Why Am I Encountering a TypeError: ‘Float’ Object Is Not Subscriptable in My Code?
In the world of programming, encountering errors is an inevitable part of the learning process, and one of the more perplexing issues developers face is the `TypeError: ‘float’ object is not subscriptable`. This error can halt your code in its tracks, leaving you scratching your head and wondering where it all went wrong. Whether you’re a seasoned coder or just starting your journey in programming, understanding the nuances of this error can save you time and frustration. In this article, we will delve into the intricacies of this common Python error, exploring its causes, implications, and how to effectively troubleshoot it.
Overview
At its core, the `TypeError: ‘float’ object is not subscriptable` error arises when a programmer attempts to access an index or key of a float object as if it were a list, tuple, or dictionary. This type of error often occurs in scenarios where the data type is mismanaged or misunderstood, leading to attempts to index into a value that simply cannot be indexed. For example, if you mistakenly treat a single floating-point number as a collection, the Python interpreter will promptly notify you of your oversight.
Understanding the context in which this error can occur is crucial for effective debugging. It often surfaces in data manipulation tasks
Understanding the Error
The error message `TypeError: ‘float’ object is not subscriptable` typically arises in Python when attempting to index or slice a float object as if it were a sequence type, such as a list or a string. In Python, only certain data types can be indexed or subscripted, and floats are not one of them. This error indicates a misuse of types, suggesting that the programmer is trying to access a specific part of a float, which is inherently a single numeric value.
Common Scenarios Leading to This Error
Several programming scenarios can lead to this error:
- Incorrect Variable Type: Assigning a float value to a variable that is later expected to be a list or string.
- Function Return Values: Functions that are expected to return a list or string but inadvertently return a float.
- Mathematical Operations: Performing mathematical operations that result in a float, followed by an attempt to index into that result.
Example Cases
To illustrate how this error can occur, consider the following examples:
“`python
Example 1: Incorrect indexing
number = 3.14
result = number[0] Raises TypeError
“`
In this case, the variable `number` is a float, and attempting to access its first element results in a `TypeError`.
“`python
Example 2: Function returning a float
def calculate_area(radius):
return 3.14 * radius ** 2
area = calculate_area(5)
print(area[0]) Raises TypeError
“`
Here, the function `calculate_area` returns a float, and the attempt to index it leads to the error.
Preventing the Error
To prevent encountering the `TypeError: ‘float’ object is not subscriptable`, consider the following strategies:
- Type Checking: Before performing indexing operations, confirm the variable is of a suitable type.
- Debugging: Use print statements or debugging tools to inspect variable types during development.
- Refactor Code: If a function is expected to return a sequence type, ensure it does so consistently.
Handling the Error
If you encounter this error in your code, follow these steps to diagnose and resolve the issue:
- Identify the Line Causing the Error: Check the traceback message to locate the specific line.
- Check Variable Types: Use the `type()` function to confirm the data type of the variable before the subscript operation.
- Modify Code Accordingly: Update the code to ensure that only subscriptable types are indexed.
Type | Subscriptable |
---|---|
List | Yes |
String | Yes |
Float | No |
Dictionary | Yes |
By adhering to these best practices, you can minimize the occurrence of this error and enhance the reliability of your code.
Understanding the Error
The `TypeError: ‘float’ object is not subscriptable` occurs in Python when you attempt to access elements of a float as if it were a list or another subscriptable type. This error typically arises from a misunderstanding of data types, particularly when working with numerical values.
- Subscriptable types include lists, tuples, strings, and dictionaries.
- Non-subscriptable types include integers and floats.
When you try to access an index of a float, Python raises this error because it does not support such operations.
Common Scenarios Leading to the Error
Several common coding patterns can inadvertently lead to this error:
- Incorrect Data Type: Assigning a float value to a variable that is later treated as a list or string.
“`python
x = 3.14
print(x[0]) Raises TypeError
“`
- Function Return Values: Functions that return a float instead of a list can cause this issue when their output is accessed incorrectly.
“`python
def calculate_average(numbers):
return sum(numbers) / len(numbers)
avg = calculate_average([1, 2, 3])
print(avg[0]) Raises TypeError
“`
- Indexing Mistakes: Misunderstanding the structure of data when accessing nested elements.
“`python
data = {‘value’: 4.5}
print(data[‘value’][0]) Raises TypeError
“`
How to Fix the Error
To resolve the `TypeError: ‘float’ object is not subscriptable`, consider the following approaches:
- Check Variable Types: Use `type()` to confirm the expected data types before performing subscript operations.
“`python
x = 3.14
print(type(x)) Use this to debug
“`
- Ensure Correct Data Structure: Validate that the variable you are trying to index is indeed a list or similar structure. If it is a float, revise your logic to avoid indexing.
- Modify Function Outputs: If a function is returning a float, ensure you handle the output correctly. For example, if you need the output in a list format:
“`python
avg = [calculate_average([1, 2, 3])] Wrap in a list
print(avg[0]) Now this works
“`
Example Code Snippet
Here’s an example demonstrating both the error and its correction:
“`python
Erroneous code
float_value = 5.67
try:
print(float_value[0]) This raises TypeError
except TypeError as e:
print(f”Error: {e}”)
Corrected code
float_value = 5.67
float_as_list = [float_value] Wrapping float in a list
print(float_as_list[0]) This works fine
“`
Preventive Measures
To minimize the chances of encountering this error in the future, consider the following practices:
- Type Annotations: Use type hints in your functions to clarify expected input and output types.
- Comprehensive Testing: Implement unit tests to catch type-related issues early in development.
- Code Reviews: Regularly review code with peers to identify potential type misuse.
By adhering to these practices, you can significantly reduce the likelihood of encountering the `TypeError: ‘float’ object is not subscriptable`.
Understanding the ‘Float’ Object Is Not Subscriptable Error in Python
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “The ‘TypeError: ‘float’ object is not subscriptable’ typically arises when a programmer attempts to access an index of a float variable as if it were a list or a dictionary. This error serves as a reminder to ensure that the data types being manipulated align with the intended operations.”
Michael Thompson (Python Developer, Tech Innovations). “This error often occurs when developers mistakenly treat a numerical value as a collection. To resolve it, one should check the variable type before performing indexing operations, ensuring that the variable is indeed a list or similar structure.”
Sarah Lee (Data Scientist, Analytics Hub). “In data processing, encountering a ‘float’ object as subscriptable can indicate a flaw in data handling or transformation. It is crucial to validate data types at every step in the pipeline to prevent such issues from disrupting analysis workflows.”
Frequently Asked Questions (FAQs)
What does the error ‘TypeError: ‘float’ object is not subscriptable’ mean?
This error indicates that you are attempting to access an index or key on a float object, which is not a valid operation since floats are not iterable.
What are common scenarios that trigger this error?
This error often occurs when you mistakenly treat a float variable as a list or dictionary, such as trying to access an element using square brackets (e.g., `float_variable[0]`).
How can I fix the ‘TypeError: ‘float’ object is not subscriptable’ error?
To resolve this error, ensure that you are only using subscripting on iterable objects like lists or dictionaries. Verify the variable type before attempting to access it via an index.
What types of objects are subscriptable in Python?
Subscriptable objects in Python include lists, tuples, strings, dictionaries, and sets. These objects can be indexed or accessed using square brackets.
Can this error occur in a loop or function?
Yes, this error can occur within loops or functions if a float is inadvertently passed or returned when an iterable is expected. Always check the data types being processed.
How can I debug this error effectively?
To debug, use print statements to check the type and value of the variable before the line causing the error. Employ tools like type hints or assertions to ensure the expected data types are maintained throughout your code.
The TypeError ‘float’ object is not subscriptable is a common error encountered in Python programming. This error occurs when a programmer attempts to access an index or slice of a float object, which is inherently not a subscriptable type. In Python, subscriptable types include lists, tuples, strings, and dictionaries, among others. Understanding the nature of data types and their properties is essential for effective debugging and code development.
One of the primary causes of this error is the confusion between different data types, particularly when working with collections or mathematical operations. For instance, if a float is mistakenly treated as a list or if a function is expected to return a list but instead returns a float, this can lead to the TypeError. It is crucial for developers to ensure that they are manipulating the correct data types and to implement proper type checking where necessary.
To avoid encountering this error, programmers should adopt best practices such as thorough testing and validation of data types before performing operations. Utilizing debugging tools and techniques can also help identify the source of the issue more quickly. Additionally, being mindful of the return types of functions and ensuring that they align with the expected data types can significantly reduce the likelihood of encountering this error in future coding endeavors.
Author Profile
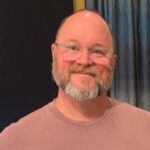
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?