How Can You Effectively Sort an Array in JavaScript?
Sorting arrays is a fundamental task in programming, and in JavaScript, it’s both an essential skill and a powerful tool. Whether you’re organizing a list of names, arranging numbers in ascending order, or preparing data for display, knowing how to sort an array efficiently can enhance your coding prowess and streamline your applications. With JavaScript’s built-in methods and the flexibility of its syntax, sorting arrays can be both straightforward and customizable, allowing you to tackle a variety of challenges with ease.
In this article, we’ll delve into the various techniques available for sorting arrays in JavaScript, exploring the built-in `sort()` method and its capabilities. We’ll also discuss how to create custom sorting functions to meet specific requirements, ensuring that you can handle any data structure you encounter. From simple numeric sorts to more complex string comparisons, understanding the nuances of array sorting will empower you to manipulate data effectively and improve your overall coding efficiency.
As we navigate through the intricacies of array sorting, you’ll discover best practices, common pitfalls, and performance considerations that will help you write cleaner, more efficient code. So, whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this guide will equip you with the knowledge you need to master array sorting in JavaScript.
Sorting Arrays Using Built-in Methods
JavaScript provides a built-in method called `sort()` that can be used to sort arrays. By default, the `sort()` method sorts the elements as strings in alphabetical and ascending order. However, it can also take a comparison function as an argument, which allows for more complex sorting mechanisms.
To sort an array of numbers in ascending order, you can use the following example:
“`javascript
let numbers = [10, 2, 5, 3];
numbers.sort((a, b) => a – b);
console.log(numbers); // Output: [2, 3, 5, 10]
“`
When using the `sort()` method, it is crucial to provide a comparison function for numerical sorting because, without it, the method will convert numbers to strings and sort them accordingly. For example:
“`javascript
let numbers = [10, 2, 5, 3];
numbers.sort(); // Output: [10, 2, 3, 5] (incorrect numerical order)
“`
Sorting in Descending Order
To sort an array in descending order, you can modify the comparison function slightly. Here’s how you can achieve this:
“`javascript
let numbers = [10, 2, 5, 3];
numbers.sort((a, b) => b – a);
console.log(numbers); // Output: [10, 5, 3, 2]
“`
This function subtracts `b` from `a`, reversing the order of the sort.
Sorting Arrays of Objects
When dealing with arrays of objects, the `sort()` method can be utilized in conjunction with a comparison function that specifies how to compare the objects. For example, consider an array of objects representing people, where you want to sort them by age:
“`javascript
let people = [
{ name: ‘Alice’, age: 25 },
{ name: ‘Bob’, age: 30 },
{ name: ‘Charlie’, age: 20 }
];
people.sort((a, b) => a.age – b.age);
console.log(people);
“`
This will sort the array of people in ascending order based on their ages.
Custom Sorting Logic
You may also require more complex sorting logic based on multiple criteria. For instance, if you want to sort by name and then by age, you can implement a comparison function as follows:
“`javascript
people.sort((a, b) => {
if (a.name < b.name) return -1;
if (a.name > b.name) return 1;
return a.age – b.age; // Sort by age if names are equal
});
“`
Comparative Overview of Sorting Methods
The following table summarizes the different sorting methods and their use cases:
Sorting Method | Description | Use Case |
---|---|---|
sort() | Sorts elements in place. | Basic sorting of strings and numbers. |
sort((a, b) => a – b) | Sorts numbers in ascending order. | Numerical sorting. |
sort((a, b) => b – a) | Sorts numbers in descending order. | Reverse numerical sorting. |
sort((a, b) => a.property – b.property) | Sorts objects based on a property. | Sorting arrays of objects. |
Utilizing the `sort()` method effectively can greatly enhance the data manipulation capabilities within your JavaScript applications.
Sorting an Array Using the `sort()` Method
The `sort()` method is a built-in function in JavaScript that sorts the elements of an array in place. By default, it sorts elements as strings in ascending order, which may not yield the expected results for numerical values.
“`javascript
let numbers = [10, 2, 5, 1, 8];
numbers.sort(); // Sorts as strings: [1, 10, 2, 5, 8]
“`
To sort numbers correctly, you need to provide a comparison function to `sort()`:
“`javascript
numbers.sort((a, b) => a – b); // Sorts numerically: [1, 2, 5, 8, 10]
“`
Comparison Function
The comparison function takes two arguments and should return:
- A negative value if the first argument is less than the second.
- Zero if they are equal.
- A positive value if the first argument is greater.
Example: Sorting Strings
“`javascript
let fruits = [‘banana’, ‘apple’, ‘cherry’];
fruits.sort(); // Sorts alphabetically: [‘apple’, ‘banana’, ‘cherry’]
“`
Sorting in Descending Order
To sort an array in descending order, simply reverse the return values of the comparison function:
“`javascript
numbers.sort((a, b) => b – a); // Sorts numerically in descending order: [10, 8, 5, 2, 1]
“`
Example: Descending String Sort
“`javascript
fruits.sort((a, b) => b.localeCompare(a)); // Sorts in reverse alphabetical order: [‘cherry’, ‘banana’, ‘apple’]
“`
Sorting Objects in an Array
When dealing with an array of objects, the `sort()` method can still be used by specifying which property to sort on.
Example: Sorting by Object Property
“`javascript
let people = [
{ name: ‘John’, age: 25 },
{ name: ‘Jane’, age: 30 },
{ name: ‘Doe’, age: 20 }
];
people.sort((a, b) => a.age – b.age); // Sorts by age: [{ name: ‘Doe’, age: 20 }, { name: ‘John’, age: 25 }, { name: ‘Jane’, age: 30 }]
“`
Sorting by Multiple Properties
When sorting by multiple properties, you can chain comparisons:
“`javascript
people.sort((a, b) => {
if (a.age === b.age) {
return a.name.localeCompare(b.name); // Sorts by name if ages are equal
}
return a.age – b.age; // Sorts by age
});
“`
Custom Sort Functions
You can create more complex sorting logic by defining custom functions. For example, to sort by length of the string:
Example: Sorting by String Length
“`javascript
let words = [‘apple’, ‘banana’, ‘fig’, ‘grapefruit’];
words.sort((a, b) => a.length – b.length); // Sorts by length: [‘fig’, ‘apple’, ‘banana’, ‘grapefruit’]
“`
Use Cases for Custom Sorting
- Sorting dates.
- Sorting by multiple criteria.
- Implementing complex sorting algorithms.
Stability of Sort
The `sort()` method is not guaranteed to be stable in all JavaScript engines. A stable sort maintains the relative order of records with equal keys. For guaranteed stability, consider using alternative sorting libraries or implementing a stable sorting algorithm, such as Merge Sort.
Example of a Stable Sort Implementation
You could implement a stable sorting algorithm if necessary:
“`javascript
function stableSort(array, compareFn) {
return array.map((value, index) => ({ value, index }))
.sort((a, b) => {
const order = compareFn(a.value, b.value);
return order !== 0 ? order : a.index – b.index; // Maintain original order for equal elements
})
.map(({ value }) => value);
}
“`
This function allows for stable sorting while using any comparison logic specified.
Expert Insights on Sorting Arrays in JavaScript
Dr. Emily Carter (Lead Software Engineer, Code Innovators Inc.). “When sorting an array in JavaScript, utilizing the built-in `sort()` method is essential. However, developers must remember that this method sorts elements as strings by default. To achieve numerical sorting, it is crucial to provide a comparison function that accurately reflects the desired order.”
Michael Chen (JavaScript Developer Advocate, Tech Solutions Group). “For optimal performance when sorting large datasets, consider using algorithms like QuickSort or MergeSort. While JavaScript’s native `sort()` is efficient for many use cases, understanding the underlying algorithm can help developers make informed decisions about performance and scalability.”
Sarah Patel (Senior Frontend Developer, Creative Web Agency). “Sorting arrays in JavaScript can also be enhanced by leveraging the `localeCompare()` method for string arrays. This method allows for locale-aware sorting, which is particularly beneficial when dealing with internationalization and ensuring that strings are sorted according to cultural norms.”
Frequently Asked Questions (FAQs)
How do I sort an array of numbers in ascending order in JavaScript?
You can sort an array of numbers in ascending order by using the `sort()` method with a comparison function: `array.sort((a, b) => a – b);`. This ensures numerical sorting rather than lexicographical sorting.
Can I sort an array of strings in JavaScript?
Yes, you can sort an array of strings using the `sort()` method. By default, it sorts strings in lexicographical (dictionary) order: `array.sort();`. You can provide a custom comparison function for case-insensitive sorting.
What is the difference between `sort()` and `reverse()` methods in JavaScript?
The `sort()` method arranges the elements of an array according to a specified order, while the `reverse()` method simply reverses the order of the elements in the array. They serve different purposes in array manipulation.
How can I sort an array of objects by a specific property?
To sort an array of objects by a specific property, use the `sort()` method with a comparison function that accesses the property: `array.sort((a, b) => a.propertyName – b.propertyName);` for numerical properties or `array.sort((a, b) => a.propertyName.localeCompare(b.propertyName));` for string properties.
Is the `sort()` method stable in JavaScript?
The stability of the `sort()` method in JavaScript is implementation-dependent. While some environments implement a stable sort, others may not guarantee that the relative order of equal elements will be preserved.
Can I sort an array in descending order in JavaScript?
Yes, to sort an array in descending order, you can modify the comparison function in the `sort()` method: `array.sort((a, b) => b – a);` for numbers or `array.sort((a, b) => b.propertyName.localeCompare(a.propertyName));` for strings.
Sorting an array in JavaScript can be accomplished using the built-in `sort()` method, which is a powerful tool for organizing data in ascending or descending order. The `sort()` method converts elements to strings by default, which can lead to unexpected results when dealing with numbers. Therefore, it is essential to provide a comparison function to ensure accurate numerical sorting. This function dictates how elements are compared, allowing for customized sorting behavior based on specific criteria.
In addition to the default sorting behavior, JavaScript offers various techniques for sorting arrays, including the use of the `localeCompare()` method for string sorting and the `reverse()` method to invert the order of sorted arrays. Understanding these methods enhances the developer’s ability to manipulate and present data effectively. Furthermore, when dealing with large datasets, it is crucial to consider the performance implications of different sorting algorithms, as the efficiency of the sorting process can significantly impact application performance.
Overall, mastering array sorting in JavaScript is a fundamental skill for developers. It not only improves data handling but also enhances user experience by presenting information in a logical order. By leveraging the built-in methods and understanding how to implement custom sorting functions, developers can achieve precise control over how data is organized and displayed in their applications
Author Profile
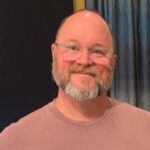
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?