and JavaScript are typically employed. However, Python can interact with frontend code through APIs.
What frameworks are commonly used for building websites in Python?
Django and Flask are the most popular frameworks. Django is a high-level framework that promotes rapid development, while Flask is lightweight and offers more flexibility for smaller applications.
Can Python be used for creating dynamic websites?
Yes, Python can be used to create dynamic websites. By utilizing frameworks like Django or Flask, developers can build applications that respond to user input and display dynamic content.
Are there any limitations to using Python for web development?
While Python is powerful, it may not be the best choice for performance-intensive applications. Additionally, the learning curve for some frameworks can be steep for beginners compared to other languages.
coding a website in Python is not only feasible but also increasingly popular due to the language’s versatility and the robust frameworks available. Python offers several web development frameworks, such as Django and Flask, which simplify the process of building and deploying web applications. These frameworks provide built-in functionalities that streamline development, allowing developers to focus on creating dynamic and interactive user experiences.
Moreover, Python’s readability and ease of use make it an excellent choice for both beginners and experienced developers. The extensive libraries and community support further enhance its capabilities, enabling developers to integrate various functionalities such as database management, user authentication, and API development seamlessly. This makes Python a strong contender in the web development landscape, rivaling traditional languages like JavaScript and PHP.
In summary, coding a website in Python is a practical and effective approach for developers looking to leverage the language’s strengths. With the right frameworks and tools, developers can create robust, scalable, and maintainable web applications. As the demand for Python in web development continues to grow, it is essential for developers to stay updated on best practices and emerging trends within this domain.
Author Profile
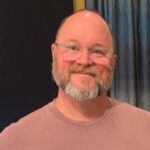
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?