How Can You Create a Stopwatch Using JavaScript?
In our fast-paced world, timing is everything. Whether you’re a fitness enthusiast tracking your workout intervals, a student timing your study sessions, or a developer looking to enhance your web applications, having a reliable stopwatch at your fingertips can make all the difference. With the power of JavaScript, you can create a functional and visually appealing stopwatch that not only serves its purpose but also enhances your coding skills. In this article, we will guide you through the process of building a stopwatch from scratch, empowering you to harness the capabilities of JavaScript while creating a useful tool.
Creating a stopwatch using JavaScript is an excellent way to dive into the world of web development. This project allows you to explore fundamental concepts such as event handling, timers, and DOM manipulation. As you embark on this journey, you’ll learn how to build a user interface that responds to user inputs, displays elapsed time, and even allows for lap functionality. By the end of the article, you’ll have a fully functional stopwatch that you can customize and expand upon.
Not only is this project a great to JavaScript programming, but it also provides a practical application that can be integrated into various web projects. Whether you’re looking to enhance your portfolio or simply want to create a handy tool for personal use, learning how
HTML Structure for Stopwatch
To create a functional stopwatch, you first need to establish an appropriate HTML structure. This layout will include buttons for starting, stopping, and resetting the stopwatch, as well as an area to display the elapsed time.
“`html
“`
The `div` with `id=”display”` will present the time, while the buttons will control the stopwatch’s functionality.
JavaScript Logic for Stopwatch Functionality
Now that you have the HTML in place, the next step is to implement the JavaScript logic. This script will manage the timer’s functionality, including starting, stopping, and resetting the stopwatch.
“`javascript
let startTime;
let updatedTime;
let difference;
let tInterval;
let running = ;
function startTimer() {
if (!running) {
startTime = new Date().getTime();
tInterval = setInterval(getShowTime, 1);
running = true;
}
}
function stopTimer() {
clearInterval(tInterval);
running = ;
}
function resetTimer() {
clearInterval(tInterval);
running = ;
difference = 0;
document.getElementById(“display”).innerHTML = “00:00:00”;
}
function getShowTime() {
updatedTime = new Date().getTime();
difference = updatedTime – startTime;
const hours = Math.floor((difference % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((difference % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((difference % (1000 * 60)) / 1000);
document.getElementById(“display”).innerHTML =
(hours < 10 ? "0" + hours : hours) + ":" +
(minutes < 10 ? "0" + minutes : minutes) + ":" +
(seconds < 10 ? "0" + seconds : seconds);
}
```
In this code, the `startTimer`, `stopTimer`, and `resetTimer` functions manage the stopwatch's state. The `getShowTime` function calculates the elapsed time and formats it for display.
Connecting HTML and JavaScript
To ensure your stopwatch functions correctly, connect your HTML buttons to their respective JavaScript functions. This can be done by adding event listeners in your script.
“`javascript
document.getElementById(“startButton”).addEventListener(“click”, startTimer);
document.getElementById(“stopButton”).addEventListener(“click”, stopTimer);
document.getElementById(“resetButton”).addEventListener(“click”, resetTimer);
“`
This code snippet ensures that clicking each button will trigger the corresponding function, allowing users to control the stopwatch effectively.
CSS Styling for Stopwatch
To enhance the visual appeal of your stopwatch, you can apply some basic CSS styles. Below is an example of how to style the stopwatch.
“`css
stopwatch {
text-align: center;
font-family: Arial, sans-serif;
margin-top: 50px;
}
display {
font-size: 48px;
margin-bottom: 20px;
}
button {
padding: 10px 20px;
font-size: 16px;
margin: 5px;
cursor: pointer;
}
“`
This CSS will center the stopwatch, increase the font size of the display, and add some padding to the buttons for better usability.
Function | Description |
---|---|
startTimer() | Initiates the stopwatch and begins tracking time. |
stopTimer() | Pauses the stopwatch, preserving the elapsed time. |
resetTimer() | Resets the stopwatch to zero and stops any ongoing timing. |
Understanding the Components of a Stopwatch
Creating a stopwatch using JavaScript involves several key components, including the timer, display, and control buttons. Each of these plays a crucial role in the functionality of the stopwatch.
- Timer: The timer keeps track of elapsed time using JavaScript’s `setInterval` function.
- Display: The display shows the current time in a readable format (e.g., minutes, seconds, milliseconds).
- Control Buttons: Typically includes start, stop, and reset buttons to manage the stopwatch functionality.
Setting Up the HTML Structure
To begin, create a basic HTML structure to house the stopwatch elements. The following code snippet demonstrates a simple layout:
“`html
“`
This structure contains a display area and three buttons for user interaction.
Implementing the Stopwatch Functionality
Next, implement the JavaScript to bring the stopwatch to life. The following code outlines the main functions required:
“`javascript
let startTime, updatedTime, difference, tInterval;
let running = ;
function startTimer() {
if (!running) {
startTime = new Date().getTime() – difference;
tInterval = setInterval(updateTime, 10);
running = true;
}
}
function stopTimer() {
clearInterval(tInterval);
running = ;
}
function resetTimer() {
clearInterval(tInterval);
difference = 0;
running = ;
document.getElementById(‘display’).innerHTML = “00:00:00”;
}
function updateTime() {
updatedTime = new Date().getTime();
difference = updatedTime – startTime;
const hours = Math.floor((difference % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((difference % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((difference % (1000 * 60)) / 1000);
const milliseconds = Math.floor((difference % 1000) / 10);
document.getElementById(‘display’).innerHTML =
(hours < 10 ? "0" + hours : hours) + ":" +
(minutes < 10 ? "0" + minutes : minutes) + ":" +
(seconds < 10 ? "0" + seconds : seconds) + "." +
(milliseconds < 10 ? "0" + milliseconds : milliseconds);
}
```
Connecting JavaScript with HTML
To ensure the buttons trigger the appropriate functions, add event listeners in your JavaScript code:
“`javascript
document.getElementById(‘start’).addEventListener(‘click’, startTimer);
document.getElementById(‘stop’).addEventListener(‘click’, stopTimer);
document.getElementById(‘reset’).addEventListener(‘click’, resetTimer);
“`
This code connects the buttons to their respective functions, allowing users to start, stop, and reset the stopwatch seamlessly.
Styling the Stopwatch
To improve the visual appeal of the stopwatch, basic CSS can be applied. Consider the following styles:
“`css
stopwatch {
text-align: center;
font-family: Arial, sans-serif;
}
display {
font-size: 2em;
margin: 20px 0;
}
button {
margin: 5px;
padding: 10px 15px;
font-size: 1em;
cursor: pointer;
}
“`
These styles enhance the layout, ensuring it is user-friendly and visually engaging.
Testing the Stopwatch
Once the implementation is complete, thoroughly test all functionalities:
- Start the stopwatch and check if the time displays correctly.
- Stop the stopwatch and ensure the timer pauses accurately.
- Reset the stopwatch and verify it returns to zero without errors.
Through careful testing, you can confirm that your stopwatch works as intended, providing a reliable tool for time tracking.
Expert Insights on Creating a Stopwatch Using JavaScript
Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “Creating a stopwatch using JavaScript is an excellent way to understand the fundamentals of timers and event handling. By leveraging the `setInterval` function, developers can create a responsive and accurate stopwatch that enhances user interaction.”
James Liu (Front-End Engineer, CodeCraft Studio). “When designing a stopwatch, it’s crucial to consider user experience. Implementing features like pause, reset, and lap functionality using JavaScript not only makes the stopwatch more versatile but also provides a more engaging experience for users.”
Sophia Martinez (Web Development Instructor, Digital Academy). “Teaching how to create a stopwatch with JavaScript is a fantastic project for beginners. It introduces key programming concepts such as functions, variables, and the DOM, while also allowing for creativity in design and functionality.”
Frequently Asked Questions (FAQs)
What are the basic components needed to create a stopwatch using JavaScript?
To create a stopwatch using JavaScript, you will need HTML for the structure, CSS for styling, and JavaScript for functionality. The primary components include buttons for start, stop, and reset, as well as a display area for the elapsed time.
How can I implement the start and stop functionality in my JavaScript stopwatch?
You can implement the start functionality by using `setInterval` to update the timer every second. For the stop functionality, use `clearInterval` to halt the timer. Ensure to maintain a variable to track the elapsed time and update the display accordingly.
What is the best way to format the elapsed time in a JavaScript stopwatch?
The elapsed time can be formatted by converting the total seconds into hours, minutes, and seconds. Use the modulus operator to calculate each component and ensure to pad single-digit numbers with a leading zero for better readability.
How can I ensure that my stopwatch works accurately across different browsers?
To ensure accuracy across different browsers, use `Date.now()` or `performance.now()` for high-resolution timing. Avoid relying solely on `setTimeout` or `setInterval`, as they may not provide consistent timing due to varying execution delays.
Is it possible to add lap functionality to a JavaScript stopwatch?
Yes, you can add lap functionality by storing the elapsed time at the moment the lap button is pressed. Create an array to hold lap times and display them in a designated area on the user interface.
How can I make my JavaScript stopwatch responsive for mobile devices?
To make your JavaScript stopwatch responsive, use CSS media queries to adjust the layout for different screen sizes. Ensure buttons are easily clickable and the display is legible on smaller screens. Additionally, consider using flexible units like percentages or viewport units for sizing.
creating a stopwatch using JavaScript involves understanding the fundamental components of time manipulation and user interface interaction. The process typically includes setting up a simple HTML structure to display the stopwatch, utilizing JavaScript functions to manage the timing logic, and employing event listeners to handle user inputs for starting, stopping, and resetting the stopwatch. By leveraging the built-in `setInterval` function, developers can effectively update the display at regular intervals, providing a real-time experience for users.
Key takeaways from this discussion highlight the importance of clear code organization and user experience considerations. Implementing features such as lap time recording and pause functionality can enhance the stopwatch’s usability. Additionally, using CSS for styling can significantly improve the visual appeal of the stopwatch, making it more engaging for users. Understanding how to manipulate time and user interactions in JavaScript is crucial for building effective and responsive applications.
Overall, the creation of a stopwatch serves as an excellent exercise for honing JavaScript skills, particularly in areas such as DOM manipulation, event handling, and asynchronous programming. By following best practices and continually refining the code, developers can create a robust and functional stopwatch that meets user needs and expectations.
Author Profile
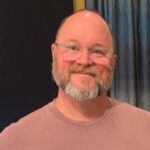
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?